
C ++ code examples before and after Ranges
- Transfer
Hello again. The translation of the following material was prepared specifically for students of the course "C ++ Developer" , classes on which will start on June 27.
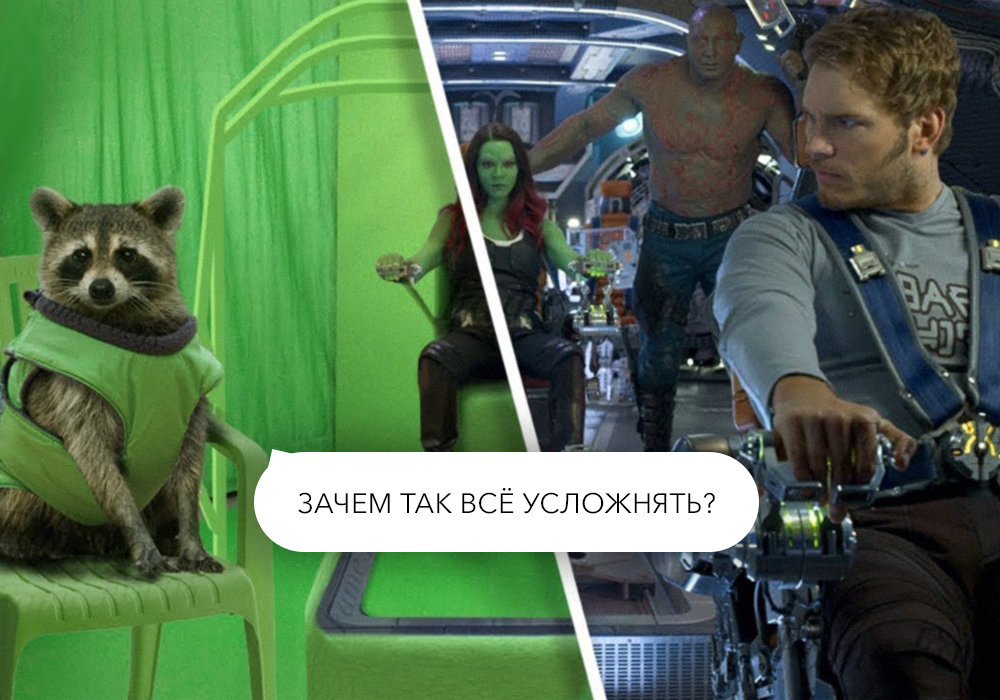
The Ranges library was adopted in C ++ 20 at a meeting of the standard committee in San Diego last November. The library provides components for processing ranges of values designed to simplify our code. Unfortunately, the Ranges library is not very well documented, which makes it harder to understand for those who would like to master it. This post is intended to provide examples of code written using and without Ranges.
Eric Niebler's Ranges library implementation is available here.. It works with Clang 3.6.2 or later, gcc 5.2 or later, and VC ++ 15.9 or later. The code examples below have been written and tested with the latest versions of compilers. It is worth noting that these examples are typical implementations and are not necessarily the only solutions that you can come up with.
Although the standard namespace for the Ranges library is
The following namespace aliases are used in the examples below:
Also, for simplification, we will refer to the following objects, functions and lambdas:
APDATE : I would like to thank Eric Nibler and everyone else who commented below, with suggestions for these code examples. I updated a few based on their reviews.
Print all the elements of the range:
Print all the elements of the range in the reverse order:
Print only the even elements of the range, but in the reverse order:
Skip the first two elements of the range and print only even ones from the following three:
Print numbers from 101 to 200:
Print all Roman numerals from 101 to 200. To convert a number to the corresponding Roman number, use the function
Print the Roman numerals of the last three numbers divisible by 7 in the range [101, 200], in reverse order.
Create a range of strings containing the roman numerals of the last three numbers that are multiples of 7 in the range [101, 200], in reverse order.
Change the unsorted range so that it retains only unique values, but in the reverse order.
Delete the two smallest and the two largest values of the range and leave the rest ordered in the second range.
Combine all rows in a given range into a single value.
Count the number of words (separated by a space) in the text.
Was the article helpful to you? Write in the comments.
