
Getting started with Windows Phone 8 Development: Lesson 2. Accessing the local application store
- Transfer
- Tutorial
Beginning of Windows Phone 8 Development: lesson 1. Application layout and event handler
Beginning of Windows Phone 8 Development: lesson 2. Accessing local application storage
Beginning of Windows Phone 8 Development: lesson 3. Navigating through pages and passing parameters
Beginning of Windows Phone 8 Development: lesson 4. Communication with services and data binding.
And so, let's proceed to the second lesson. I hope it will be more interesting for you. Many developers for smartphones are interested in the issue of the availability of storage on the device for their applications, as well as its size and use. Each application has its own isolated local storage. It is independent of other applications and the OS, and only this application has access to it.
Two questions immediately arise: the ability to use an SD card and the size of available space. The answer to the first question is yes, you can use an SD card. The answer to the second question is unlimited. That is all the free space.
As for the application that we will create in this lesson, it will use local storage for reading / writing text messages and a counter. The counter will increase every time you start the application, and the last message you entered will also be displayed.
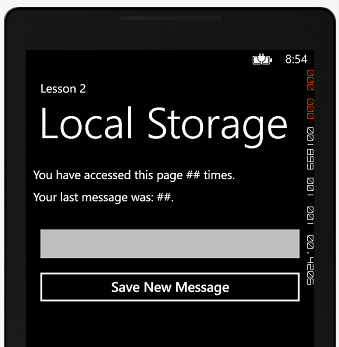
As you already noticed, two TextBlocks are used to display messages and a counter, and one TextBox is used to enter a new message. The layout, as always, uses XAML and my favorite StackPanel:
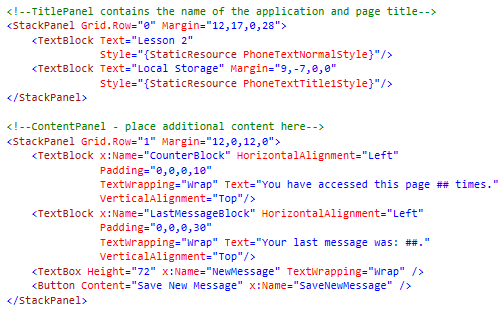
This time for TextBlocks we set the Name properties, as we will transfer the contents to them from the outside. Now consider what happens when the page loads.
Before adding logic, make sure that the necessary namespaces are added:
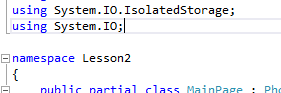
When loading a page, first of all, you need to make sure that the file in which the counter is stored and the message exists. If it exists, then we read the necessary data and pass it to the global variable. If there is no file, then we write in the counter “0”, and in the messages “Empty” (No messages ..!) Of the new file. I will use a file called LS.txt (short for Local Storage).
Well, of course, I will pass the values to TextBlocks replacing the “##” characters in the string with the variable values using the string.Replace function.
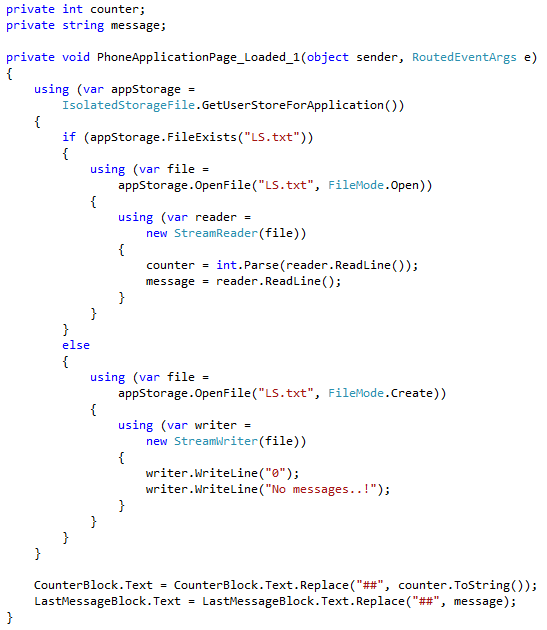
If the application closes when you click the Back or Start buttons, we replace the LS.txt file with a new one, after updating the data with the current ones. First we increase the counter, and then we record the message.

Well, at the end of everything, you need to save a new message and exit the application by raising the OnNavigatedFrom event.
We pass the contents of the TextBox to a variable, and display a text message to the user about the successful save.
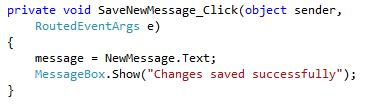
Start by pressing F5 and wait for the download. After loading, we see the counter shows 0, and there are no messages. We recruit new and save.
