
Create a video library with PHPixie
- Tutorial

After my previous post about choosing a lightweight Framework, I googled and saw that for some of them there is not a single Russian-language tutorial. So I decided to write a series of posts in order to fill this niche. I think it’s worth starting with those that I stopped at the end, and since quite a lot has already been written for Silex, this post will be devoted to PHPixie.
For those who have not read my comparison of frameworks, PHPixie is a small and by my standards very fast framework with a modular structure, well-implemented DI, lack of statics and simple architecture.
On the official site, all the tutorials are written using the example of fairies, but I’ll try to be original and we will create a library of videos from Youtube. That is, we will be able to add, delete and view the video. We’ll also learn how to use the YouTube’s API to get meta information and tambnail on the way.
Project creation
PHPixie can be installed either using Composer, or by downloading a snapshot of the finished system from the site. There is almost no difference, since the snapshot is regularly updated and is a basic project with three libraries installed (core, db and orm). For simplicity, we will use the second method, the link to the snapshot is here phpixie.com/phpixie.zip . The server must be configured on the / web / folder inside the project. That's all, now we can go to our host and see a small greeting.
$ Pixie object
The $ pixie object is an implementation of the DI Container and the Locator Service, something similar to the $ app in Silex. It is available as a controller property and is passed to almost every constructor, $ pixie also contains factoring methods for each framework class. Proper use of it allows you to completely avoid class binding and therefore simplify testing. Unlike Silex, adding methods and properties to $ pixie only happens by extending the class. The base project already has the class App \ Pixie which inherits from PHPixie \ Pixie, just in it we can add our own code to have access to it everywhere. It should be noted that this is not a singleton and it is necessary to pass it between classes explicitly, this approach was chosen to avoid any global state in the code, similarly, the framework never uses static methods. Each module, for example db or orm supplies its own class with factor methods, these classes are written in our App \ Pixie in $ modules. Then we can request them as a property, for example, using $ pixie-> db-> query () and $ pixie-> orm-> get (), which introduces additional structure and allows you to avoid the soup of the methods. In our case, we do not need to change anything in App \ Pixie.
Database
We will use only one simple plate to store information about the video, here is its structure:
CREATE TABLE `videos` (
`id` INT(10) NOT NULL AUTO_INCREMENT,
`video_id` VARCHAR(20) NOT NULL,
`title` VARCHAR(255) NOT NULL,
PRIMARY KEY (`id`)
);
Now we prescribe the connection settings to the database in /assets/config/db.php , for example:
return array(
'default' => array(
'user'=>'root',
'password' => '',
'driver' => 'PDO',
'connection'=>'mysql:host=localhost;dbname=videos'
)
);
here, I think everything is quite trivial.
Model
Any MVC structure begins with a model, in our case it is only one, Video. Anyone familiar with Kohana will immediately feel at home; to create a model, simply expand the \ PHPixie \ ORM \ Model class :
namespace App\Model;
class Video extends \PHPixie\ORM\Model{}
The binding to the table occurs by default from the class name. In our case, it is so and nothing needs to be changed. Access to the model can be obtained using $ pixie. The most striking code examples:
//Создание и сохранение
$video = $pixie->get('video');
$video->title = 'Test';
$video->save();
//Поиск
$video = $pixie->get('video')->where('title','Test')->find();
//Поиск всех
$videos = $pixie->get('video')->find_all();
Basic markup
In the base project that we downloaded, there is a subspecies of the controller, class App \ Pixie. His work boils down to rendering the main.php template available as a property of $ view. Our controllers simply pass different data to this template, including $ subview - the name of the subpattern to be inserted. I really liked the fact that the logic of how the master pages and subpatterns work is not spelled out in the framework itself, but is taken out to the project itself, which allows you to remake it as you wish. Our main.php will look something like this:
We have only two subpatterns: list.php (for the list of added clips) and view.php (for viewing the selected video). Here they are:
Routing
I know that you have already been waiting for the code itself, but before we go to the controller we need to register it in /assets/config/routes.php. In fact, only one route is registered in the base project and it looks like / controller / method / id , which in principle suits us, but it would be very useful to change the default controller from Hello to our Videos.
return array(
'default' => array('(/(/(/)))', array(
// Дефолтные значения
'controller' => 'Videos',
'action' => 'index'
)
),
);
Controller
Since we need 3 pages in the controller there should be 3 methods. This is where we start:
namespace App\Controller;
class Videos extends \App\Page {
public function action_index(){
$this->view->subview = 'list';
$this->view->videos = $this->pixie->orm->get('video')->find_all();
}
public function action_add(){
$this->redirect('/');
//Об этом чуть позже
}
public function action_view(){
$this->view->subview = 'view';
//Берем id с URL: /video/view/3
$id = $this->request->param('id');
$this->view->video = $this->pixie->orm->get('video')
->where('id', $id)
->find();
}
}
The tasks of selecting all and one video are trivial.
Now consider adding a new video. The video identifier in the YouTube link is passed by the 'v' parameter, for example www.youtube.com/watch?v=75nBenOWul0 , we can get it in many ways, but it seemed to me the most accurate using parse_url (), like this:
parse_str(parse_url( $url, PHP_URL_QUERY ), $vars );
$video_id = $vars['v'];
You can get information about the video, in our case its name, using the API at youtube.com/get_video_info?video_id=
public function action_add(){
if($url = $this->request->post('url')){
parse_str(parse_url( $url, PHP_URL_QUERY ), $vars );
$video_id = $vars['v'];
$response = file_get_contents("http://youtube.com/get_video_info?video_id=".$video_id);
parse_str($response, $data);
$video = $this->pixie->orm->get('video');
$video->title = $data['title'];
$video->video_id = $video_id;
$video->save();
}
$this->redirect('/');
}
And voila! Here's a screenshot of the result by the way:
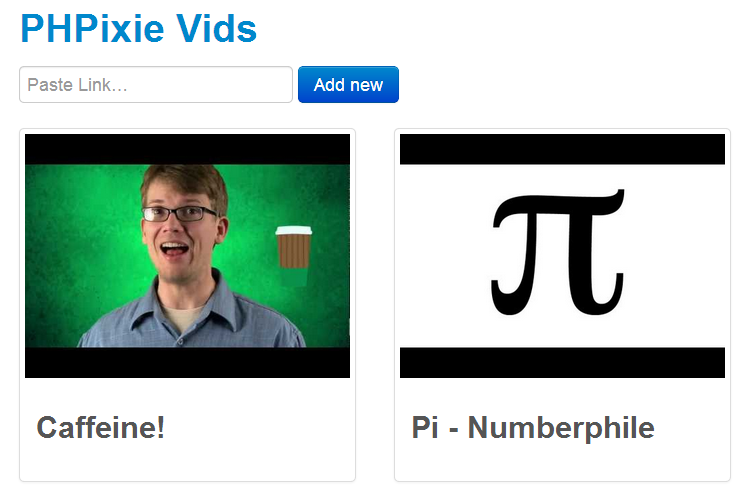
If you were expecting a more complex controller or configuration, then there are none, and the framework itself is almost not noticeable, this allows you to concentrate just on writing your own code instead of learning someone else's.
I hope you enjoyed the post, and another time we will learn to write on Lithium.