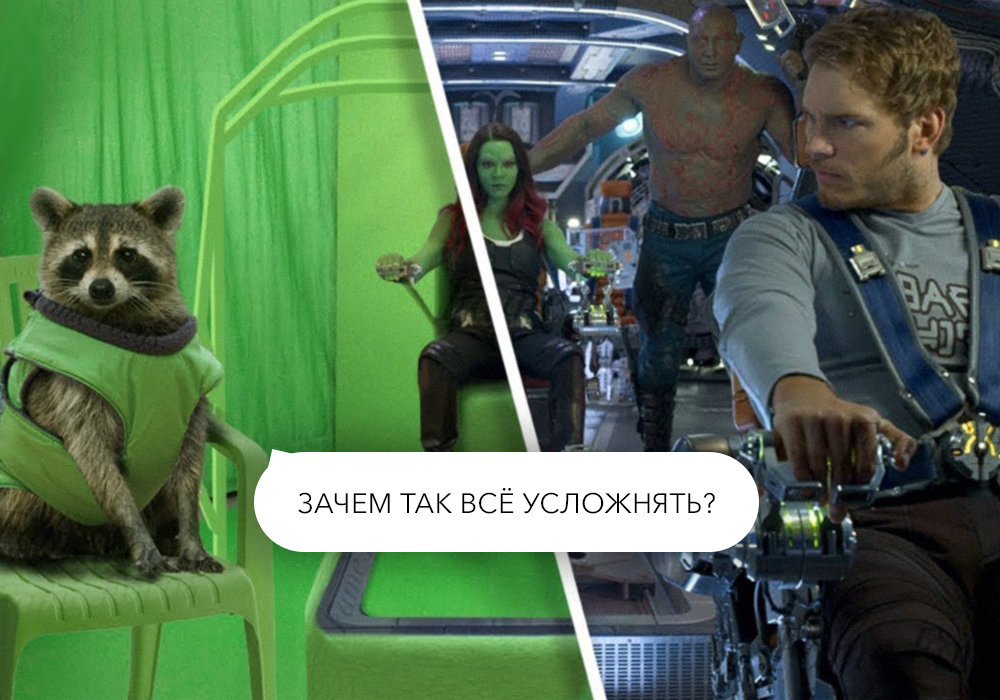
The Ranges library was adopted in C ++ 20 at a meeting of the standard committee in San Diego last November. The library provides components for processing ranges of values designed to simplify our code. Unfortunately, the Ranges library is not very well documented, which makes it harder to understand for those who would like to master it. This post is intended to provide examples of code written using and without Ranges.
Eric Niebler's Ranges library implementation is available here.. It works with Clang 3.6.2 or later, gcc 5.2 or later, and VC ++ 15.9 or later. The code examples below have been written and tested with the latest versions of compilers. It is worth noting that these examples are typical implementations and are not necessarily the only solutions that you can come up with.
Although the standard namespace for the Ranges library is
std::ranges
, in this current library implementation it is ranges::v3
. The following namespace aliases are used in the examples below:
namespace rs = ranges::v3;
namespace rv = ranges::v3::view;
namespace ra = ranges::v3::action;
Also, for simplification, we will refer to the following objects, functions and lambdas:
std::string to_roman(int value)
{
std::vector> roman
{
{ 1000, "M" },{ 900, "CM" },
{ 500, "D" },{ 400, "CD" },
{ 100, "C" },{ 90, "XC" },
{ 50, "L" },{ 40, "XL" },
{ 10, "X" },{ 9, "IX" },
{ 5, "V" },{ 4, "IV" },
{ 1, "I" }
};
std::string result;
for (auto const & [d, r]: roman)
{
while (value >= d)
{
result += r;
value -= d;
}
}
return result;
}
std::vector v{1,1,2,3,5,8,13,21,34};
auto print_elem = [](auto const e) {std::cout << e << '\n'; };
auto is_even = [](auto const i) {return i % 2 == 0; };
APDATE : I would like to thank Eric Nibler and everyone else who commented below, with suggestions for these code examples. I updated a few based on their reviews.
Print all the elements of the range:
To the ranges | After rangs |
---|---|
C ++ | C ++ |
|
|
Print all the elements of the range in the reverse order:
To the ranges | After rangs |
---|---|
C ++ | C ++ |
|
|
Print only the even elements of the range, but in the reverse order:
To the ranges | After rangs |
---|---|
C ++ | C ++ |
|
|
Skip the first two elements of the range and print only even ones from the following three:
To the ranges | After rangs |
---|---|
C ++ | C ++ |
|
|
Print numbers from 101 to 200:
To the ranges | After rangs |
---|---|
C ++ | C ++ |
|
|
Print all Roman numerals from 101 to 200. To convert a number to the corresponding Roman number, use the function
to_roman()
shown above.To the ranges | After rangs |
---|---|
C ++ | C ++ |
|
|
Print the Roman numerals of the last three numbers divisible by 7 in the range [101, 200], in reverse order.
To the ranges | After rangs |
---|---|
C ++ | C ++ |
|
|
Create a range of strings containing the roman numerals of the last three numbers that are multiples of 7 in the range [101, 200], in reverse order.
To the ranges | After rangs |
---|---|
C ++ | C ++ |
|
|
Change the unsorted range so that it retains only unique values, but in the reverse order.
To the ranges | After rangs |
---|---|
C ++ | C ++ |
|
|
Delete the two smallest and the two largest values of the range and leave the rest ordered in the second range.
To the ranges | After rangs |
---|---|
C ++ | C ++ |
|
|
Combine all rows in a given range into a single value.
To the ranges | After rangs |
---|---|
C ++ | C ++ |
|
|
Count the number of words (separated by a space) in the text.
To the ranges | After rangs |
---|---|
C ++ | C ++ |
|
|
Was the article helpful to you? Write in the comments.