Blockchain Derivatives: Solving the $ 500 Trillion Problem and Winning the Consensus 2018 Hackathon
On May 11 and 12, 2018, the 8base team, represented by Evgeny Semushin, Gabe Kelly, and I decided to test my strength and fight for the AlphaPoint prize on the Hackathon Consensus 2018 , which was held in New York. A total of 33 teams participated in the hackathon, and among its sponsors were such giants as CoinDesk, Microsoft, IBM, Hyperledger and Quorum.
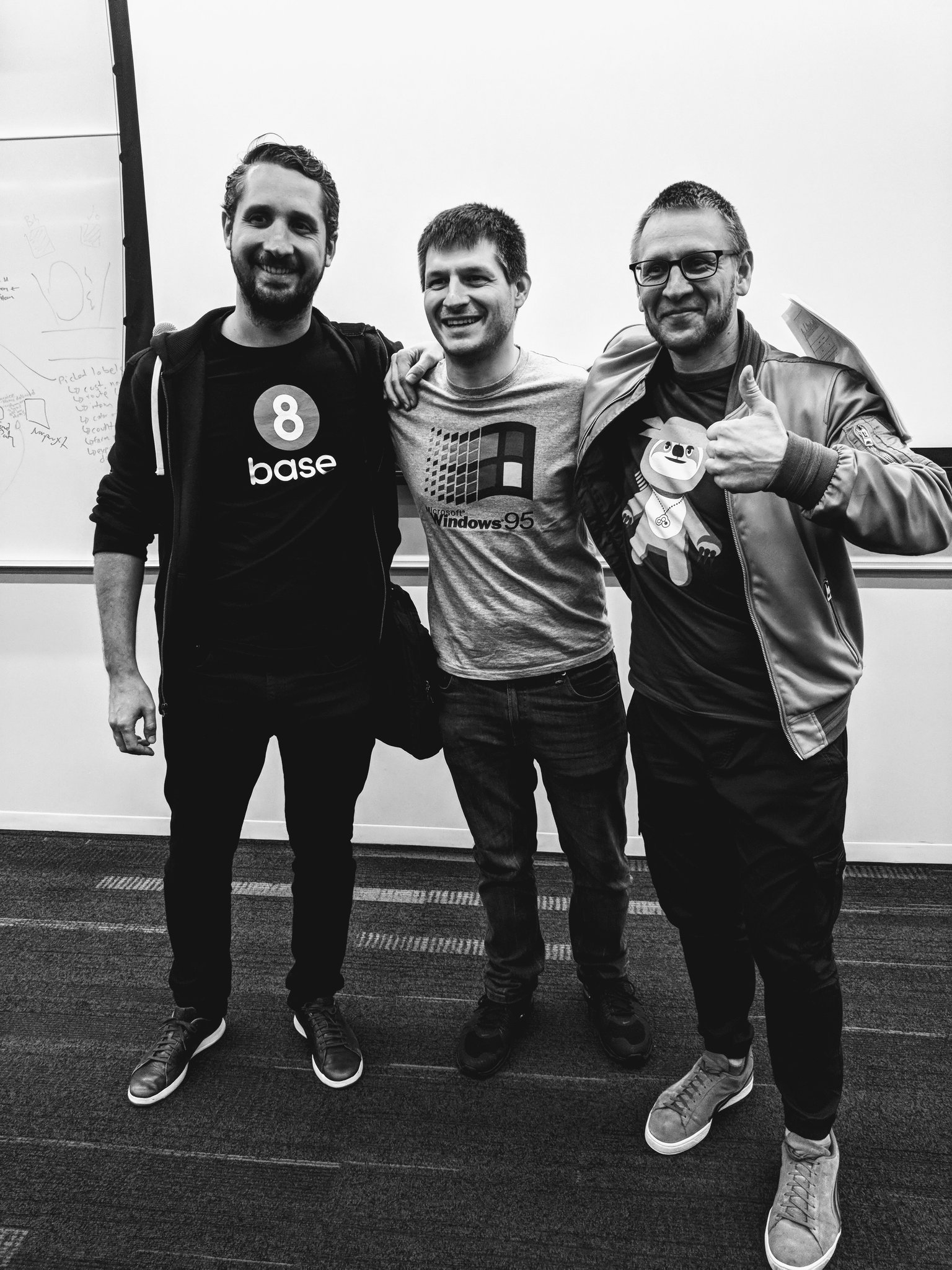
At the AlphaPoint competition, it was necessary to write an application tied to a cryptocurrency exchange, so we hoped that my experience in the hedge fund would be very helpful. We wanted to create something more than a front end to an existing blockchain solution. Our goal was to solve the real problem by applying the unique features of the blockchain architecture, our own experience in developing smart contracts and knowledge of the internal mechanics of finance.
