Window with JavaFX buttons:
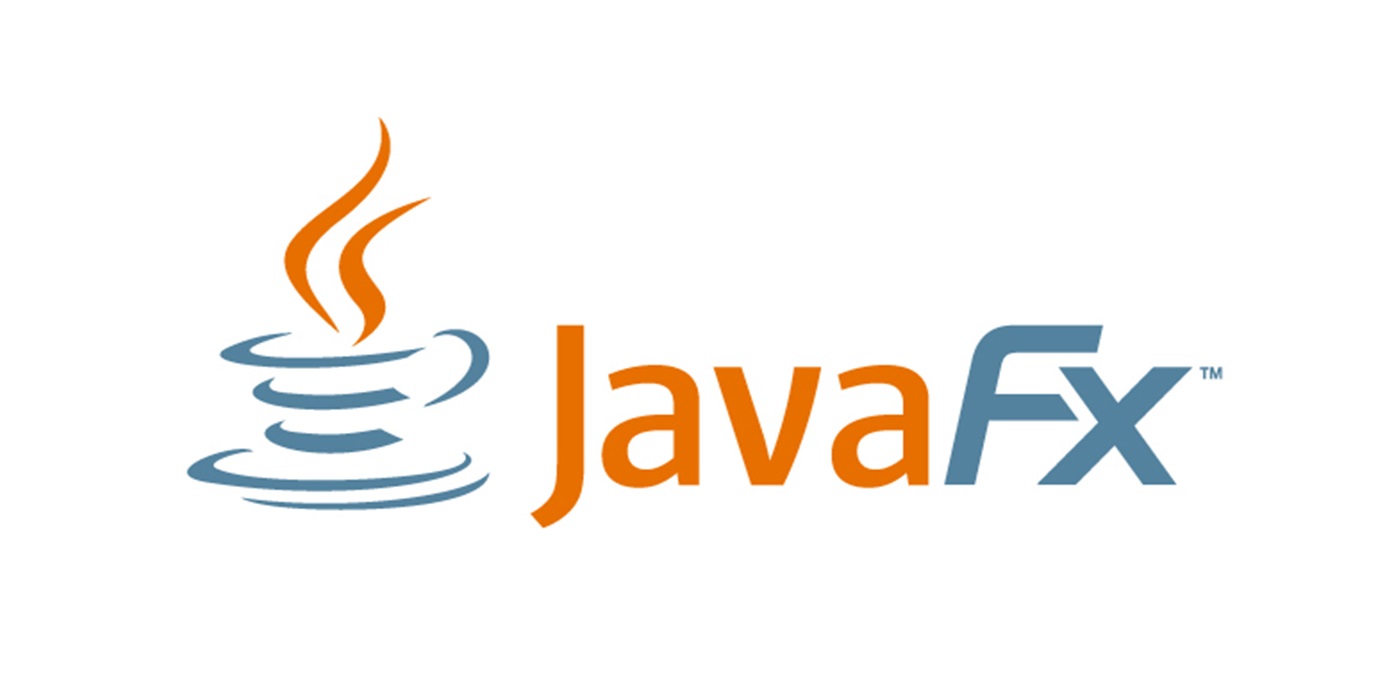
My knowledge in creating a graphical interface was until recently zero. Therefore, it was decided to slightly browse the Internet and create some window with some buttons that, when clicked, will cause something to happen. Perhaps in this text someone will find answers to questions that have arisen once; At the same time, I hope for serious and competent criticism.
What will happen?
Since my goal is to deal with JavaFX, we need to come up with some function at our window. The idea is this: create a set of people (let's assume that they work in a company), for each of which the name, age, salary and marital status will be known. Our window will, firstly, display information about each of them, secondly, change the salary of any employee, thirdly, be able to add a new employee, and finally, quadruplicate, will be able to filter employees depending on the values of their attributes. The result should be something like this:

Getting started
First of all, we create the main class Company, where the application will be launched from:
package company;
publicclassCompany{
publicstaticvoidmain(String[] args){
}
}
In this class, we will extend the capabilities of the Application class. From it we mainly need the start () method, in which the main action will take place (we will skip errors that occur in this method; in other words, if something goes wrong, our window will not close, but an error will appear in the console). :
package company;
import javafx.application.Application;
import javafx.stage.Stage;
publicclassCompanyextendsApplication{
publicvoidstart(Stage primaryStage)throws Exception {
}
publicstaticvoidmain(String[] args){
launch(args);
}
}
Fine. In order to work with representatives of employees of the company, we will create a class User: objects of this class and will act as employees.
