We master the simplest PIC microcontroller. Part 1
The choice of a microcontroller is usually carried out for the necessary tasks. For study, a popular MK with a minimal set of peripherals is well suited: PIC16F628A.
The first step is to download the documentation for the selected microcontroller. Just go to the manufacturer’s website and download Datasheet .
On the first pages the main characteristics of MK ( Russian description ) are listed .
The main points that we will need:
Pin layout:

Vdd - power.
Vss is the earth.
This is the minimum necessary for the work of MK.
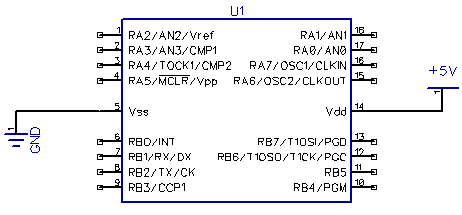
16 MK legs remain accessible. It is not difficult to calculate that the use of each leg by any module reduces the maximum number of digital ports used.
As I already wrote in previous articles, I considered the JAL compiler with the IDE JALEdit the simplest and easiest.
Download JALPack , install.
This package contains all the necessary libraries, as well as examples of their use.
Launch JALEdit. We open an example of a program for our microcontroller: 16f628a_blink.jal, so as not to spoil the source, immediately save it to a new file, for example, 16f628a_test.jal.
All code can be divided into 4 blocks:
By pressing F9 (or the corresponding button), the program will compile into ready-made firmware, and you will see how many MK resources will be used:
If you read the comments, it will become clear that this program is designed to use external 20MHz quartz.
Since we don’t have it yet, we’ll deal with the configuration and rewrite the program for using the internal generator.
Different microcotrollers have different sets of configuration bits. You can find out the purpose of each bit in the datasheet (p. 97).
In the connected library, each bit and each of its values is assigned a readable variable, it remains only to select the parameters we need.
Change the configuration for yourself:
We modify the program so that the LED blinks only when the button is pressed.
Having solved this problem, we will learn how to work with digital ports in both input mode and output mode.
Choose an unused MK leg. Take RB5 (pin 11) for example. This foot does not have additional functions, so we will not need it anywhere else.
In the digital output mode, MK can attract either food or ground to the leg.
You can connect the load to both plus and minus. The difference will only be when and in which direction the current will flow.

In the first case, current will flow from the MC when the unit is set, and in the second, to the MK when the unit is zero.
In order for the LED to light from a logical unit, let us dwell on the first option.
To limit the current through the leg (a maximum of 25 mA to a digital input or 200 mA to all ports), a current-limiting resistor is installed. Using the simplest formula, we calculate the minimum value of 125 Ohms. But since we don’t need a limit, let's take a 500 Ohm resistor (or rather, the closest suitable one).
To connect a more powerful load, you can use transistors in various versions.
Take the second unused leg anywhere - RB4 (pin 10, the PGM function specified in the pinout refers to LVP, which we disabled).
In digital input mode, the microcontroller can read two states: the presence or absence of voltage. So we need to connect the button so that in one state a plus goes on the leg, and in the second state - the earth connects to the leg.
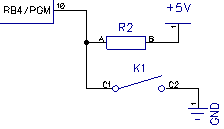
In this embodiment, the resistor is used as a pull-up. Typically, a 10 kΩ resistor is used for bracing.
You can connect not only the button, the main thing to remember is the limitation of current through the MK.
Until we forget that we have activated an external reset, we will add a similar button to the MCLR foot (pin 4).
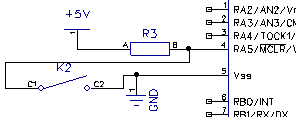
After pressing this button, the MK will start the program from scratch.
We assign variables to our LED and button:
Now, assigning the led variable the values 1 or 0 (on or off, true or false, other aliases ..) we will pull the MK to the desired foot either plus or minus, thereby lighting and extinguishing the LED, and when reading the button variable we will get 1 if the button is not pressed and 0 if the button is pressed.
Now we will write the necessary actions for us in an infinite loop (these actions will be performed continuously. In the absence of an infinite loop, the MK will freeze):
The delay is considered simple: the
frequency of the generator is 4MHz. The operating frequency is 4 times less: 1 MHz. Or 1 clock = 1 μs. 500,000 μs = 0.5 s.
We compile the firmware:
Now we need to write this firmware to MK, assemble the device according to the scheme and verify that everything worked out as it should.
All the same scheme:
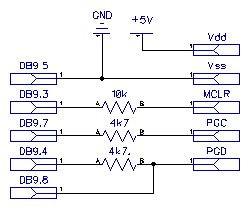
We look at the pinout:
We solder ...

