
Android Shortcuts Review
- Tutorial
With the version of Android OS 7.1 (API 25) Google offers us a new mechanism for working with applications - Android Shortcuts . We wrote a short review of this feature, created for the convenience of users.

Translated from English “shortcut” is the shortest way, this name reflects well the main purpose of shortcuts. They allow us to get to some specific point in the application and perform a specific action in just a couple of clicks. Shortcuts look good, for example, if the user needs to:
• select a predefined route in the navigator;
• start a conversation with a friend in the messenger;
• continue the game from the last save point.

A shortcut includes:
1) an intent or an array of intentions that trigger a specific action;
2) the identifier or ID that identifies this particular shortcut;
3) short and long messages displayed when it is displayed;
4) the icon to the left of the text.
To better understand the mechanics of working with shortcuts, consider their classification.
Android offers 2 types of shortcuts (there are actually three, but more on that below): static and dynamic. Next, consider each type of shortcut with a few examples and explanations.
A description of static shortcuts is contained in the
Removing the shortcut or changing its components will not work in any way until the application is updated.
It is recommended to use static shortcuts for those actions that will not change in any way due to user manipulations with the application and will always be available. For example, open a new search query, go to notification settings, start a new conversation. However, using a static shortcut, for example, in order to send the user immediately to the authorization screen is not a good idea. But what if it is already authorized? Then this action does not make sense. In such cases, dynamic shortcuts will come in handy.
They can be added, deleted, changed in real time, that is, depending on certain events. Let us return to the authorization example: if the user is not authorized, then add this shortcut, as soon as it is authorized, delete it.
All interaction with shortcuts programmatically occurs through the ShortcutManager class , available for API version 23, however most of its methods are available only for API version 25. Operations with them are quite simple, but there are some nuances that will be discussed in detail later.
Removing and modifying dynamic shortcuts is also quite simple:
In fact, there is another kind of shortcuts - if translated literally, “pinned” or “pinned” shortcuts. They look like this:
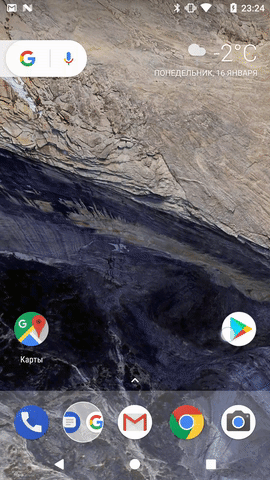
You cannot programmatically create such shortcuts, nor can you delete them. This can only be done by the user. But with the help of the code they can be disabled (
When disabling any shortcut, you can set the corresponding message that will be displayed to the user when you click on it.
We got acquainted with shortcuts and the basic principles of their work as a whole. Now you can move on to the details, features and practical tips.
1. When adding shortcuts in most cases, they will be displayed from the first added to the last, the first added will be closest to the application icon. Static will be displayed first, then dynamic.
2. When creating a shortcut, you must set the long and short messages. A long message is indicated in the list, if it does not fit, then a short one. Google recommends setting a short message to no more than 10 characters, and a long message to no more than 25.
3. Although you can add a maximum of five shortcuts, Google recommends not adding more than four. And in the list more than four shortcuts will not be displayed (the last added one will not appear). Why you need a fifth shortcut is a mystery, because it also does not appear on tablets.
4. The attribute of
5. It is important that Android itself does not regulate the number of added shortcuts. If for some reason you want to add the sixth shortcut, the application will simply crash by throwing it away
6. If you do not set the action attribute to at least one shortcut intent, then in the case of a dynamic shortcut, the application will crash when this shortcut is created, and in the case of a static shortcut, the shortcut will simply not be created. Note that some types cannot be put in extras of shortcut intents, for example
7. If you try to call
8. When adding several intents to a shortcut that are processed by different activities, you can build a certain stack of activities. The lowest in this stack will be the activity that processed the first added intent.
9. If the user is locked on the device, then he will not be able to use shortcuts - they are contained in the encrypted storage.