Class user
package company;
publicclassUser{
User(String name, int age, int salary, boolean married) {
this.name = name;
this.age = age;
this.salary = salary;
this.married = married;
}
publicvoidchangeSalary(int x){
salary = (salary + x <= 0)? 0: salary + x;
}
public String toString(){
return name;
}
public String getAge(){
return String.valueOf(age);
}
public String getSalary(){
return String.valueOf(salary);
}
public String getMarried(){
return (married)? ("married"):("single");
}
String name;
int age;
int salary;
boolean married;
}
Create
So far, when you start the application, nothing happens. To appear at least a window, you must add it. This will do.
package company;
import javafx.application.Application;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.stage.Stage;
publicclassCompanyextendsApplication{
publicvoidstart(Stage primaryStage)throws Exception {
Scene scene = new Scene(root, WIDTH, HEIGHT);
primaryStage.setTitle("Company");
primaryStage.setScene(scene);
primaryStage.show();
}
publicstaticvoidmain(String[] args){
launch(args);
}
Group root = new Group();
finalprivateint WIDTH = 1000;
finalprivateint HEIGHT = 600;
}
What we just did: we added a scene size
Add a drop-down list with our employees. Create an HBox, in which we put our ComboBox drop-down list of the UserBox and the button that will display information about the employee (we will place the HBox in the VBox):
package company;
import java.util.ArrayList;
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.control.ComboBox;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.scene.text.Text;
import javafx.stage.Stage;
publicclassCompanyextendsApplication{
publicvoidstart(Stage primaryStage)throws Exception {
users.add(new User("Alice", 20, 150, false));
users.add(new User("Bob", 34, 300, true));
users.add(new User("Peter", 18, 100, false));
users.add(new User("Kate", 38, 300, true));
users.add(new User("Steve", 31, 250, true));
users.add(new User("Alan", 62, 500, true));
users.add(new User("Julia", 33, 320, true));
users.add(new User("Patric", 37, 300, true));
users.add(new User("Alexander", 34, 280, true));
users.add(new User("George", 28, 180, true));
users.add(new User("Mary", 22, 190, false));
userBox.getItems().addAll(users);
root.getChildren().add(strings);
strings.setPadding(new Insets(10, 30, 10, 30));
strings.setSpacing(20);
strings.getChildren().add(new Text("Select the user"));
strings.getChildren().add(buttonBox);
buttonBox.setSpacing(10);
buttonBox.getChildren().add(userBox);
Scene scene = new Scene(root, WIDTH, HEIGHT);
primaryStage.setTitle("Company");
primaryStage.setScene(scene);
primaryStage.show();
}
publicstaticvoidmain(String[] args){
launch(args);
}
Group root = new Group();
VBox strings = new VBox();
HBox buttonBox = new HBox();
ComboBox<User> userBox = new ComboBox<>();
finalprivateint WIDTH = 1000;
finalprivateint HEIGHT = 600;
private ArrayList<User> users = new ArrayList<>();
}

What are VBox, HBox and ComboBox
HBox is a set of buttons, texts, input fields, each object of which is located successively horizontally. Similar to it, the VBox is the same, but stores its objects (children) vertically. We will use the following scheme: we will create a VBox in which we will put several HBoxes, in each of which we will put a sequence of buttons and fields.
ComboBox is just a dropdown list itself.
HBox is a set of buttons, texts, input fields, each object of which is located successively horizontally. Similar to it, the VBox is the same, but stores its objects (children) vertically. We will use the following scheme: we will create a VBox in which we will put several HBoxes, in each of which we will put a sequence of buttons and fields.
