
Introduction to the development of WinRT-applications in HTML / JavaScript. Application styling
- Tutorial
This article continues the series of materials (the first part ) devoted to the basics of developing WinRT-applications in HTML / JS for Windows 8. We will successively go from the starting almost empty template to a full-fledged application with the server part and live tiles.
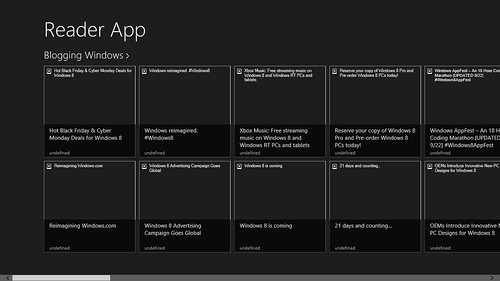
Let me remind you that in the first article we learned how to add our own data sources and settled on displaying this data in raw form on the application pages.
In this article, we will look at the appearance of our application: add pictures, change headers, styles, tiles, etc. All work can be done in Visual Studio, although some things are more convenient and easier to change in Expression Blend.
First of all, let's try to extract images from the posts, if they are, of course, there. To do this, open the js \ data.js file and go to the getItemsFromRSSFeed function , which parses individual posts.
Add the following code before the line “var postItem = {” :
Here we create a temporary element with the content of the post and extract ( querySelector ) the first picture from it to use it as a background on the tiles for the posts. If there is no picture, use an empty string, which we will pass into the corresponding CSS rule.
Add the following lines at the end to the post description object ( postItem ), remembering to add a comma with the line above:
It should look something like this:
If you now run the project for debugging, you will see that visually nothing has changed.
Open the pages \ groupedItems \ groupedItems.html file . Inside this file, templates are used to describe page structure and data binding. Find the element template starting with the line:
In the two lines below you will see a description of the picture:
If you are familiar with the basics of HTML, you probably already guessed why nothing has changed in the display: the format we used above is designed to be used in CSS, and not an explicit link to the image via img.
Delete this line to insert an image.
Go up one level and replace the line
to the following:
Here we use the capabilities of data binding ( data-win-bind attributes ) with a template for projecting properties in data onto attributes in html markup and DOM elements.
Try to run the application for debugging: It got better, but still not very good. For example, as we expected, in some posts there are no images - and instead of them we got a lack of pictures or blank stubs. Let's put a colored background for such posts. To do this, open the groupedItems.css file in the same folder . Find the following line describing the display of an individual item:

Add a background description at the end of this CSS rule:
Additionally, you can do a few more manipulations on the background image, for example, center it, cancel repetitions and set the zoom mode:
Launching the application: Now let's move on to fixing the display of text content.

Return to the groupedItems.html file and the item template description ( itemtemplate ). At the end of the element you will find a description of the subtitle ( item-subtitle ). In our case, there is no subtitle, but there is a date, so replace this line:
to the following:
If you try to run now, you will see that the date has moved somewhere and climbed onto the header.
To fix this, go back to the CSS file. Find the subtitle description:
Replace it with the appropriate date display description:
In this case, we also added right-alignment and text display in upper case.
Now let's try to make the title of the record larger and change its position on the tile.
First you need to move it to a higher level. Go to the groupedItems.html file and inside the element template, take out the header description one level higher by adding a wrapper. It should look something like this:
Return to the CSS-file, in it you need to display the hierarchy change and register updated styles.
After the rule .groupeditemspage .groupeditemslist .item {...} add a new one:
Immediately after it, transfer the description of the header below ( .item-overlay should be replaced by .item-title-container ):
Try to start the project: To remove the backing left from the original layout, go down the code in the CSS file to the lines:

Replace the specified color with transparent .
Try to increase the size of the text on the date yourself and change the font to “Segoe UI Semibold” and the application name to another: As your homework, try changing the appearance of the tiles so that they look like this (hint: gradients will help you): Go inside the group and inside a separate blog. Similar actions to change the appearance must be done for all internal pages: Put the application in Snapped mode (if you work in Expression Blend, you can change the display mode on the Device tab). Here you also need to adjust the styles taking into account the chosen style. Styles for Snap mode are adjusted using Media Queries:





The finished project at the current stage can be downloaded here: http://aka.ms/win8jsreadertemplate_v1 .
In the next part, we will improve data handling and add support for several contracts.
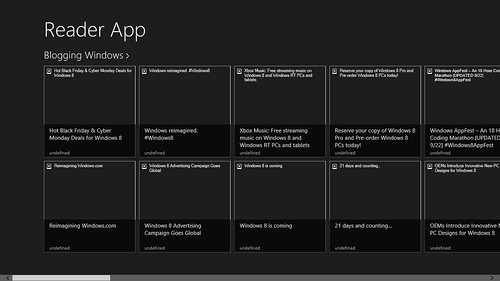
Let me remind you that in the first article we learned how to add our own data sources and settled on displaying this data in raw form on the application pages.
In this article, we will look at the appearance of our application: add pictures, change headers, styles, tiles, etc. All work can be done in Visual Studio, although some things are more convenient and easier to change in Expression Blend.
XAML / C #. If you are interested in developing using XAML and C #, I recommend paying attention to a similar series of articles by my colleague, Stas Pavlov: We deal with the development of Windows 8 applications on XAML / C #, implementing a simple RSS Reader .
Extract Pictures
First of all, let's try to extract images from the posts, if they are, of course, there. To do this, open the js \ data.js file and go to the getItemsFromRSSFeed function , which parses individual posts.
Add the following code before the line “var postItem = {” :
// извлечение ссылки на изображение в посте
var tempElement = document.createElement("div");
tempElement.innerHTML = postContent;
var image = tempElement.querySelector("img");
var imglink = (image != null) ? "url('" + image.src + "')" : "";
Here we create a temporary element with the content of the post and extract ( querySelector ) the first picture from it to use it as a background on the tiles for the posts. If there is no picture, use an empty string, which we will pass into the corresponding CSS rule.
Note : here we can also get into a situation where there is no explicit picture in the post, but there is an invisible one used to count the number of views. This is exactly the situation for the blogs used during the article, however, these images are transparent, so in our case this is not critical.
Add the following lines at the end to the post description object ( postItem ), remembering to add a comma with the line above:
// ссылка на картинку
backgroundImage: imglink
It should look something like this:
var postItem = {
…
link: post.querySelector("link").textContent,
// ссылка на картинку
backgroundImage: imglink
};
If you now run the project for debugging, you will see that visually nothing has changed.
Open the pages \ groupedItems \ groupedItems.html file . Inside this file, templates are used to describe page structure and data binding. Find the element template starting with the line:
In the two lines below you will see a description of the picture:

