5 typical tasks for JavaScript interviews: parsing and solutions
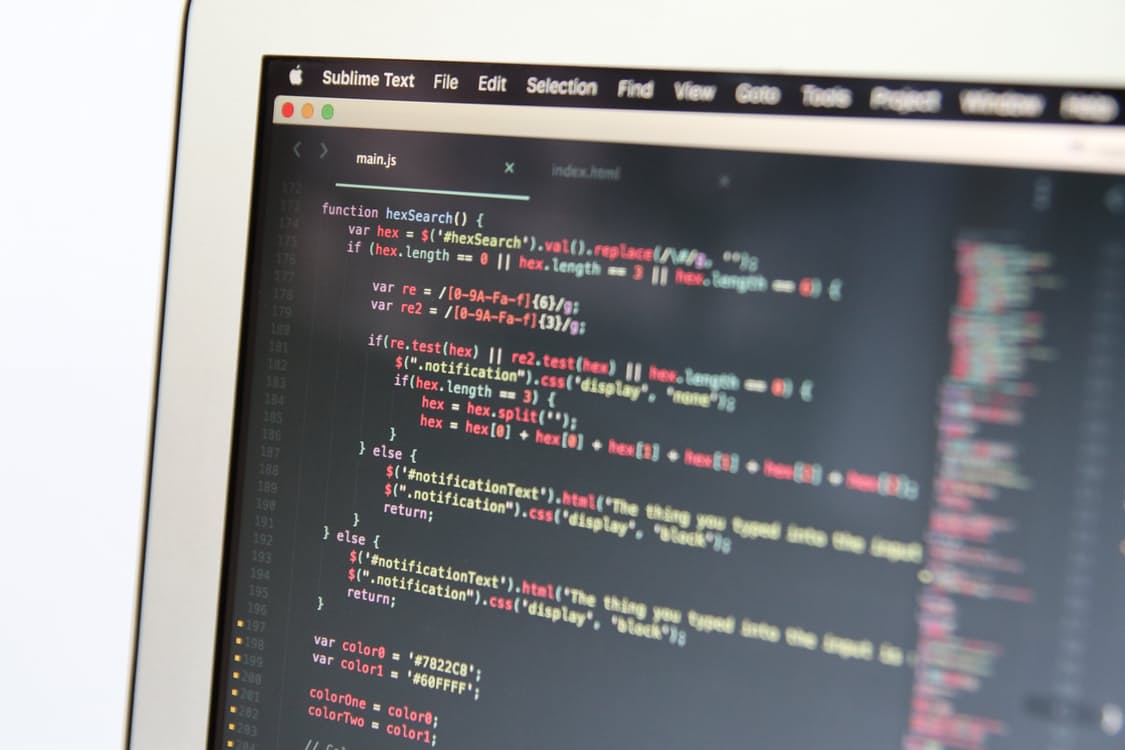
From a translator: they published an article by Maria Perna (Maria Antonietta Perna), which talks about typical tasks in JavaScript , most often offered to job seekers-developers at interviews. The article will be useful primarily to novice programmers. Below are examples of solving problems, if it seems to you that they are not too good, and there is a better option - suggest an alternative in the comments.
Interviews at technology companies have long been a byword. This is not to be surprised - a successful interview gives you the opportunity to get a good job. But this is not so simple, since it is often necessary to solve complex problems.
And more often than not, most of these tasks are not related to the work that the applicant will perform, but they still need to be solved. Sometimes you have to do it on the board, without checking with Google or any other source. Yes, the situation is gradually changing, and some companies refuse such interviews, but many employers still adhere to this tradition. This article is devoted to the analysis of typical JavaScript-tasks, which are often used as tasks for applicants.
We remind you: for all readers of “Habr” - a discount of 10,000 rubles when registering for any Skillbox course using the “Habr” promo code.
Skillbox recommends: Practical course "Mobile Developer PRO" .
The main thing is careful preparation for your interview
Yes, before you begin to disassemble the tasks, let's look at general tips for preparing for an interview.
The main thing is to prepare in advance. Check how well you remember the algorithms and data structures, and pull up knowledge in areas that you are not too familiar with. There are many online platforms that help prepare for interviews. We recommend GeeksforGeeks , Pramp , Interviewing.io and CodeSignal .
It is worth learning to pronounce the decision out loud.It is advisable to tell the applicants what you are doing, and not just write on the board (or type code in the computer, also silently). Thus, if you make a mistake in the code, but the decision will be generally correct, you can increase your chances of success.
