Maniac minimization (in pursuit of byte)
Hello World,
This topic is about how you can pre-refactor code so as to improve its minimization. I recently minimized the
Helios Kernel library (which I wrote about the day before yesterday ) before the release . The library source code weighs 28,112 bytes, it has generous comments, and therefore it is compressed with a half-kick by the YUI compressor to 7083 bytes. Not that it seemed to me that 7 kilobytes is too bold. But just by looking at the minimized code with my own eyes, I could see a bunch of places where you could save more:
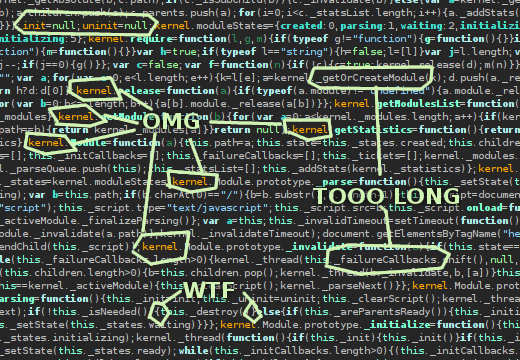
Let's see what can be done with the code to turn 7083 bytes into4009 3937.
But before you begin, two caveats:
In general, this article is not about comparing minimizers, but in the process I noticed that the YUI compressor has a featurebug : it does not remove curly braces from code blocks consisting of one line. Moreover, he adds curly braces, even if they were not in the original (marked with the WTF tag in the first picture ). I took it as rudeness and, without hesitation, switched to using the online minimizer http://jscompress.com/ . However, the rest of the arguments apply to any minimizer of your choice.
To get started, let's wrap all the code in a large anonymous function that will be called right away (if this is not initially done). Then we can use the local scope of this function. How this will save bytes will be shown below. The most compact way to wrap code in an anonymous function is as follows:
Surely, the code has a large number of auxiliary objects that are not included in the public API. Since there is no native way in Javascript to indicate that the object is private, some kind of convention is usually used. Most often, such objects are named starting with an underscore: " _ ". Usually, the minimizer replaces the names of local variables with single-letter ones, but leaves the names of “private” objects unchanged, because it does not make bold assumptions as to how we designate “private” objects. But it doesn’t matter to us how these objects will be called in the minimized code, so you can rename them manually:
Here you need to be careful. First, remember to replace the names of private functions and variables not only in declarations, but also where they are used. Secondly, you need to keep track of the logic of the code, and avoid name intersections. For example, if a function a has already been declared for some type in the prototype, the private property of this object cannot be called the same name. This is an obvious thing, but it is easy to miss if you do not pay special attention to it.
In addition, private objects are often declared not only in all sorts of constructors / initializers. Javascript allows you to complement objects on the fly. In theory, all private identifiers in the code can be carefully replaced with single-letter ones:
“Public” objects are those that are part of the API, and we need them to be named exactly as they were originally named. But if the “public” object is used inside the code too often (well, say, at least once), and its name is too long (well, say, more than two bytes), then it makes sense to make it an alias:
In this example, after such a change, the variable a will be declared as local, and the variable myObject as global (provided that the identifier myObject is used for the first time.
Now you can go over the code, find all objects that are not only declared, but also used, and make them an alias:
And again, the main thing is not to get confused in the scope and not to name variables from the same scope by the same name. In the examples above, an object of type MyObj already has a private property b and a private method c , and the new local variables b and c fall into the scope of the Big Anonymous Function, into which we wrapped all the code at the very beginning (we wrapped it, right? ?)
In addition, we can do aliases to some public properties, but only to those that contain complex objects:
If we do aliases for simple objects, this will copy the contents, and the alias will point to another object.
Now let's take advantage of the fact that you can declare variables separated by commas, using the word var once. In the simplest case, it looks like this:
In general, you need to pull all the declarations to the top of the function and write them using one var . I’ll write about optimizing the for () loop below. And you also need to collect all the local declarations inside our Large Hadron Functions and also put them under one var at the beginning. These are exactly the aliases that we created in the previous section. All code should convert like this:
Note that in this example, the variables b , c and the like remain declared local to the Big Function. This way we will save as many vars as there were in the function (well, except for one).