10. If the user wants to support the application on another device, then only “fixed” shortcuts will be recreated on it. Dynamic and static are not recreated, but static will be redefined during application installation. If you are worried about supporting the application on different devices, and you have not set a flag
11. Android developers also suggest us using the method
Finally, a couple of not yet mentioned tips from Android developers.
1. Update shortcuts (
2. Follow the shortcut design guide . This is a small document that is easy to follow.
Android Shortcuts is a powerful application tool. Serious multi-functional applications should use it to keep up with the times. I hope this guide inspires you to add such a mechanism to your applications and helps you implement it painlessly. You should not use shortcuts in your application if you know that they are needed there just like a fifth wheel cart. Remember the relevance, because shortcuts are not added to expand the functionality, but for the convenience of the user.

general review
Translated from English “shortcut” is the shortest way, this name reflects well the main purpose of shortcuts. They allow us to get to some specific point in the application and perform a specific action in just a couple of clicks. Shortcuts look good, for example, if the user needs to:
• select a predefined route in the navigator;
• start a conversation with a friend in the messenger;
• continue the game from the last save point.

Main components
A shortcut includes:
1) an intent or an array of intentions that trigger a specific action;
2) the identifier or ID that identifies this particular shortcut;
3) short and long messages displayed when it is displayed;
4) the icon to the left of the text.
To better understand the mechanics of working with shortcuts, consider their classification.
Types of Shortcuts
Android offers 2 types of shortcuts (there are actually three, but more on that below): static and dynamic. Next, consider each type of shortcut with a few examples and explanations.
Static Shortcuts
A description of static shortcuts is contained in the
xml
.file. They are created once, they cannot be deleted, added and changed without updating the application, but they are quite simple to create.Creation example
File
And a couple of lines in
shortcuts.xml
contained in res/xml/
:
And a couple of lines in
AndroidManifest.xml
:
Removing the shortcut or changing its components will not work in any way until the application is updated.
It is recommended to use static shortcuts for those actions that will not change in any way due to user manipulations with the application and will always be available. For example, open a new search query, go to notification settings, start a new conversation. However, using a static shortcut, for example, in order to send the user immediately to the authorization screen is not a good idea. But what if it is already authorized? Then this action does not make sense. In such cases, dynamic shortcuts will come in handy.
Dynamic shortcuts
They can be added, deleted, changed in real time, that is, depending on certain events. Let us return to the authorization example: if the user is not authorized, then add this shortcut, as soon as it is authorized, delete it.
All interaction with shortcuts programmatically occurs through the ShortcutManager class , available for API version 23, however most of its methods are available only for API version 25. Operations with them are quite simple, but there are some nuances that will be discussed in detail later.
Creation example
ShortcutManager shortcutManager = getSystemService(ShortcutManager.class);
ShortcutInfo shortcut = new ShortcutInfo.Builder(this, "id1")
.setShortLabel("Search")
.setLongLabel("Start new search")
.setIcon(Icon.createWithResource(context, R.drawable.shortcut_search))
.setIntent(new Intent(Intent.ACTION_VIEW,
Uri.parse("shortcutapp://search")))
.build();
shortcutManager.setDynamicShortcuts(Collections.singletonList(shortcut));
Removing and modifying dynamic shortcuts is also quite simple:
Delete
ShortcutManager shortcutManager = getSystemService(ShortcutManager.class);
shortcutManager.removeDynamicShortcuts(Arrays.asList("id1", "id2"));
Change
ShortcutManager shortcutManager = getSystemService(ShortcutManager.class);
shortcutManager.updateShortcuts(Arrays.asList(shortcut1, shortcut2));
Fixed shortcuts
In fact, there is another kind of shortcuts - if translated literally, “pinned” or “pinned” shortcuts. They look like this:
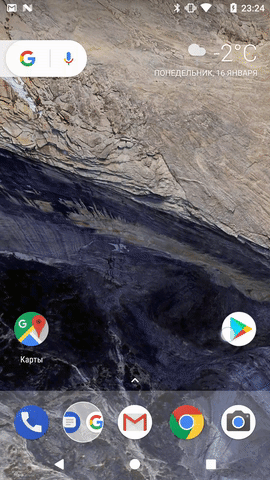
You cannot programmatically create such shortcuts, nor can you delete them. This can only be done by the user. But with the help of the code they can be disabled (
disableShortcuts()
). You can pin both static and dynamic shortcuts.Static case
In the case of a static shortcut, only the OS itself can fix the corresponding fixed one, but only if this static shortcut is absent after updating. If you try to manually disable it, it will pop up
IllegalArgumentException
with a message that static shortcuts cannot be changed dynamically.Dynamic case
If you remove the dynamic shortcut, then the rendered (“fixed”) corresponding shortcut will remain in place, and it can be used. On the one hand, this is a serious advantage that allows you to expand the number of shortcuts, but on the other hand, it is a potential source of bugs, since the removal of a dynamic shortcut can also be associated with the removal of the corresponding functionality for processing the action.
