Development of a full-fledged API - application for SolidWorks software package. Bolt model
Introduction
The previous material outlined the basic principles of developing API applications for the SolidWorks 3D modeling system. In this article, I would like to show the development of a real API - an application designed to increase the efficiency of a design engineer.
One of the design stages is the development of a three-dimensional model of the product. Complex assemblies use many standardized fasteners developed directly by the designer himself.
Of course, an alternative option is to use configurations in SolidWorks, however, if you need to use non-standardized fasteners, the engineer will not know in advance what sizes and configurations he will need. Accordingly, the development and assembly of complex parts will be constantly interrupted by the need to model fasteners.
As part of this work, an API was developed - an application for automatically creating a bolt model with specified geometric parameters.
Targets and goals
The aim of this work is to increase the labor efficiency of an engineer - designer at the stage of modeling the designed product. In accordance with this goal, the following tasks were identified:
• Development of a standard model of a bolt;
• Creating an API - application;
• Development of a library that implements model building;
Development tools
• SolidWorks;
• C #;
• WPF;
• VSTA (Visual Studio Tools for Applications);
Model development
As a result of modeling, a three-dimensional model was obtained, presented in the figure below.
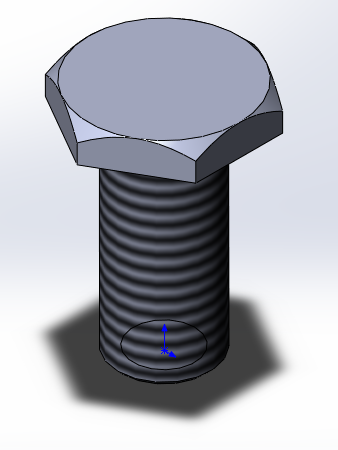
Interface Design
The technology of Windows Presentation Foundation was chosen as a tool for developing the application interface. XAML markup for the interface is shown in the listing.
Параметры изделия: Диаметр резьбы: Высота головки: Размер под ключ: Длина:
As a result, a simplified application interface was developed, as shown in the figure below.
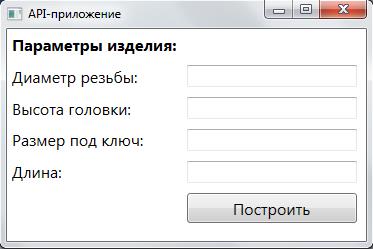
Software implementation
public partial class MainWindow : Window
{
public MainWindow() {
InitializeComponent();
}
public SldWorks swApp;
void Button_Click_1(object sender, RoutedEventArgs e) {
ModelDoc2 swDoc = null;
PartDoc swPart = null;
DrawingDoc swDrawing = null;
AssemblyDoc swAssembly = null;
bool boolstatus = false;
int longstatus = 0;
int longwarnings = 0;
swDoc = ((ModelDoc2)(swApp.ActiveDoc));
OpenDoc(ref swDoc, ref longstatus, ref longwarnings);
swApp.ActivateDoc2("Болт", false, ref longstatus);
swDoc = ((ModelDoc2)(swApp.ActiveDoc));
ModelView myModelView = null;
boolstatus = GrooveDiameter(swDoc, boolstatus);
boolstatus = SetBoltHeadHeight(swDoc, boolstatus);
boolstatus = SetDimensionUnderKey(swDoc, boolstatus);
boolstatus = SetLength(swDoc, boolstatus);
}
//Задать длину болта
static bool SetLength(ModelDoc2 swDoc, bool boolstatus) {
boolstatus = swDoc.Extension.SelectByID2("", "FACE", 0.011912467420266637, 0.01846469011343288, 0.0037872311733622155, false, 0, null, 0);
boolstatus = swDoc.Extension.SelectByID2("D1@Эскиз1@Болт.SLDPRT", "DIMENSION", 0.047351053302585169, 0.019301296053367275, 0.0028786441805853363, true, 0, null, 0);
boolstatus = swDoc.EditRebuild3();
swDoc.ClearSelection2(true);
return boolstatus;
}
//Задать размер под ключ болта
static bool SetDimensionUnderKey(ModelDoc2 swDoc, bool boolstatus) {
boolstatus = swDoc.Extension.SelectByID2("", "FACE", -0.00036826155354674484, 0.051999999999850388, -0.0013844273092900039, false, 0, null, 0);
boolstatus = swDoc.Extension.SelectByID2("D4@Эскиз1@Болт.SLDPRT", "DIMENSION", 0.0079871009577274082, 0.035833016414888383, -0.013439947146820423, true, 0, null, 0);
boolstatus = swDoc.EditRebuild3();
swDoc.ClearSelection2(true);
return boolstatus;
}
//Задать высоту головки болта
static bool SetBoltHeadHeight(ModelDoc2 swDoc, bool boolstatus) {
boolstatus = swDoc.Extension.SelectByID2("", "FACE", 0.0025916945023696791, 0.022486595775220126, 0.012228373547031879, false, 0, null, 0);
boolstatus = swDoc.Extension.SelectByID2("D2@Эскиз1@Болт.SLDPRT", "DIMENSION", 0.032400529167230879, 0.043963039647934993, 0, true, 0, null, 0);
boolstatus = swDoc.EditRebuild3();
swDoc.ClearSelection2(true);
return boolstatus;
}
//Задать диаметр резьбы болта
static bool GrooveDiameter(ModelDoc2 swDoc, bool boolstatus) {
boolstatus = swDoc.Extension.SelectByID2("", "FACE", -0.00081167638456329516, 0.026711469979688613, 0.0089633242408808655, false, 0, null, 0);
boolstatus = swDoc.Extension.SelectByID2("D3@Эскиз1@Болт.SLDPRT", "DIMENSION", 0.0056429925389302749, 0.040324953527420437, 0, true, 0, null, 0);
boolstatus = swDoc.EditRebuild3();
swDoc.ClearSelection2(true);
return boolstatus;
}
//Открыть документ
void OpenDoc(ref ModelDoc2 swDoc, ref int longstatus, ref int longwarnings) {
swDoc = ((ModelDoc2)(swApp.OpenDoc6("D:\\ХАБР\\Item2\\Болт.SLDPRT", 1, 0, "", ref longstatus, ref longwarnings)));
}
}
Conclusion
The main goal of such API applications is to automate the process of creating a model. In the framework of this work, only automation modeling of fasteners (bolts) was considered. In the future, the following automation issues will be considered:
• API - an application for assembling a model of typical structures in the SolidWorks software package;
• API - an application for the automation of the development of design documentation;
• API - an application for modeling complex products.