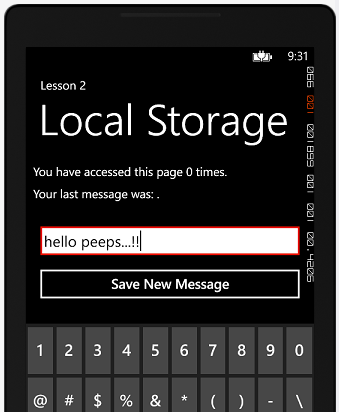
After clicking on Save we see a text message. After a successful save, the increment of the counter is called, and the application closes itself.
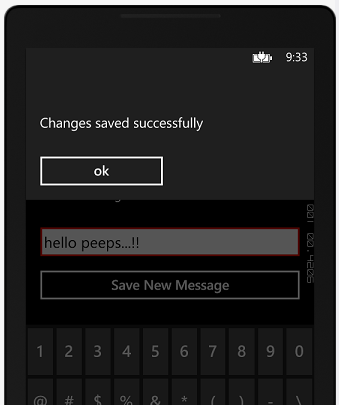
Now run it again. Everything works. Everything is being updated.
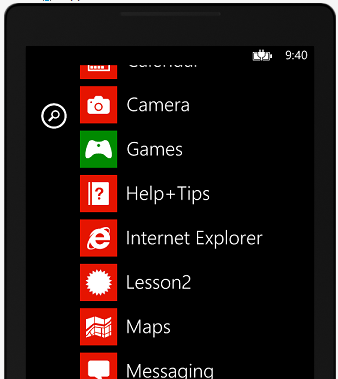
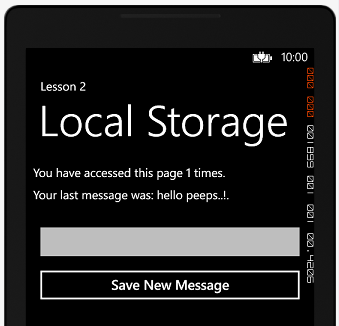
Beginning of Windows Phone 8 Development: lesson 2. Accessing local application storage
Beginning of Windows Phone 8 Development: lesson 3. Navigating through pages and passing parameters
Beginning of Windows Phone 8 Development: lesson 4. Communication with services and data binding.
And so, let's proceed to the second lesson. I hope it will be more interesting for you. Many developers for smartphones are interested in the issue of the availability of storage on the device for their applications, as well as its size and use. Each application has its own isolated local storage. It is independent of other applications and the OS, and only this application has access to it.
Two questions immediately arise: the ability to use an SD card and the size of available space. The answer to the first question is yes, you can use an SD card. The answer to the second question is unlimited. That is all the free space.
As for the application that we will create in this lesson, it will use local storage for reading / writing text messages and a counter. The counter will increase every time you start the application, and the last message you entered will also be displayed.
Appearance
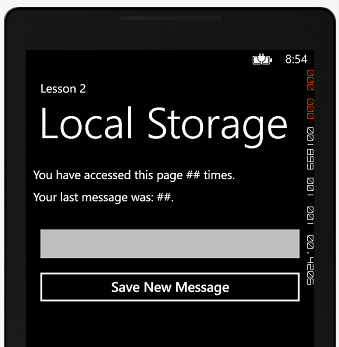
As you already noticed, two TextBlocks are used to display messages and a counter, and one TextBox is used to enter a new message. The layout, as always, uses XAML and my favorite StackPanel:
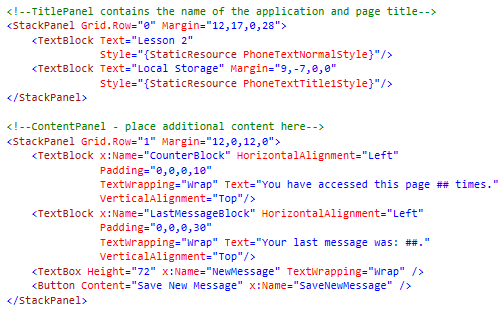
The code
This time for TextBlocks we set the Name properties, as we will transfer the contents to them from the outside. Now consider what happens when the page loads.
Page loading
Before adding logic, make sure that the necessary namespaces are added:
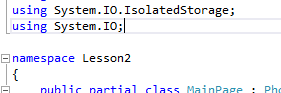
The code
using System.I0.IsolatedStorage;
using System.I0;
namespace Lesson2 {
....
When loading a page, first of all, you need to make sure that the file in which the counter is stored and the message exists. If it exists, then we read the necessary data and pass it to the global variable. If there is no file, then we write in the counter “0”, and in the messages “Empty” (No messages ..!) Of the new file. I will use a file called LS.txt (short for Local Storage).
Well, of course, I will pass the values to TextBlocks replacing the “##” characters in the string with the variable values using the string.Replace function.