Before deleting a dynamic shortcut, make sure that the pinned one will also be disabled, otherwise it may cause problems in the application.
Before deleting a dynamic shortcut, make sure that the pinned one will also be disabled, otherwise it may cause problems in the application.
When disabling any shortcut, you can set the corresponding message that will be displayed to the user when you click on it.
Example
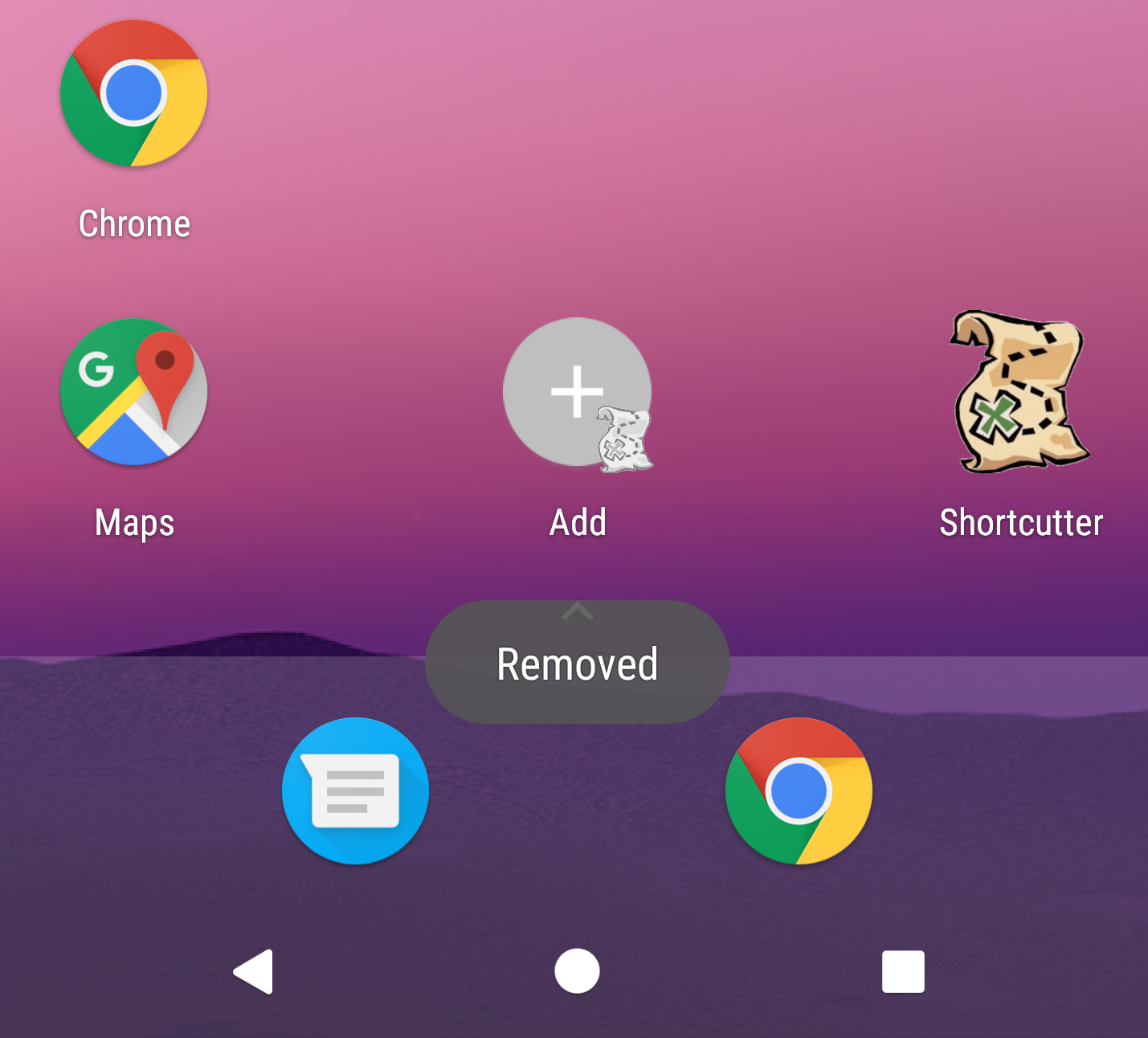
ShortcutManager shortcutManager = getSystemService(ShortcutManager.class);
shortcutManager.disableShortcuts(Collections.singletonList(id), "Removed");
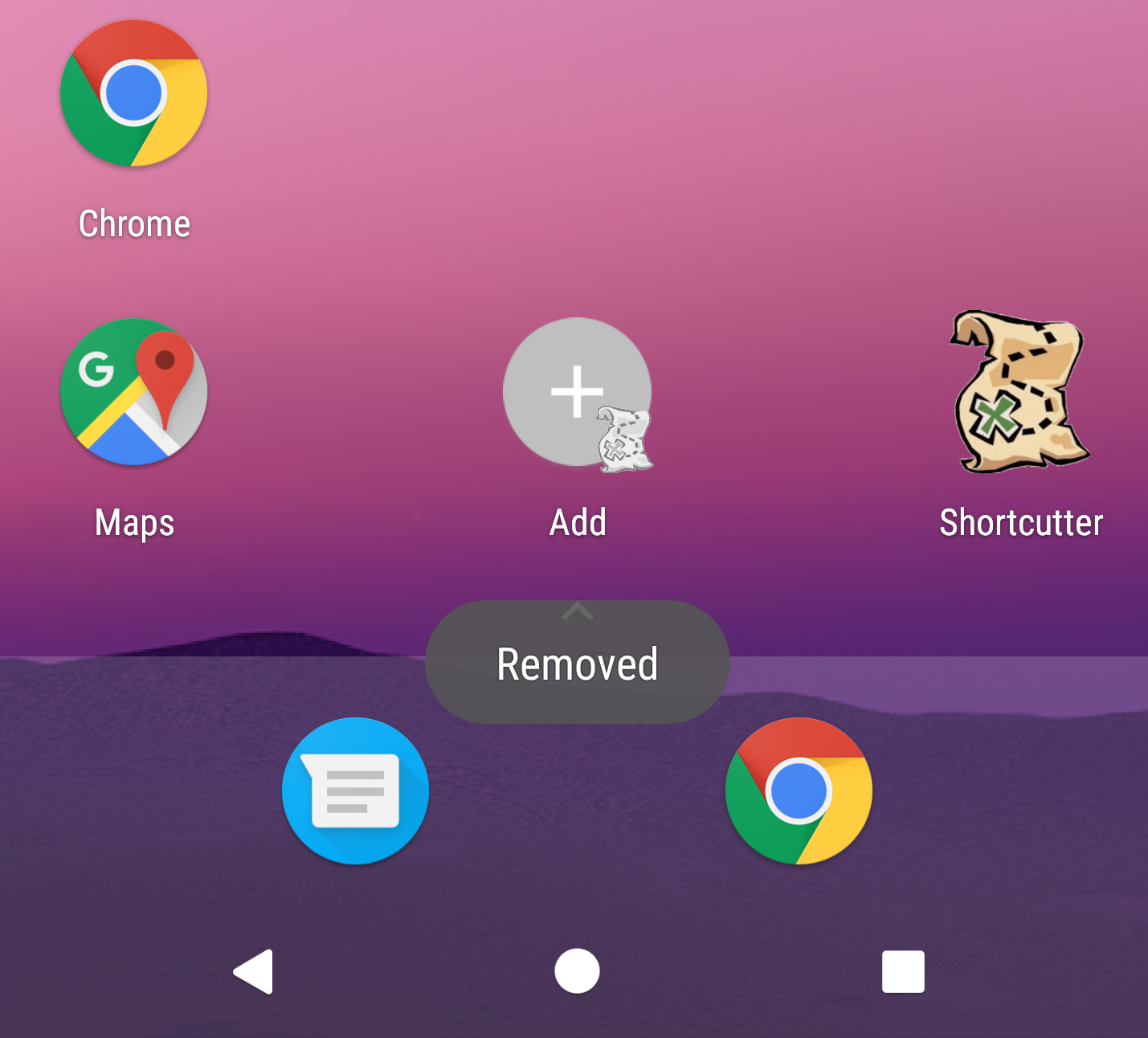
We got acquainted with shortcuts and the basic principles of their work as a whole. Now you can move on to the details, features and practical tips.
Details and subtleties of application
