We work with SteamWorks. Part 1
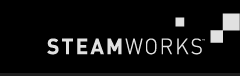
At the moment, access to SteamWorks can be obtained if you are a "game studio" and want to distribute your application in steam or through the Steam Greenlight service, thereby becoming a steam partner. Details here . Open SteamWorks is an open source Steam api implementation (a disassembled version of the steam libraries.)
“Open SteamWorks designed for people who know what they do” - Ryan Stecker. So the author of the open version answered the question (not mine) about the availability of additional documentation, all documentation that is available iscomments in source codes . You can download the latest version of Open SteamWorks from the site .
I will use Visual Studio, if you have mingw at hand, you can use it. Let's start creating a console application (you can use the test platform example from open steamworks).
We include the necessary header files.
#include
#include
We connect the necessary libraries to compile our application.
#pragma comment( lib, "../Resources/Libs/Win32/steamclient" )
#pragma comment( lib, "../Resources/Libs/Win32/steam" )
We get the Steam base with version 12 of the interface.
In a disassembled form, this compiles as a search in the registry for the path to the Steam and connection to it.
CSteamAPILoader loader;
auto *Client = (ISteamClient012 *)loader.GetSteam3Factory()(STEAMCLIENT_INTERFACE_VERSION_012, NULL);
Check if we were able to get the base.
if ( !Client )
{
printf("Unable to get ISteamClient.");
}
We create a pipe for interaction.
HSteamPipe pipe = Client->CreateSteamPipe();
Check the pipe.
if ( !pipe )
{
printf("Unable to get pipe");
}
We are connected to the global user (the launched Steam).
HSteamUser user = Client->ConnectToGlobalUser( pipe );
Checking connectivity to a global user.
if ( !user )
{
printf("Unable connect to global user");
}
We get access to api v 12 user interface.
auto *User = (ISteamUser012 *)Client->GetISteamUser( user, pipe, STEAMUSER_INTERFACE_VERSION_012);
Get access to api v.13 and v. 1 friends interface.
auto *Friends = (ISteamFriends001 *)Client->GetISteamFriends(user, pipe, STEAMFRIENDS_INTERFACE_VERSION_001);
auto *Friends13 = (ISteamFriends013 *)Client->GetISteamFriends(user, pipe, STEAMFRIENDS_INTERFACE_VERSION_013);
I caution those who will use IClient interfaces (IClientUtils, IClientFriends, etc.), all these interfaces stop working immediately after the global steam update, your application will throw errors, use ISteam (stable) described above, if you really need to use functions from IClient interfaces, and this sometimes happens, copy all the important DLL files for your application (you can see it in the import table) and keep it in the folder with your application.
Now we will try to do the simplest thing, change the status in the incentive. (api for changing status is available in the first version of the interface)
Friends->SetPersonaState(k_EPersonaStateSnooze);
We compile, run and now we have the status of sleeping in the incentive.
We get the number of friends.
int friendcount = Friends->GetFriendCount();
printf("%d",friendcount);
Change your name
Friends->SetPersonaName("Big_balls");
We sort through our friends, get the SteamID (64bits) structure of the current friend by index, check the status of the current friend, if the status is online and the name of the friend Crey, send him a message.
for(int i = 1; i < friendcount + 1; i++)
{
CSteamID thisfriend = Friends->GetFriendByIndex(i);
if(Friends->GetFriendPersonaState(thisfriend) == k_EPersonaStateOnline && strstr(Friends- >GetFriendPersonaName(thisfriend),"Crey") != 0)
{
char myMsg[] = "My friend Crey is online.";
Friends->SendMsgToFriend(thisfriend, k_EChatEntryTypeEmote,myMsg,strlen(myMsg)+1);
}
I think now everyone understands that with the help of SteamWorks you can easily write a trade bot to exchange things at a “given rate” and a lot of interesting things.
In the following parts, we will consider working with IScreenshots, IUserstats (achievements), callbacks, and maybe it comes to writing a bot.