
Compact Java Servlet for Mobile Web
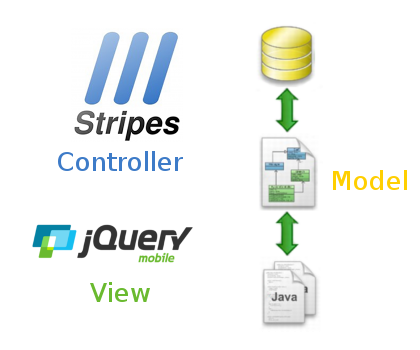
The main area of programming for me is the development of software for automation of accounting in trade. I came across the opportunity to use Java servlets for this in 2009, when along with the latest version of Openbravo POS that was released for desktop, there was a restaurant module for PDA. The main idea at that time for Openbravo POS developers was not to complicate the desktop version of the application, to take narrow business logic into a separate small application, the main highlight of which was the compact web interface for access from any device, and not just where Java can be run c Swing. It was then assumed that the servlet would not only work with the same database as the desktop version, but also the container for it would be integrated into the desktop application, after which the user automatically gained access to POS within the range of Wi- Fi network. This idea has not been further developed within the community, but since 2012 I have continued to use the principles laid down for my clients then, and in this article I will tell readers how to use the Stripes Frameworkin conjunction with jQuery Mobile and ORMLite , get a tool for the rapid development of small mobile web-oriented servlets.
Tools
Usually, when you are looking for a development tool, you try to find something universal that you can learn once to use repeatedly for quite some time. In 2012, this is exactly what I came across when I tried to redo the source code of the Openbravo POS restaurant module. It was based on the Struts 1 framework , which I was not happy with at all to learn about writing XML schemas, but for good luck, just at that moment, I came across an overview of Java ecosystem technologies from RebelLabs . One of the sections of this review was devoted to the popularity of web frameworks among developers. Stripes was included in this 10 with an honorable 2%. With its development, I began to learn the basics of developing Java servlets. It also helped me that by that time on Habré in the "Sandbox" there was already a review article about this framework.
In Stripes, everything is subject to the logic of reaction to controllers (ActionBean), which are responsible for gaining access to the data model for further display to the user through JSP page templates. Since I needed a mobile web interface, for the visual part I chose jQuery Mobile , the main plus of which is the ability to use only HTML5 markup with little touch on JavaScript to build a dynamic reaction to controller actions.
The third element responsible for working with the data model, I first chose a very simple Persist ORM / DAO, but when its functionality became insufficient, I changed it to a more powerful ORMLite . The main thing in the choice was the ease of using the existing representation model and the absence of the need to write SQL queries, managing, as in the case of Stripes, only with annotations.
Model-View-Controller
The key to Stripes is a 100% focus on the MVC concept (Model-View-Controller). And the main goal of creating this framework in 2005 was to create a more lightweight implementation of the MVC concept for Java EE than was implemented in the then-popular Struts framework. By version 1.5 in 2008, this goal was fully achieved by the Stripes authors and this framework began to gain popularity among developers.
When designing the structure of the servlet source code, you should try not to go beyond the Model-View-Controller paradigm, which is not very difficult to do for most tasks when creating CRUD applications. For example, in the source code for the product directory, it will look like this:
Data Source, Source SQL Table
CREATE TABLE PRODUCTS (
ID VARCHAR(255) NOT NULL,
NAME VARCHAR(255) NOT NULL,
CODE VARCHAR(255) NOT NULL,
PRICESELL DOUBLE NOT NULL,
CATEGORY VARCHAR(255) NOT NULL,
PRIMARY KEY (ID),
CONSTRAINT PRODUCTS_FK_1 FOREIGN KEY (CATEGORY) REFERENCES CATEGORIES(ID)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
CREATE UNIQUE INDEX PRODUCTS_CODE_INX ON PRODUCTS(CODE);
CREATE UNIQUE INDEX PRODUCTS_NAME_INX ON PRODUCTS(NAME);
Annotation data model for ORMLite
@DatabaseTable(tableName = "PRODUCTS")
public class Product {
public static final String ID = "ID";
public static final String NAME = "NAME";
public static final String CODE = "CODE";
public static final String PRICESELL = "PRICESELL";
public static final String CATEGORY = "CATEGORY";
@DatabaseField(generatedId = true, columnName = ID)
private UUID id;
@DatabaseField(columnName = NAME, unique = true, canBeNull = false)
private String name;
@DatabaseField(columnName = CODE, unique = true, canBeNull = false)
private String code;
@DatabaseField(columnName = PRICESELL, canBeNull = false)
private BigDecimal pricesell;
@DatabaseField(foreign = true,
columnName = CATEGORY,
foreignColumnName = ProductCategory.ID,
foreignAutoRefresh = true,
canBeNull = false)
private ProductCategory productCategory;
// ...
