We get Vk music through a third-party API
This time it started after the audio methods were closed in the execute method.
I decided to see how the sites that provide music can be downloaded. I was interested in the site vrit.me.
I climbed into the network tab and saw an interesting query:
a photo
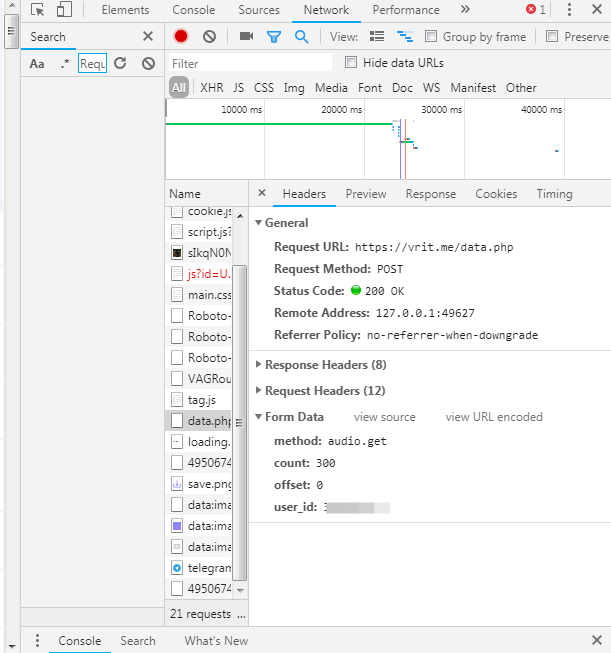


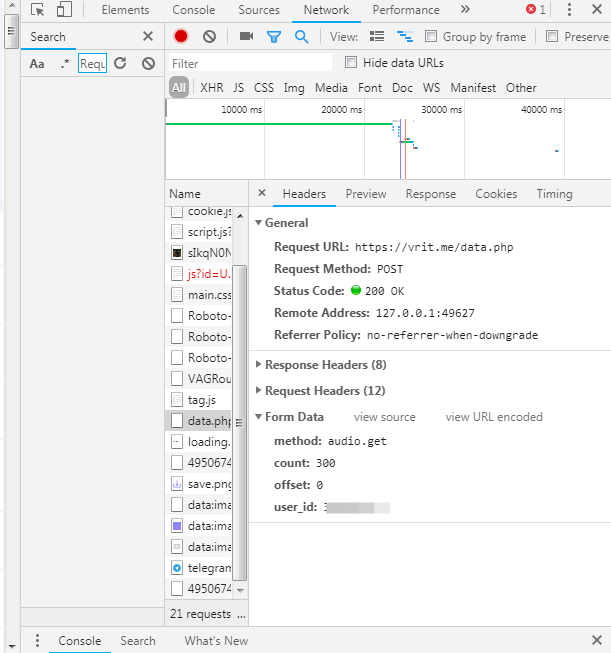

That is, you can fake a POST request to this site, and use it as an API for music vk, which I immediately implemented:
import json,requests
s = requests.post("https://vrit.me/data.php",data={
"method": "audio.get",
"count": 1000000000,
"offset": 0,
"user_id":-52922518})
s = json.loads(s.text)
print(s)
As a result, I received the following answer:
{'response': {'count': 2, 'items': [{'id': 456239018, 'owner_id': -52922518, 'artist': 'CORVUS', 'title': 'Осеннее смятение [ЗС]', 'duration': 126, 'date': 1474194635, 'url': 'https://cs1-81v4.vkuseraudio.net/p18/894f30b49d3571.mp3?extra=5xovvbyqXrdr0Ixl9FLteg-pRRC29pGr_yO8mDgqNN_4kLlxJe1gHST8S8bVy2IQt0wYFAC1tMCnF7p5ujeB7K1jFPfYSCaEuxjh5P92VT81AMd9AlIJx2GQp613xHxCRbXCynv6fqdhFcPwvyZaRvg', 'lyrics_id': 370291093, 'genre_id': 18, 'is_licensed': True, 'is_hq': True, 'track_genre_id': 11, 'access_key': '7b762a5b22b452d0ca'}, {'id': 456239017, 'owner_id': -52922518, 'artist': 'CORVUS', 'title': 'Записки сумасшедших [ЗС]', 'duration': 132, 'date': 1470474699, 'url': 'https://cs1-81v4.vkuseraudio.net/p4/7f6c08b134e0a7.mp3?extra=MgBr8oDpO-7f2l_qmtHZGAMD608vcqWxA8OLNgcyZDdA8aVc2Jlj9bDW48FW8S5zDA9jO-geAEUaF_LvFUP_DFiGZEFo-5B92YhcMYMpyuvi6tFt_nO4QVwjJjNhG-Ln3dOfkC4KY2Cywk_attG5fSQ', 'lyrics_id': 370291112, 'genre_id': 18, 'is_licensed': True, 'is_hq': True, 'track_genre_id': 11, 'access_key': '8717a672801e7a15fa'}]}}
BUT! because any link to the audio is attached to the computer’s IP address,

