
RGB strip visualization
So, dear friends, I’ll start a little from far away. I decided to order an inexpensive debugging STMF4-DISCOVERY with eBay from America, I waited a long time, I wanted to indulge in the F4 series controller, but a month later I was waiting for a bummer in the mail. Instead of a delicious scarf, natural crap came in - an RGB strip based on the WS2801 controller. The strip is waterproof with connectors and addressable LEDs individually, but first things first!
The first thing you need to try it in! Let's get started!
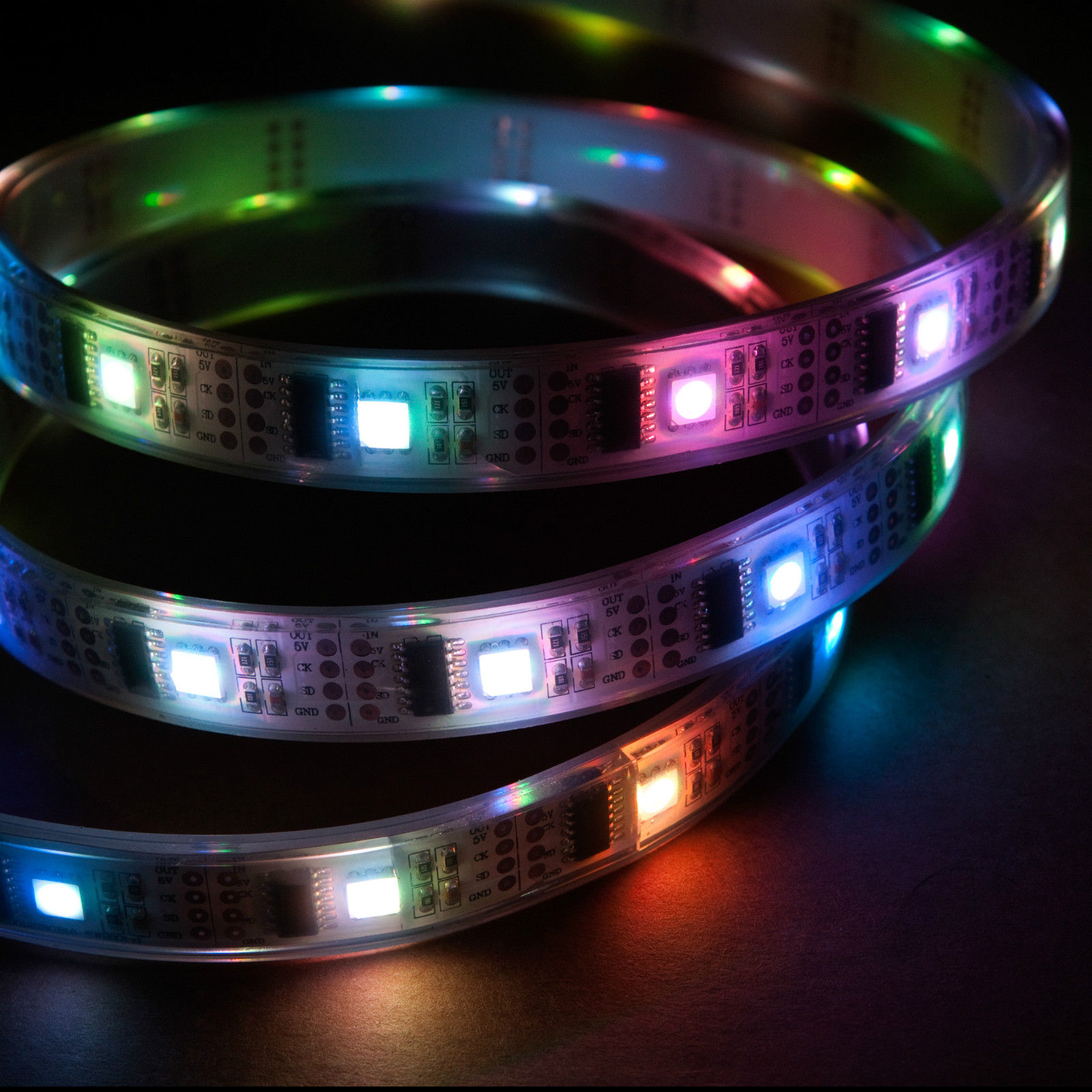

What application options can you find this bad thing? Having looked a little at the Internet, the following options for using the band were identified:
Thus, for our simple test project, we will choose an option that will be perfectly worked out on the table and we will spend a lot of time and money on solving the problem.
So, the goal will be: creating an informer strip that displays the processor load or any other value.
To solve the problem we need:
As a controller, I found in my desk an Arduino Nano, which my hands had not reached for a long time to use for anything of value. In addition to the scarf, a mockup with stuck conductors was also found there, which means you can create.
Judging by the seller’s description of the cents, it is built on WS2801 controllers controlled by SPI or I2C, the choice of the developer. An interesting feature of this tape are RGB diodes and separately controlled driver microcircuits. They are controlled by a sequence of packages, according to the number of strip segments. The control timing chart is shown below:

As can be seen from the figure, one chip expects three bytes of brightness for each channel. If the data is transmitted without pauses, then each microcircuit, after capturing the parcel, starts outputting a sequence for the next receiver.
The pinout is written on the tape itself and in the description, so there’s nothing complicated:
Directly connect our tape to the controller on the breadboard, as shown in the figure:

As a final stroke, we fix the ends of the tape with tape to make a ring.
Writing your own implementation of the control protocol for the strip is not difficult, but since we didn’t have to spend much time with us, we turn to the ready-made library for managing various stripes of FastLED , which is ported to many controllers and supports various LED drivers in the tapes.
The hardware must meet the following requirements:
The first requirement is very important, because beautiful effects should not block the program, i.e. immediately after receiving data from the port, the command should be processed and the behavior should change. Thus, the use of the delay function for a specified time is excluded, it will only be used to set a delay of 1 ms after the entire tape has been filled in.
The tape control protocol includes the following commands:
The response returns command echo lines starting with the characters " >> ", for example:
The tape object is configured in the setup function, I will give it below:
For example, I’ll give an implementation of the non-blocking snake rendering function:
Operating modes we implement the following:
In order not to be tormented quickly, you can rivet a C # control program that implements the protocol:

The program implements the control protocol described above and the ability to send the value of processor congestion and RAM fullness with a given period (the current network speed is planned).
So, all this economy needs to be checked, for which we will use the free processor test utility Hot CPU Tester 4.3 , the composition of the tests was specially cut so that you could see a “live” change in the effects of the tape. The list of tests is shown in the figure:

Test results and the process of functioning of the informer can be seen in the video:
Source code:
LEDInformer.rar I plan to
use this device either in the car or to decorate the insides of my High Tower computer, in order to show what is happening with it in beautifully different colors!
The first thing you need to try it in! Let's get started!
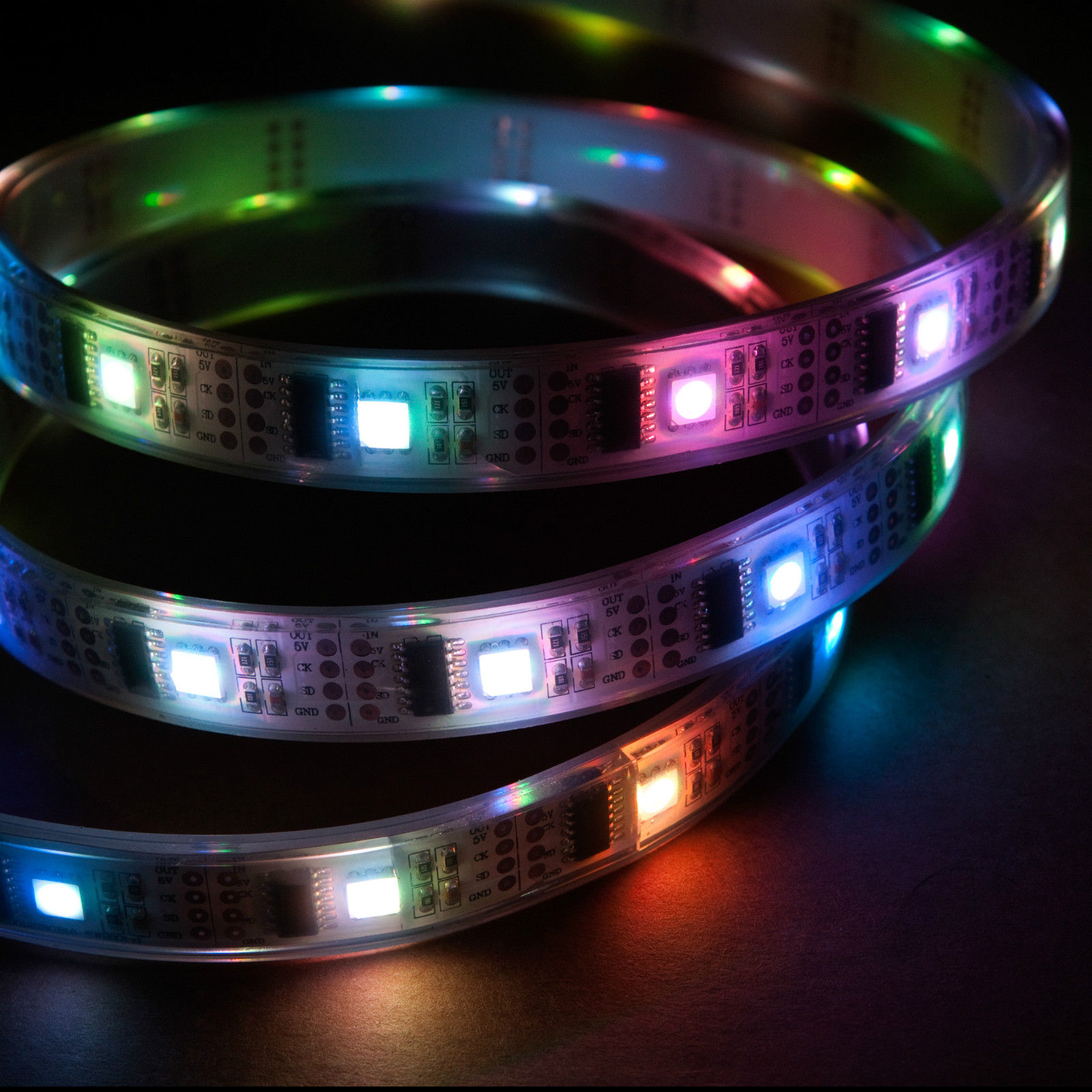