And still it is necessary to watch that code logic has not changed. We are changing the order of the lines, so theoretically it can happen that some object will be used before it is initialized, this cannot be allowed.
For each declared type and its constructor, you can save a lot of money on the word protoype - it's too long. To do this, we describe the entire prototype for future objects of this type in the form of a single hash:
As you can see, for this you need to remember to replace " = " with " : " and separate method declarations with commas. This method will not work for the case when you need to supplement some prototype for a type constructor declared somewhere else (because we completely redefine the prototype with such a record).
Almost all cycles and many conditions can be optimized:
But here, too, you need to be careful so as not to violate the logic of the code.
It happens that there are values that are used more than once. They can also be put into variables:
It often happens that the code contains redundant information "for clarity", which you can get rid of. But here, as elsewhere, you need to carefully monitor what we remove:
This is an interesting trick, which is useful in cases where one local variable is declared inside the function (or if the variable is declared without initialization). Here we save on one var , but we have to duplicate the variable name:
Here we use parameters instead of local variables, but they behave exactly the same. This trick is not suitable in those cases when the function takes an unknown number of parameters in advance. Most often, it allows you to get rid of almost all var 's in the code.
After processing the code in the described ways, I fed the script to jscompress.com . After a little thought, he gave me such a mess for 4009 bytes. Bon Appetit!

By the way, I will give out pluses to karma to those who find and describe in the comments what else you can trim in this mess :-)
nano_freelancer suggested some good ideas:
In addition, most nulls can also be replaced with 0 (but not all).
Code size reduced to 3937 bytes :-)
Offtopic: source and minimized codes that I worked with are available for download on the project home page: http://home.gna.org/helios/kernel/
This topic is about how you can pre-refactor code so as to improve its minimization. I recently minimized the

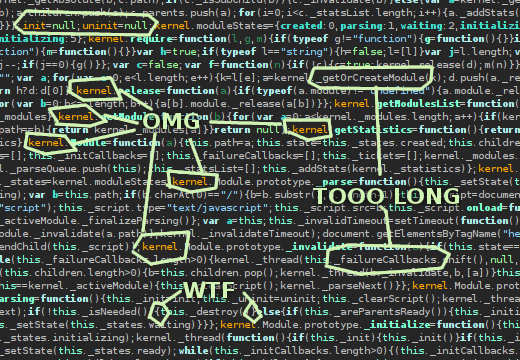
Let's see what can be done with the code to turn 7083 bytes into
But before you begin, two caveats:
- We will not use all sorts of "dirty" tricks (like var a = this or var f = false ), which theoretically lead to a slowdown. Performance is assumed to be more important than file size.
- At each step, I ran the code through a set of tests. It often happens that after some change everything stops working. If during the manual optimization process you do not test the code (or if you don’t have any tests at all), then the code that will turn out in the end will most likely not work.
Minimizer Selection
In general, this article is not about comparing minimizers, but in the process I noticed that the YUI compressor has a feature
Big Anonymous Function
To get started, let's wrap all the code in a large anonymous function that will be called right away (if this is not initially done). Then we can use the local scope of this function. How this will save bytes will be shown below. The most compact way to wrap code in an anonymous function is as follows:
It was | has become |
---|---|
|
|
"Private" objects
Surely, the code has a large number of auxiliary objects that are not included in the public API. Since there is no native way in Javascript to indicate that the object is private, some kind of convention is usually used. Most often, such objects are named starting with an underscore: " _ ". Usually, the minimizer replaces the names of local variables with single-letter ones, but leaves the names of “private” objects unchanged, because it does not make bold assumptions as to how we designate “private” objects. But it doesn’t matter to us how these objects will be called in the minimized code, so you can rename them manually:
It was | has become |
---|---|
|
|
|
|
|
|
Here you need to be careful. First, remember to replace the names of private functions and variables not only in declarations, but also where they are used. Secondly, you need to keep track of the logic of the code, and avoid name intersections. For example, if a function a has already been declared for some type in the prototype, the private property of this object cannot be called the same name. This is an obvious thing, but it is easy to miss if you do not pay special attention to it.