The problem must be comprehended before proceeding with the solution. In some cases, you can superficially understand the task and then go the wrong way. It may be worth asking a few clarifying questions to the interviewer.
You need to practice writing code manually, not on a PC. It happens that during interviews the applicant is given a marker and a board where there are no tips or automatic formatting. When searching for a solution, write your code on a piece of paper or directly on the board. If you keep everything in mind, you can forget something important.
JavaScript template tasks
Probably some of these tasks are already familiar to you. You either went through interviews where you had to solve something similar, or you practiced on them while learning JavaScript. Well, now it's time to solve them again, with a detailed explanation of the process.
Palindrome A
palindrome is a word, sentence or sequence of characters that can be read exactly the same way in the usual direction or in the opposite. For example, “Anna” is a palindrome, but “table” and “John” are not.
Statement
Dan string; you need to write a function that allows you to return true if the string is a palindrome, and false if not. In this case, you need to consider spaces and punctuation marks.
palindrome ('racecar') === true
palindrome ('table') === false
Parse the task
The main idea here is to turn the line in the opposite direction. If the “reverse” line is completely identical to the original, then we got a palindrome and the function should return true. If not, false.
Solution
Here is the code that solves the palindrome.
const palindrome = str => {
// turn the string to lowercase
str = str.toLowerCase()
// reverse input string and return the result of the
// comparisong
return str === str.split('').reverse().join('')
}
The first step is to convert the characters of the input string to lowercase. This is a guarantee that the program will compare exactly the characters themselves, and not the case or something else.
The second step is to reverse the line. This is easy to do: you need to convert it to an array using the .split () method (String library). Then we flip the array using .reverse () (Array library). The last step is to convert the inverse array to a string using .join () (Array library).
Now all that is needed is to compare the "reverse" string with the original, returning the result true or false.
FizzBuzz
One of the most popular interview tasks.
Staging
It is required to write a function that displays numbers from 1 to n to the console, where n is an integer that the function takes as a parameter, with the following conditions:
- fizz output instead of multiples of 3;
- buzz output instead of multiples of 5;
- fizzbuzz output instead of numbers that are multiples of both 3 and 5.
Example
Fizzbuzz (5)
Result
// 1
// 2
// fizz
// 4
// buzz
Parse the task
The main thing here is the way to search for multiple numbers using JavaScript. It can be implemented using the module operator or the remainder -%, which allows you to show the remainder when dividing two numbers. If the remainder is 0, this means that the first number is a multiple of the second.
12% 5 // 2 -> 12 is not a multiple of 5
12% 3 // 0 -> 12 is multiple of 3
So, if we divide 12 by 5, we get 2 with the remainder of 2. If we divide 12 by 3, then we get 4 with the remainder 0. In the first case 12 is not a multiple of 5, in the second - 12 is a multiple of 3.
Solution The
following code will be the optimal solution:
const fizzBuzz = num => {
for(let i = 1; i <= num; i++) {
// check if the number is a multiple of 3 and 5
if(i % 3 === 0 && i % 5 === 0) {
console.log('fizzbuzz')
} // check if the number is a multiple of 3
else if(i % 3 === 0) {
console.log('fizz')
} // check if the number is a multiple of 5
else if(i % 5 === 0) {
console.log('buzz')
} else {
console.log(i)
}
}
}
The function performs the necessary checks using conditional statements and produces the result that the user needs. The task should pay attention to the order of the if ... else statements: start with a double condition (&&) and end with the case when multiple numbers could not be found. As a result, we cover all options.
Anagram
This is the name of a word that contains all the letters of another word in the same quantity, but in a different order.
Statement
We need to write a function that checks whether two lines are anagrams, and the case of letters does not matter. Only characters are taken into account; spaces or punctuation are not taken into account.
anagram ('finder', 'Friend') -> true
anagram ('hello', 'bye') -> false
Parse the task
It is important to consider that it is necessary to check each letter in two input lines and their number in each line.
finder -> f: 1 friend -> f: 1
i: 1 r: 1
n: 1 i: 1
d: 1 e: 1
e: 1 n: 1
r: 1 d: 1
To store anagram data, select a structure such as a JavaScript object literal. The key in this case is the letter symbol, the value is the number of its repetitions in the current line.
There are other conditions:
- You need to make sure that the case of letters is not taken into account when comparing. Just convert both strings to lowercase or uppercase.
- We exclude from the comparison all non-characters. It is best to work with regular expressions .