What application options can you find this bad thing? Having looked a little at the Internet, the following options for using the band were identified:
- An incomprehensible thing for pampering and bullying colleagues
- Decorative lighting (car, window, cottage, etc.)
- Visual display of a parameter (sound, voltage, frequency, load, etc.)
Thus, for our simple test project, we will choose an option that will be perfectly worked out on the table and we will spend a lot of time and money on solving the problem.
So, the goal will be: creating an informer strip that displays the processor load or any other value.
To solve the problem we need:
- Arduino nano
- Bread board
- Several conductors
- Insulating tape
- Mini-USB cable
Stage 1. Hardware
As a controller, I found in my desk an Arduino Nano, which my hands had not reached for a long time to use for anything of value. In addition to the scarf, a mockup with stuck conductors was also found there, which means you can create.
Judging by the seller’s description of the cents, it is built on WS2801 controllers controlled by SPI or I2C, the choice of the developer. An interesting feature of this tape are RGB diodes and separately controlled driver microcircuits. They are controlled by a sequence of packages, according to the number of strip segments. The control timing chart is shown below:

As can be seen from the figure, one chip expects three bytes of brightness for each channel. If the data is transmitted without pauses, then each microcircuit, after capturing the parcel, starts outputting a sequence for the next receiver.
The pinout is written on the tape itself and in the description, so there’s nothing complicated:
- Red 5V DC
- Green Clock
- Yellow data
- Brown ground
Directly connect our tape to the controller on the breadboard, as shown in the figure:

As a final stroke, we fix the ends of the tape with tape to make a ring.
Stage 2. Programming the hardware and software
Writing your own implementation of the control protocol for the strip is not difficult, but since we didn’t have to spend much time with us, we turn to the ready-made library for managing various stripes of FastLED , which is ported to many controllers and supports various LED drivers in the tapes.
The hardware must meet the following requirements:
- The main program loop should not be blocked
- The control protocol should be simple and straightforward.
- The device works in SLAVE mode
The first requirement is very important, because beautiful effects should not block the program, i.e. immediately after receiving data from the port, the command should be processed and the behavior should change. Thus, the use of the delay function for a specified time is excluded, it will only be used to set a delay of 1 ms after the entire tape has been filled in.
The tape control protocol includes the following commands:
SET MODE% d \ n | Set operation mode |
SET COLOR% d \ n | Set primary color |
SET VALUE% d \ n | Set a value from 0 to 1000 |
The response returns command echo lines starting with the characters " >> ", for example:
>> SET VALUE 673
The tape object is configured in the setup function, I will give it below:
void setup() {
Serial.begin(115200);
// Тип контроллера, номер пина данных, номер пина синхр., тип развертки, массив значений и его длина
FastLED.addLeds(leds, NUM_LEDS);
}
For example, I’ll give an implementation of the non-blocking snake rendering function:
void runner(CRGB color, int period, uint8_t s) {
static int pos = 0; /* Текущее положение ползунка */
static long last = millis();
if(millis() - last > period) { // Реализация временной задержки
last = millis();
clear_led();
if(pos < NUM_LEDS - s) { // Реализация
for(uint8_t j = 0; j < s; ++j) {
leds[pos + j] = color;
}
FastLED.show();
++pos;
} else {
pos = 0;
}
}
}
Operating modes we implement the following:
- Running segment with a given offset speed
- Filling a tape with a given color for a given length
- Filling the tape with a color change depending on the value (0 - 300 green, 301 - 660 yellow, 661 - 1000 red)
- A running segment with a given offset speed with varying colors
- Filling a tape with a given color for a given length with differently changing colors
- Color Check Mode According to FastLED Library Documentation
In order not to be tormented quickly, you can rivet a C # control program that implements the protocol:

The program implements the control protocol described above and the ability to send the value of processor congestion and RAM fullness with a given period (the current network speed is planned).
Stage 3. Debugging and testing
So, all this economy needs to be checked, for which we will use the free processor test utility Hot CPU Tester 4.3 , the composition of the tests was specially cut so that you could see a “live” change in the effects of the tape. The list of tests is shown in the figure:

Test results and the process of functioning of the informer can be seen in the video:
Source code:
LEDInformer.rar I plan to
use this device either in the car or to decorate the insides of my High Tower computer, in order to show what is happening with it in beautifully different colors!