
We use standard ListFragment elements as intended
In one of the projects I was faced with the fact that the application needed to display lists of results for various queries (search by words, dates, tags, etc.). Since the lists were repeated in different Activities, the most obvious solution was to use fragments, specifically create your own ListFragment class and reuse it in the project.

ListFragment is just designed to display various lists and is noteworthy in that it has its own fields and methods for working with the list, as well as XML markup with a minimal set of views. Thanks to all this, we can even not create our own XML markup for the fragment using the standard one.
Before we start working with ListFragment, let's look at a little what is inside?
By default, markup from ~ \ sdk \ platforms \ android-XX \ data \ res \ layout \ list-content.xml is used
. Some representations of this markup have identifiers, thanks to which we can customize the parent container, list and empty list text.
If we go over the sources of the ListFragment class, we will see:
So, create our class:
And include it in the markup of our Activity (or you can install the fragment programmatically)
We start the application, go to our Activity and immediately see the loading animation:

Now, in order to hide the animation and display the list, we just need to install the adapter by calling the setAdapter (ListAdapter adapter) method. In this project, I used the Loader in the Activity and the OttoBus event, but there are plenty of options to pass the list object to the fragment, so please ignore the method itself.
Immediately after installing the adapter, the animation will disappear and we will see:
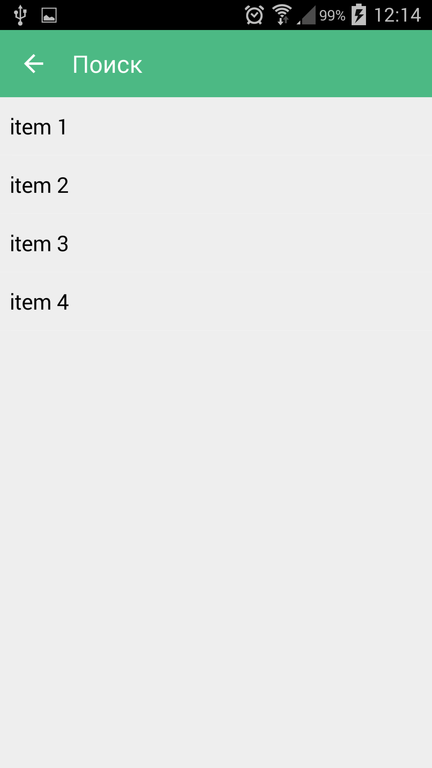
And if our list suddenly turns out to be empty, we will get another screen:

To install your empty text, use the method:
Also in the ListFragment class there is a method for processing list clicks, which we can override
You can not use standard markup, and set your own, but do not forget to specify the correct identifiers for your views.
Total ListFragment has its own views and work logic.
I hope this article allows you to accept to evaluate whether you have enough built-in implementation or whether you need to write your own.
PS Personally, I liked the simplicity and speed of implementation in the project, where I was able to successfully use one fragment class immediately in 4 different Activities.
Pros:
* It is not necessary to create XML markup.
* No need to declare submissions in your class.
* No need to implement the logic of showing / hiding animation, list and empty-text.
* No need to install a "listener"
* Less code.
Minuses:
* You need to learn the methods of the ListFragment class and how it works.
* You can not change the built-in animation even through its own layout.
* It is impossible to redefine the logic of hiding / showing animation, list and empty-text.
PS Join the chat of Russian-speaking Android developers .

ListFragment is just designed to display various lists and is noteworthy in that it has its own fields and methods for working with the list, as well as XML markup with a minimal set of views. Thanks to all this, we can even not create our own XML markup for the fragment using the standard one.
Before we start working with ListFragment, let's look at a little what is inside?
By default, markup from ~ \ sdk \ platforms \ android-XX \ data \ res \ layout \ list-content.xml is used
. Some representations of this markup have identifiers, thanks to which we can customize the parent container, list and empty list text.
If we go over the sources of the ListFragment class, we will see:
View mListContainer; // родительский контейнер (идентификатор R.id.listContainer)
final private AdapterView.OnItemClickListener mOnClickListener // "слушатель" клика на элемент списка
ListAdapter mAdapter; // адаптер списка
ListView mList; // представление списка (идентификатор android.R.id.list)
TextView mEmptyView; // текстовое представление для случая пустого списка, будем называть его empty-текст (идентификатор android.R.id.empty)
View mProgressContainer; // представление для анимации загрузки
So, create our class:
import android.app.ListFragment;
public class MyListFragment extends ListFragment{
}
And include it in the markup of our Activity (or you can install the fragment programmatically)
We start the application, go to our Activity and immediately see the loading animation:

Now, in order to hide the animation and display the list, we just need to install the adapter by calling the setAdapter (ListAdapter adapter) method. In this project, I used the Loader in the Activity and the OttoBus event, but there are plenty of options to pass the list object to the fragment, so please ignore the method itself.
@Subscribe
public void onMyEvent(MyEvent loaderEvent) throws InterruptedException {
String[] listItems = {"item 1", "item 2", "item 3","item 4"}; // пример списка
Thread.sleep(2000); // имитация времени загрузки
ArrayAdapter mArrayAdapter = new ArrayAdapter(getActivity(), android.R.layout.simple_list_item_1, listItems); // адаптер списка
setListAdapter(mArrayAdapter); // установка адаптера
}
Immediately after installing the adapter, the animation will disappear and we will see:
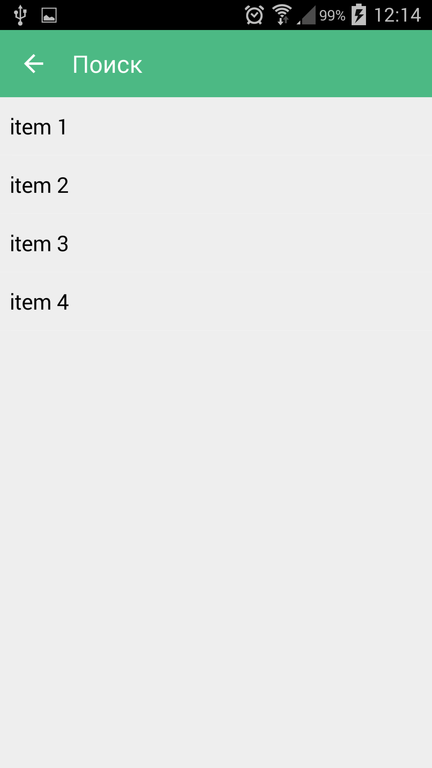
And if our list suddenly turns out to be empty, we will get another screen:

To install your empty text, use the method:
setEmptyText(getResources().getString(R.string.error));
Also in the ListFragment class there is a method for processing list clicks, which we can override
@Override
public void onListItemClick(ListView l, View v, int position, long id) {
// ваш ход господа
}
You can not use standard markup, and set your own, but do not forget to specify the correct identifiers for your views.
Total ListFragment has its own views and work logic.
I hope this article allows you to accept to evaluate whether you have enough built-in implementation or whether you need to write your own.
PS Personally, I liked the simplicity and speed of implementation in the project, where I was able to successfully use one fragment class immediately in 4 different Activities.
Pros:
* It is not necessary to create XML markup.
* No need to declare submissions in your class.
* No need to implement the logic of showing / hiding animation, list and empty-text.
* No need to install a "listener"
* Less code.
Minuses:
* You need to learn the methods of the ListFragment class and how it works.
* You can not change the built-in animation even through its own layout.
* It is impossible to redefine the logic of hiding / showing animation, list and empty-text.
PS Join the chat of Russian-speaking Android developers .