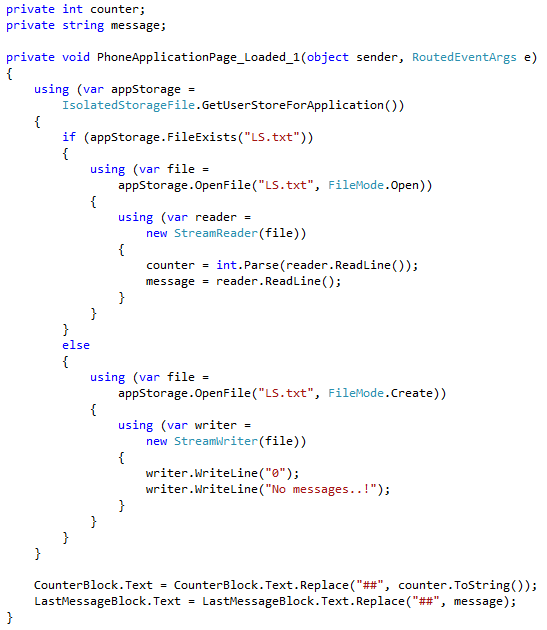
The code
private int counter;
private string message;
private void PhoneApplicationPage_Loaded_1(object sender, RoutedEventArgs e)
{
using (var appStorage = IsolatedStorageFile.GetUserStoreForApplication())
{
if (appStorage.FileExists("LS.txt"))
{
using (var file = appStorage.OpenFile("LS.txt", FileMode.Open))
{
using (var reader = new StreamReader(file))
{
counter = int.Parse(reader.ReadLine()); message = reader.ReadLine();
}
}
}
else
{
using (var file = appStorage.OpenFile("LS.txt", FileMode.Create))
{
using (var writer = new StreamWriter(file))
{
writer.WriteLine("0");
writer.WriteLine("No messages..!");
}
}
}
}
CounterBlock.Text=CounterBlock.Text.Replace("##",counter.ToString());
LastMessageBlock.Text = LastMessageBlock.Text.Replace("##", message);
}
Actions when closing the application
If the application closes when you click the Back or Start buttons, we replace the LS.txt file with a new one, after updating the data with the current ones. First we increase the counter, and then we record the message.

The code
protected override void OnNavigatedFrom(System.Windows.Navigation.NavigationEventArgs e)
{
base.OnNavigatedFrom(e);
using (var appStorage = IsolatedStorageFile.GetUserStoreForApplication())
{
using (var file = appStorage.OpenFile("LS.txt", FileMode.Create))
{
using (var writer = new StreamWriter(file))
{
writer.WriteLine((counter+1).ToString();
writer.WriteLine(message);
}
}
}
}
Well, at the end of everything, you need to save a new message and exit the application by raising the OnNavigatedFrom event.
Saving a new message
We pass the contents of the TextBox to a variable, and display a text message to the user about the successful save.
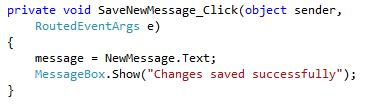
The code
private void SaveNewMessage_Click(object sender, RoutedEventArgs e)
{
message = NewMessage.Text;
MessageBox.Show("Changes saved successfully");
}
Testing
Start by pressing F5 and wait for the download. After loading, we see the counter shows 0, and there are no messages. We recruit new and save.
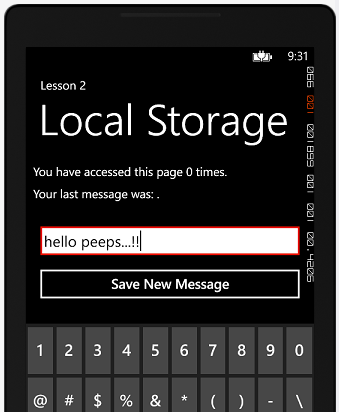
After clicking on Save we see a text message. After a successful save, the increment of the counter is called, and the application closes itself.
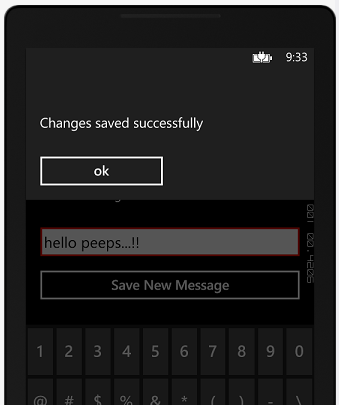
Now run it again. Everything works. Everything is being updated.
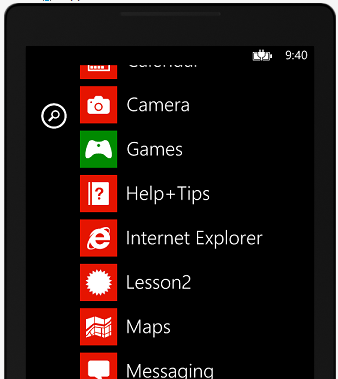
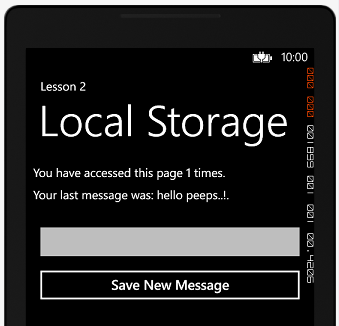