ComboBox is just a dropdown list itself.
Now we want to create a button that will display information about the selected employee. Add the button itself and the text field (TextField) to which the text will be displayed. These two objects will be added to the HBox:
package company;
/* */publicclassCompanyextendsApplication{
publicvoidstart(Stage primaryStage)throws Exception {
/* */
buttonBox.setSpacing(10);
buttonBox.getChildren().add(userBox);
buttonBox.getChildren().add(buttonGetInfo);
buttonBox.getChildren().add(textInfo);
/* */
}
/* */
Button buttonGetInfo = new Button("Info");
Text textInfo = new Text();
/* */
}

But while this button does nothing. In order for her to do something, she needs to assign an action:
buttonGetInfo.setOnAction(new EventHandler<ActionEvent>() {
@Overridepublicvoidhandle(ActionEvent e){
User u = (User) userBox.getSelectionModel().getSelectedItem();
if (u != null) {
textInfo.setText("Age is " + u.getAge() + ", " +
"Salary is " + u.getSalary() + ", " +
"Relationship: " + u.getMarried());
} else {
textInfo.setText("User not selected");
}
}
});

Next steps
Absolutely the same way we add two more HBoxes: in the second one there will be a change in salary, in the third one - adding a new employee. Due to the fact that the logic here is absolutely the same, let us skip the explanations of these moments and immediately show the result:

Code
package company;
import java.util.ArrayList;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ComboBox;
import javafx.scene.control.TextField;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.scene.text.Text;
import javafx.stage.Stage;
publicclassCompanyextendsApplication{
publicvoidstart(Stage primaryStage)throws Exception {
users.add(new User("Alice", 20, 150, false));
users.add(new User("Bob", 34, 300, true));
users.add(new User("Peter", 18, 100, false));
users.add(new User("Kate", 38, 300, true));
users.add(new User("Steve", 31, 250, true));
users.add(new User("Alan", 62, 500, true));
users.add(new User("Julia", 33, 320, true));
users.add(new User("Patric", 37, 300, true));
users.add(new User("Alexander", 34, 280, true));
users.add(new User("George", 28, 180, true));
users.add(new User("Mary", 22, 190, false));
userBox.getItems().addAll(users);
root.getChildren().add(strings);
strings.setPadding(new Insets(10, 30, 10, 30));
strings.setSpacing(20);
strings.getChildren().add(new Text("Select the user"));
strings.getChildren().add(buttonBox);
strings.getChildren().add(new Text("Change the salary"));
strings.getChildren().add(changeSalaryBox);
strings.getChildren().add(new Text("Add new User"));
strings.getChildren().add(addUserBox);
buttonBox.setSpacing(10);
buttonBox.getChildren().add(userBox);
buttonBox.getChildren().add(buttonGetInfo);
buttonBox.getChildren().add(textInfo);
changeSalaryBox.setSpacing(10);
changeSalaryBox.getChildren().add(buttonChangeSalary);
changeSalaryBox.getChildren().add(howMuchChange);
addUserBox.setSpacing(10);
addUserBox.getChildren().add(new Text("Name: "));
addUserBox.getChildren().add(name);
addUserBox.getChildren().add(new Text("Age: "));
addUserBox.getChildren().add(age);
addUserBox.getChildren().add(new Text("Salary: "));
addUserBox.getChildren().add(salary);
addUserBox.getChildren().add(new Text("Married: "));
addUserBox.getChildren().add(married);
addUserBox.getChildren().add(buttonAddUser);
buttonGetInfo.setOnAction(new EventHandler<ActionEvent>() {
@Overridepublicvoidhandle(ActionEvent e){
User u = (User) userBox.getSelectionModel().getSelectedItem();
if (u != null) {
textInfo.setText("Age is " + u.getAge() + ", " +
"Salary is " + u.getSalary() + ", " +
"Relationship: " + u.getMarried());
} else {
textInfo.setText("User not selected");
}
}
});
buttonChangeSalary.setOnAction(new EventHandler<ActionEvent>() {
