Simple Telegram bot in Python in 30 minutes
On Habré, and not only that, so much has been said about bots that it’s even too much. But having become interested in this topic a couple of weeks ago, I still could not find normal material: all the articles were either for dummies at all and were limited to sending a message in response to a user message, or were irrelevant. This prompted me to write an article that would explain to a novice like me how to write and launch a more or less meaningful bot (with the possibility of expanding functionality).
The simplest and most described part. Very briefly: you need to find the @BotFather bot , write him / start , or / newbot , fill in the fields that he asks (the name of the bot and its short name), and get a message with the bot token and a link to the documentation. The token must be saved, preferably reliable, since this is the only key for authorizing the bot and interacting with it.
As already mentioned in the title, we will write the bot in Python. This article will describe how to work with the PyTelegramBotAPI (Telebot) library. If you do not have Python installed, then first you need to do this: in the Linux terminal, you need to enter
If you use Windows, then you need to download Python from the official site.
After, in the Linux terminal, or the Windows command prompt, enter
Now you are ready to write the code.
A small digression. A telegram can tell the bot about user actions in two ways: through a response to a server request (Long Poll), and through Webhook, when the Telegram server itself sends a message that someone wrote to the bot. The second method clearly looks better, but requires a dedicated IP address, and SSL is installed on the server. In this article I want to talk about writing a bot, not setting up a server, so we will use Long Poll.
Open your favorite text editor, and let's write the bot code!
The first thing to do is import our library and connect the bot token:
Now declare a method for receiving text messages:
In this section of code, we declared a listener for text messages and a method for processing them. The content_types field can take different values, and not only one, for example
Will respond to text messages, documents and audio. You can read in more detail in the official documentation.
Now let's add a little functionality to our method: if the user writes “Hello” to us, then we will say “Hello, how can I help you?”, And if they write us the “/ help” command, we will tell the user to write "Hi":
This piece of code does not require comments, as it seems to me. Now we need to add only one line to our code (outside of all methods).
Now our bot will constantly ask the Telegram server “Did someone write to me?” And if we write to our bot, the Telegram will send it our message. We save the whole file, and write in the console
Where bot.py is the name of our file.
Now you can write a bot and look at the result:

Sending messages is undoubtedly fun, but it’s even more fun to have a dialogue with the user: ask him questions and get answers to them. Suppose now our bot will ask the user in turn his name, surname and age. For this we will use the bot's register_next_step_handler method:
And so, we recorded the user data. This example shows a very simplified example, for good, you need to store intermediate data and user states in the database, but today we are working with a bot and not with databases. The final touch - we ask users to confirm that everything is entered correctly, but not just like that, but with buttons! To do this, edit the get_age method code a little
And now our bot sends the keyboard, but if you click on it, then nothing will happen. Because we did not write a handler method. Let's write:
It remains only to append one line to the beginning of the file:
That's all, save and run our bot:
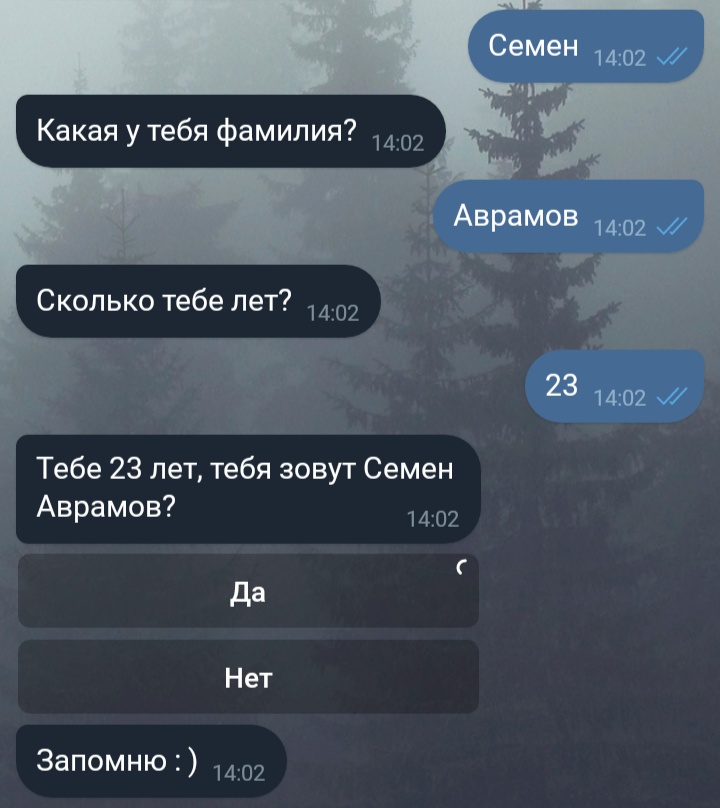
Part 1: Register a bot
The simplest and most described part. Very briefly: you need to find the @BotFather bot , write him / start , or / newbot , fill in the fields that he asks (the name of the bot and its short name), and get a message with the bot token and a link to the documentation. The token must be saved, preferably reliable, since this is the only key for authorizing the bot and interacting with it.
