
React Hook Router A Modern React Router Alternative

Free translation of How React Hooks can replace React Router .
Posted by Peter Ekene Eze.
Since the advent of React Hooks, a lot has changed. The opportunities provided by hooks allowed us to reconsider our approach to certain concepts in React, including routing.
This post is in no way intended to be written off or downplayed by React Router. We will explore other possibilities and see how we can improve routing with React applications using hooks.
To this end, we compare React Router and hooksrouter with illustrative examples. First, let's take a closer look at the React Router.
React router
React Router is a popular declarative way to manage routes in React applications. It reduces the amount of work involved in manually configuring routes for all pages and screens in the application. React Router provides us with three main components that help us do routing - Route, Link and BrowserRouter.
Routing in React Router
Imagine we are creating a three-page React application. Usually we use a React Router for routing and write something like this:
import Users from "./components/Users";
import Contact from "./components/Contact";
import About from "./components/About";
function App() {
return (
);
}
Component
Hooks as Alternative Routing
The hookrouter module exports the useRoutes () hook, which checks the predefined route object and returns the result. In the routes object, you define your routes as keys, and their values as functions that will be called when routes match. Example code on hooks:
import Users from "./components/Users";
import Contact from "./components/Contact";
import About from "./components/About";
const Routes = {
"/": () => ,
"/about": () => ,
"/contact": () =>
};
export default Routes;
This way of writing routes is more attractive. Why? Because we did not need to do a lot of work. With React Router we had to render the component
import {useRoutes} from 'hookrouter';
import Routes from './router'
function App() {
const routeResult = useRoutes(Routes)
return routeResult
}
This gives us exactly the same result we would get using a React Router, but with a cleaner and easier implementation.
React Router Navigation
React Router includes a component that helps us customize application navigation and manage interactive routing. We have an application with three pages, let's display them on the screen and move on to them when pressed:
import { Route, Link, BrowserRouter as Router } from "react-router-dom";
import Users from "./components/Users";
import Contact from "./components/Contact";
import About from "./components/About";
function App() {
return (
- About
- Users
- Contact
);
}
We created the navigation that is needed to go from one page of the application to another. Here is a good example of how this works.

We use hooks for navigation
The hookrouter module provides a wrapper over an HTML tag . It is available as a react component and is 100% compatible with the native tag . The only difference is that it moves the navigation onto the history stack instead of actually loading a new page.
const routes = {
"/user": () => ,
"/about": () => ,
"/contact": () =>
};
function App() {
const routeResult = useRoutes(routes);
return (
);
}