@Overridepublicvoidhandle(ActionEvent e){
User u = (User) userBox.getSelectionModel().getSelectedItem();
if(u != null) {
u.changeSalary(Integer.parseInt(howMuchChange.getText()));
textInfo.setText("Age is " + u.getAge() + ", " +
"Salary is " + u.getSalary() + ", " +
"Relationshp: " + u.getMarried());
howMuchChange.clear();
}
}
});
buttonAddUser.setOnAction(new EventHandler<ActionEvent>() {
@Overridepublicvoidhandle(ActionEvent e){
String m = married.getText();
boolean mm = (m.equals("married"))? true:false;
User u = new User(name.getText(), Integer.parseInt(age.getText()),
Integer.parseInt(salary.getText()), mm);
users.add(u);
userBox.getItems().addAll(u);
name.clear();
age.clear();
salary.clear();
married.clear();
}
});
Scene scene = new Scene(root, WIDTH, HEIGHT);
primaryStage.setTitle("Company");
primaryStage.setScene(scene);
primaryStage.show();
}
publicstaticvoidmain(String[] args){
launch(args);
}
Group root = new Group();
VBox strings = new VBox();
HBox buttonBox = new HBox();
ComboBox<User> userBox = new ComboBox<>();
Button buttonGetInfo = new Button("Info");
Text textInfo = new Text();
HBox changeSalaryBox = new HBox();
Button buttonChangeSalary = new Button("Change salary");
TextField howMuchChange = new TextField();
HBox addUserBox = new HBox();
Button buttonAddUser = new Button("Add User");
TextField name = new TextField();
TextField age = new TextField();
TextField salary = new TextField();
TextField married = new TextField();
finalprivateint WIDTH = 1000;
finalprivateint HEIGHT = 600;
private ArrayList<User> users = new ArrayList<>();
}
Filters
Now add the "filtering" of employees by feature. Again, the lists for filters will be added using the ComboBox using the same logic.
package company;
/* */publicclassCompanyextendsApplication{
publicvoidstart(Stage primaryStage)throws Exception {
/* */
ageFilterBox.getItems().addAll(
"no matter",
"over 20",
"over 30",
"over 40"
);
salaryFilterBox.getItems().addAll(
"no matter",
"over 150",
"over 250",
"over 500"
);
relationshipFilterBox.getItems().addAll(
"no matter",
"married",
"single"
);
/* */
strings.getChildren().add(filters);
strings.getChildren().add(resultFilter);
/* */
filters.setSpacing(10);
filters.getChildren().add(new Text("Age"));
filters.getChildren().add(ageFilterBox);
filters.getChildren().add(new Text("Salary"));
filters.getChildren().add(salaryFilterBox);
filters.getChildren().add(new Text("Relationship"));
filters.getChildren().add(relationshipFilterBox);
filters.getChildren().add(filter);
/* */
filter.setOnAction(new EventHandler<ActionEvent>() {
@Overridepublicvoidhandle(ActionEvent e){
int age;
int index = ageFilterBox.getSelectionModel().getSelectedIndex();
age = (index == 0)? 0: (index == 1)? 21: (index == 2)? 31: 41;
int salary;
index = salaryFilterBox.getSelectionModel().getSelectedIndex();
salary = (index == 0)? 0 : (index == 1)? 151 : (index == 2)? 251:501;
boolean relate;
index = relationshipFilterBox.getSelectionModel().getSelectedIndex();
relate = (index == 1)? true: (index == 2)? false: true;
List<User> list;
if(index != 0) { list = users.stream().
filter(u -> u.age > age).
filter(u -> u.salary > salary).
filter(u -> u.married == relate).
collect(Collectors.toList()); }
else { list = users.stream().
filter(u -> u.age > age).
filter(u -> u.salary > salary).
collect(Collectors.toList()); }
String res = "";
for(User u: list) {
res += u.toString() + ", ";
}
resultFilter.setText(res);
}
});
/* */
}
/* */
HBox filters = new HBox();
ComboBox<String> ageFilterBox = new ComboBox<>();
ComboBox<String> salaryFilterBox = new ComboBox<>();
ComboBox<String> relationshipFilterBox = new ComboBox<>();
Button filter = new Button("filter");
Text resultFilter = new Text();
/* */
}

Done!