1. When adding shortcuts in most cases, they will be displayed from the first added to the last, the first added will be closest to the application icon. Static will be displayed first, then dynamic.
View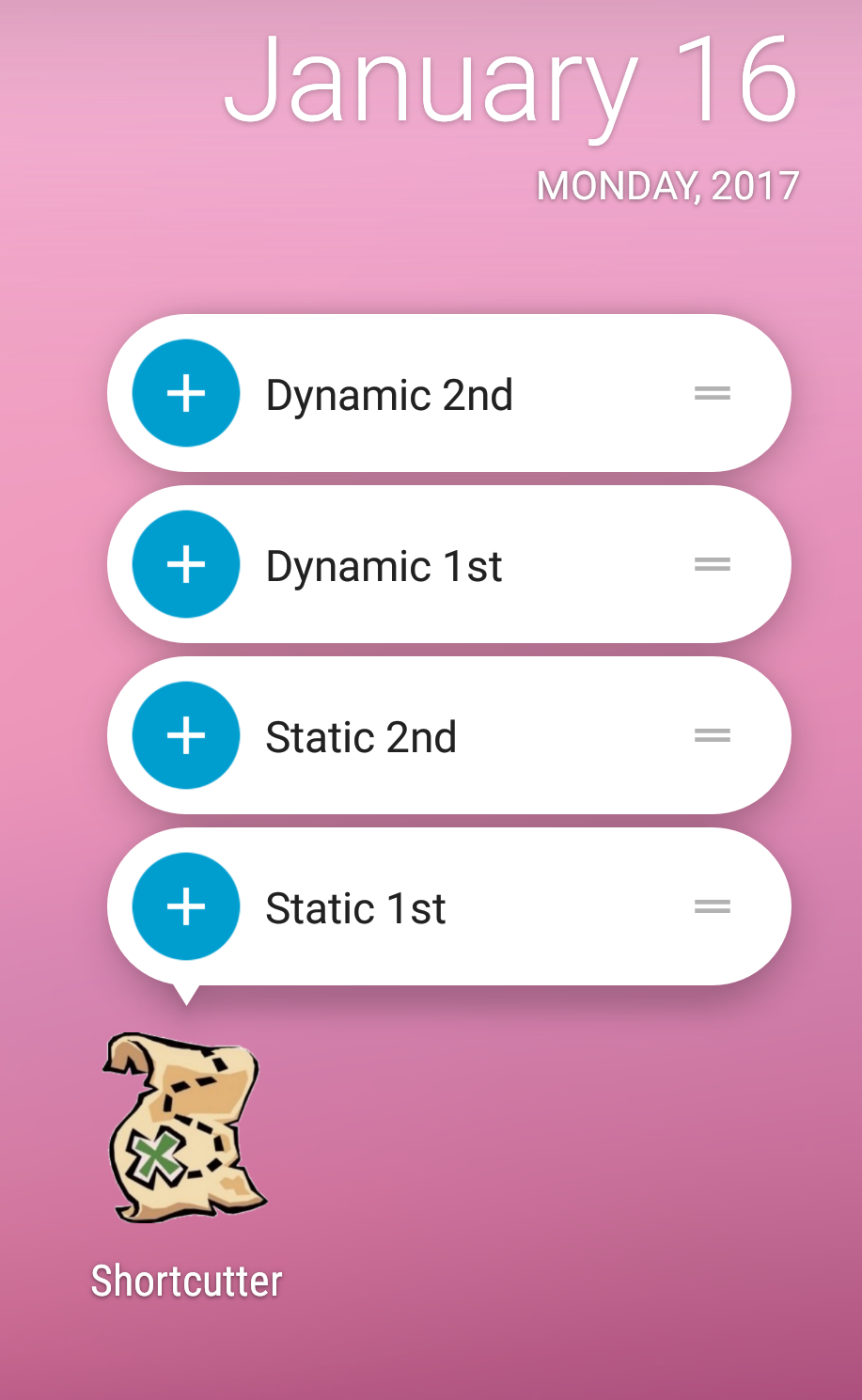

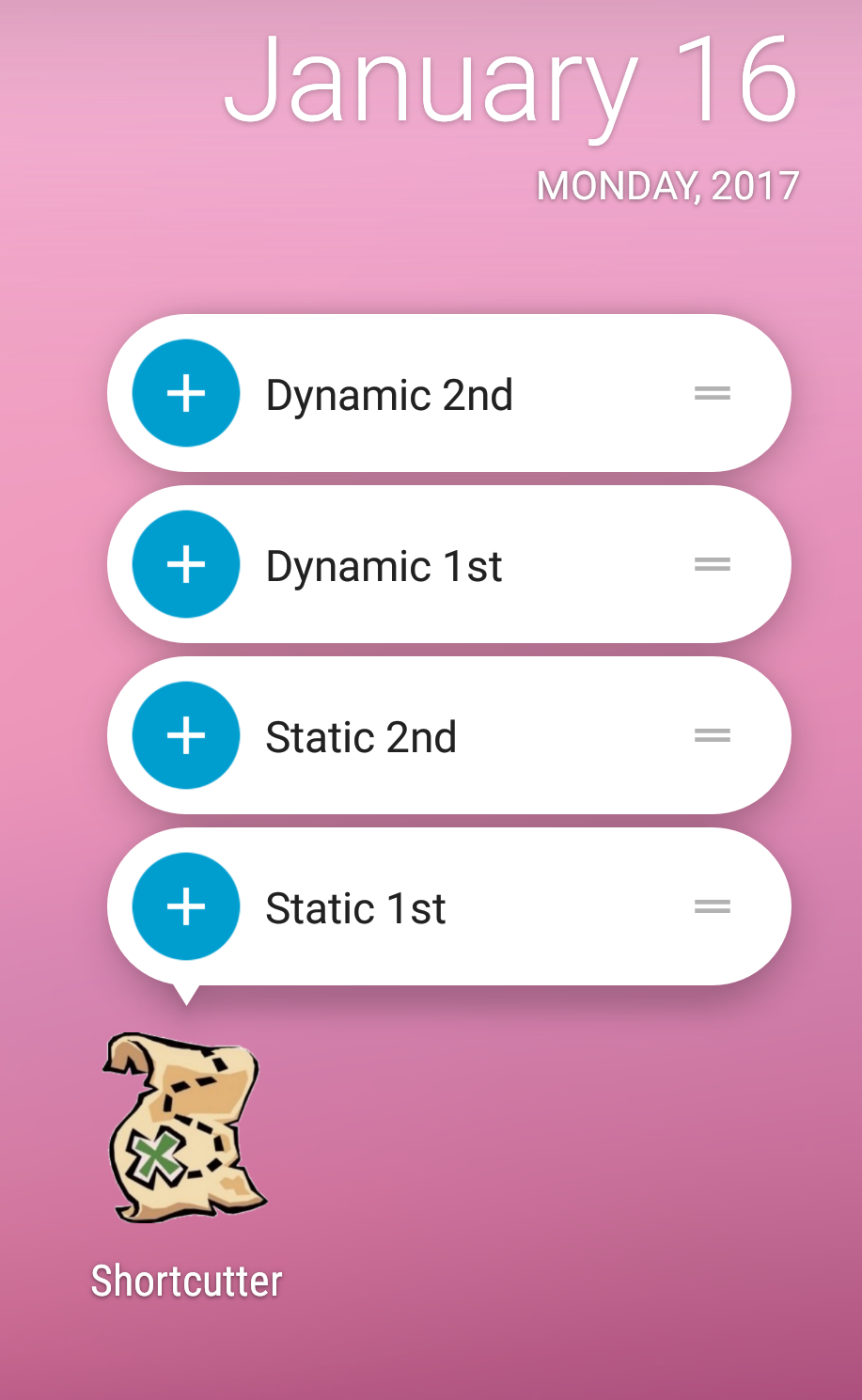

Shortcut Ranks
Android Development claims that each shortcut has a rank - it can be obtained using
getRank()
. But you cannot set this rank yourself; the OS installs it for your own reasons. So far, in all cases that we have met, this rank has been set depending on the order of addition. The rank, by the way, is a non-negative number, and the lower this number, the “priority” the shortcut is considered, the closer it will be to the application icon (rank 0 is the closest).2. When creating a shortcut, you must set the long