then when I opened it on my PC, I received an error:

Then I decided to see how the link is generated on the vrit.me website itself. It turned out that this link is substituted into another link and as a result, the output is a link of the form
https://vrit.me/download?artist=Егор Крид&title=ЭТОМОЕ&url=https://cs1-60v4.vkuseraudio.net/p20/6d11e54193b7e0.mp3?extra=CZi_FWKxxoYdOTg7Sz4cksgJ_l12bqsxH8wZFPRoN6t7qf4at_MDouTA6MDmsLiaoFrDJDswVzKozagVNVCskf3LiR3ry-JvP9WHgisWn7nq7BradXYcffgAlQH2VTWoTFDgpVwhdRZMUV6ATpr6KQ

That is, we need only the resulting link to “enter” into another link. Implementation:
for i in range(len(data["response"]["items"])):
url = data["response"]["items"][i]["url"]
title = data["response"]["items"][i]["title"]
artist = data["response"]["items"][i]["artist"]
data["response"]["items"][i]["url"] = "https://vrit.me/download?title={title}&artist={artist}&url={url}".format(url=url,title=title,artist=artist)
Other methods with audio
I also tried to call such methods as “audio.getById”, “audio.search”, “audio.getCount”, “audio.getLyrics”, “audio.getAlbums” but only one of them works “audio.search”, and you cannot search for the music by user, only in the global search. Code:
s = requests.post("https://vrit.me/data.php",data={
"method": "audio.search",
"count": 3,
"offset": 0,
"q":q})
data = json.loads(s.text)
if"response"in data:
data = data["response"]
for i in range(len(data["items"])):
url = data["items"][i]["url"]
title = data["items"][i]["title"]
artist = data["items"][i]["artist"]
data["items"][i]["url"] = "https://vrit.me/download?title={title}&artist={artist}&url={url}".format(url=url,title=title,artist=artist)
Final code
import requests,json
classaudio():defget(owner_id):
s = requests.post("https://vrit.me/data.php",data={
"method": "audio.get",
"count": 1000000000,
"offset": 0,
"user_id": owner_id})
data = json.loads(s.text)
if"response"in data:
data = data["response"]
for i in range(len(data["items"])):
url = data["items"][i]["url"]
title = data["items"][i]["title"]
artist = data["items"][i]["artist"]
data["items"][i]["url"] = "https://vrit.me/download?title={title}&artist={artist}&url={url}".format(
url=url,
title=title,artist=artist)
return data
defsearch(q):
s = requests.post("https://vrit.me/data.php",data={
"method": "audio.search",
"count": 300,
"offset": 0,
"q":q})
data = json.loads(s.text)
if"response"in data:
data = data["response"]
for i in range(len(data["items"])):
url = data["items"][i]["url"]
title = data["items"][i]["title"]
artist = data["items"][i]["artist"]
data["items"][i]["url"] = "https://vrit.me/download?title={title}&artist={artist}&url={url}".format(
url=url,
title=title,artist=artist)
return data
You can call this class like this:
import bot_vk#pip install bot_vk==1.7
info1 = bot_vk.audio.get(owner_id=1234567)
info2 = bot_vk.audio.search(q="imagine dragons")
PS This article presents an example of receiving music from one of the sites. There are many more similar sites from which you can also make an “API”. Most likely the site vrit.me will soon cease to be valid, and you will need to use other sites.
ATTENTION! The author of this post is not responsible for any of your actions. This post is created for informational purposes only!