
Raspberry Pi and a cup of Java, please! (Part 2)
- Tutorial

This article is a translation of the second part of Java Raspberry Pi articles. The translation of the first part aroused great interest in the possibility of using Java on the Raspberry Pi. The original of this article was published in the 16th issue of MagPi .
This is the second article about using Raspberry Pi for programming in Java. After reading it, you will learn more about the designs of this language and will be able to try to write more complex programs. It can also be useful for those who want to learn about C, since the basic Java syntax is the same as C.
At the beginning of this article, I will describe control structures in more detail. And then I will go on to describe the numeric, string and logical data types, giving examples of their use. With all of this in mind, more complex execution logic will be added to our sample programs. Well, let's get to work!
More about control structures
In the first article in MagPi # 14, we introduced two control constructs: if and while . I know that you remember this, but just in case, I remind you. The if construction block will be executed only if the value of the logical expression in parentheses is true. For example:
if (a > 3) { c = c + a; }
We add a and c only if the values of a are greater than 3. The expression in parentheses, called the state, can take the value true (true) or false (false), i.e. boolean data type (Read more about the logical data type below in the text of the article).
The if construct may include a block that starts when false . For example:
if (score > 50) {
System.out.println("You won! :)");
} else {
System.out.println("You lost. :(");
}
The next construction we examined was while . A block of the while construct can be executed only in cases when the state of the expression in brackets is true , i.e. it can be one or more times, or maybe not once. For example:
int f = 10;
while (f < 20) {
System.out.println(f);
f++;
}
Integers from 10 to 19 will be printed until the variable f becomes equal to 20 and the result of the expression changes to false . Also, did you notice the fourth line? Well, if you notice. Two plus signs after the variable f increase it by 1. This is a short view of the record:
f = f + 1;
Similarly:
f--;
Minus signs reduce the value assigned to the variable f . Otherwise, it will look like this:
f = f - 1;
Now let's look at the for construct . The for construct is similar to while , but for it, on one line, you can combine execution criteria and an expression that change the value of a variable. Therefore, enumeration of numbers from 10 to 19 can be written using for :
for (int f = 10; f < 20; f++) {
System.out.println(f);
}
Yes, this option looks more compact than the while example . However, while , in some cases, may be more convenient for looping.
Section in parentheses to design for divided into three parts. The first is to initialize the variable at the beginning, the second is to check the variable at each repeat of the cycle, the third is to change the variable at the end of each cycle.
Now open your editor and create a new program:
public class Countdown {
public static void main(String[] args)
throws InterruptedException {
for (int t = 10; t >= 0; t--) {
System.out.println("-> " + t);
Thread.sleep(1000);
}
System.out.println("-> Fire!");
}
}
We compile it with the javac command and run java (more in the previous article ). This program will count 10 seconds and then print “Fire!”. How does the program change the variable t ? Double minus reduces the value of t from 10 to 0. But why then did you need the String Thread.sleep (1000); ? It creates in the program a delay of 1 second (1000 milliseconds) at each step of the cycle. Using the Thread.sleep method , you only delay the scope of the for block . Now let's leave it as it is, I will talk about handling exceptions later.
Let's complicate task # 3 : Create a new program Countup.java for counting from 1 to 20 with a delay of one second. And when the score reaches 15 we display the message “Five to go ...”.
Well, finally, take another look at switch . The switch construct checks the values of the variable and, depending on what the variable in parentheses is, executes the code after the colon. Example:
import java.util.Random;
public class CardinalDirection {
public static void main(String[] args) {
Random r = new Random();
int dir = r.nextInt(4);
switch (dir) {
case 0:
System.out.println("North");
break;
case 1:
System.out.println("West");
break;
case 2:
System.out.println("South");
break;
default:
System.out.println("East");
break;
}
}
}
In this example, an integer is randomly generated in the range from 0 to 3. But, interestingly, the value of the number is not shown, it is only needed to indicate. Each of the blocks is executed only if the value of the variable matches the value after case . Note I did not set case for the number 3, I used the default construct . This is a good example of the fact that it is better to always set default , so that for any non-matching value this block of commands is selected.