We have created a set of smart contracts on the Ethereum network, which allows us to form decentralized derivatives and manage their software on a blockchain basis. With our solution, any user can become a guarantor for a contract for the sale or purchase of any ERC20 asset without any external management of requirements and their execution. As a result, we won the main prize.
Under the cut the nuances of the implementation of this project.
Derivatives - a serious thing. The capitalization of the global derivatives market is estimated at almost $ 500 trillion. If we compare this with the cryptocurrency market (about $ 0.4 trillion), the amount of cash (about $ 40 trillion) and the global stock market (about $ 70 trillion), then it becomes obvious how important are the derivatives in the global economy. Just look at this infographic . Banks, funds and companies are actively using options, futures, swaps, and other derivatives to manage risk.

Market capitalization of various assets:
The main difficulty with contracts for derivatives is related to the fact that they usually use leverage, since the potential risks are higher than the sums the parties actually have. Because of this, a complex system of settlements arises, as well as the requirements for the minimum margin and requests for increasing the margin: the level of security must remain such that exit from a transaction is always more expensive than its execution.
All this collapsed in 2008. Entangled in the intricacies of derivatives contracts, Wall Street players lost control of the situation. As a result, the domino effect worked: a flurry of requests for increasing margins and the inability to execute them led to the collapse of Lehman Brothers and other players. The US government had to intervene and act as a guarantor of Wall Street for gigantic amounts of unfulfilled derivatives contracts. We all know what this led to ...

BlockSigma is a set of smart contracts for organizing cryptocurrency derivatives transactions and managing such transactions. Collateral management is based on the blockchain, therefore, in order to control the compliance with the minimum margin requirements for funds fixed in a smart contract, no intermediary is required.
The main innovation is that the margin management is completely based on the blockchain, without centralized control and the predictive flow of quotes. To do this, we integrated the Bancor protocol.. The Bancor protocol is already being actively introduced into exchange trading and daily services operations for millions of dollars. Bancor uses a deterministic price function based on the ratio of supply and demand for a particular asset. Accordingly, the price of the basic token can be determined directly in the blockchain, which allows us to accurately formulate the requirements for the minimum margin and create requests to increase the margin in time.
The logic of smart contracts is structured so that the option buyer forcibly closes the position as soon as the security in the smart contract falls below the mandatory minimum margin requirement. The function of determining the minimum margin takes into account the excess reserve, which creates economic incentives for the option buyer to closely monitor the position and get this reserve (along with the excess) when it is forcibly closed in case of violation of the minimum margin requirement. In turn, the seller (guarantor) of the option also has economic incentives to replenish collateral on time if the transaction fails so as not to lose the excess reserve.

The complete solution source code is published in the GitHub repository .
The solution consists of three main Solidity files: BlockSigmaBase.sol, BlockSigmaCall.sol, and BlockSigmaPut.sol. BlockSigmaBase implements common logic, and BlockSigmaCall and BlockSigmaPut inherit it and implements the logic of purchase and sale contracts, respectively.
