
CodeIgniter Command Line library - a small assistant for working with CLI
As you probably know, php has an interesting function for processing data coming from the command line: getopt . But there is one small problem - it does not work correctly in CodeIgniter, and it’s not very convenient.
And since I had a bit of free time, the desire to write something my own open-source, try github (sitting on hg + bitbucket), and finally do something useful for the stubborn CodeIgniter and its community, I decided to write my crutch , designed to slightly brighten the spelling of cli scripts on this framework.
It can do the very least - look for the given arguments, validate them (if the required ones are not specified) and display hints for using your script, and if desired, it can easily be finished for use without reference to CodeIgniter:
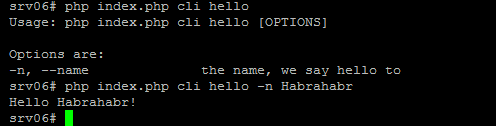
Actually, when creating the library, I tried to make its use familiar to people working with CodeIgniter, so its use is somewhat similar to using the standard Form_Validation library .
1. First, download the library from the github and put it in the * / application / libraries / folder .
2. Then create a controller that will be used on the command line:
Pay attention to the line where it is checked whether this controller starts from the command line. We don’t want any villain to get access to our script through the website!
3. Now create a method using our library:
So, setting the config, we initialize our library and check the incoming parameters for validity, just like in Form_validation!
If it is not valid - there are no parameters, or some error occurred, we simply call the method, which will tell us what is wrong:
If everything went as it should, then we can execute our code by getting the argument parameters using the get_argument () method . You can refer to them both by their full name and by alias:
4. Now that everything is ready, open the command line and check our script:
Since you did not enter any arguments, he will friendlyly tell you how to use it:
So, you need to enter a name:
Which will output the following:
UPD: in addition, the script can also take as a parameter a long string in quotation marks, after clearing it from them:
As you can see, the library is very primitive, and implements only the very base of functions that might be required when working with the command line. Nevertheless, it can save a little time on developing system cli scripts used, say, for tasks in cron. This is really convenient - you can use all the written code of your models and libraries to create system tasks, such as, for example, generating a site map or recounting any indicators.
I also want to warn in advance that while the version of the library is still quite raw, and any bugs may occur. I will be happy to hear your comments on this matter, and I will also be glad if you contribute by joining the development on github .
And since I had a bit of free time, the desire to write something my own open-source, try github (sitting on hg + bitbucket), and finally do something useful for the stubborn CodeIgniter and its community, I decided to write my crutch , designed to slightly brighten the spelling of cli scripts on this framework.
It can do the very least - look for the given arguments, validate them (if the required ones are not specified) and display hints for using your script, and if desired, it can easily be finished for use without reference to CodeIgniter:
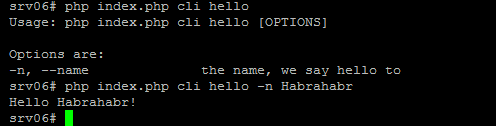
Using
Actually, when creating the library, I tried to make its use familiar to people working with CodeIgniter, so its use is somewhat similar to using the standard Form_Validation library .
This is best seen with an example:
1. First, download the library from the github and put it in the * / application / libraries / folder .
2. Then create a controller that will be used on the command line:
input->is_cli_request()) {
show_404();
}
}
}
Pay attention to the line where it is checked whether this controller starts from the command line. We don’t want any villain to get access to our script through the website!
3. Now create a method using our library:
public function hello() {
// Add an argument for cli interaction
$argumets = array(
array('alias' => '-n',
'arg' => '--name',
'help' => 'the name, we say hello to',
'type' => 1)
);
// Initialize library with arguments array
$this->load->library("command_line", $argumets);
// Validate input
if (!$this->command_line->valid_input()) {
// If not valid, print some help
print $this->command_line->get_help();
} else {
// Else do your code
print("Hello " . $this->command_line->get_argument('-n') . "!" . PHP_EOL);
}
}
Let's analyze this code in more detail.
First, we created an array with possible parameters for our script:- arg is the main name of the argument (e.g. --name ).
Mandatory if alias is not specified - alias - The abbreviated name of the element (e.g. -n ).
Mandatory if arg is not specified - help - a line that will be displayed when asking for help (with us it says that this is the name of the person)
- type - one of the three possible types of argument: 0 - for optional, 1 - for mandatory and 2 - for triggers (boolean).
It is worth noting that national and triggers themselves determine their value. For example, unspecified will take the value FALSE , and the specified trigger without parameters will become TRUE . If you do not specify the required one, while submitting any of the existing parameters to the input, an error will come out and the script will not be executed.
So, setting the config, we initialize our library and check the incoming parameters for validity, just like in Form_validation!
if (!$this->command_line->valid_input())
If it is not valid - there are no parameters, or some error occurred, we simply call the method, which will tell us what is wrong:
print $this->command_line->get_help();
If everything went as it should, then we can execute our code by getting the argument parameters using the get_argument () method . You can refer to them both by their full name and by alias:
$this->command_line->get_argument('-n') === $this->command_line->get_argument('--name')
4. Now that everything is ready, open the command line and check our script:
php index.php cli home
Since you did not enter any arguments, he will friendlyly tell you how to use it:
Usage: php index.php cli hello [OPTIONS]
Options are:
-n, --name the person's name, we say hello to
So, you need to enter a name:
php index.php cli home -n Habrahabr
Which will output the following:
Hello Habrahabr!
UPD: in addition, the script can also take as a parameter a long string in quotation marks, after clearing it from them:
php index.php cli home -n "Petr Ivanovich"
Hello Petr Ivanovich!
Conclusion
As you can see, the library is very primitive, and implements only the very base of functions that might be required when working with the command line. Nevertheless, it can save a little time on developing system cli scripts used, say, for tasks in cron. This is really convenient - you can use all the written code of your models and libraries to create system tasks, such as, for example, generating a site map or recounting any indicators.
I also want to warn in advance that while the version of the library is still quite raw, and any bugs may occur. I will be happy to hear your comments on this matter, and I will also be glad if you contribute by joining the development on github .