Well, well, that’s all I wanted to talk about control structures. Of course there are other more exotic constructs like continue or do-while, but they are not used so often and I will leave them to you, for independent study.
Well, now you can move on to a completely different topic in Java.
A couple of bits about numbers
Sooner or later, you will need mathematical calculations, this chapter gives the basics of why you will need to begin to study them. In Java, every numerical variable is one of the basic types of language. Java has four integer types (without decimal places), two floating point (with decimal places) and two high precision. For use in a program, you declare a variable once, then, as the program runs, change its values. For example:
int trees; // declaration
trees = 17665785; // assignment
But you can immediately declare a variable to assign a value to it, for this we combine two lines into one:
// declaration & assignment
int trees = 17665785;
Did you notice // ? Two slashes indicate the beginning of a comment; Java ignores everything that comes after them. In this way, you can leave after them any notes and reminders on the implementation of the program, so that in a couple of months or even years, remember how your program should work.
And now the range of available values for the four types of integer variables:
byte: от -128 до 127
short: от -32 768 до 32 767
int: от -2 147 483 648 до 2 147 483 647
long: от -9 223 372 036 854 775 808
до 9 223 372 036 854 775 807
More often you will need the third type ( int ). But if you need decimal (floating point) numbers, there are two data types in Java:
float: от ±1,401298e-45 до ±3,402823e+38
double: от ±4,94065645841246e-324
до ±1,79769313486231e+308
Typically, for mathematical calculations, they prefer the double type (15 decimal places) than the float type (7 decimal places).
In Java, four arithmetic operators + , - , * and / , and also parentheses to prioritize the calculation. Below are the calculations with these operators:
public class Numbers {
public static void main(String[] args) {
int x = 5;
System.out.println(7 + x * 3); // 22
System.out.println((7 + x) * 3); // 36
System.out.println(7 / 3); // 2
// 1 (remainder)
System.out.println(16 % 3);
// 2.33333333
System.out.println(7.0 / 3.0);
System.out.println(3.14159265 * x * x);
}
}
Multiplication and division operations take precedence over addition and subtraction. Pay attention to the action of the operators / and % when dividing integers, if in the first case (operator / ) only the integer part remains after division, then in the second case (operator % ), on the contrary, the integer part is discarded, and as a result, the remainder 1 of the division remains 7 to 3.
Do you need more math functions? Ok, call the Math class . An example is this formula:
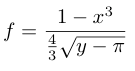
In Java, it will look like this:
double f = Math.abs(1.0 - Math.pow(x, 3.0)) /
(4.0 / 3.0 * Math.sqrt(y - Math.PI)));
When we write integer and fractional numbers, we highlight this with a dot. For example 3 integer, and 3.0 fractional.
The following program displays all integers from 1 to 10, not divisible by 3, without a remainder:
public class Sum {
public static void main(String[] args) {
int total = 0;
for (int n = 1; n <= 10; n++) {
if (n % 3 != 0) {
total = total + n;
System.out.println(total);
}
}
System.out.println("Total: " + total);
}
}
As a result, we get 1, 2, 4, 5, 7, 8, 10 and their total is 37.
Let's complicate task # 4 : Change the last program and display the numbers that are not divisible without remainder by 4.
If you need more details, what other features are available in the Math class :
docs.oracle.com/javase/7/docs/api/java/lang/Math.html
But what if 15 decimal places are not enough? Then you need the types BigInteger and BigDecimal , these are classes for carrying out calculations with very high accuracy. If necessary, then one hundred, one thousand or one million decimal places. Example:
import java.math.BigDecimal;
public class HighPrecision {
public static void main(String[] args) {
BigDecimal a = new BigDecimal("2.7182818284"
+ "590452353602874713526624977572"
+ "4709369995"); // 50 decimal places
BigDecimal b = new BigDecimal("3.1415926535"
+ "89793238462643383279502884197169399"
+ "375105820974944"); // 60 dec. places
System.out.println("e*pi="
+ a.multiply(b));
}
}
Run the program and you will see everything for yourself.