If you are familiar with the basics of HTML, you probably already guessed why nothing has changed in the display: the format we used above is designed to be used in CSS, and not an explicit link to the image via img.
Note : one of the reasons why CSS is preferable in this case is the more flexible display settings and, in particular, the possibility of manipulating the image, including maintaining the aspect ratio.
Delete this line to insert an image.
Go up one level and replace the line
to the following:
Here we use the capabilities of data binding ( data-win-bind attributes ) with a template for projecting properties in data onto attributes in html markup and DOM elements.
Try to run the application for debugging: It got better, but still not very good. For example, as we expected, in some posts there are no images - and instead of them we got a lack of pictures or blank stubs. Let's put a colored background for such posts. To do this, open the groupedItems.css file in the same folder . Find the following line describing the display of an individual item:

.groupeditemspage .groupeditemslist .item {
Add a background description at the end of this CSS rule:
background-color: rgb(0, 204, 255);
Additionally, you can do a few more manipulations on the background image, for example, center it, cancel repetitions and set the zoom mode:
background-position: 50% 50%;
background-repeat: no-repeat;
background-size: cover;
Launching the application: Now let's move on to fixing the display of text content.

Updating Text Styles
Return to the groupedItems.html file and the item template description ( itemtemplate ). At the end of the element you will find a description of the subtitle ( item-subtitle ). In our case, there is no subtitle, but there is a date, so replace this line:
to the following:
If you try to run now, you will see that the date has moved somewhere and climbed onto the header.
To fix this, go back to the CSS file. Find the subtitle description:
.groupeditemspage .groupeditemslist .item .item-overlay .item-subtitle {
-ms-grid-row: 2;
width: 220px;
}
Replace it with the appropriate date display description:
.groupeditemspage .groupeditemslist .item .item-overlay .item-date {
-ms-grid-row: 2;
width: 220px;
text-transform: uppercase;
text-align:right;
}
In this case, we also added right-alignment and text display in upper case.
Note : note that it uses the CSS 3 Grid Layout module to position elements. We will not dwell on the details of its use, but if you plan to create applications for the Windows Store in HTML / JS, we recommend that you study its capabilities well.
Now let's try to make the title of the record larger and change its position on the tile.
First you need to move it to a higher level. Go to the groupedItems.html file and inside the element template, take out the header description one level higher by adding a wrapper. It should look something like this:
Return to the CSS-file, in it you need to display the hierarchy change and register updated styles.
After the rule .groupeditemspage .groupeditemslist .item {...} add a new one:
.groupeditemspage .groupeditemslist .item .item-title-container {
-ms-grid-row: 1;
margin: 10px;
padding: 8px;
opacity: 0.85;
}
Immediately after it, transfer the description of the header below ( .item-overlay should be replaced by .item-title-container ):
.groupeditemspage .groupeditemslist .item .item-title-container .item-title {
overflow: hidden;
width: 220px;
display: inline;
font-size: 1.6em;
line-height: 1.5em;
font-family: 'Segoe UI Light';
background: rgb(145, 0, 145);
box-shadow: rgb(145, 0, 145) 0 0 0 8px;
}
Try to start the project: To remove the backing left from the original layout, go down the code in the CSS file to the lines:

.groupeditemspage .groupeditemslist .item .item-overlay {
background: rgba(0,0,0,0.65);
}
Replace the specified color with transparent .
Try to increase the size of the text on the date yourself and change the font to “Segoe UI Semibold” and the application name to another: As your homework, try changing the appearance of the tiles so that they look like this (hint: gradients will help you): Go inside the group and inside a separate blog. Similar actions to change the appearance must be done for all internal pages: Put the application in Snapped mode (if you work in Expression Blend, you can change the display mode on the Device tab). Here you also need to adjust the styles taking into account the chosen style. Styles for Snap mode are adjusted using Media Queries:





@media screen and (-ms-view-state: snapped) {
...
}
Note: in this project we did not change the theme (the default is dark). The theme is set in the CSS file that is connected from the WinJS library. You will easily find it in the title of each page:
Try changing dark to light to see the difference. Please note that not only the background color has changed, but also the color of the text and other elements. In our case, this can lead to undesirable consequences, since some of the colors used are designed for the dark background color and white color of the main text.
Project
The finished project at the current stage can be downloaded here: http://aka.ms/win8jsreadertemplate_v1 .
Further
In the next part, we will improve data handling and add support for several contracts.