Pure c ++
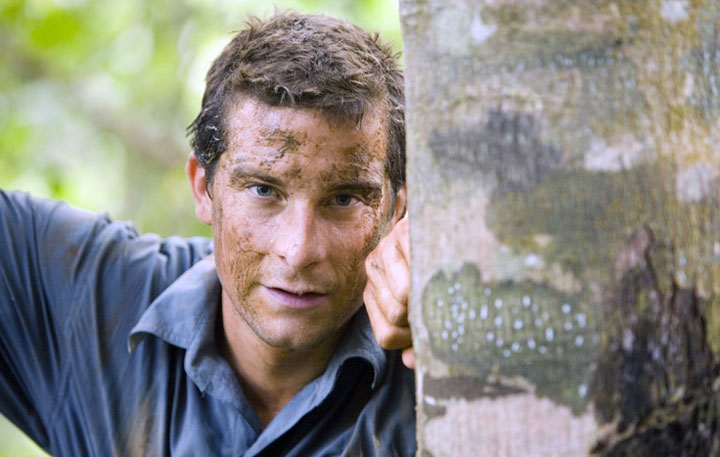
Let's get acquainted.
I am Serge. (It's not me on the photo). I work at Intel. Together with my colleagues I write the GPA . I've been programming for 20 years now. Well, if you count from school. Recently, a lot of different thoughts have accumulated that I want to share with someone. Tell someone about what is good and what is bad. One can also tell emptiness (it’s even calmer, no one distracts and does not bother with His Most Correct Opinion), but it is not very effective. Therefore, I will merge my thoughts here. Suddenly someone will come in handy ...As an introduction
Many believe that there are two related languages - C and C ++. At the same time, C ++ is supposedly the same C, with only two pluses, i.e. OOP. This is a very common misconception. "Actually, this is not so." C and C ++ are completely different languages that have virtually nothing in common. However, historically, C ++ is syntactically compatible with C, i.e. can compile programs written in C. Because of this feature, there is no clear line between the languages and there is a lot of code written in an eerie mixture of these languages. Moreover, the proportion of this mixture can vary even within the framework of one program by one author. We will call such creativity the language “Ts with classes”. Sometimes the use of “C with classes” can be a conscious choice dictated by circumstances and the task to be solved. Unfortunately, more often than not, such a code simply demonstrates the huge difference between the author’s ambitions and real abilities. If you are just at the very beginning of the journey, it is better to take something more stringent, such as pure C or Pascal. And then come here when it becomes crowded in these languages.Speaking of circumstances and tasks. Who do you think is a “real programmer”? No, not a hacker. And not the one who knows ALL programming languages. And not even the one who is able to write the coolest program. A programmer is one who can solve the set algorithmic problem in the stated time with a given level of quality. Reread this definition several times slowly, pondering each word. Try to understand such a simple thing that without a task, deadlines and quality of programming does not happen! It can be creativity, training, experiment, research, anything else, but not programming. Because the result of programming is a program that serves people, which, since its inception, every day, repeatedly solves its problem for them. Even Wikipedia in the definition of the term programmer 3 times mentions the word “task”! Understand that no one needs a perfect program written in an infinitely long time. People are more willing to use at least something that solves their problems here and now, well, in extreme cases, tomorrow. Also, few people will use the foul-smelling craft, sloppy somehow, when there are already many simpler and more pleasant solutions to their problems. Almost no one will use the "cool trinket" for a long time. A variety of animals running around the screen, tricked out special effects of animation of windows and menus, the Most Correct Operating Systems, etc. - All this junk is doomed to create a WOW effect among the younger generation, after which it is honored to go into oblivion. I can talk about quality assurance. Explain something about the timing. But you will have to look for tasks yourself. - All this junk is doomed to create a WOW effect among the younger generation, after which it is honored to go into oblivion. I can talk about quality assurance. Explain something about the timing. But you will have to look for tasks yourself. - All this junk is doomed to create a WOW effect among the younger generation, after which it is honored to go into oblivion. I can talk about quality assurance. Explain something about the timing. But you will have to look for tasks yourself.
I think it's time to talk about C ++. I hope you already read thick and smart books about this language, wrote and compiled your First Program? If not yet, go read and write, we’ll talk when you understand at least the syntax of the language. If you are still here, you probably already know that this language supports OOP at the syntax level. However, one important thing to understand for yourself, so as not to slip into the "Ts with classes." In C ++, everything is a class. I'll try on my fingers:
void foo();
...
foo();
This is the most C. It is even without classes. If we are writing a program in C ++, then it’s more correct to write the same thing like this:
class Foo
{
public:
static void foo();
};
...
Foo::foo();
Still sometimes it is convenient to do like this:
class foo
{
public:
foo();
};
...
foo();
Or even like this:
class Foo
{
public:
void operator()();
};
...
Foo foo;
foo();
In exceptional cases, you can do this:
namespace Foo
{
void foo();
}
...
Foo::foo();
Except for the last option (I don't like it, but sometimes it can be useful) you probably noticed the class keyword everywhere. The fact is that class does not mean at all an object from OOP, as they usually write in thick and smart books. It’s just a language construct that combines several different entities into a single whole and endows them with features. For example, it is very common to use classes to define an “interface” —object behavior.
class IFoo
{
public:
virtual void foo() = 0;
};
In such a class there are no "fields" that could store anything. Also, there are no methods that could do something. The idea is in a pure, concentrated form. However, it is through such interfaces that the modules “communicate” in large software projects.
Here is another controversial example of using the class:
template class DoIt
{
private:
TypeA m_it;
public:
template DoIt(TypeB it) : m_it(it) {}
operator TypeA() {return m_it;}
};
...
TypeC c;
TypeD d = DoIt< TypeD >(c);
Why so hard? And then, to divide the template argument into two parts and set one of them explicitly, and force the compiler to take the other from the arguments of the constructor call. This is a very beautiful way to write a “template function” with arbitrary types of arguments and given a return type.
There is such a play on words: The more cheese - the more holes in it, the more holes in the cheese - the less cheese in it actually. As a result, the more cheese, the less cheese. With C ++, this is exactly what happens. In this language, simple and banal things are written in very bulky and confusing constructions. However, it is precisely these constructions that help to make complex things simple in this language. Take for example your first program on “C with classes”
#include
int main(void)
{
std::cout << "Здраствуй Мир!!!\n";
std::cout << "До свиданья\n";
return 0;
}
and make a C ++ program out of it:
#include
class App
{
public:
int Run()
{
std::cout << "Здраствуй Мир!!!\n";
return 0;
}
~App()
{
std::cout << "До свиданья.\n";
}
};
int main(void)
{
try
{
App().Run();
}
catch(...)
{
throw;
}
return 0;
}
The main function is for us the inevitable legacy of C and getting rid of it without unnecessary problems is difficult. We will consider its implementation as part of the language. Now imagine that we need to add a complex thing to our program:
#include
#include
class App
{
public:
int Run()
{
std::cout << "Здраствуй Мир!!!\n";
throw std::runtime_error("Что-то плохое в нашей программе случилось.");
return 0;
}
~App()
{
std::cout << "До свиданья.\n";
}
};
int main(void)
{
try
{
App().Run();
}
catch(...)
{
throw;
}
return 0;
}
In the last version, our program will be crashed by an exception thrown from somewhere inside. However, despite the poor ending, she will be able to correctly complete her final part. As you can see, the complexity has not changed our program. It is such tricks that allows you to do "real" C ++. If you think that everything can always be overlaid with try ... catch sections, I have bad news for you. Such code will be almost impossible to support.