and short messages. A long message is indicated in the list, if it does not fit, then a short one. Google recommends setting a short message to no more than 10 characters, and a long message to no more than 25.
Additional facts
If both messages are too large, a short one will be displayed in an abbreviated form. Also, “fixed” shortcuts in any case are signed with a short message, even if the short message has more characters than the long one.
3. Although you can add a maximum of five shortcuts, Google recommends not adding more than four. And in the list more than four shortcuts will not be displayed (the last added one will not appear). Why you need a fifth shortcut is a mystery, because it also does not appear on tablets.
4. The attribute of
targetPackage
static shortcuts has one feature - it can only have a hardcode value. If you use different suffixes for different builds of the application applicationId
, then when declaring a shortcut, specifying the targetPackage value with a link, for example, @string/shortcut_target_package
will fail. You will have to create a file shortcuts.xml
separately for each buildFlavour
application and register the necessary targetPackage
manually with the desired suffix applicationId
.5. It is important that Android itself does not regulate the number of added shortcuts. If for some reason you want to add the sixth shortcut, the application will simply crash by throwing it away
IllegalArgumentException: Max number of dynamic shortcuts exceeded
. Keep track of the number of added shortcuts yourself. 6. If you do not set the action attribute to at least one shortcut intent, then in the case of a dynamic shortcut, the application will crash when this shortcut is created, and in the case of a static shortcut, the shortcut will simply not be created. Note that some types cannot be put in extras of shortcut intents, for example
Serializable
, valid types can be seen in PersistableBundle sources . 7. If you try to call
getSystemService(ShortcutManager.class)
on the device below 23 API, the application will crash with ClassNotFoundException
.8. When adding several intents to a shortcut that are processed by different activities, you can build a certain stack of activities. The lowest in this stack will be the activity that processed the first added intent.
Practical implementation

9. If the user is locked on the device, then he will not be able to use shortcuts - they are contained in the encrypted storage.
