We talk with the Rails application through XMPP (Jabber)
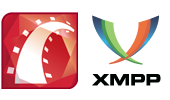
The article will tell you how to receive messages from your Rails application using the XMPP protocol (Jabber) and vice versa, manage the application by sending commands through XMPP.
Bit of theory
XMPP (Extensible Messaging and Presence Protocol), formerly known as Jabber, is an XML-based, open, free-to-use protocol for instant messaging in near real-time mode. Originally designed to be easily extensible, the protocol, in addition to sending text messages, supports the transfer of voice, video and files over the network.
Unlike commercial instant messaging systems such as AIM, ICQ, WLM and Yahoo, XMPP is a decentralized, extensible, and open system. Anyone can open their instant messaging server, register users on it and interact with other XMPP servers. Based on the XMPP protocol, many private and corporate XMPP servers are already open. Among them there are quite large projects, such as Facebook, Google Talk, Yandex, VKontakte and LiveJournal.
If you don’t have a jabber account yet (I’ll call XMPP jabber in the old way), you can quickly get it on Jabber.ru or use Google Talk . There will be no problems with the client for the jabber, almost any “ace”, for example, Miranda, will do.or qip .
What is it for?
I use message sending:
- To search for broken links. When a user on my site gets to a 404 page, I get a message with the address of this page and the address of the page from which this transition was.
- For instant information about the order of goods, payment, etc.
- To receive messages from users who do not require a response. For example, when filling out the “wish form”.
I give the commands to the Rails application:
- To reload the application. This is much faster than climbing the server via ssh and reloading the rails with a long command.
- To add / remove rows in the database. I was too lazy to write a full-fledged interface for working with a database, which very rarely needs to add / remove several records.
Well, you can use the Rails + XMPP bundle for these and any bolder tasks. For example, uploading files and other content to the server, chats with users, etc.
What do we need?
We will need XMPP4R gem . Install:
gem install xmpp4r
Immediately, I note that the gem behaves very unstably under windows, so it is better to work with it on Linux.
Next, we need a jabber account for our Rails application, i.e. The address from which it will send you messages and receive commands from you. Register an account.
Sending messages
Connection and authentication of the robot (I will call the part of our Rails application responsible for communication).
require "xmpp4r" robot = Jabber::Client::new(Jabber::JID::new("robot@xmpp.ru")) robot.connect robot.auth("password")
I think everything is clear here, robot@xmpp.ru is the address of the jabber account that you registered for the application, password is the password to it. Now send the message.
message = Jabber::Message::new("you@xmpp.ru", "Хелло, ворлд!") message.set_type(:chat) robot.send message
Where you@xmpp.ru is your jabber address. That's all. A message has already popped up from your tray.
Feedback
Now the most interesting part is that we will send commands to the robot (via the jabber client). To do this, first connect the robot and make it declare its presence and willingness to communicate. Those. the robot will be online, the green light in the jabber client will be on.
robot = Jabber::Client::new(Jabber::JID::new("robot@xmpp.ru")) robot.connect robot.auth("password") robot.send(Jabber::Presence.new.set_show(nil))
Now, enable the waiting cycle for incoming messages. At the same time, we will verify that messages come from the correct address, i.e. some intruder is not trying to command the robot.
robot.add_message_callback do |message| #ожидание сообщения if message.from.to_s.scan("you@xmpp.ru").count > 0 #сообщение с правильного адреса case message.body #перебираем варианты команд для робота when "hello" message = Jabber::Message::new("you@xmpp.ru", "И тебе привет!") message.set_type(:chat) robot.send message when "restart" File.open(Rails.root.to_s + "/tmp/restart.txt", "a+") end else #сообщение с чужого адреса message = Jabber::Message::new("you@xmpp.ru", "Ко мне ломится незнакомец!") message.set_type(:chat) robot.send message end end
After receiving the message “hello” , the robot will say hello to us, and when it receives “restart” , it will restart the Rails application (if it works through Passenger).
Final class
In the previous example, the robot received a command from us to restart the application and ... died. It needs to be revived again by running the appropriate script. Of course, I do not want to do this every time after restarting the application. Therefore, I propose to launch the robot with the application. I wrote a daemon that includes a class that simplifies working with XMPP, and also allows the robot to live regardless of reboots.
We will arrange this class in a separate ruby file, for example, “xmpp_daemon.rb” , and put it in the “config / initializers” folder , which will allow it to run along with the rails.
#coding: utf-8 require "xmpp4r" class Xmpp #параметры подключения @@my_xmpp_address = "you@xmpp.ru" #ваш джаббер-адрес @@robot_xmpp_address = "robot@xmpp.ru" #джаббер-адрес робота @@robot_xmpp_password = "password" #пароль от джаббер-аккаунта робота @@site_name = "sitename.ru" #имя сайта, для идентификации источника сообщений #подключение и аутентификация на xmpp-сервере def self.connect @@robot = Jabber::Client::new(Jabber::JID::new(@@robot_xmpp_address)) @@robot.connect @@robot.auth(@@robot_xmpp_password) end #отправка сообщения def self.message(text) self.connect message = Jabber::Message::new(@@my_xmpp_address, "[#{@@site_name}]\n#{text}") message.set_type(:chat) @@robot.send message end #прием сообщений def self.listen self.connect @@robot.send(Jabber::Presence.new.set_show(nil)) @@robot.add_message_callback do |message| #ожидание сообщения if message.from.to_s.scan(@@my_xmpp_address).count > 0 #сообщение с правильного адреса case message.body #перебираем варианты команд для робота when "hello" Xmpp.message "И тебе привет!" when "restart" Xmpp.message "Перезагрузка..." File.open(Rails.root.to_s + "/tmp/restart.txt", "a+") end else #сообщение с чужого адреса Xmpp.message "Ко мне ломится незнакомец!" end end end end Xmpp.listen
Now, from any Rails application controller, we can easily send a message.
Xmpp.message "Хелло, ворлд!"
Thank you for your attention, I hope this is useful to someone.
Manageru - Zakgo - Enspe