
Semi-Prezi in 10 Minutes in .NET and WPF
It will be about creating a program for playing a presentation like the Prezi web service, although a similar concept could be seen earlier in PowerPoint 2010 in one of the standard templates.
The difference from the usual presentation in the form of slides is the presence of a solid background, like a canvas for content, where moving from one area with content to another occurs by moving, zooming in and out.
So, we are doing something similar in .NET and WPF. Personally, I like VB.NET (probably due to the lack of {...}, which occupy an entire line in C #).
Required: VisualStudio, Framework 4 and a healthy picture, for example 4800x3800, drawn in Photoshop with some content (you can stick a couple of slides with export from PowerPoint):
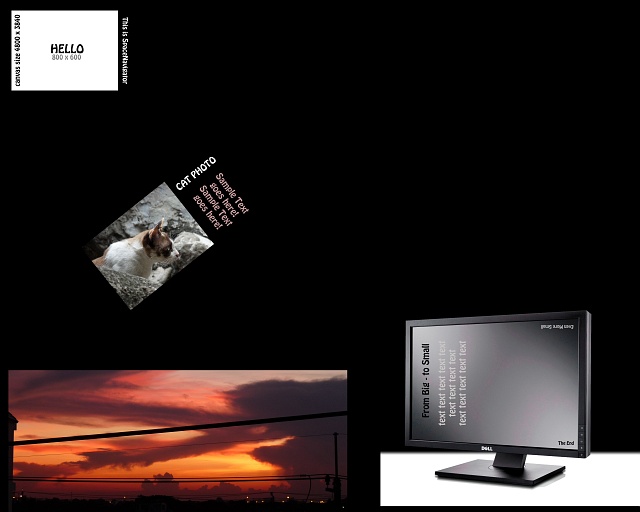
In VisualStudio we create an empty WPF project and add the following things to the “form”:
ImageBg background image with by stretching under the form, the ScrollViewer component with hidden bars and into it another Image1 image without stretching.
We set the variables:
We do preloading the images in Window_Loaded: The bg.jpg files with some background (for example, black) and content.jpg need to be put in the Debug folder. You can also add a .png transparency mask to the content, but so far this is superfluous. After loading the image, we prescribe the transformation parameters there: i.e. a transformation group is created, which includes three separate types of transformation. We set the initial state of the content so that the large picture fits into the screen with a small gap (humanly this is done by the Stretch and Margin properties, but in this case it is necessary through transformation): Downloading the lists of steps from the file:
File structure: each line is a new step, and there are 5 parameters in the line, separated by a comma (scale, angle, x and y coordinates, time of movement) We
add movement processing between steps: There are just 4 animations for scale, angle and coordinates (of course, variables can be was set in advance for code cleanliness, but it’s also almost compact). Actually that's all in essence. It remains to write a set of steps in the text file loc.ini, for example: And activate switching between steps in the code: If you clicked on the left side of the content image - one step back, the right one - forward. For normal operation, of course, you need a tuning mode with the subsequent saving of new parameters. But I won’t describe it here, but the working beta version can be downloaded here
. To run, you need Framework 4 .
You can also improve the program in many ways - add support for vector content (which will be closer to Prezi), save the project, add multi-touch to configure, quick navigation, change the canvas content and more.
The difference from the usual presentation in the form of slides is the presence of a solid background, like a canvas for content, where moving from one area with content to another occurs by moving, zooming in and out.
So, we are doing something similar in .NET and WPF. Personally, I like VB.NET (probably due to the lack of {...}, which occupy an entire line in C #).
Required: VisualStudio, Framework 4 and a healthy picture, for example 4800x3800, drawn in Photoshop with some content (you can stick a couple of slides with export from PowerPoint):
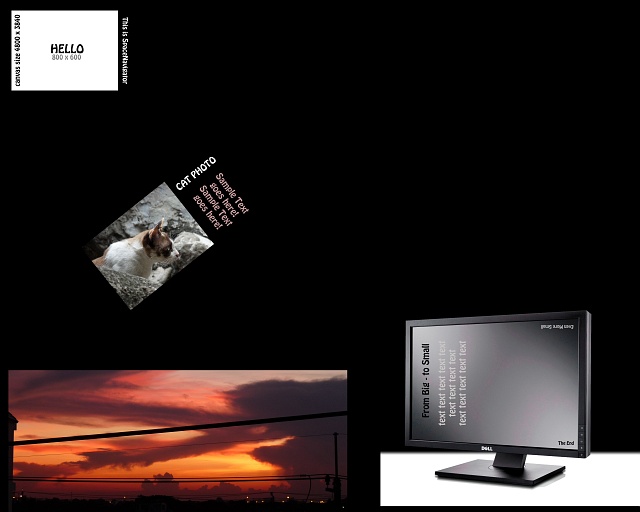
In VisualStudio we create an empty WPF project and add the following things to the “form”:
ImageBg background image with by stretching under the form, the ScrollViewer component with hidden bars and into it another Image1 image without stretching.
