
PassGenJS. We generate passwords in Javascript with the indication of reliability
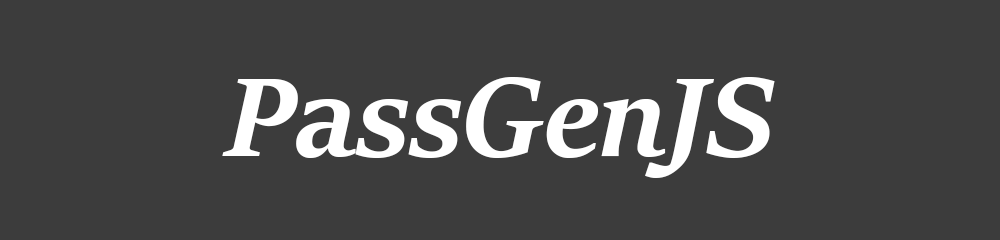
In one project, there was a need to generate a strong password on the client. I looked for a ready-made solution, but did not find anything suitable.
All the libraries that were found did not fit for a number of reasons - they generated a password simply by the desired length, there was no way to check the password strength. It was decided to write your own “bicycle” - as an alternative solution, and experience would not be superfluous. The result was a PassGenJS library .
What's under the hood:
- No dependencies
- Password generation according to the specified parameters (number of letters, numbers, characters, etc.)
- Password generation by reliability value (1 - weak, 4 - highly reliable)
- Password generation by% reliability (from 0 to 100%)
- Password strength verification through entropy calculation
I will give some examples:
We generate a strong password:
PassGenJS.getPassword({score: 3});
// Результат - 8!G$}6&={a(_>
We generate a very simple password:
PassGenJS.getPassword({score: 1});
// Результат - 82oN
We generate a password with 60% reliability:
PassGenJS.getPassword({reliabilityPercent: 60});
// Результат - YyopjU5atXBMG
We generate a password with character parameters:
PassGenJS.getPassword({
symbols: 2, // Количество спец. символов
letters: 2, // Количество букв
numbers: 1, // Количество цифр
lettersUpper: 5 // Количество букв в верхнем регистре
});
// Результат - m6A:k=WYPP
It is also possible to check the password strength:
PassGenJS.getScore("YyopjU5atXBMG");
// Результат:
{
"password": "YyopjU5atXBMG", // Пароль
"score": 3, // Надёжность пароля (4 - наилучший)
"entropy": 77, // Энтропия пароля
"reliability": 60.15625, // Надёжность пароля (абсолютное значение)
"reliabilityPercent": 60 // Надёжность пароля в %
}
The estimation algorithm used in this plugin is based on the general principles of information theory.
As an estimate of password strength, the value of its entropy is used. Read more on Wikipedia .
Under the entropy (information capacity) password implies a measure of randomness of the selection sequence of characters that make up a password, evaluated methods of information theory. As the formula used to determine the password entropy,

where N is the number of possible characters, and L is the number of characters in the password. H is measured in bits.
As part of the plugin, the following conditions are used to determine the strength of the password by its entropy:
- N <56 - Weak (1)
- 56 <= N <64 - Medium (2)
- 64 <= N <128 - Reliable (3)
- N> 128 - Highly Reliable (4)
if (entropy > 0 && entropy < 56) {
score = 1;
} else if (entropy >= 56 && entropy < 64) {
score = 2;
} else if (entropy >= 64 && entropy < 128) {
score = 3;
} else if (entropy >= 128) {
score = 4;
}
I will be glad to healthy criticism and any feedback. If someone finds the plugin useful, then you can develop it further:
- jquery plugin
- password length restrictions
- exclusion of certain characters
- adding arbitrary letters (via parameter passing)
- etc.