Decision
// helper function that builds the
// object to store the data
const buildCharObject = str => {
const charObj = {}
for(let char of str.replace(/[^\w]/g).toLowerCase()) {
// if the object has already a key value pair
// equal to the value being looped over,
// increase the value by 1, otherwise add
// the letter being looped over as key and 1 as its value
charObj[char] = charObj[char] + 1 || 1
}
return charObj
}
// main function
const anagram = (strA, strB) => {
// build the object that holds strA data
const aCharObject = buildCharObject(strA)
// build the object that holds strB data
const bCharObject = buildCharObject(strB)
// compare number of keys in the two objects
// (anagrams must have the same number of letters)
if(Object.keys(aCharObject).length !== Object.keys(bCharObject).length) {
return false
}
// if both objects have the same number of keys
// we can be sure that at least both strings
// have the same number of characters
// now we can compare the two objects to see if both
// have the same letters in the same amount
for(let char in aCharObject) {
if(aCharObject[char] !== bCharObject[char]) {
return false
}
}
// if both the above checks succeed,
// you have an anagram: return true
return true
}
Note the use of Object.keys () in the snippet above. This method returns an array containing the names or keys in the same order as they appear in the object. In this case, the array will be like this:
['f', 'i', 'n', 'd', 'e', 'r']
Thus, we get the properties of the object without having to perform a volume loop. In the task, you can use this method with the .length property - to check whether the same number of characters is in both lines - this is an important feature of anagrams.
Search for vowels
A fairly simple task that often comes across in interviews.
Statement
We need to write a function that takes a string as an argument and returns the number of vowels,
Vowels are "a", "e", "i", "o", "u".
Example:
findVowels ('hello') // -> 2
findVowels ('why') // -> 0
Solution
Here is the simplest option:
const findVowels = str => {
let count = 0
const vowels = ['a', 'e', 'i', 'o', 'u']
for(let char of str.toLowerCase()) {
if(vowels.includes(char)) {
count++
}
}
return count
}
It is important to pay attention to the use of the .includes () method. It is available for both strings and arrays. It should be used to determine if an array contains a specific value. This method returns true if the array contains the specified value, and false if not.
There is a more concise solution to the problem:
const findVowels = str => {
const matched = str.match(/[aeiou]/gi)
return matched ? matches.length : 0
}
This involves the .match () method, which allows you to implement an efficient search. If the regular expression as an argument to the method is found inside the specified string, then the array of matching characters becomes the returned value. Well, if there are no matches, then .match () returns null.
Fibonacci
A classic problem that can be found in interviews at various levels. It is worth recalling that the Fibonacci sequence is a series of numbers, where each subsequent is the sum of the previous two. So, the first ten numbers look like this: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34.
Statement
We need to write a function that returns the nth record in a certain sequence, and n is the number that passed as an argument to the function.
fibonacci (3) // -> 2
This task involves looping as many times as specified in the argument, returning the value at the appropriate position. This method of statement of the problem requires the use of cycles. If you use recursion instead, the interviewer may like it and give you some extra points.
Decision
const fibonacci = num => {
// store the Fibonacci sequence you're going
// to generate inside an array and
// initialize the array with the first two
// numbers of the sequence
const result = [0, 1]
for(let i = 2; i <= num; i++) {
// push the sum of the two numbers
// preceding the position of i in the result array
// at the end of the result array
const prevNum1 = result[i - 1]
const prevNum2 = result[i - 2]
result.push(prevNum1 + prevNum2)
}
// return the last value in the result array
return result[num]
}
In the results array, the first two numbers are contained in a row, since each record in the sequence consists of the sum of the two previous numbers. At the very beginning, there are no two numbers that you can take to get the next number, so the cycle cannot generate them in automatic mode. But, as we know, the first two numbers are always 0 and 1. Therefore, you can initialize an array of results manually.
As for recursion, everything here is simpler and more complicated at the same time:
const fibonacci = num => {
// if num is either 0 or 1 return num
if(num < 2) {
return num
}
// recursion here
return fibonacci(num - 1) + fibonacci(num - 2)
}
We continue to call fibonacci (), passing in ever smaller numbers as arguments. We stop when the argument passed is 0 or 1.
Conclusion
Most likely, you have already encountered any of these tasks if you were interviewed for the work of the frontend- or JavaScript-developer (especially if this is the junior level). But if they didn’t come across to you, they can come in handy in the future - at least for general development.
Skillbox recommends:
- Applied Python Data Analyst online course .
- Online course "Profession frontend-developer . "
- Practical annual course "PHP-developer from 0 to PRO" .