Software navigation
The hookrouter module gives us access to the navigate () hook, to which we can pass the URL, and it will redirect the user to this URL. Each call to the navigate () function represents a forward navigation, as a result, users can click the back button in the browser to return to the previous URL. This happens by default.
navigate('/user/');
However, if you need a different behavior, you can make a replacement in your browser history. The navigate () hook accepts three parameters - navigate (url, [replace], [queryParams]), the second parameter is used to change the replacement behavior. It deletes the current history entry and replaces it with a new one. To achieve this effect, simply set its argument to true.
navigate('/user', true);
React router switch
React Router Uses Component
import { Route, Link, BrowserRouter as Router, Switch } from "react-router-dom";
import Users from "./components/Users";
import Contact from "./components/Contact";
import Home from "./components/About";
import NoPageFound from "./components/NoPageFound.js";
function App() {
return (
- Home
- Users
- Contact
);
}
Thus, when switching to an undefined route, the React Router displays the NoPageFound component. In this way, we can inform users about where they are and what happens while navigating the application.
Alternative switch on hooks
Since we define a route object that contains all our routes, and simply pass this object to the useRoutes () hook, it becomes very easy to display routes by condition. If we define the default NoPageFound component for rendering when the selected route is undefined, we only need to pass this file for rendering along with our result function as follows:
import { useRoutes, A } from "hookrouter";
import routes from "./router";
import NoPageFound from "./components/NoPageFound";
function App() {
const routeResult = useRoutes(routes);
return (
);
}
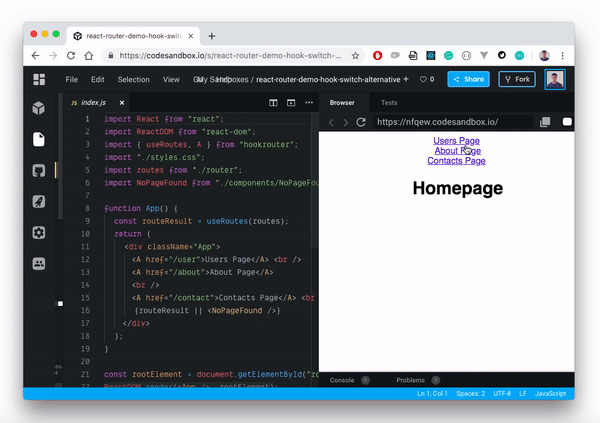
Compared to using the component in React Router to render pages by default, it looks a bit cleaner and more readable.
React Router Redirects
A redirect occurs when we want to dynamically direct a user from one route to another. For example, during authorization, when a user successfully logs in, we would like to redirect him from the route '/ login' to the route '/ dashboard'.
With React Router, we can do this in several ways - using a history object or component
import React from 'react'
class Login extends React.Component {
loginUser = () => {
// if (user is logged in successfully)
this.props.history.push('/dashboard')
}
render() {
return (
)
}
}
export default Login
Hook redirects
The hookrouter module provides us with a useRedirect () hook, which can take the source route and the target route as parameters.
useRedirect('/user', '/dashboard');
This will automatically redirect users to the '/ dashboard' route when the '/ user' path is found. For example, if we don’t want to show any users, but instead automatically redirect the user to his / dashboard, we will write it like this:
import {useRoutes, useRedirect} from 'hookrouter';
import dashboard from "./components/Dashboard";
const routes = {
'/home': () => ,
'/dashboard': () =>
};
const Users = () => {
useRedirect('/user', '/dashboard');
const routeResult = useRoutes(routes);
return routeResult
}

It is worth noting that the useRedirect () hook causes a replacement of the navigation history. As a result, there will be only one entry in the navigation history. This means that if the redirection occurs from '/ user' to '/ dashboard, as was the case in the last code fragment under consideration, the route' / user 'will not be displayed in the browsing history. We will only have the '/ dashboard' route.
Handling URL Parameters in React Router
URL parameters help us display components based on their dynamic URLs. This works similarly with nested routes, but in this case, the routes do not change, but are updated.
For example, if there were different users in our application, it would make sense to identify them separately with their individual routes, such as 'user / user1 /' and 'users / user2 /', etc. To do this, we need to use the URL parameters. In React Router, we simply pass a parameter (e.g. id) starting with a colon in the path property of the component
Using hooks to process URL parameters
There are practically no differences in the handling of URL parameters in the hookrouter and React Router. The design is the same (i.e., you can pass URL parameters to target routes using a colon and parameter name).
However, there is a difference in how the hookrouter works. It reads all URL parameters and places them in the object. This is done using the keys that you defined in the routes object. Then all the named parameters will be redirected to the result function of your route as a combined object.
const routes = {
'/user/:id': ({id}) =>
}
Using the destructuring of the object, we simply take the id property from the props object and then apply it to our component. Thus, we get exactly the same result as with React Router.
Conclusion
We looked at an alternative routing method in React applications. React Router is a great tool, however, with the advent of hooks in React, a lot has changed, including how routing works. This hook-based module offers a more flexible and clean way to handle routes in small projects.
In addition to the main functionality discussed in the post, hookrouter has a number of additional features, such as:
- Lazy loading components
- Nested Routes
- Transferring additional data to the route
- Navigation interception
- Server Rendering Support
The hookrouter project has excellent documentation in which you can quickly find a question of interest.
The article used materials from the documentation for the hookrouter
and the original article How React Hooks can replace React Router .
Git repository hookrouter
UPD: when writing an article, I did not immediately see that you can choose the type of publication "Translation", I apologize to readers, put a link to the original in the header and indicated the author.