We connect to the computer.
Download and run WinPic800 .
Go to Settings-> the Hardware , select JDM and the port number on which hangs the programmer
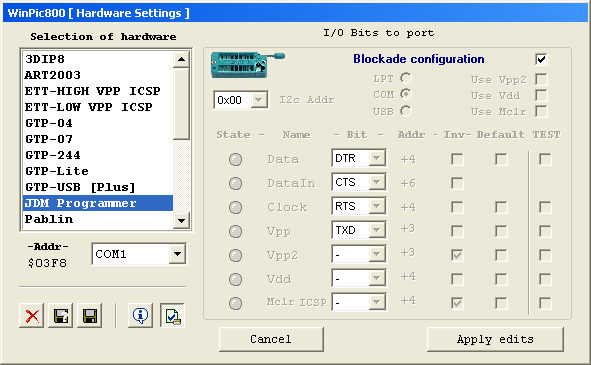
Click the Hardware the Test , then Detect Device
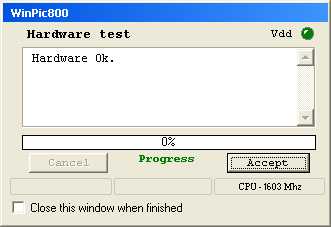
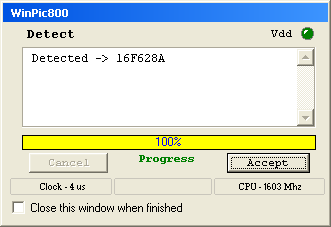
Open our firmware pic628a_test.hex

tab Setting You can check that the configuration bits on display is correct, if you want then you can change

Program All , then Verify All

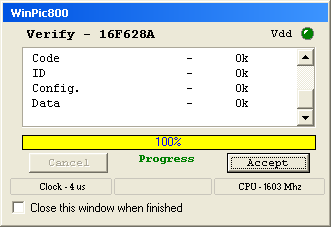
If no errors have occurred, continue to solder.
Final Scheme:
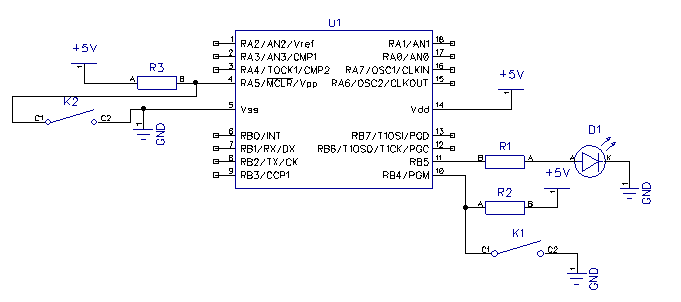
The button on the MCLR can be soldered at will, but a pull-up is required.
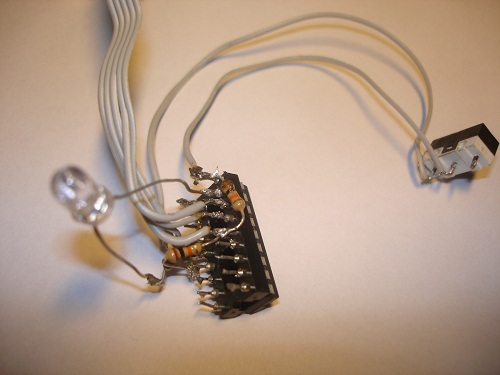
The result of the work can be seen in the video.
So, we have the simplest device on the microcontroller: an LED flasher.
Now we need to learn how to use all the remaining peripherals, but more on that in the next article .
The first step is to download the documentation for the selected microcontroller. Just go to the manufacturer’s website and download Datasheet .
On the first pages the main characteristics of MK ( Russian description ) are listed .
The main points that we will need:
- the microcontroller contains an internal generator at 4 MHz, you can also connect external quartz with a frequency of up to 20 MHz
- 16 feet of the microcontroller can be used as digital inputs / outputs
- there are 2 analog comparators
- 3 timers
- Ccp module
- USART module
- 128 bytes non-volatile EEPROM
Pin layout:

Vdd - power.
Vss is the earth.
This is the minimum necessary for the work of MK.
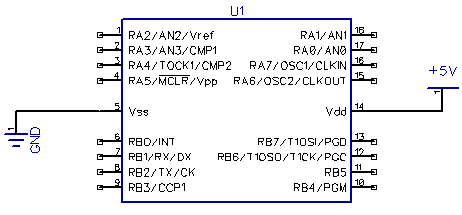
16 MK legs remain accessible. It is not difficult to calculate that the use of each leg by any module reduces the maximum number of digital ports used.
Compiler
As I already wrote in previous articles, I considered the JAL compiler with the IDE JALEdit the simplest and easiest.
Download JALPack , install.
This package contains all the necessary libraries, as well as examples of their use.
Launch JALEdit. We open an example of a program for our microcontroller: 16f628a_blink.jal, so as not to spoil the source, immediately save it to a new file, for example, 16f628a_test.jal.
All code can be divided into 4 blocks:
- MK selection and configuration
include 16f628a -- подключение библиотеки нашего МК
--
-- This program assumes a 20 MHz resonator or crystal
-- is connected to pins OSC1 and OSC2.
pragma target clock 20_000_000 -- oscillator frequency
-- configuration memory settings (fuses)
pragma target OSC HS -- HS crystal or resonator
pragma target WDT disabled -- no watchdog
pragma target LVP disabled -- no Low Voltage Programming
pragma target MCLR external -- reset externally
-- - declaration of variables, procedures, functions
alias led is pin_A0
pin_A0_direction = output - settings and calculations to the main cycle
enable_digital_io() -- переключение всех входов\выходов на цифровой режим
- endless cycle of basic actions MK
forever loop
led = on
_usec_delay(250000)
led = off
_usec_delay(250000)
end loop
By pressing F9 (or the corresponding button), the program will compile into ready-made firmware, and you will see how many MK resources will be used:
Code: 58/2048 Data: 4/208 Hardware Stack: 0/8 Software Stack: 80
If you read the comments, it will become clear that this program is designed to use external 20MHz quartz.
Since we don’t have it yet, we’ll deal with the configuration and rewrite the program for using the internal generator.
Configuration
Different microcotrollers have different sets of configuration bits. You can find out the purpose of each bit in the datasheet (p. 97).
In the connected library, each bit and each of its values is assigned a readable variable, it remains only to select the parameters we need.
-- Symbolic Fuse definitions
-- -------------------------
--
-- addr 0x2007
--
pragma fuse_def OSC 0x13 { -- oscillator
RC_CLKOUT = 0x13 -- rc: clkout on ra6/osc2/clkout, rc on ra7/osc1/clkin
RC_NOCLKOUT = 0x12 -- rc: i/o on ra6/osc2/clkout, rc on ra7/osc1/clkin
INTOSC_CLKOUT = 0x11 -- intosc: clkout on ra6/osc2/clkout, i/o on ra7/osc1/clkin
INTOSC_NOCLKOUT = 0x10 -- intosc: i/o on ra6/osc2/clkout, i/o on ra7/osc1/clkin
EC_NOCLKOUT = 0x3 -- ec
HS = 0x2 -- hs
XT = 0x1 -- xt
LP = 0x0 -- lp
}
pragma fuse_def WDT 0x4 { -- watchdog timer
ENABLED = 0x4 -- on
DISABLED = 0x0 -- off
}
pragma fuse_def PWRTE 0x8 { -- power up timer
DISABLED = 0x8 -- disabled
ENABLED = 0x0 -- enabled
}
pragma fuse_def MCLR 0x20 { -- master clear enable
EXTERNAL = 0x20 -- enabled
INTERNAL = 0x0 -- disabled
}
pragma fuse_def BROWNOUT 0x40 { -- brown out detect
ENABLED = 0x40 -- enabled
DISABLED = 0x0 -- disabled
}
pragma fuse_def LVP 0x80 { -- low voltage program
ENABLED = 0x80 -- enabled
DISABLED = 0x0 -- disabled
}
pragma fuse_def CPD 0x100 { -- data ee read protect
DISABLED = 0x100 -- disabled
ENABLED = 0x0 -- enabled
}
pragma fuse_def CP 0x2000 { -- code protect
DISABLED = 0x2000 -- off
ENABLED = 0x0 -- on
}
- OSC - the clock source configuration
can take 8 different values, 4 of which we may need- INTOSC_NOCLKOUT - internal generator (4M Hz)
- HS - External High Frequency Quartz (8-20 MHz)
- XT = external quartz (200 kHz - 4 MHz)
- LP - external low-frequency quartz (up to 200 kHz)
- WDT - watchdog timer.
The main work of this timer is to restart the microcontroller when it reaches the end.
Whatever the reboot does not occur, it must be promptly reset.
Thus, if a failure occurs, the timer counter will no longer be reset to zero, which will reset the MK. Sometimes it’s convenient, but at the moment we don’t need it. - PWRTE is the next timer.
When activated, it will reset the MK until the power rises to the desired level. - BROWNOUT - MK reset when power drops below normal.
- MCLR - activation of the possibility of external reset MK.
When the function is turned on, the MK will be in constant cut until there is a positive voltage on the MCLR leg (pin 4).
To reset the MK, just install the button that closes pin 4 to the ground. - LVP - activation of the low voltage programming feature.
When activated, one digital input will switch to LVP mode (pin 10). If you apply 5V to this leg, the MK will go into programming mode. For normal operation, MK is required to keep 0V on this leg (connected to the ground).
We will use a programmer that uses increased voltage, because LVP is not required to be activated. - CPD - protection of the EEPROM from reading by the programmer.
- CP - protection of FLASH (firmware) from reading by the programmer.
Change the configuration for yourself:
pragma target clock 4_000_000 -- указываем рабочую частоту, необходимо для некоторых функций расчета времени
-- конфигурация микроконтроллера
pragma target OSC INTOSC_NOCLKOUT -- используем внутренний генератор
pragma target WDT disabled -- сторожевой таймер отключен
pragma target PWRTE disabled -- таймер питания отключен
pragma target MCLR external -- внешний сброс активен
pragma target BROWNOUT disabled -- сбос при падении питания отключен
pragma target LVP disabled -- программирование низким напряжением отключено
pragma target CPD disabled -- защита EEPROM отключена
pragma target CP disabled -- защита кода отключена
Blinking LED at the touch of a button
We modify the program so that the LED blinks only when the button is pressed.
Having solved this problem, we will learn how to work with digital ports in both input mode and output mode.
Digital output
Choose an unused MK leg. Take RB5 (pin 11) for example. This foot does not have additional functions, so we will not need it anywhere else.
In the digital output mode, MK can attract either food or ground to the leg.
You can connect the load to both plus and minus. The difference will only be when and in which direction the current will flow.