Here is the contract interface:
Now consider the main business processes for a sales contract. Take, for example, the contract for the sale of EOS tokens denominated in DAI, which allows you to effectively hedge assets in EOS against the US dollar. The life cycle of such a contract will be as follows:
Now consider how collateral management is implemented. In our example, the price of an EOS at the time of issuing an option contract was $ 12. Suppose it dropped to $ 9. Then the required reserve will be equal to 2 + max (0, 10 - 9) = 3. This means that the seller must additionally deposit 1 DAI for each contract that he acts as a guarantor. If the seller cannot do this, then the buyer will have an economic incentive to forcibly close the position:
This motivates the buyer to forcibly close positions as early as possible in order to quickly make a profit.
This is by no means a ready-made solution. His main goal is to show the breakthrough capabilities of the blockchain and smart contracts for automating mutual settlements and managing the provision of option contracts. Traditionally, these services are provided by intermediaries, and also require manual work and the payment of commissions.
Although our solution is very promising in this regard, we see a number of serious difficulties:
If you notice any problems that are not listed, write about them in the comments.
The solution described in general terms reflects the fundamental changes that the blockchain will bring to the financial industry over the coming years. In addition to more efficient settlements and collateral management, this technology will introduce transparent, self-executing and uniform rules for all market participants.
Our 8base team does not specialize in niche solutions and protocols described in the article. We expect explosive growth of similar revolutionary technologies in the coming years in the wake of digital transformation. Accordingly, we focus on building the next level of infrastructure in order to give business users easy access to these technologies and the ability to integrate them into enterprise software to achieve business goals.
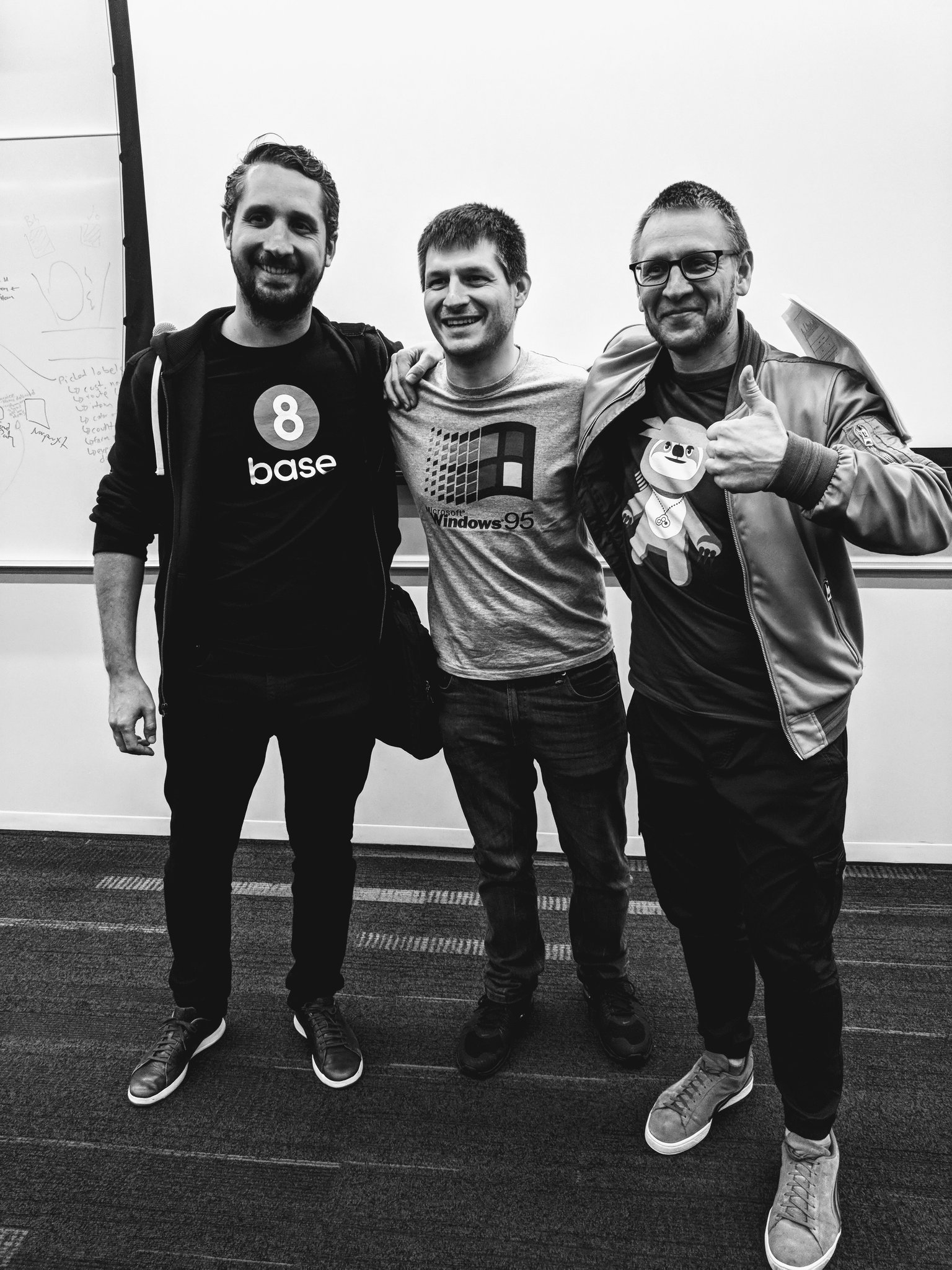
At the AlphaPoint competition, it was necessary to write an application tied to a cryptocurrency exchange, so we hoped that my experience in the hedge fund would be very helpful. We wanted to create something more than a front end to an existing blockchain solution. Our goal was to solve the real problem by applying the unique features of the blockchain architecture, our own experience in developing smart contracts and knowledge of the internal mechanics of finance.
We have created a set of smart contracts on the Ethereum network, which allows us to form decentralized derivatives and manage their software on a blockchain basis. With our solution, any user can become a guarantor for a contract for the sale or purchase of any ERC20 asset without any external management of requirements and their execution. As a result, we won the main prize.