A couple of bits about strings
You already had the opportunity in each of our examples to become familiar with the use of strings. The type String is a sequence of letters, numbers, and characters. The simplest example is “Hi John!” But most often, strings are used to search for text or to compose large messages from several parts. To do this, you can use the + sign , it will combine several lines into one:
String qty = "50";
String message = "I found " + qty + " books.";
If you need to get part of the string, then use the substring method . Each character in a string has a position. The numbering is 0. In the following example, we need to get the word "house", its characters are located from 4 to 8 positions:
String line = "The house is blue.";
String word = line.substring(4, 8 + 1);
Well, if you need to find out exactly where the beginning of the word "house" is, then use indexOf :
String line = "The house is blue.";
int position = line.indexOf("house"); // 4
int red = line.indexOf("red)"; // -1
Note indexOf is written with a capital "O". In the last line of the example we are trying to find the words “red”, but it is not there and the method returned -1 to us.
You can find out the length of a string using the length () method . To get individual characters, we use charAt () , the following example shows how to decompose a string into characters and display them in letters.
public class LetterByLetter {
public static void main(String[] args) {
if (args.length == 0) {
System.out.println("Please type"
+ " a word.");
} else {
String word = args[0];
for (int i = 0; i < word.length(); i++){
System.out.println(word.charAt(i));
}
}
}
}
After compiling javac , run the program by specifying the command line word after the name of our class.
java LetterByLetter telephone
As I said in the last article, through the args variable, the line entered at startup is passed to your program (in this example, it is “telephone”). Try not to enter this word and the result will be different. The control constructions ensure the operation of the program so that from the beginning we get the size of the string and only then we select the letters in order.
There are many methods for working with strings. This is described in more detail in the documentation docs.oracle.com/javase/7/docs/api/java/lang/String.html
Let's complicate task # 5 : Change the previous example and make each letter uppercase. Hint: use the method that immediately makes all letters of the string uppercase, this method can be found in the documentation at the link above.
A couple of bits about logic
The boolean boolean type can have only two options for the variable value: true or false . It is most often used as an indicator or as a switch for control structures if , while or for . An example of a boolean variable declaration:
boolean painted = false;
And so we change its value:
painted = true;
Or use for while :
while (painted) {
// do something
}
You can also use operators for logical variables ! (NOT), && (AND) and || (OR). The operator ! returns the return value of a variable. The && operator returns true if both sides of the expression are true and the || returns true if at least one of them is true . Example:
boolean a = true;
boolean b = false;
boolean c = !a; // false
boolean d = a || b; // true
boolean e = a && b; // false
boolean f = (!a && b) || a; // true
In the future, you may encounter operators & and | . These are also the AND and OR operators, but for numeric variables. But the version of the && and || more efficient, since there is no difference in the arrangement of the operands. In the following example, you can see that the result does not depend on the location of the operands:
boolean g = a || b; // true
boolean h = b && a; // false
Numeric and string variables can also return a boolean type result . This is a comparison result for control structures. Example:
int total = 156;
if (total > 100) {
// do something
}
The expression in parentheses compares the variable total , if total is greater than 100, the result will be true , otherwise false . You can use the following six operators to compare two numbers:
== равно
!= не равно
> больше
< меньше
>= больше или равно
<= меньше или равно
All returned values will be of type boolean , and you can combine them with other operators of type boolean , for example:
int sides = 6;
boolean painted = false;
if (sides >= 4 && !painted) {
// paint it!
}
Conclusion
This article included several topics, and it turned out to be more complicated than the first, but I tried to make it so that you read the basics of Java more quickly.
In the next article, we will get acquainted with the methods, classes and objects of Java, with some class libraries included in Java. Then you can make a program generating a card.