10. If the user wants to support the application on another device, then only “fixed” shortcuts will be recreated on it. Dynamic and static are not recreated, but static will be redefined during application installation. If you are worried about supporting the application on different devices, and you have not set a flag
allowBackup = false
in the Manifesto, you only need to worry about manually recreating dynamic shortcuts. 11. Android developers also suggest us using the method
reportShortcutUsed(String id)
when performing an action from the corresponding shortcut - both when clicking on the shortcut, and locally in the application, if the action is the same. According to the developers, there should be statistics on the use of certain actions from shortcuts, and based on these statistics certain shortcuts can be offered to the user. Perhaps it is here that the potential of the fifth shortcut should be revealed.Principle of operation
Suppose, for example, that a user every night at 7 o’clock selects the way home in the navigator and this action is saved in a shortcut. Based on this, this shortcut will be more priority when showing the next time at 7 pm. However, in practice, I have not yet encountered a single instance of the implementation of this feature, so I can not confirm or deny this information.
Finally, a couple of not yet mentioned tips from Android developers.
1. Update shortcuts (
updateShortcuts()
) if their contents and meaning have not changed, and only the displayed message or icon has changed. If you want to remove one shortcut and add another, then do not use the update, perform the delete and then add operations. Neglecting this advice, you can get all sorts of incidents with "fixed" shortcuts. 2. Follow the shortcut design guide . This is a small document that is easy to follow.
Main
• the icon should be round in the general case (diameter - 44 dp);
• inside the icon should be a simple icon, like a plus sign (size - 24 x 24 dp);
• colors should be consistent with the overall theme of the application;
• when choosing an icon, remember that it will be displayed on the “fixed” shortcuts along with the main application icon at the bottom right, so you should not choose something like this:
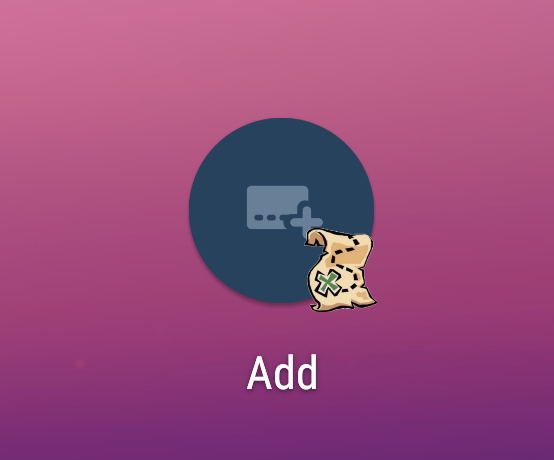
• inside the icon should be a simple icon, like a plus sign (size - 24 x 24 dp);
• colors should be consistent with the overall theme of the application;
• when choosing an icon, remember that it will be displayed on the “fixed” shortcuts along with the main application icon at the bottom right, so you should not choose something like this:
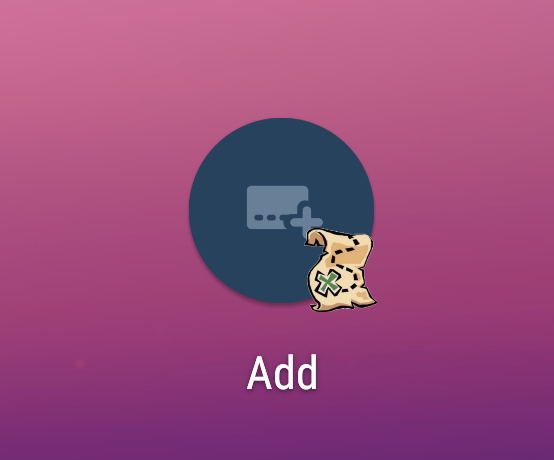
Conclusion
Android Shortcuts is a powerful application tool. Serious multi-functional applications should use it to keep up with the times. I hope this guide inspires you to add such a mechanism to your applications and helps you implement it painlessly. You should not use shortcuts in your application if you know that they are needed there just like a fifth wheel cart. Remember the relevance, because shortcuts are not added to expand the functionality, but for the convenience of the user.