
Developing an oAuth Protocol-Based Application for the Twitter API in PHP
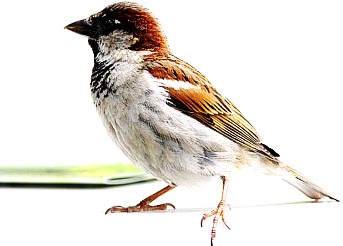
In this article I will talk about working with the Twitter API using the OAuth protocol in PHP.
The OAuth protocol provides the application with access to user data without passing the user login and password to it. New application authorization rules require the use of “OAuth” technology to work with Twitter starting August 31.
The test application, which will turn out in the end, will be able to display the user's message feed, the feed of the latest statuses of his followers, and by clicking on the button next to each follower or friend status, you can read the entire feed of this user.
So, for starters, create a new application at this link.
Now we have the necessary data that should be saved in config.php
define('CONSUMER_KEY', 'Your consumer key');
define('CONSUMER_SECRET', 'You consumer secret');
define('OAUTH_CALLBACK', 'http://yousite.ru/callback.php');
On the connection page with twitter connect.php we connect the above data and check its availability: We begin the connection process (go to the redirect.php page): We connect the library for working with the Twitter API and the application account data to redirect.php Create a TwitterOAuth object based on the account recording of our application: Next time we get tokens from Twitter and save them: a time-based token send the user authorization in Twitter: If the received token old, the net session and send the user to a page with of the connections to Twitter: On page clearsessions.php call:
if (CONSUMER_KEY === '' || CONSUMER_SECRET === '') {
echo 'You need a consumer key and secret to test the sample code. Get one from https://twitter.com/apps'; exit;}
$content = 'Sign in with Twitter';
require_once('twitteroauth/twitteroauth.php');
require_once('config.php');
$connection = new TwitterOAuth(CONSUMER_KEY, CONSUMER_SECRET);
$_SESSION['oauth_token'] = $token = $request_token['oauth_token'];
$_SESSION['oauth_token_secret'] = $request_token['oauth_token_secret'];
$url = $connection->getAuthorizeURL($token);
if (isset($_REQUEST['oauth_token']) && $_SESSION['oauth_token'] !== $_REQUEST['oauth_token']) {
$_SESSION['oauth_status'] = 'oldtoken';
header('Location: ./clearsessions.php');
session_start();
session_destroy();
header('Location: ./connect.php');
If everything went well, then we create a TwitterOAuth object based on our application account and temporary tokens: Now we get the Twitter access key, which must be saved for future use: Temporary tokens are no longer needed: Next, go to our application page : On the index page .php save the user token to a variable: Create a TwitterOAuth object based on our application account and user tokens: Now we can use various methods to get user data. For example, get user account information.
$connection = new TwitterOAuth(CONSUMER_KEY, CONSUMER_SECRET, $_SESSION['oauth_token'], $_SESSION['oauth_token_secret']);
$access_token = $connection->getAccessToken($_REQUEST['oauth_verifier']);
$_SESSION['access_token'] = $access_token;
unset($_SESSION['oauth_token']);
unset($_SESSION['oauth_token_secret']);
header('Location: ./index.php');
$access_token = $_SESSION['access_token'];
$connection = new TwitterOAuth(CONSUMER_KEY, CONSUMER_SECRET, $access_token['oauth_token'], $access_token['oauth_token_secret']);
$content_user = $connection->get('account/verify_credentials');
$array_user = (array)$content_user;
$user_id=$array_user["id"];
Based on the id of the user, you can get messages from his feed by indicating id in the parameter array (in the parameter array you can also indicate the number of displayed entries):
$content_friends=$connection->get('statuses/friends_timeline',array('id' => $user_id));
Recent messages of all user followers:
$content_followers=$connection->get('statuses/followers', array('id' => $user_id));
Own user message feed (by default, only 20 entries are displayed, the maximum number of displayed 200).
$content_userline=$connection->get('statuses/user_timeline', array('id' => $user_id,'count'=>200));
Other methods can be called on the same principle.
General Twitter feed: api.twitter.com/1/statuses/public_timeline.xml The
public messages that you wrote: api.twitter.com/1/statuses/mentions.xml?count=5&page=1
My retweets: api.twitter.com /1/statuses/retweeted_by_me.xml?count=5&page=3
Retweets me:api.twitter.com/1/statuses/retweeted_to_me.xml?count=5&page=3
And other methods.
echo print_r($content_friends); // выводим результат запроса
The result when receiving the message feed will be an array of stdClass Object elements:
[0] => stdClass Object
(
[created_at] => Sat Sep 04 11:01:48 +0000 2010
[in_reply_to_screen_name] =>
[source] => IncredebleTB
[ retweeted] =>
[truncated] =>
[in_reply_to_status_id] =>
[in_reply_to_user_id] =>
[contributors] =>
[place] =>
[coordinates] =>
[user] => stdClass Object
(
[show_all_inline_media] =>
[profile_background_image_url] => s.twimg.com/a/1283555538/images/themes/theme16/bg.
[favourites_count] => 0
[profile_image_url] => a0.twimg.com/profile_images/1105647496/robot_normal.jpg
[description] => Web design, web application development, website development
[contributors_enabled] =>
[profile_sidebar_fill_color] => f2f2f2
[ url] => dandreev.com
[geo_enabled] =>
[profile_background_tile] =>
[screen_name] => incredibleTB
[lang] => en
[created_at] => Mon Apr 26 20:32:14 +0000 2010
[profile_sidebar_border_color] => a8a8a8
[location] => Moscow
[verified] =>
[follow_request_sent] =>
[following] => 1
[profile_background_color] => 9AE4E8
[followers_count] =>99
[protected] =>
[profile_text_color] => 141414
[name] => Dmitry Andreev
[listed_count] => 0
[profile_use_background_image] => 1
[time_zone] => Quito
[friends_count] => 48
[id] => 137459247
[statuses_count ] => 900
[notifications] =>
[utc_offset] => -18000
[profile_link_color] => 0084B4
)
[geo] =>
[retweet_count] =>
[id] => 22967688386
[favorited] =>
[text] => Craigslist Censored: Adult Section Comes Down bit.ly/9evRKN via (@techcrunch)
)
You can access them, for example, like this: The library for oAuth in php can be downloaded here
$array_friends = (array)$content_friends;
foreach ($array_friends as $friends_sets)
{
$friends_sets=(array)$friends_sets; // преобразуем stdClass Object в массив
$friend_name=$friends_sets["user"]->name; // доступ к полям stdClass Object
}
.
The application that turned out in the end is here . The application contains 3 pagination tapes (followers, friends and a personal tape of a user). Additionally, the jTweetsAnywhere library is connected, which allows displaying information about each user (the Connect with Twitter button at the top).