
ASP.NET MVC 3: A detailed overview of innovations
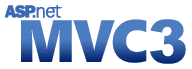
More than four months after the final release of the ASP.NET MVC 2 framework , the development team is pleased to present the first preliminary version of ASP.NET MVC 3 . The announcement and download link can be found here .
This article will give an overview of the innovations and changes that have occurred in the new version of the framework.
Compatibility and support for the .NET Framework 4.0
Unlike the previous version, ASP.NET MVC 3 only supports the fourth version of the .NET Framework . In addition, developer components for integration into Visual Studio are only supported for Visual Studio 2010 and Visual Web Developer Express 2010 .
ASP.NET MVC 3 framework can be installed together with ASP.NET MVC 2, they can be used separately from each other.
Upgrading from a previous version to ASP.NET MVC 3
The easiest way to update projects from previous versions of the framework to a new preliminary one is to create a new MVC 3 project and transfer all the components of the old project into it: controllers, views, auxiliary files.
To manually upgrade projects based on ASP.NET MVC 2 to the new version of ASP.NET MVC 3 Preview 1, you must do the following:
- replace in all web.config files the lines System.Web.Mvc, Version = 2.0.0.0 with the lines System.Web.Mvc, Version = 3.0.0.0 ;
- remove from the project a link to the System.Web.Mvc assembly of the second version and add the assembly of the third version of the framework;
- edit .csproj project files , replacing the value of the ProjectTypeGuids element with {F85E285D-A4E0-4152-9332-AB1D724D3325} to {E53F8FEA-EAE0-44A6-8774-FFD645390401} ;
- in case the project refers to the assemblies compiled for ASP.NET MVC 2, add the following code to the configuaton section of the web.config file:
Innovations in ASP.NET MVC 3 Preview 1
The new version of the framework has a number of important innovations.
Razor View Engine Support
Starting with this version, ASP.NET MVC introduces support for the new Razor view markup syntax . Not so long ago, Razor was introduced as the basic syntax for the views in the WebMartix web development tool project . Razor is now available for developing MVC applications.
Razor is a syntax optimized for working with HTML code. The ASP.NET Web Forms engine focuses a lot on controls, and its code looks overloaded among HTML code. Razor, on the other hand, offers an extremely optimized solution that will succinctly integrate presentation logic into HTML code.
Consider an example. Below is a form built using the old presentation engine (left) and the new Razor engine (right).


The main difference between Razor that catches your eye is the lack of opening and closing sets “<%%>”. Indeed, even the @using grouping syntax does not contain closing elements and is represented only by the trailing “}” character. Compare this with the need to write “<%}%>” in the WebForms version, and you will understand what the main focus of Razor development is - the productivity of the developer by reducing the code and its brevity.
Especially, the convenience of Razor is manifested when creating branches in the code, for example, here is what some code looks like in WebForms and Razor:

![image [40] image [40]](http://microgeek.ru/upload/blog/aspnet/a7b/a7bfce70692963a046ca55754f9aa940.png)
It’s very tedious to type branches that require clipping conditions from the markup with the elements “<%%>”. On the other hand, look at how harmoniously markup branches look when using Razor. The same advantage is demonstrated in many other cases, such as cycles.
Razor has differences not only in the different syntax of opening and closing characters. So, for example, below is the code for configuring the page and setting its title for WebFroms:

The same code, but in the Razor style, is presented below:

You can learn much more details about the Razor presentation engine from this translation of Scott Guthrie's article.
Support for multiple presentation engines in Visual Studio
With the creation of the new Razor presentation engine, MVC developers took care of giving the developer the choice of which mechanism he would use. Visual Studio tools now have the ability to select a view engine when creating a new view.

Dynamic properties of View and ViewModel
Since MVC3 only supports .NET 4.0, the framework developers were able to take advantage of the new .NET version in ASP.NET MVC.
One of these benefits is support for new dynamic types. In MVC 3, the familiar ViewData property is represented as two dynamic implementations of View and ViewModel . This allows you instead of the following code:
ViewData ["Title"] = "The Title"; ViewData ["Message"] = "Hello World!";
initialize the values differently:
ViewModel.Title = "The Title";
ViewModel.Message = "Hello World!";
The ability to instantly create extensions for objects is a dynamic type property in .NET 4.0. Access to fields in the context of the view in this case will be simplified:
View.Title
View.Message
Dependency Injection Support
In MVC 3, developers increased the possibilities of expanding the framework and added support for injecting code out of the box without the need to resort to their own implementations.
ASP.NET MVC 3 Preview 1 adds the ability to inject in the following places:
- creation of a controller factory;
- creation of controllers;
- different stages of the work of presentation engines, both Web Forms and Razor;
- action filters

After that, the developer registers a new instance using the global static class, which is defined as follows:

The developer calls the SetCurrent method to set the new implementation of IServiceLocator .
After adding a new implementation, the ASP.NET MVC 3 engine will use it for the following purposes:
- instantiation of the controller factory and the controllers themselves;
- instantiation of presentation engines and presentation pages themselves;
- instantiation of action filters.
- for the Model Binder mechanism ;
- for providers of values (Value Providers);
- for validation providers;
- for model metadata providers.
- ControllerBuilder.GetControllerFactory will first refer to the existing implementation of IServiceLocator and only then, if there is no implementation, use the old mechanism for creating the controller factory;
- When creating controllers, the DefaultControllerFactory will first refer to the existing implementation of IServiceLocator and only then, if there is no implementation, use the old mechanism for creating controllers;
- the function DefaultControllerFactory.ReleaseController - release the controller - will be done only through the implementation of IServiceLocator , if there is no implementation, then the default implementation from the special interface IMvcServiceLocator will be used ;
- ViewEngineCollection , as before, will allow you to add collections of view engines, but besides this in MVC 3 this collection will virtually contain all view engines registered in the implementation of IServiceLocator . Which means method calls. FindView or. FindPartialView will return generalized values, either manually added or defined using the IServiceLocator implementation ;
- WebFormView has been updated so that pages and controls are created using the IServiceLocator implementation or the old way through Activator.CreateInstance , if you cannot create the page through the IServiceLocator implementation .
Global filters
MVC 3 adds a global filter mechanism that allows you to define the filters that will be invoked each time the actions of any controller are called. To add a filter, the global static class GlobalFilters is used :
GlobalFilters.Filters.Add (new MyActionFilter ());
You can override the global filter mechanism by creating an injection for the new IFilterProvider interface . This will allow you to create your own logic for executing global filters.
JsonValueProviderFactory Value Provider
The new value provider will allow your actions to receive requests with parameter sets in JSON format and map them to the parameters of the action method. Previously, this functionality was hosted in the ASP.NET MVC Futures library .
For example, if a POST request with the application / json MIME type contains the value:
{“ProductName”: “Milk”, “Cost”: “12.0”}
Then, using the JsonValueProviderFactory provider , these values are automatically assigned to the parameter with the ProductModel type in the next step :
[HttpPost]
public ActionResult SaveProduct (ProductModel productModel)
{
...
}
where the type ProductModel is declared as
public class ProductModel
{
publiс string ProductName {get; set;}
public string Cost {get; set;}
}
Support for .NET 4.0 Validation Attributes and IValidatableObject
The ValidationAttribute class was updated in .NET 4.0 and expanded with the new overloaded IsValid method with a parameter of type ValidationContext . This parameter, in addition to the new property value, contains the validation context and an instance of the validation object. This will allow you to check not only the current property, but also other properties of the object and validate based on their values.

The example above demonstrates a comparison of two properties of an object passed in the context of validation.
In addition, a new IValidatableObject interface has been added to .NET 4.0 , which will allow you to define the validation logic at the class level of your model.

The model defined above implements the IValidatableObject interfacefor your own validation. MVC 3 supports this interface and validates the model based on it.
Interface IClientValidatable
This new interface is announced as

It is designed to provide third-party validation frameworks with information on supporting client validation.
Support for .NET 4 Metadata Attributes
MVC 3 supports the new metadata attributes that were introduced in .NET 4. For example, DisplayAttribute. A complete list of the new attributes that are really used in MVC 3 is not yet available, there is no information on this issue, and the project source codes are not yet available.
IMetadataAware
The new interface is defined as

It is designed to be able to determine the moment of creating metadata for the model and the ability to perform additional actions at this moment. This interface is used by classes inherited from AssociatedMetadataProvider, for example, the DataAnnotations metadata class, DataAnnotationsModelMetadataProvider.
New types of action results
MVC 3 introduced a number of new types for returning action results.
HttpNotFoundResult
A simple action result type that returns a result indicating that the requested resource was not found (HTTP 404). The controllers contain a new helper method, HttpNotFound (), which returns the type HttpNotFoundResult :

HttpStatusCodeResult
The new class is declared as HttpStatusCodeResult

Class is intended to return an arbitrary HTTP code as the result of an action.
As you can see, it contains two constructors that take the digital value of the HTTP code and the second takes an additional line with a description of the result.
Constant redirect
MVC3 adds support for returning action results as a permanent redirect (HTTP 301). This can be done using the following new controller methods:
- RedirectPermanent - redirects to the specified URL;
- RedirectToRoutePermanent - redirects to the current route (or you can specify optional parameters routeName and routeValues );
- RedirectToActionPermanent - redirects to the specified action (optional parameters controllerName and routeValues can be specified ).
Changes incompatible with MVC2
The order of execution of exception filters has been changed. Previously, the exception filters applied to the controller were executed earlier than the filters applied to the action (if they had the same Order value). Now and further this behavior is changing. The exception filters for actions will be executed first, followed by the filters applied to the controllers. If the Order value for these filters is different, then they will be executed as before according to the order defined in Order.
Known Issues
This release has a number of issues that will be addressed in future releases:
- Нет подсветки синтаксиса и поддержки IntelliSense в Visual Studio для движка представлений Razor;
- Отсутствует возможность использовать функцию Go To Controller при редактировании файлов .cshtml (Razor);
- Отсутствуют доступ к сниппетам Visual Studio при редактировании кода с движком представлений Razor.
Заключение
According to the results of the first preliminary release, we can confidently say that the new version of ASP.NET MVC 3 will bring a lot of new features. Functionality is already available for continuous study, and I think that with future releases the number of new features will only increase.
That is why, in order to study and test the new functionality, preliminary versions are issued in parts. I urge all ASP.NET MVC developers to pay close attention to the MVC3 Preview 1 release and devote their time to exploring its new features.