In the first case, current will flow from the MC when the unit is set, and in the second, to the MK when the unit is zero.
In order for the LED to light from a logical unit, let us dwell on the first option.
To limit the current through the leg (a maximum of 25 mA to a digital input or 200 mA to all ports), a current-limiting resistor is installed. Using the simplest formula, we calculate the minimum value of 125 Ohms. But since we don’t need a limit, let's take a 500 Ohm resistor (or rather, the closest suitable one).
To connect a more powerful load, you can use transistors in various versions.
Digital input
Take the second unused leg anywhere - RB4 (pin 10, the PGM function specified in the pinout refers to LVP, which we disabled).
In digital input mode, the microcontroller can read two states: the presence or absence of voltage. So we need to connect the button so that in one state a plus goes on the leg, and in the second state - the earth connects to the leg.
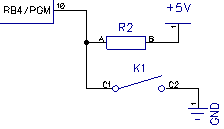
In this embodiment, the resistor is used as a pull-up. Typically, a 10 kΩ resistor is used for bracing.
However, a pull-up resistor is not always necessary. All legs of the PORTB (RB0-RB7) have an internal pull-up, programmable. But the use of external braces is much more reliable.
You can connect not only the button, the main thing to remember is the limitation of current through the MK.
Reset button
Until we forget that we have activated an external reset, we will add a similar button to the MCLR foot (pin 4).
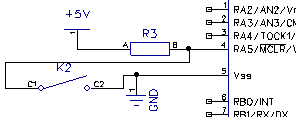
After pressing this button, the MK will start the program from scratch.
Firmware
We assign variables to our LED and button:
enable_digital_io() -- переключение всех входов\выходов на цифровой режим
--
alias led is pin_B5 -- светодиод подключен к RB5
pin_B5_direction = output -- настраиваем RB5 как цифровой выход
--
alias button is pin_B4 -- кнопка подключена к RB4
pin_B4_direction = input -- настраиваем RB4 как вход
led = off -- выключаем светодиод
Now, assigning the led variable the values 1 or 0 (on or off, true or false, other aliases ..) we will pull the MK to the desired foot either plus or minus, thereby lighting and extinguishing the LED, and when reading the button variable we will get 1 if the button is not pressed and 0 if the button is pressed.
Now we will write the necessary actions for us in an infinite loop (these actions will be performed continuously. In the absence of an infinite loop, the MK will freeze):
forever loop
led = off -- выключаем светодиод
_usec_delay(500000) -- ждем 0,5 сек
if Button == 0 then -- если кнопка нажата, выполняем действия
led = on -- зажигаем светодиод
_usec_delay(500000) -- ждем 0,5 сек
end if
end loop
The delay is considered simple: the
frequency of the generator is 4MHz. The operating frequency is 4 times less: 1 MHz. Or 1 clock = 1 μs. 500,000 μs = 0.5 s.
We compile the firmware:
Errors: 0 Warnings: 0
Code: 60/2048 Data: 4/208 Hardware Stack: 0/8 Software Stack: 80
Now we need to write this firmware to MK, assemble the device according to the scheme and verify that everything worked out as it should.
Programmer
All the same scheme:
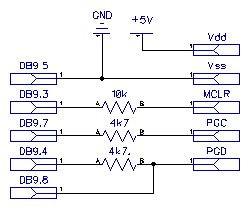
We look at the pinout:
- PGD - pin 13
- PGC - pin 12
- MCLR (Vpp) - pin 4
- Vdd - pin 14
- Vss - pin 5
We solder ...