Under the cut the nuances of the implementation of this project.
Problem
Derivatives - a serious thing. The capitalization of the global derivatives market is estimated at almost $ 500 trillion. If we compare this with the cryptocurrency market (about $ 0.4 trillion), the amount of cash (about $ 40 trillion) and the global stock market (about $ 70 trillion), then it becomes obvious how important are the derivatives in the global economy. Just look at this infographic . Banks, funds and companies are actively using options, futures, swaps, and other derivatives to manage risk.

Market capitalization of various assets:
- Crypto - cryptocurrency
- Liquid money - cash
- Global public stock markets - global stock market
- Derivatives - derivatives
The main difficulty with contracts for derivatives is related to the fact that they usually use leverage, since the potential risks are higher than the sums the parties actually have. Because of this, a complex system of settlements arises, as well as the requirements for the minimum margin and requests for increasing the margin: the level of security must remain such that exit from a transaction is always more expensive than its execution.
All this collapsed in 2008. Entangled in the intricacies of derivatives contracts, Wall Street players lost control of the situation. As a result, the domino effect worked: a flurry of requests for increasing margins and the inability to execute them led to the collapse of Lehman Brothers and other players. The US government had to intervene and act as a guarantor of Wall Street for gigantic amounts of unfulfilled derivatives contracts. We all know what this led to ...
Decision

BlockSigma is a set of smart contracts for organizing cryptocurrency derivatives transactions and managing such transactions. Collateral management is based on the blockchain, therefore, in order to control the compliance with the minimum margin requirements for funds fixed in a smart contract, no intermediary is required.
The main innovation is that the margin management is completely based on the blockchain, without centralized control and the predictive flow of quotes. To do this, we integrated the Bancor protocol.. The Bancor protocol is already being actively introduced into exchange trading and daily services operations for millions of dollars. Bancor uses a deterministic price function based on the ratio of supply and demand for a particular asset. Accordingly, the price of the basic token can be determined directly in the blockchain, which allows us to accurately formulate the requirements for the minimum margin and create requests to increase the margin in time.