Part 2: Preparing to Write Code
As already mentioned in the title, we will write the bot in Python. This article will describe how to work with the PyTelegramBotAPI (Telebot) library. If you do not have Python installed, then first you need to do this: in the Linux terminal, you need to enter
sudo apt-get install python python-pip
If you use Windows, then you need to download Python from the official site.
After, in the Linux terminal, or the Windows command prompt, enter
pip install pytelegrambotapi
Now you are ready to write the code.
Part 3: Receive messages and say “Hello”
A small digression. A telegram can tell the bot about user actions in two ways: through a response to a server request (Long Poll), and through Webhook, when the Telegram server itself sends a message that someone wrote to the bot. The second method clearly looks better, but requires a dedicated IP address, and SSL is installed on the server. In this article I want to talk about writing a bot, not setting up a server, so we will use Long Poll.
Open your favorite text editor, and let's write the bot code!
The first thing to do is import our library and connect the bot token:
import telebot;
bot = telebot.TeleBot('%ваш токен%');
Now declare a method for receiving text messages:
@bot.message_handler(content_types=['text'])
def get_text_messages(message):
In this section of code, we declared a listener for text messages and a method for processing them. The content_types field can take different values, and not only one, for example
@bot.message_handler(content_types=['text', 'document', 'audio'])
Will respond to text messages, documents and audio. You can read in more detail in the official documentation.
Now let's add a little functionality to our method: if the user writes “Hello” to us, then we will say “Hello, how can I help you?”, And if they write us the “/ help” command, we will tell the user to write "Hi":
if message.text == "Привет":
bot.send_message(message.from_user.id, "Привет, чем я могу тебе помочь?")
elif message.text == "/help":
bot.send_message(message.from_user.id, "Напиши привет")
else:
bot.send_message(message.from_user.id, "Я тебя не понимаю. Напиши /help.")
This piece of code does not require comments, as it seems to me. Now we need to add only one line to our code (outside of all methods).
bot.polling(none_stop=True, interval=0)
Now our bot will constantly ask the Telegram server “Did someone write to me?” And if we write to our bot, the Telegram will send it our message. We save the whole file, and write in the console
python bot.py
Where bot.py is the name of our file.
Now you can write a bot and look at the result:

Part 4: Buttons and message threads
Sending messages is undoubtedly fun, but it’s even more fun to have a dialogue with the user: ask him questions and get answers to them. Suppose now our bot will ask the user in turn his name, surname and age. For this we will use the bot's register_next_step_handler method:
name = '';
surname = '';
age = 0;
@bot.message_handler(content_types=['text'])
def start(message):
if message.text == '/reg':
bot.send_message(message.from_user.id, "Как тебя зовут?");
bot.register_next_step_handler(message, get_name); #следующий шаг – функция get_name
else:
bot.send_message(message.from_user.id, 'Напиши /reg');
def get_name(message): #получаем фамилию
global name;
name = message.text;
bot.send_message(message.from_user.id, 'Какая у тебя фамилия?');
bot.register_next_step_handler(message, get_surnme);
def get_surname(message):
global surname;
surname = message.text;
bot.send_message('Сколько тебе лет?');
bot.register_next_step_handler(message, get_age);
def get_age(message):
global age;
while age == 0: #проверяем что возраст изменился
try:
age = int(message.text) #проверяем, что возраст введен корректно
except Exception:
bot.send_message(message.from_user.id, 'Цифрами, пожалуйста');
bot.send_message(message.from_user.id, 'Тебе '+str(age)+' лет, тебя зовут '+name+' '+surname+'?')