Poor soldering is one of the main problems of device inoperability.
Do not repeat my bad habits: do not use a hinged installation.
In this case, the tail from the old PS / 2 mouse inserted into the mouse connector was used as 5V power.
We connect to the computer.
Download and run WinPic800 .
Go to Settings-> the Hardware , select JDM and the port number on which hangs the programmer
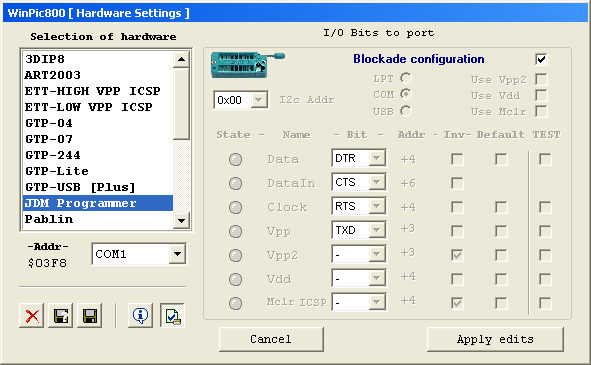
Click the Hardware the Test , then Detect Device
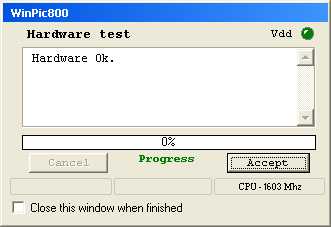
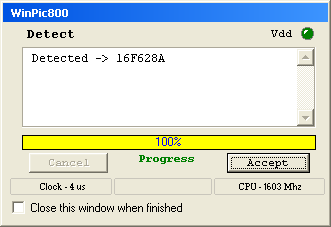
Open our firmware pic628a_test.hex