The logic of smart contracts is structured so that the option buyer forcibly closes the position as soon as the security in the smart contract falls below the mandatory minimum margin requirement. The function of determining the minimum margin takes into account the excess reserve, which creates economic incentives for the option buyer to closely monitor the position and get this reserve (along with the excess) when it is forcibly closed in case of violation of the minimum margin requirement. In turn, the seller (guarantor) of the option also has economic incentives to replenish collateral on time if the transaction fails so as not to lose the excess reserve.
Implementation

The complete solution source code is published in the GitHub repository .
The solution consists of three main Solidity files: BlockSigmaBase.sol, BlockSigmaCall.sol, and BlockSigmaPut.sol. BlockSigmaBase implements common logic, and BlockSigmaCall and BlockSigmaPut inherit it and implements the logic of purchase and sale contracts, respectively.
Here is the contract interface:
contract BlockSigmaBase is StandardToken {
/**
* @dev Constructor
* @param _underlyingTokenAddress address of the underlying ERC20 token.
* @param _currencyTokenAddress address of the currency ERC20 token.
* @param _strike option strike denominated in the currency token.
* @param _exp expiration timestamp.
* @param _minReserve minimum (excess) reserve
* @param _underlyingBancorConverter address of the Bancor converter contract that converts underlying token into BNT.
* @param _currencyBancorConverter address of the Bancor converter contract that converts currency token into BNT.
* @param _issuer address that is allowed to issue(underwrite) new contracts.
*/functionBlockSigmaBase(address _underlyingTokenAddress, address _currencyTokenAddress,
uint256 _strike, uint256 _exp, uint256 _minReserve,
address _underlyingBancorConverter, address _currencyBancorConverter,
address _issuer)public;
/**
* @dev get the required maintenance margin level in currency token.
*/functiongetRequiredReserve()publicviewreturns(uint256);
/**
* @dev Get price of the underlying token expressed in currency token from Bancor
*/functiongetUnderlyingPrice()publicviewreturns(uint256);
/**
* @dev Ability for writer to issue(underwrite) new contracts
* @param amount how many contracts to issue (in wei).
*/functionissue(uint256 amount)publiconlyIssuerreturns(bool);
/**
* @dev Buyer can exercise the option anytime before the maturity. Buyer deposits currency(call) or underlying tokens(put).
*/functionexercise()publicreturns(bool);
/**
* @dev Writer delivers the underlying tokens(call) or currency(put).
* @param to which buyer to deliver to.
*/functiondeliver(address to)publiconlyIssuerreturns(bool);
/**
* @dev Buyer can force liquidation if exercise is past due or reserve is below requirement
*/functionforceLiquidate()publicreturns(bool);
/**
* @dev Writer can deposit additional reserve
* @param amount how much currency token to deposit.
*/functiondepositReserve(uint256 amount)publicreturns(bool);
/**
* @dev Writer can withdraw reserve
* @param amount how much currency token to withdraw.
*/functionwithdrawReserve(uint256 amount)publiconlyIssuerreturns(bool);
/**
* @dev Is reserve below the requirement?
*/functionisReserveLow()publicviewreturns(bool);
/**
* @dev Is option series expired?
*/functionisExpired()publicviewreturns(bool);
/**
* @dev Can option be liquidated?
*/functioncanLiquidate()publicviewreturns(bool);
}
Now consider the main business processes for a sales contract. Take, for example, the contract for the sale of EOS tokens denominated in DAI, which allows you to effectively hedge assets in EOS against the US dollar. The life cycle of such a contract will be as follows:
- Any user can publish a BlockSigmaPut contract for a specific issue of options . When publishing, you must specify: (1) basic token, (2) currency token, (3) expiration date, (4) strike, (5) address of the issuer authorized to act as guarantor for contracts in this issue and (6) minimum reserve requirement under the contract. Example: EOS sale until July 1, 2018 with a strike of 10 DAI and a minimum reserve of 2 DAI. Such a contract gives the buyer the right to sell the EOS to the guarantor for 10 DAI at any time before July 1, 2018. The requirement for the minimum margin for each contract in this case will be 2 + max (0, 10 - the current price of EOS).
