
Convenient image manipulation in Django
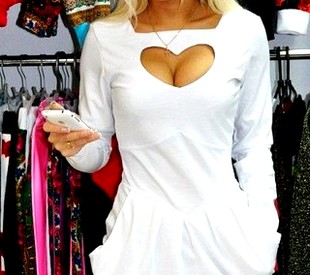
Imagekit is an application that allows you to manipulate images without changing the original image. I think convenience and so it is clear, you can have several options for the picture and always be able to return to the source.
Allows you to resize, create previews, apply watermarks.
Installation
To work with imagekit, you need PIL or PILLOW.
Put from PyPI:
pip install django-imagekit
Add 'imagekit' to INSTALLED_APPS
Use in the model
import PIL
...
from PIL import Image
from imagekit.models.fields import ImageSpecField
from imagekit.processors import ResizeToFit, Adjust,ResizeToFill
class Jobseeker(models.Model):
def get_file_path(self, filename):
extension = filename.split('.')[-1]
filename = "%s.%s" % (uuid.uuid4(), extension)
return os.path.join("images", filename)
...
photo = models.ImageField(verbose_name=u'Poster',upload_to=get_file_path,max_length=256, blank=True, null=True)
photo_small =ImageSpecField([Adjust(contrast=1.2, sharpness=1.1),
ResizeToFill(50, 50)], image_field='photo',
format='JPEG', options={'quality': 90})
photo_medium =ImageSpecField([Adjust(contrast=1.2, sharpness=1.1),
ResizeToFit(300, 200)], image_field='photo',
format='JPEG', options={'quality': 90})
photo_big =ImageSpecField([Adjust(contrast=1.2, sharpness=1.1),
ResizeToFit(640, 480)], image_field='photo',
format='JPEG', options={'quality': 90})
Usage in the template
In view.py we pass the object
def some_def(request):
...
photo = Jobseeker.objects.get()
...
return (render_to_response('jobseeker/resume_template.html',
{
...
'photo': photo,
...
}, context_instance=RequestContext(request))
)
and in the template we can refer to the following fields:
photo.photo_small.url # превью размером 50х50
photo.photo_medium.url # изображение приведенное к размерам 300х200
photo.photo_big.url # изображение приведенное к размерам 640х480
# можем обратиться к свойствам картинки
photo.photo_small.width #вернет 50
photo.photo.width #вернет ширину оригинальной картинки
In this example, we used 2 image processors, namely: ResizeToFit and ResizeToFill.
In fact, there are several more - processors Module
ResizeToFit(width=None, height=None, upscale=None, mat_color=None, anchor='c')
Purpose: an image with the specified dimensions will be created, on which the original image is superimposed, proportionally compressed, so as not to go beyond the specified frames.
width = None, height = None - width and height, respectively.
upscale - a Boolean value that determines whether the image will be enlarged if it is less than the specified parameters
mat_color - fill color of the area unfilled with the original image.
ResizeToFill(width, height)
Purpose: to resize to the specified sizes, everything that goes beyond the specified sizes will be trimmed.
The attributes indicate the shinira and height, respectively.
Work examples
Original photo
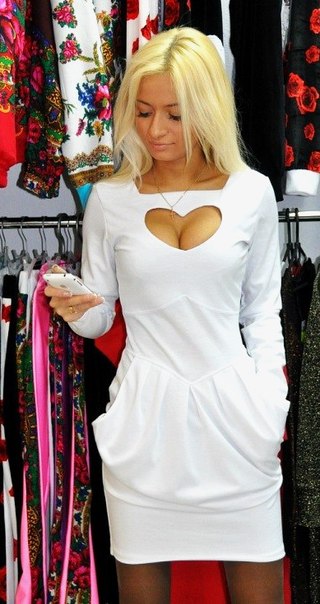
ResizeToFill
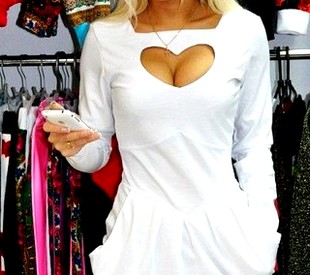
ResizeToFit using the mat_color parameter to show the actual dimensions of the new image

All additional pictures created using imagekit are saved in the cache folder, all originals are saved in a separate folder. They are created at the first visit to the page in which they are used. At any time, all pictures can be deleted without harm to the original and recreated with new parameters.
All this greatly facilitates the work with images, and saves the nerves of the developer, if the initial settings were suddenly not correct, and the originals were not thought of saving.
Links:
Github
Documentation