Full code
Company class (main)
Class user
package company;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
import javafx.application.Application;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ComboBox;
import javafx.scene.control.TextField;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.scene.text.Text;
import javafx.stage.Stage;
publicclassCompanyextendsApplication{
@Overridepublicvoidstart(Stage primaryStage)throws Exception {
users.add(new User("Alice", 20, 150, false));
users.add(new User("Bob", 34, 300, true));
users.add(new User("Peter", 18, 100, false));
users.add(new User("Kate", 38, 300, true));
users.add(new User("Steve", 31, 250, true));
users.add(new User("Alan", 62, 500, true));
users.add(new User("Julia", 33, 320, true));
users.add(new User("Patric", 37, 300, true));
users.add(new User("Alexander", 34, 280, true));
users.add(new User("George", 28, 180, true));
users.add(new User("Mary", 22, 190, false));
userBox.getItems().addAll(users);
ageFilterBox.getItems().addAll(
"no matter",
"over 20",
"over 30",
"over 40"
);
salaryFilterBox.getItems().addAll(
"no matter",
"over 150",
"over 250",
"over 500"
);
relationshipFilterBox.getItems().addAll(
"no matter",
"married",
"single"
);
root.getChildren().add(strings);
strings.setPadding(new Insets(10, 30, 10, 30));
strings.setSpacing(20);
strings.getChildren().add(new Text("Select the user"));
strings.getChildren().add(buttonBox);
strings.getChildren().add(new Text("Change the salary"));
strings.getChildren().add(changeSalaryBox);
strings.getChildren().add(new Text("Add new User"));
strings.getChildren().add(addUserBox);
strings.getChildren().add(filters);
strings.getChildren().add(resultFilter);
buttonBox.setSpacing(10);
buttonBox.getChildren().add(userBox);
buttonBox.getChildren().add(buttonGetInfo);
buttonBox.getChildren().add(textInfo);
changeSalaryBox.setSpacing(10);
changeSalaryBox.getChildren().add(buttonChangeSalary);
changeSalaryBox.getChildren().add(howMuchChange);
addUserBox.setSpacing(10);
addUserBox.getChildren().add(new Text("Name: "));
addUserBox.getChildren().add(name);
addUserBox.getChildren().add(new Text("Age: "));
addUserBox.getChildren().add(age);
addUserBox.getChildren().add(new Text("Salary: "));
addUserBox.getChildren().add(salary);
addUserBox.getChildren().add(new Text("Married: "));
addUserBox.getChildren().add(married);
addUserBox.getChildren().add(buttonAddUser);
filters.setSpacing(10);
filters.getChildren().add(new Text("Age"));
filters.getChildren().add(ageFilterBox);
filters.getChildren().add(new Text("Salary"));
filters.getChildren().add(salaryFilterBox);
filters.getChildren().add(new Text("Relationship"));
filters.getChildren().add(relationshipFilterBox);
filters.getChildren().add(filter);
Scene scene = new Scene(root, WIDTH, HEIGHT);
primaryStage.setTitle("Company");
primaryStage.setScene(scene);
primaryStage.show();
buttonGetInfo.setOnAction(new EventHandler<ActionEvent>() {
@Overridepublicvoidhandle(ActionEvent e){
User u = (User) userBox.getSelectionModel().getSelectedItem();
if (u != null) {
textInfo.setText("Age is " + u.getAge() + ", " +
"Salary is " + u.getSalary() + ", " +
"Relationship: " + u.getMarried());
} else {
textInfo.setText("User not selected");
}
}
});
buttonChangeSalary.setOnAction(new EventHandler<ActionEvent>() {
@Overridepublicvoidhandle(ActionEvent e){
User u = (User) userBox.getSelectionModel().getSelectedItem();
if(u != null) {
u.changeSalary(Integer.parseInt(howMuchChange.getText()));
textInfo.setText("Age is " + u.getAge() + ", " +
"Salary is " + u.getSalary() + ", " +
"Relationshp: " + u.getMarried());
howMuchChange.clear();
}
}
});
buttonAddUser.setOnAction(new EventHandler<ActionEvent>() {