- The specified issuer acts as a guarantor for a certain number of contracts, placing the necessary reserve in a currency token. For the example above, with an EOS current market price of $ 12, the issuer must place 2 + max (0, 10 - 12) = 2 DAI to issue 1 contract for an option. Issued contracts will be considered as ordinary ERC20 tokens, which will allow them to be listed on the exchange for sale.
- A buyer who wants to execute a contract must give permission to transfer the EOS into a smart option contract and then call the exercise method. After the start of the contract, the seller will have 24 hours to transfer the payment in the currency token. In our example, the seller must give 10 DAI for each EOS token.
- The issuer gives permission to transfer DAI tokens from his account to the smart option contract and calls the deliver method. The executed smart contract automatically settles the transaction: transfers DAI and EOS to the buyer and the seller (issuer), respectively, and the reserve returns to the seller.
- (Optional) If the seller did not make the payment within 24 hours, the buyer can call the forceLiquidate method to return the hosted EOS along with a reserve to cover the incorrect actions of the seller.
Now consider how collateral management is implemented. In our example, the price of an EOS at the time of issuing an option contract was $ 12. Suppose it dropped to $ 9. Then the required reserve will be equal to 2 + max (0, 10 - 9) = 3. This means that the seller must additionally deposit 1 DAI for each contract that he acts as a guarantor. If the seller cannot do this, then the buyer will have an economic incentive to forcibly close the position:
- If the buyer executes the option, he will receive a payment of 10 - 9 = 1 DAI for each contract (that is, he will buy EOS on the market for $ 9 and sell it to the issuer for $ 10).
- On the other hand, if the buyer forcibly closes the position, he will receive the entire reserve, which for our example amounts to 2 DAI for each contract.
This motivates the buyer to forcibly close positions as early as possible in order to quickly make a profit.
Difficulties
This is by no means a ready-made solution. His main goal is to show the breakthrough capabilities of the blockchain and smart contracts for automating mutual settlements and managing the provision of option contracts. Traditionally, these services are provided by intermediaries, and also require manual work and the payment of commissions.
Although our solution is very promising in this regard, we see a number of serious difficulties:
- Bancor protocol has not been tested under stringent conditions. It uses a deterministic price function, converging to stock prices through arbitration. So far, it is not clear how this mechanism will work with much greater volatility and / or with serious price gaps.
- The mechanism for managing collateral is not sensitive to price gaps. Theoretically, a situation is possible in which the liability of the guarantor exceeds the reserve, which is why the guarantor will want to break the deal, and the buyer will not receive full compensation. To avoid this, you can increase the minimum reserve. In addition, you can do the traditional way: users of the system contribute funds to the distributed “insurance pool”, which automatically covers shortages in exceptional cases.
- Since Bancor uses a deterministic price function, various manipulations are possible to increase payments to one side of an option contract to the detriment of the other side. This can happen only with a small reserve of the Bancor converter (i.e., at a high price sensitivity) relative to the sum of the options positions. In this case, the manipulation of the price in Bancor may be more profitable than the payment on options as a result of such manipulation. However, this problem is typical not only for cryptocurrency, but also for traditional markets (for example, arson at home for the sake of getting insurance).
- Uncertainty in regulation. We have not studied the aspects of regulation, since so far our project has purely research objectives.
If you notice any problems that are not listed, write about them in the comments.
findings
The solution described in general terms reflects the fundamental changes that the blockchain will bring to the financial industry over the coming years. In addition to more efficient settlements and collateral management, this technology will introduce transparent, self-executing and uniform rules for all market participants.
Our 8base team does not specialize in niche solutions and protocols described in the article. We expect explosive growth of similar revolutionary technologies in the coming years in the wake of digital transformation. Accordingly, we focus on building the next level of infrastructure in order to give business users easy access to these technologies and the ability to integrate them into enterprise software to achieve business goals.
Sources on GitHub