IR remote control on stm32
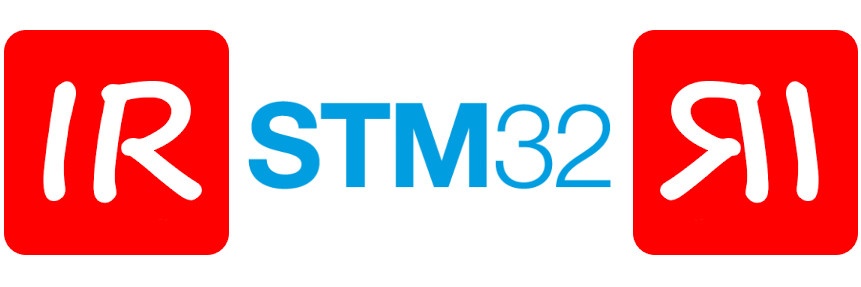
Hello.
Description of the library for reading, decoding and subsequent sending of infrared signals from various household remotes using the stm32 microcontroller. Based on the IRremote library for arduino, and adapted for stm32.
The library uses one timer, both for receiving and for sending a signal. The receiver is connected to any pin (GPIO_Input), and the transmitter is connected to one of the channels of the timer operating in PWM Generation (PWM) mode. In the example, the first channel of timer No. 4 is used - PB6 (transmitter) and pin PB5 (receiver).
To receive a signal, the timer operates in interrupt mode - every 50 μs it checks the status of the input pin, and during transmission it switches to PWM Generation mode, sends a signal, and switches back to interrupt mode.
Settings are in the file IRremote.h
extern TIM_HandleTypeDef htim4;
// настройка таймера для приема - переполнение каждые 50 мкс (в данном случае системная частота 72Мгц)
#define MYPRESCALER 71 // получаем частоту 1МГц
#define MYPERIOD 49 // 50 мкс
// настройка таймера для отправки - указать системную частоту таймера
#define MYSYSCLOCK 72000000
// настройка пина для приёма recive_IR
#define RECIV_PIN (HAL_GPIO_ReadPin(recive_IR_GPIO_Port, recive_IR_Pin))
If you will configure another timer, you need to specify the corresponding structure name - htim4, and do the same in the IRremote.с and irSend.с files. I was too lazy to define all this economy. When choosing another timer in Cuba, you need to specify only the channel and the internal clock source ... The

program will configure the rest itself. If you select a different channel number, then you also need to rename it in the irSend.s file.
With the rest of the settings, I think everything is clear - based on the system frequency (in the example of 72 MHz), the values of the pre-splitter and overflow are substituted for interrupt every 50 μs. The following indicates the frequency and reading of the pin.
Below are the defines that define the protocols involved ...
//////////////////////////////////// активированные протоколы ////////////////////////////////////////
#define DECODE_RC5 1 // чтоб отключить декодирование протокола RC5 нужно указать 0
#define SEND_RC5 1 // чтоб отключить отправку сигнала по протоколу RC5 нужно указать 0
#define DECODE_RC6 1
#define SEND_RC6 1
...
Disabling unnecessary protocols reduces the size of the program. In principle, you can generally cut out the functions of unused protocols and the corresponding files (files have characteristic names).
The program is extremely simple, the my_decode (& results) function decodes the received signal and displays the button code, protocol type and packet length ...
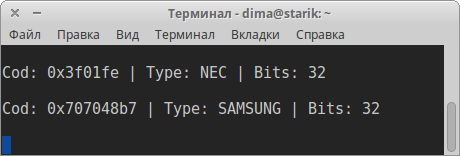
I have no other remotes.
To send the decoded signal, use the function with the corresponding name ...
sendSAMSUNG(0x707048b7, 32);
my_enableIRIn();
The my_enableIRIn () function is necessary, it disables the PWM and puts the timer in receive mode. The same function is used for initialization (before an infinite loop). Because of this function, you will not be able to catch your own signal - this can be solved, but I see no point in this.
If it is not possible to determine the type of protocol ...

... then there is nothing to worry about, the button code is received anyway.
If you need to not only receive an unknown signal, but also send it, then you need to uncomment the lines for "raw data output" ...
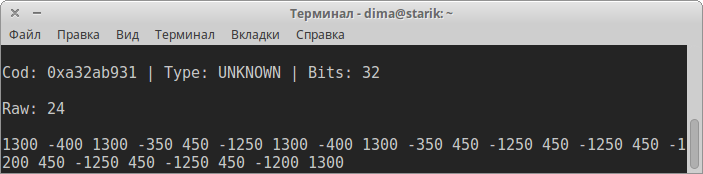
... and send the read data using the sendRaw () function ...
uint8_t khz = 38; // частоту указать экспериментальным путём, основные используемые от 36 до 40 кГц
unsigned int raw_signal[] = {1300, 400, 1300, 400, 450, 1200, 1300, 400, 1300, 400, 450, 1200, 500, 1200, 450, 1250, 450, 1200, 500, 1200, 450, 1250, 1300};
sendRaw(raw_signal, sizeof(raw_signal) / sizeof(raw_signal[0]), khz);
my_enableIRIn(); // переинициализирование приёма (нужна обязательно)
Sending me “raw” does not work for me.
The library uses a DWT counter for microsecond delays. As far as I know, not all stm32 have it, and it is possible that not everywhere it is equally configured. If your stone does not have DWT, then you need to come up with something to replace in the custom_delay_usec (unsigned long us) function at the end of the irSend.s file, the setting is at the beginning.
It's all.
Library