
DIY cloud API for mobile applications. Part 1
Instead of joining
At the dawn of programming, and until very recently, a program was something complete, completely ready-to-use, an independent unit that performed its functions and only them.
However, with the advent of mobile devices, rich logic websites, and social networks, things began to change. Now programs that do not go online do not know how to put something on Facebook and generally work on their own, practically have no right to life. Even professional tools, such as Microsoft Office 2013 , began to support cloud storage for document sharing.
The world is changing. Now, to make money selling software, you do not have to write your own operating systemor antivirus, spending a lot of time and resources. It’s enough to just ask your wife and together develop a world hit . Therefore, many today dream of creating their own evil birds or cut-out, studying development for iOS , Android , Windows Phone .
Suppose you wrote your application and published it in one of the stores. Everything is fine, you make a profit, but you want more. You understand that you need to write applications also for other platforms in order to expand the user base. This is where the first ambush lies: how to minimize the amount of code that you write if the applications work for the most degree the same and differ only in appearance (and that is not a fact) and in the programming language?
The answer to this question will be the old man OOP, dressed in fashionable clothes and changed his name. If you take the general logic from the application code to a certain general service and place this service on the Internet so that all applications can connect to it, then to implement a mobile application on a specific platform, you only need to write the code for displaying data from the server. Sounds familiar, right? This is very similar to the MVC pattern . Here Model is a service on the Internet that receives and gives data, while View and Controller are implemented on a mobile device and can be simplified as much as possible. As a model of the device of such a service, today they are increasingly using the so-called RESTful API - A software interface that can be accessed through standard HTTP methods.
And everything seems to look good and it already seems that a solution has been found. However, problems begin when you begin to deploy the backend service on the server yourself. I am a programmer, and when it comes to installing and configuring a server, I immediately feel uneasy. Firstly, this is a completely new knowledge that needs to be studied so that everything works as it should. Secondly, in order for everything to work really as it should and not fall with an increased load or attack, you need to smoke mana even more and more persistently. You can try to find a host that will save you from fussing with servers and pre-install PHP and / or something else. But then the problem will remain that it will be necessary to independently implement all the necessary server event handlers to implement a full REST API. And this again takes up your good time and spends it on unnecessary things.
Why am I all this?
I myself have been tormented by the question for a long time, but how can I organize my server backend for the application. At first I wrote something of my own using WCF services. Then came the ASP.NET Web API , which made life pretty simple. But today I want to tell about something else. As a fan of easy-to-use things, I could not get past the relatively new service that appeared in the Windows Azure cloud platform . The name of this service is the Windows Azure Mobile Services Custom API.
This service, along with other useful features of Windows Azure Mobile Services, is a PaaS solution and provides the ability to quickly deploy a cloud-based RESTful API, which can be accessed by a program in any language and platform. The basis of this solution was no longer the new, but rather popular Node.js technology . The Custom API is a fully functional Node.js application with all its consequences - it is a complete solution without compromise. And taking into account the fact that native SDKs were written for working with it for all three popular mobile platforms, this solution becomes even more interesting.
Further in this part I want to talk about how to create and start using the cloud backend in Windows Azure and access it from a mobile device. Do not switch!
Creating a cloud backend
Creating a cloud backend, like any other service in Windows Azure, comes from the cloud management portal . First you need to create a mobile service, having come up with some kind of sane name:

Next, you need to select the SQL database in which the mobile service data will be stored. There is an option to create a free copy for 20Mb. For testing opportunities - enough for the eyes. And if you like it, you can always upgrade to more serious decisions.
After clicking on the arrow next and entering the parameters of the database server (create a new one or use the existing administrator login / password and other boredom), a new mobile service will begin to be created in the cloud. This usually happens extremely quickly, in less than a minute. When the service is created and you go into it, you will see something like this window:

Remember it, it will come in handy later.
API Creation
To create your first cloud API, simply go to the API tab and click the Create a Custom API button:
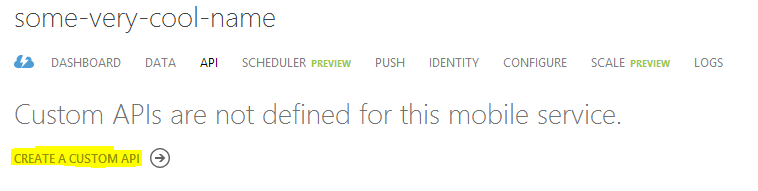
If you have previously worked with Windows Azure mobile services, the next window will be familiar to you. It is necessary to specify the name of the future API in it, as well as one of the four levels of access to its various methods. Let's leave everything as default, then only those clients who have an authorization parameter will be able to connect to our API:

Once the new API has been created, we can start editing it. Initially, you should see a script similar to this:

For educational / test purposes, I suggest changing it slightly to get it like this:
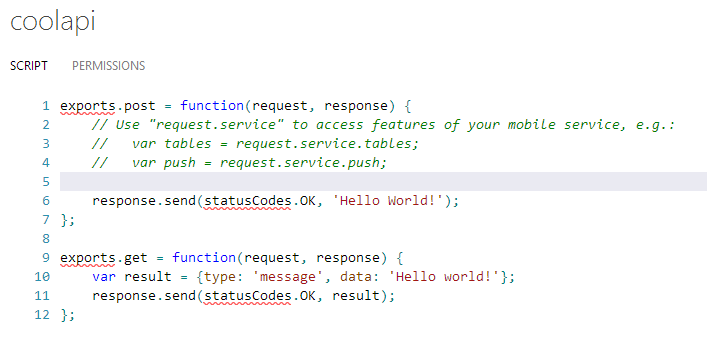
The main change in this case was that in the POST request handler data began to be returned as a simple string, and a variable was added to GET, and its object has more than one field. I have done so for illustrative purposes, to illustrate the various possibilities of working with data.
API usage
For this article, we will use the test application that Windows Azure Mobile Services kindly provides us. To do this, go back to the Quick Create page (this is the one with the cloud and lightning on the icon), select Windows Phone 8 (although pay attention to the rich selection) and click Create A New Windows Phone App:
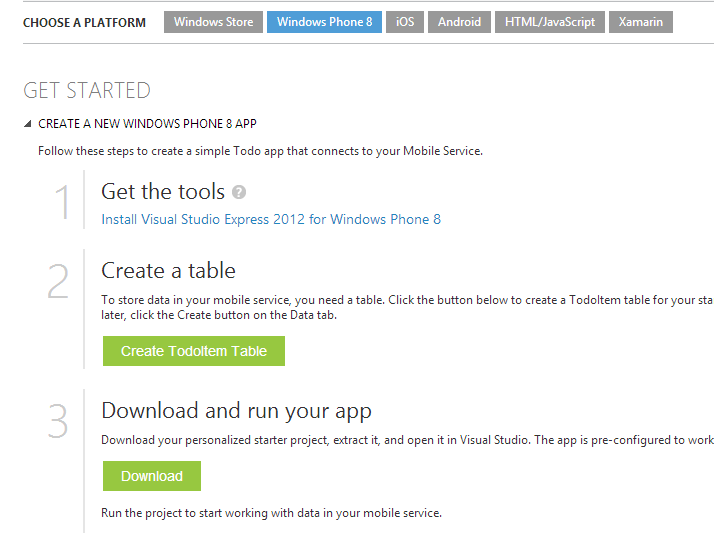
After creating the desired tablet (TodoItem) and downloading the application on the Download button, open it in Visual Studio.
First of all, we are interested in two things. The file App.xaml.cs has a line similar to this:
public static MobileServiceClient MobileService = new MobileServiceClient(
"https://mva-test-api.azure-mobile.net/",
"тут_набор_непонятных_символов"
);
Using this field, we will communicate with our newly made mobile service. A set of obscure characters - this is ApplicationKey, the individual key of your service, holt it and cherish it.
Let's launch the application and at least see how it looks:
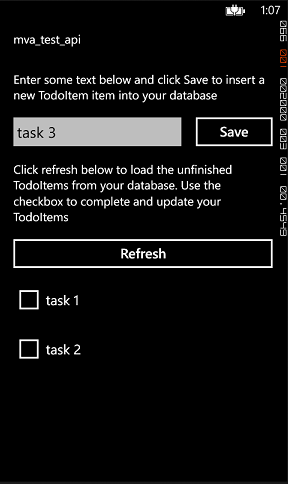
Well, not an hit, but for a start it’s not bad. Let's endow it with additional logic and call our API methods. We go to the MainPage.xaml.cs file. From him, I want to ensure that when you click on the Save button, access to services occurs, after which the result is simply written to the debug console. To do this, add the following code to the end of the InsertTodoItem method:
// Обращение к POST
var result = await App.MobileService.InvokeApiAsync("CoolAPI", null, HttpMethod.Post, null, null);
Debug.WriteLine(result.StatusCode);
var stringData = await result.Content.ReadAsStringAsync();
Debug.WriteLine(stringData);
// Обращение к GET
var resultJson = await App.MobileService.InvokeApiAsync("CoolAPI", HttpMethod.Get, null);
Debug.WriteLine(resultJson);
The main method in this code can be called InvokeApiAsync. He is responsible for calling an API method. This method is overloaded and endowed with a different set of parameters. The example shows that in the case of POST we pass as many as 5 parameters, and in the case of GET, only 3. This is due to the fact that the method for POST is designed to result in a regular string (remember the implementation of the script on the backend), and the option with GET - to work with a JSON object (the result will be Newtonsoft.Json ).
If you now start the application and click the Save button, then something like this will be visible in the Debug console of the application:
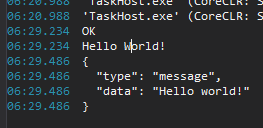
How are they
I did not just ask to pay attention to the possibility of choosing the type of application on the Quick Create page in the Windows Azure Control Panel. The fact is that the native SDK for working with mobile services is written not only for Windows devices. Their libraries are released for both iOS and Android, so all the same can be used on these platforms. Here is an example of ObjectiveC code that will do roughly the same thing as in the example:
[self.client invokeAPI:@"CoolAPI" data:nil HTTPMethod:"POST"
parameters:nil headers:nil completion:^(NSData *result,
NSHTTPURLResponse *response, NSError *error) {
NSLog(@"%i", response.statusCode);
NSString *stringData = [[NSSatring alloc] initWithData:result encoding:NSUTF8StringEncoding];
}];
As you can see - a "clean" ObjectiveC, without fraud. Similarly for Android, WinRT and even for the web version (HTML and JavaScript).
What's next
I initially planned to write only one article at once about all the features of server code in Windows Azure Mobile Services, but there is too much material. So now we are done, and in the next part I will talk about how you can work on a cloud backend in a team using Git. And also about how to expand functionality with general scripts and NPM packages.