AndroidSocialNetworks - convenient work with social networks
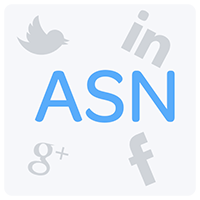
AndroidSocialNetworks is a library for Android that does work with social services. Networks are easier. If you have ever tried to work with social. networks, you know how hard it is. You need to read the documentation for each social. network, download and connect an SDK or a third-party library, take care of the life cycle of http requests. AndroidSocialNetwork should make your life easier, it contains a common interface for Facebook, Twitter, LinkedIn and Google Plus - just build SocialNetworkManager using SocialNetworkManager.Build -> add it to FragmentManager -> configure AndroidManifest.xml -> work with configured social networks. networks. You can do login, post status updates, post photos, add / remove friends.
How to use
First we need to initialize SocialNetworkManager, this is done using SocialNetworkManager.Builder. SocialNetworkManager is a Fragment with retainedInstance (true).
This is necessary in order to be able to monitor the life cycle.
After we initialized the SocialNetworkManager, it must be added to the FragmentManager. Note that for compatibility, you must use the SupportFragmentManager.
mSocialNetworkManager = (SocialNetworkManager) getFragmentManager().findFragmentByTag(SOCIAL_NETWORK_TAG);
if (mSocialNetworkManager == null) {
mSocialNetworkManager = SocialNetworkManager.Builder.from(getActivity())
.twitter(, )
.linkedIn(, , "r_basicprofile+rw_nus+r_network+w_messages")
.facebook()
.googlePlus()
.build();
getFragmentManager().beginTransaction().add(mSocialNetworkManager, SOCIAL_NETWORK_TAG).commit();
}
Since adding a fragment is a transaction, we cannot immediately start using SocialNetworkManager, for this we have a callback called SocialNetworkManager.OnInitializationCompleteListener.
mSocialNetworkManager.setOnInitializationCompleteListener(this);
If, for example, we need to show whether Twitter is connected, we need to update the UI in onSocialNetworkManagerInitialized.
Each request method may receive a listener, or it may not receive, in 2 cases the global one will be called if it is installed:
Set the global listener on Login
@Override
public void onSocialNetworkManagerInitialized() {
super.onSocialNetworkManagerInitialized();
for (SocialNetwork socialNetwork : mSocialNetworkManager.getInitializedSocialNetworks()) {
socialNetwork.setOnLoginCompleteListener(this);
}
}
Calling username for LinkedIn
mSocialNetworkManager.getLinkedInSocialNetwork().requestLogin();
The result is in the global callback.
@Override
public void onLoginSuccess(int socialNetworkID) {
}
@Override
public void onError(int socialNetworkID, String requestID, String errorMessage, Object data) {
}
If we do not want to use global callbacks, we can use local ones:
mSocialNetworkManager.getGooglePlusSocialNetwork().requestLogin(new DemoOnLoginCompleteListener());
private class DemoOnLoginCompleteListener implements OnLoginCompleteListener {
@Override
public void onLoginSuccess(int socialNetworkID) {
}
@Override
public void onError(int socialNetworkID, String requestID, String errorMessage, Object data) {
}
}
Such a mechanism can be used not only for requestLogin, all main methods support 2 types of callback job.
Its implementation of social. the network
If you do not have enough available social. networks, and you want to add support to another, you can do this using the addSocialNetwork (SocialNetwork socialNetwork) method.
Note that you need to do this in onSocialNetworkManagerInitialized. Also note that the ID of your social. The network must be unique. 1, 2, 3, 4 - already reserved.
Available Methods
Full list

Social Support networks
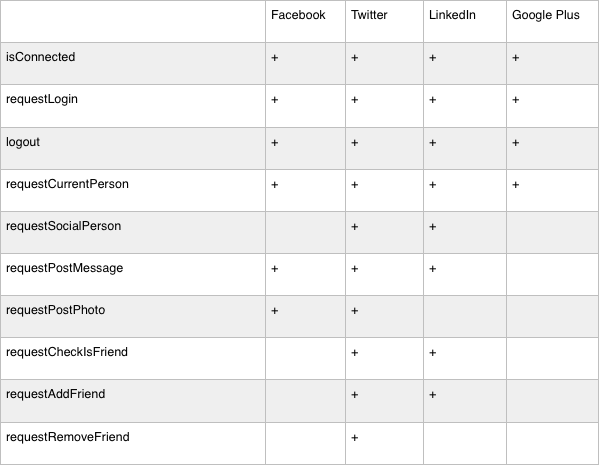
How it works
As you can see, SocialNetworkManager is a snippet (from support v4 for compatibility). This is necessary so that you can conveniently track the life cycle of the application. It is also made retainedInstance (true) so that network requests normally survive lifecycle changes ...