And so, we recorded the user data. This example shows a very simplified example, for good, you need to store intermediate data and user states in the database, but today we are working with a bot and not with databases. The final touch - we ask users to confirm that everything is entered correctly, but not just like that, but with buttons! To do this, edit the get_age method code a little
def get_age(message):
global age;
while age == 0: #проверяем что возраст изменился
try:
age = int(message.text) #проверяем, что возраст введен корректно
except Exception:
bot.send_message(message.from_user.id, 'Цифрами, пожалуйста');
keyboard = types.InlineKeyboardMarkup(); #наша клавиатура
key_yes = types.InlineKeyboardButton(text='Да', callback_data='yes'); #кнопка «Да»
keyboard.add(key_yes); #добавляем кнопку в клавиатуру
key_no= types.InlineKeyboardButton(text='Нет', callback_data='no');
keyboard.add(key_no);
question = 'Тебе '+str(age)+' лет, тебя зовут '+name+' '+surname+'?';
bot.send_message(message.from_user.id, text=question, reply_markup=keyboard)
And now our bot sends the keyboard, but if you click on it, then nothing will happen. Because we did not write a handler method. Let's write:
@bot.callback_query_handler(func=lambda call: True)
def callback_worker(call):
if call.data == "yes": #call.data это callback_data, которую мы указали при объявлении кнопки
.... #код сохранения данных, или их обработки
bot.send_message(call.message.chat.id, 'Запомню : )');
elif call.data == "no":
... #переспрашиваем
It remains only to append one line to the beginning of the file:
from telebot import types
That's all, save and run our bot:
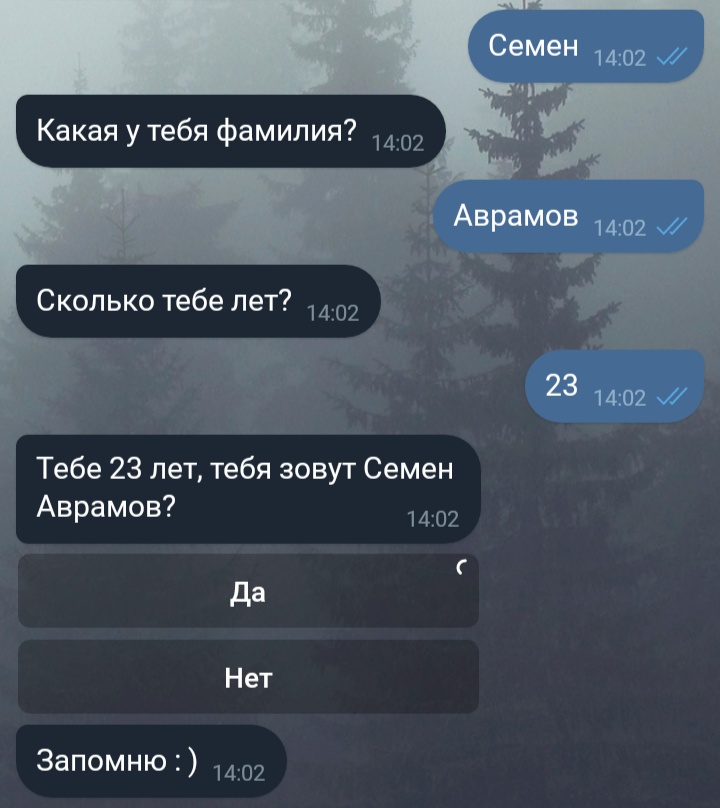