tab Setting You can check that the configuration bits on display is correct, if you want then you can change

Program All , then Verify All

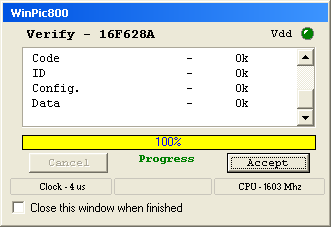
If no errors have occurred, continue to solder.
Result
Final Scheme:
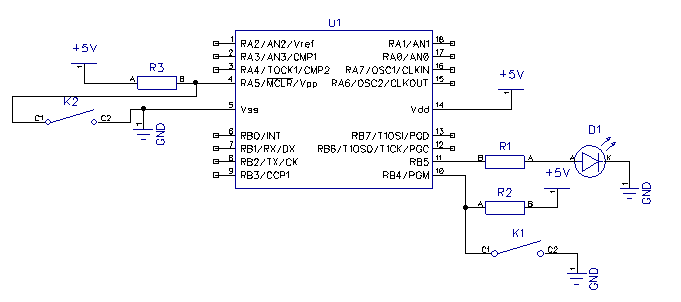
From the programmer, only high voltage (12V) on the MCLR interferes with us. In order not to solder the entire programmer, you can solder only one wire ... Or just do not connect the programmer to the COM port. The remaining wires will not interfere with us (and the connected power and ground will only simplify soldering).
The button on the MCLR can be soldered at will, but a pull-up is required.
When reconnecting the programmer, the resistor will need to be removed, otherwise it will pull 12V to the power.
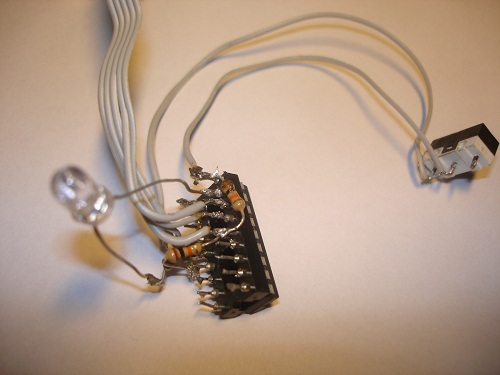
The result of the work can be seen in the video.
So, we have the simplest device on the microcontroller: an LED flasher.
Now we need to learn how to use all the remaining peripherals, but more on that in the next article .