
19 obscure tricks
- Transfer
The note is not new, but I'm sure that now not everyone knows all of the following (Hereinafter, the italics of the translator).
When I look at my own code and on someone else’s code on rails, I often see unrealized opportunities for using certain rail techniques. I would like to list some tips and tricks to improve the efficiency of the application and code, as a reminder for myself and for you.
Measuring speed in controllers is very simple. Use the benchmark method in any model.
Your code, of course, will be better ;-) Regular SQL logs will not be written inside the benchmark method, only the results.
acts_as_nested_set - almost everyone (ho-ho) is very familiar with acts_as_tree, but acts_as_nested_set appeared imperceptibly in the rails . This is similar to acts_as_tree, but with the convenience: you can select all the children (and their heirs) of one node in one go. There is a list of methods .
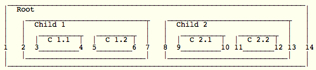
Simple collections through to_proc - tired of writing
Turning arrays into sentences - if you are in the show(view) you need to display a list of names, you take an array of type
Sending a file to a user - usually static files are transferred over a direct URL bypassing the rail application. However, in some situations it may be useful to hide the location of the file, especially if you are sending something of value, like an e-book. It may also be necessary to restrict the sending of files only to logged-in users. Solves the problem
Fetching page elements via RJS - changing an element in RJS is easy, but what if we don’t know which element we need to change, and would like to be addressed through a CSS request? This is possible with the select method. Eg
Existence check - when executing Model.find (id), we will get an exception if the id element was not found. To avoid this, first run Model.exists? (Id) to find out if there is one.
Numeric helpers for common tasks - they are infrequently used, but nonetheless very useful:
Simple routing testing- test_routing - a helper that replaces the default "routes" in routes.rb for experiments. For instance:
More details here .
A lot of interesting things about the request -
Further increase productivity- by default, rails record sessions on the local file system. Many change this to ActiveRecordStore to record sessions in the database. Memcached will be an even faster alternative, but it is not so easy to install (and it will not work if the servers are strangers, etc.). Nevertheless, it can be done faster than ActiveRecordStore through the use of Stefan Kaes SQLSessionStore . The plugin bypasses the disadvantages of ActiveRecordStore through its SQL session saving technique.
Caching static content at startup - if you have data that does not change from restart to restart, cache it. For example, it could be a YAML or XML file in / config-e with application settings, and it can be loaded into a constant in environment.rb, making access faster and easier.
HTML-checking and validity (the X) - is not for everyone, but if your output is valid, then there are chances that your impression (view) rendered correctly. Raymond Scott ( Scott Raymond ) has developed a helper assert_valid_markup , which can be used for functional testing.
More neat testing of HTML output - combine the Hpricot parser from wy ( why ) with a special test extension, and get powerful tests like
Running long processes separately in the background - there is a small framework
Beautiful IDLs in URLs - replace
Allocation functionality chunks in Engin - all have heard about the plug-ins, but sadly few ispolzuyuet Engin ( engines )! Engins are plugins on steroids ( or barbiturates ). They can have their own models, controllers and displays, and they can integrate with any application. This allows you to highlight common pieces of functionality (logins, user management, content management, etc.) into separate “engines” that can be included in different projects in minutes. Say no to writing stupid logins! Engines are cool, but they have to be much cooler.
Calculations - Would you like to calculate the maximum, minimum, average, amount for the data in the table? It is possible with Calcuations of
Outputting data in XML or YAML is not only to create a .rxml Builder-a template for output in XML. There
When I look at my own code and on someone else’s code on rails, I often see unrealized opportunities for using certain rail techniques. I would like to list some tips and tricks to improve the efficiency of the application and code, as a reminder for myself and for you.
Measuring speed in controllers is very simple. Use the benchmark method in any model.
User.benchmark ("adding and deleting 1000 users") do 1000.times do User.create (: name => 'something') x = User.find_by_name ('something') x.destroy end end
Your code, of course, will be better ;-) Regular SQL logs will not be written inside the benchmark method, only the results.
acts_as_nested_set - almost everyone (ho-ho) is very familiar with acts_as_tree, but acts_as_nested_set appeared imperceptibly in the rails . This is similar to acts_as_tree, but with the convenience: you can select all the children (and their heirs) of one node in one go. There is a list of methods .