@Overridepublicvoidhandle(ActionEvent e){
String m = married.getText();
boolean mm = (m.equals("married"))? true:false;
User u = new User(name.getText(), Integer.parseInt(age.getText()),
Integer.parseInt(salary.getText()), mm);
users.add(u);
userBox.getItems().addAll(u);
name.clear();
age.clear();
salary.clear();
married.clear();
}
});
filter.setOnAction(new EventHandler<ActionEvent>() {
@Overridepublicvoidhandle(ActionEvent e){
int age;
int index = ageFilterBox.getSelectionModel().getSelectedIndex();
age = (index == 0)? 0: (index == 1)? 21: (index == 2)? 31: 41;
int salary;
index = salaryFilterBox.getSelectionModel().getSelectedIndex();
salary = (index == 0)? 0 : (index == 1)? 151 : (index == 2)? 251:501;
boolean relate;
index = relationshipFilterBox.getSelectionModel().getSelectedIndex();
relate = (index == 1)? true: (index == 2)? false: true;
//resultFilter.setText(String.valueOf(age) + " " + String.valueOf(salary) + " "+ relate);
List<User> list;
if(index != 0) { list = users.stream().
filter(u -> u.age > age).
filter(u -> u.salary > salary).
filter(u -> u.married == relate).
collect(Collectors.toList()); }
else { list = users.stream().
filter(u -> u.age > age).
filter(u -> u.salary > salary).
collect(Collectors.toList()); }
String res = "";
for(User u: list) {
res += u.toString() + ", ";
}
resultFilter.setText(res);
}
});
}
publicstaticvoidmain(String[] args){
launch(args);
}
Group root = new Group();
VBox strings = new VBox();
HBox buttonBox = new HBox();
HBox changeSalaryBox = new HBox();
HBox addUserBox = new HBox();
HBox filters = new HBox();
ComboBox<User> userBox = new ComboBox<>();
ComboBox<String> ageFilterBox = new ComboBox<>();
ComboBox<String> salaryFilterBox = new ComboBox<>();
ComboBox<String> relationshipFilterBox = new ComboBox<>();
finalprivateint WIDTH = 1000;
finalprivateint HEIGHT = 600;
private ArrayList<User> users = new ArrayList<>();
Button buttonGetInfo = new Button("Info");
Text textInfo = new Text();
Button buttonChangeSalary = new Button("Change salary");
TextField howMuchChange = new TextField();
Button buttonAddUser = new Button("Add User");
TextField name = new TextField();
TextField age = new TextField();
TextField salary = new TextField();
TextField married = new TextField();
Button filter = new Button("filter");
Text resultFilter = new Text();
}
Class user
package company;
publicclassUser{
User(String name, int age, int salary, boolean married) {
this.name = name;
this.age = age;
this.salary = salary;
this.married = married;
}
publicvoidchangeSalary(int x){
salary = (salary + x <= 0)? 0: salary + x;
}
public String toString(){
return name;
}
public String getAge(){
return String.valueOf(age);
}
public String getSalary(){
return String.valueOf(salary);
}
public String getMarried(){
return (married)? ("married"):("single");
}
String name;
int age;
int salary;
boolean married;
}
What was it
The program itself does not represent any utility and was written solely in order to get acquainted with JavaFX and its tools. In some places, for example, when salary changes, options are not taken into account when a user is not selected or when a line is entered: in these cases an error will appear. The same applies to the fields where information is entered when adding new employees. And, finally, an error will occur if no value is selected in any of the drop-down lists. If desired, everyone can add their own “bypass” of these moments.
When writing filters, by the way, I used the notorious StreamAPI and
Sources:
- JavaFX 8 - Oracle Help Center
- A little bit about the creation and historical modifications of JavaFX
- Wonderful article vedenin1980 about StreamAPI and
expressions