![]()
![]()
We set the variables:
'базовый путь к файлам
Dim base_dir As String = System.AppDomain.CurrentDomain.BaseDirectory()
'трансформация перемещением
Dim trTranslate As New TranslateTransform
'трансформация поворотом
Dim trRotate As New RotateTransform
'трансформация масштабированием
Dim trScale As New ScaleTransform
'шаг (как-бы слайд)
Dim stp As Integer = 0
'кол-во шагов
Dim stp_count As Integer = 0
'функция нелинейности для анимации перемещения
Dim ease As New PowerEase
'параметры изначального положения (масштаб, угол, координаты)
Dim init_s, init_a As Double
Dim init_x, init_y As Integer
'параметры нового шага (масштаб, угол, координаты и время перемещения)
Dim new_z(99) As Double
Dim new_a(99) As Double
Dim new_x(99) As Double
Dim new_y(99) As Double
Dim new_t(99) As Double
We do preloading the images in Window_Loaded: The bg.jpg files with some background (for example, black) and content.jpg need to be put in the Debug folder. You can also add a .png transparency mask to the content, but so far this is superfluous. After loading the image, we prescribe the transformation parameters there: i.e. a transformation group is created, which includes three separate types of transformation. We set the initial state of the content so that the large picture fits into the screen with a small gap (humanly this is done by the Stretch and Margin properties, but in this case it is necessary through transformation): Downloading the lists of steps from the file:
ImageBg.Source = New BitmapImage(New Uri(base_dir + "bg.jpg"))
Image1.Source = New BitmapImage(New Uri(base_dir + "content.jpg"))
Dim transf_grp As New TransformGroup
transf_grp.Children.Add(trTranslate)
transf_grp.Children.Add(trRotate)
transf_grp.Children.Add(trScale)
Image1.RenderTransform = transf_grp
init_s = Math.Round(Grid1.ActualWidth / (Image1.Source.Width + 50), 2)
init_a = 0
init_x = 50
init_y = (Grid1.ActualHeight - (Image1.Source.Height) * init_s) * 2
trScale.ScaleX = init_s
trScale.ScaleY = init_s
trRotate.Angle = init_a
trTranslate.X = init_x
trTranslate.Y = init_y
If File.Exists(base_dir + "loc.ini") Then
Dim oRead As System.IO.StreamReader = File.OpenText(base_dir + "loc.ini")
Dim data(99) As String
Dim i As Integer = 0
Do While oRead.Peek >= 0
i += 1
data(i) = oRead.ReadLine()
Dim param() As String = data(i).Split(",")
new_z(i) = CDbl(param(0))
new_a(i) = CDbl(param(1))
new_x(i) = CDbl(param(2))
new_y(i) = CDbl(param(3))
new_t(i) = CDbl(param(4))
If new_z(i) <> 0 Then stp_count += 1
Loop
oRead.Close()
End If
File structure: each line is a new step, and there are 5 parameters in the line, separated by a comma (scale, angle, x and y coordinates, time of movement) We
add movement processing between steps: There are just 4 animations for scale, angle and coordinates (of course, variables can be was set in advance for code cleanliness, but it’s also almost compact). Actually that's all in essence. It remains to write a set of steps in the text file loc.ini, for example: And activate switching between steps in the code: If you clicked on the left side of the content image - one step back, the right one - forward. For normal operation, of course, you need a tuning mode with the subsequent saving of new parameters. But I won’t describe it here, but the working beta version can be downloaded here
Private Sub NavigationStep()
Dim Z_anim As New DoubleAnimation(trScale.ScaleX, new_z(stp), TimeSpan.FromSeconds(new_t(stp)))
Dim A_anim As New DoubleAnimation(trRotate.Angle, new_a(stp), TimeSpan.FromSeconds(new_t(stp) / 2))
Dim X_anim As New DoubleAnimation(trTranslate.X, new_x(stp), TimeSpan.FromSeconds(new_t(stp)))
Dim Y_anim As New DoubleAnimation(trTranslate.Y, new_y(stp), TimeSpan.FromSeconds(new_t(stp)))
ease.EasingMode = EasingMode.EaseInOut
Z_anim.EasingFunction = ease
A_anim.EasingFunction = ease
X_anim.EasingFunction = ease
Y_anim.EasingFunction = ease
trScale.BeginAnimation(ScaleTransform.ScaleXProperty, Z_anim)
trScale.BeginAnimation(ScaleTransform.ScaleYProperty, Z_anim)
trRotate.BeginAnimation(RotateTransform.AngleProperty, A_anim)
trTranslate.BeginAnimation(TranslateTransform.XProperty, X_anim)
trTranslate.BeginAnimation(TranslateTransform.YProperty, Y_anim)
End Sub
1.5,0,-70,-40,1.5
2,-100,-1200,-100,2
3,85,110,-640,1
4,35,-735,-1800,0.5
Private Sub Image1_MouseUp(ByVal sender As System.Object, ByVal e As System.Windows.Input.MouseButtonEventArgs) Handles Image1.MouseUp
If Not setup_mode And Not sel_mode Then
If e.GetPosition(Grid1).X < Grid1.ActualWidth / 2 Then
stp -= 1
If stp <= 0 Then stp = stp_count
NavigationStep()
End If
If e.GetPosition(Grid1).X >= Grid1.ActualWidth / 2 Then
stp += 1
If stp = stp_count + 1 Then stp = 1
NavigationStep()
End If
End If
End Sub
. To run, you need Framework 4 .
You can also improve the program in many ways - add support for vector content (which will be closer to Prezi), save the project, add multi-touch to configure, quick navigation, change the canvas content and more.