
ECMA-262 (JavaScript) standard in pictures, part 1
- From the sandbox
- Tutorial

A lot of articles have been written about the JavaScript device. First of all, it is "JavaScript. The kernel." Dmitry Soshnikov , translation of an article by Richard Kornford and a post by Dmitry Frank . But in order to get a good grasp of any technology, it is better to turn to the primary sources. In this case, to the ECMA-262 standard ECMAScript Language Specification . I see this post as a lightweight way to start exploring the standard. I recommend that you follow the links, read the specification text and create your own diagrams.
How JavaScript closures work
The main ECMAScript structures are execution context , lexical environment and environment record . They are connected as follows:
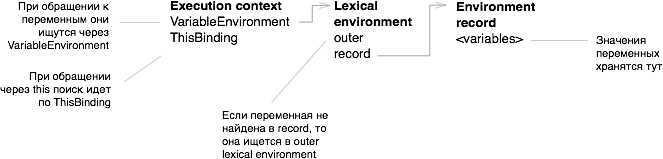
In addition to VariableEnvironment, in the execution context there is also LexicalEnvironment , more details about the differences can be found in ECMAScript 5 spec: LexicalEnvironment versus VariableEnvironment .
ThisBinding will be discussed in the next part of the article.
The environment record stores the values of variables. If you declare
var a = 1
, then in the current record appears {a: 1}. In lexical environment, besides record, there is also an outer field . Outer refers to an external lexical environment , for example, in the case of nested functions. The search for variables begins with the VariableEnvironment of the current context. If a variable with this name is not found in record , then it is searched in the outer environment by chain .
When the program starts , global context andenvironment . As a record , a global object is used .

When the interpreter encounters the function keyword,it creates a FunctionObject . Areference to the current lexical environment is written to the scope property of thecreated FunctionObject . A record ispractically equivalent to a record, except that in the first case, a FunctionObject will be created upon entering the block containing, and in the second, upon execution of a specific line. Each call to the function creates a new context ,
function f(){}
var f = function(){}
function f()

environment and record . The context is pushed onto the stack; upon exiting the function, it is destroyed. In the outer of the created environment , the scope of the called FunctionObject is written. If the function was declared in a global context, then outer will point to the global environment .

Now consider closure when one function returns another.
var x = 1;
function f() {
var x = 2;
function g() {
return x;
}
return g;
}
f()();
When the function f is called , context and environment for f are created , as well as FunctionObject for
function g
. The scope writes a reference to the current environment from the VariableEnvironment . When exiting f, the context is destroyed, but the environment will remain, since there is a link to it from the returned FunctionObject . 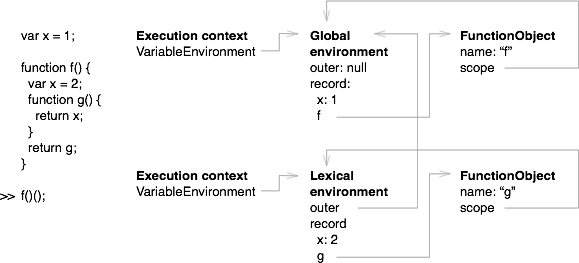
When the function g returned from f is called , the context and environment for g are created . In the outer newenvironment is written to the scope from the called FunctionObject . The search for the variable x begins with the current VariableEnvironment and then continues through outer . As a result, the value will be returned
x = 2
. 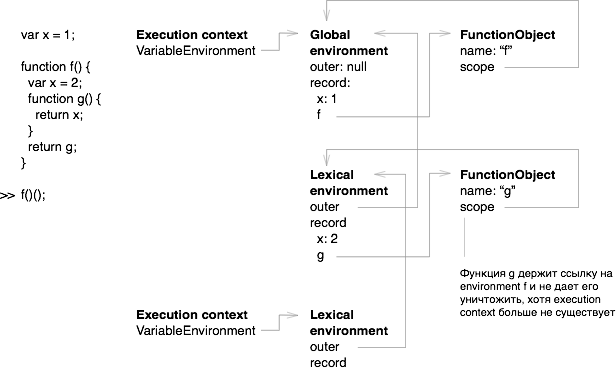
In the next part of the article, we will examine how this works from the point of view of ECMAScript .