SocialNetworkManager.Builder just prepares a Bundle with parameters.
public SocialNetworkManager build() {
Bundle args = new Bundle();
if (!TextUtils.isEmpty(twitterConsumerKey) && !TextUtils.isEmpty(twitterConsumerSecret)) {
args.putString(PARAM_TWITTER_KEY, twitterConsumerKey);
args.putString(PARAM_TWITTER_SECRET, twitterConsumerSecret);
}
if (!TextUtils.isEmpty(linkedInConsumerKey) && !TextUtils.isEmpty(linkedInConsumerSecret)
&& !TextUtils.isEmpty(linkedInPermissions)) {
args.putString(PARAM_LINKEDIN_KEY, linkedInConsumerKey);
args.putString(PARAM_LINKEDIN_SECRET, linkedInConsumerSecret);
args.putString(PARAM_LINKEDIN_PERMISSIONS, linkedInPermissions);
}
if (facebook) {
args.putBoolean(PARAM_FACEBOOK, true);
}
if (googlePlus) {
args.putBoolean(PARAM_GOOGLE_PLUS, true);
}
SocialNetworkManager socialNetworkManager = new SocialNetworkManager();
socialNetworkManager.setArguments(args);
return socialNetworkManager;
}
Inside SocialNetworkManager are all initialized social. networks are stored in HashMap
private Map mSocialNetworksMap = new HashMap();
In callbacks lifecycle, we go through all the social. networks and forward the values:
public void onStop() {
…
for (SocialNetwork socialNetwork : mSocialNetworksMap.values()) {
socialNetwork.onStop();
}
}
Each social. the network must inherit from the abstract class SocialNetwork.
It is necessary to override the getID () method, precisely by the return value of this social method. network and will vary. In my implementations, I made public ID for convenience, so that you can use socialNetwork.getID () in switch.
public class GooglePlusSocialNetwork extends SocialNetwork …
public static final int ID = 3;
Lifecycle methods are not required to be redefined, only when necessary.
To implement requestLogin, requestPerson methods, etc. it is necessary to redefine methods with callbacks. This is necessary due to the support of both global and local listeners.
Callbacks are stored in Maps.
protected Map mGlobalListeners = new HashMap();
protected Map mLocalListeners = new HashMap();
When we add a global callback, it is added to mGlobalListeners, then for any call without a callback, we call the method with callback, passing null.
public void requestSocialPerson(String userID) {
requestSocialPerson(userID, null);
}
A method with callback registers a listener.
public void requestSocialPerson(String userID, OnRequestSocialPersonCompleteListener onRequestSocialPersonCompleteListener) {
registerListener(REQUEST_GET_PERSON, onRequestSocialPersonCompleteListener);
}
The implementation of registerListener is quite simple:
private void registerListener(String listenerID, SocialNetworkListener socialNetworkListener) {
if (socialNetworkListener != null) {
mLocalListeners.put(listenerID, socialNetworkListener);
} else {
mLocalListeners.put(listenerID, mGlobalListeners.get(listenerID));
}
}
Network requests
To perform network requests, either SDK methods are used:
Request request = Request.newMeRequest(currentSession, new Request.GraphUserCallback() {
@Override
public void onCompleted(GraphUser me, Response response) {
…
request.executeAsync();
or AsyncTasks, don’t be afraid of Task Asinks, if you remember, we use them in the Fragment in retainedInstance (true). Good article on this topic: http://www.androiddesignpatterns.com/2013/04/retaining-objects-across-config-changes.html
Important
- The library does not care about the state of your application and the life cycle, you must do it yourself!
- If you use Google Plus, add this code to your activity:
@Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { super.onActivityResult(requestCode, resultCode, data); /** * This is required only if you are using Google Plus, the issue is that there SDK * require Activity to launch Auth, so library can't receive onActivityResult in fragment */ Fragment fragment = getSupportFragmentManager().findFragmentByTag(BaseDemoFragment.SOCIAL_NETWORK_TAG); if (fragment != null) { fragment.onActivityResult(requestCode, resultCode, data); } }
Link to the library: https://github.com/antonkrasov/AndroidSocialNetworks
Sample application: AndroidSocialNetworks APIDemos