In addition, private objects are often declared not only in all sorts of constructors / initializers. Javascript allows you to complement objects on the fly. In theory, all private identifiers in the code can be carefully replaced with single-letter ones:
It was | has become |
---|---|
|
|
"Public" objects
“Public” objects are those that are part of the API, and we need them to be named exactly as they were originally named. But if the “public” object is used inside the code too often (well, say, at least once), and its name is too long (well, say, more than two bytes), then it makes sense to make it an alias:
It was | has become |
---|---|
|
|
In this example, after such a change, the variable a will be declared as local, and the variable myObject as global (provided that the identifier myObject is used for the first time.
Now you can go over the code, find all objects that are not only declared, but also used, and make them an alias:
It was | has become |
---|---|
|
|
|
|
|
|
And again, the main thing is not to get confused in the scope and not to name variables from the same scope by the same name. In the examples above, an object of type MyObj already has a private property b and a private method c , and the new local variables b and c fall into the scope of the Big Anonymous Function, into which we wrapped all the code at the very beginning (we wrapped it, right? ?)
In addition, we can do aliases to some public properties, but only to those that contain complex objects:
It was | has become |
---|---|
|
|
If we do aliases for simple objects, this will copy the contents, and the alias will point to another object.
Putting Var 's
Now let's take advantage of the fact that you can declare variables separated by commas, using the word var once. In the simplest case, it looks like this:
It was | has become |
---|---|
|
|
|
|
In general, you need to pull all the declarations to the top of the function and write them using one var . I’ll write about optimizing the for () loop below. And you also need to collect all the local declarations inside our Large Hadron Functions and also put them under one var at the beginning. These are exactly the aliases that we created in the previous section. All code should convert like this:
It was | has become |
---|---|
|
|
Note that in this example, the variables b , c and the like remain declared local to the Big Function. This way we will save as many vars as there were in the function (well, except for one).
And still it is necessary to watch that code logic has not changed. We are changing the order of the lines, so theoretically it can happen that some object will be used before it is initialized, this cannot be allowed.
Prototypes
For each declared type and its constructor, you can save a lot of money on the word protoype - it's too long. To do this, we describe the entire prototype for future objects of this type in the form of a single hash:
It was | has become |
---|---|
|
|
As you can see, for this you need to remember to replace " = " with " : " and separate method declarations with commas. This method will not work for the case when you need to supplement some prototype for a type constructor declared somewhere else (because we completely redefine the prototype with such a record).
Optimization of cycles and conditions
Almost all cycles and many conditions can be optimized:
It was | has become |
---|---|
|
|
|
|
|
|
But here, too, you need to be careful so as not to violate the logic of the code.
Frequently Used Values
It happens that there are values that are used more than once. They can also be put into variables:
It was | has become |
---|---|
|
|
|
|
|
|
Throw away all unnecessary
It often happens that the code contains redundant information "for clarity", which you can get rid of. But here, as elsewhere, you need to carefully monitor what we remove:
It was | has become |
---|---|
|
|
|
|
Bonus: remove var 's
This is an interesting trick, which is useful in cases where one local variable is declared inside the function (or if the variable is declared without initialization). Here we save on one var , but we have to duplicate the variable name:
It was | has become |
---|---|
|
|
|
|
Here we use parameters instead of local variables, but they behave exactly the same. This trick is not suitable in those cases when the function takes an unknown number of parameters in advance. Most often, it allows you to get rid of almost all var 's in the code.
What happened as a result
After processing the code in the described ways, I fed the script to jscompress.com . After a little thought, he gave me such a mess for 4009 bytes. Bon Appetit!

By the way, I will give out pluses to karma to those who find and describe in the comments what else you can trim in this mess :-)
Update
nano_freelancer suggested some good ideas:
- replace all true and false with 1 and 0, respectively
you can put a comma after the loop statement and put all the statements from statements separated by a comma (instead of a semicolon) - save 2 bytes (curly braces). But this is only applicable for cases where statement itself does not contain complex operators.for (initial;condition;loop statement) {statements}
In addition, most nulls can also be replaced with 0 (but not all).
Code size reduced to 3937 bytes :-)
Offtopic: source and minimized codes that I worked with are available for download on the project home page: http://home.gna.org/helios/kernel/