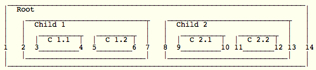
Simple collections through to_proc - tired of writing
post.collect { |p| p.title }
or post.select { |p| p.activated? }.collect{ |p| p.title}
? Using a small hack, translate the characters into procedures. You can write post.collect(&:title)
or post.select(&:activated?).collect(&:title)
! Here about it much more . Turning arrays into sentences - if you are in the show(view) you need to display a list of names, you take an array of type
['Peter', 'Fred', 'Chris']
, connect with commas and add “and” before the last one, which could be fed up. The method is to_sentence
in a hurry to help: it names.to_sentence
will return "Peter, Fred, and Chris"
. (Yes, this is not portable and English-centric. In the notes to the original article there is more about this.) Sending a file to a user - usually static files are transferred over a direct URL bypassing the rail application. However, in some situations it may be useful to hide the location of the file, especially if you are sending something of value, like an e-book. It may also be necessary to restrict the sending of files only to logged-in users. Solves the problem
send_file
. Files are transmitted in 4096 bytes, so even large files will not slow down the system.Fetching page elements via RJS - changing an element in RJS is easy, but what if we don’t know which element we need to change, and would like to be addressed through a CSS request? This is possible with the select method. Eg
page.select('#items li').each { |item| item.hide }
. Powerful thing! Existence check - when executing Model.find (id), we will get an exception if the id element was not found. To avoid this, first run Model.exists? (Id) to find out if there is one.
Numeric helpers for common tasks - they are infrequently used, but nonetheless very useful:
number_to_currency(1234567.948) # => $1,234,567.95
either human_size(1234567890) # => 1.1GB
or number_with_delimiter(999999999) # => 999,999,999
. There are others. (The same note regarding localization.) Simple routing testing- test_routing - a helper that replaces the default "routes" in routes.rb for experiments. For instance:
with_routing do | set | set.draw {set.connect ': controller /: id /: action'} assert_equal ( ['/ content / 10 / show', {}], set.generate (: controller => 'content',: id => 10,: action => 'show') ) end
More details here .
A lot of interesting things about the request -
request.post?
and request.xhr?
- are popular ways to look at POST and ajax requests, but there are others that are not so well known. For example, it request.subdomains
will return an array of subdomains that can be used for authorization, request.request_uri
give the full local URI, request.host
this is the fully qualified host name, request.method
will return the method in lower case, and request.ssl?
determine whether it is SSL. Further increase productivity- by default, rails record sessions on the local file system. Many change this to ActiveRecordStore to record sessions in the database. Memcached will be an even faster alternative, but it is not so easy to install (and it will not work if the servers are strangers, etc.). Nevertheless, it can be done faster than ActiveRecordStore through the use of Stefan Kaes SQLSessionStore . The plugin bypasses the disadvantages of ActiveRecordStore through its SQL session saving technique.
Caching static content at startup - if you have data that does not change from restart to restart, cache it. For example, it could be a YAML or XML file in / config-e with application settings, and it can be loaded into a constant in environment.rb, making access faster and easier.
HTML-checking and validity (the X) - is not for everyone, but if your output is valid, then there are chances that your impression (view) rendered correctly. Raymond Scott ( Scott Raymond ) has developed a helper assert_valid_markup , which can be used for functional testing.
More neat testing of HTML output - combine the Hpricot parser from wy ( why ) with a special test extension, and get powerful tests like
assert_equal "My title", tag('title')
or assert element('body').should_contain('something')
. This is ideal for testing custom templates. Anyway, it's better than that assert_tag
! Running long processes separately in the background - there is a small framework
BackgrounDRb
from Ezra Zigmuntovich (Ezra Zygmuntovich ), which runs as a daemon and accepts tasks from a rail application, and runs them independently. A powerful thing, helps in sending letters, receiving URLs, and other things, which can slow down the execution time of the request of the main application. The demo task increases the variable by 1, after which it does it sleep
for 1 second. Next, we make the rail method, which polls this variable, and we feel the difference. Here are more . Beautiful IDLs in URLs - replace
to_param
in your model and return something like "#{id}-#{title.gsub(/[^a-z0-9]+/i, '-')}"
, for an URL type yoursite.com/posts/show/123-post-title-goes-here...
. It is much more pleasant for the user, and there is no need to change anything in Post.find(params[:id])
, since non-digital characters will be ejected automatically. Full explanation here . (link broken, it seems)Allocation functionality chunks in Engin - all have heard about the plug-ins, but sadly few ispolzuyuet Engin ( engines )! Engins are plugins on steroids ( or barbiturates ). They can have their own models, controllers and displays, and they can integrate with any application. This allows you to highlight common pieces of functionality (logins, user management, content management, etc.) into separate “engines” that can be included in different projects in minutes. Say no to writing stupid logins! Engines are cool, but they have to be much cooler.
Calculations - Would you like to calculate the maximum, minimum, average, amount for the data in the table? It is possible with Calcuations of
ActiveRecord
.Person.average('age'), Person.maximum(:age, :group => 'last_name'), Order.sum('total')
: all of this is now being embodied. Most of this can be configured with additional options, read if you do not already have this in your code. Outputting data in XML or YAML is not only to create a .rxml Builder-a template for output in XML. There
ActiveRecord
is a method to_xml
that outputs the result in XML. It works with simple objects, and with whole tables: User.find(:all).to_xml
. Inkluda also supported: Post.find(:all, :include => [:comments]).to_xml
. Same thing in YAML using to_yaml
.