// Перечисления методов get и set.
// ...
}
In the beginning, using ORMLite, the structure of the data model and its relationship with the relational database (the value of the fields
tableName
and columnName
) are defined . Then, through getters and setters, the model provides access to the remaining classes and methods of the servlet.Stripes controller for data input / output
public class ProductCreateActionBean extends ProductBaseActionBean {
private static final String PRODUCT_CREATE = "/WEB-INF/jsp/product_create.jsp";
@DefaultHandler
public Resolution form() {
// Открытие формы для заполнения.
return new ForwardResolution(PRODUCT_CREATE);
}
public Resolution add() {
// ...
// После проверки полученных значений, создание записи в таблице.
// ...
}
@ValidateNestedProperties({
@Validate(on = {"add"},
field = "name",
required = true,
trim = true,
maxlength = 255),
@Validate(on = {"add"},
field = "code",
required = true,
trim = true,
minlength = 8,
maxlength = 13),
@Validate(on = {"add"},
field = "priceSell",
required = true,
converter = BigDecimalTypeConverter.class),
@Validate(field = "productCategory.id",
required = true,
converter = UUIDTypeConverter.class)
})
@Override
public void setProduct(Product product) {
super.setProduct(product);
}
}
The controller intercepts the data transmitted by the user and processes them according to the specified annotations. For example, performing
Resolution form()
, having received the value of the key field productCategory.id
, it passes it through UUIDTypeConverter
, checking if it meets the criteria acceptable for the UUID.JSP template with jQueryMobile markup and Stripes fields
In the page template, jQuery Mobile markup can also be used for preliminary verification of input data, for example, in a visual form, offering the user on a mobile device different keyboards for text and numbers (the
input
parameter type
is equal to "text"
or "number"
).What is it suitable for?
At the end of last 2013, RebelLabs published a new review of the development prospects of the framework in the future 2014 . Stripes was not included in this review, since most of the attention was paid to more polar frameworks like it, such as Spring, Grails or Vaadin. But it seems to me that if you use jQuery Mobile and ORMLite together with him, then he will now find his niche in the Java developer tools. In at least 4 of the 7 areas of application proposed by RebelLabs, you can feel quite confident with it:
- CRUD applications - about this was higher, if everything is done sequentially and according to one scheme, it is very difficult to do poorly. The main thing in designing is to try to make each controller action as complete as possible, but not crowded.
- Mobile applications - here the main burden rests with jQuery Mobile, for the Java programmer there is a definite plus that in addition to HTML5 you can not touch JavaScript and CSS.
- Application prototypes - this is the main reason why I use this bundle, having typed from 10 ok servlets in the portfolio, you can easily shuffle each component of each of them under different business processes.
- Porting desktop applications - and this is where it all started from me, and it is for such an application that I can recommend first of all the alloy of these three frameworks.
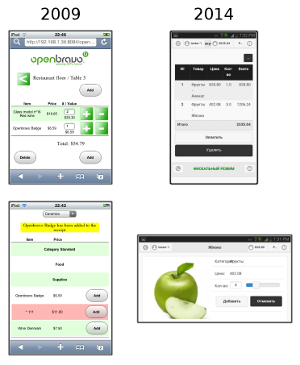
This is the result, a new interface with jQuery Mobile version 1.4 in 2014, compared with the interface that the Openbravo POS PDA module had in 2009.
As an example of the practical implementation of the article, a small CRUD servlet project for the product catalog: github.com/nordpos-mobi/product-catalog