Office 365. An example of working with the Microsoft Graph API in Angular5 using ADAL JS. ADAL JS vs MSAL JS
- Tutorial
The last time we discussed the authorization mechanism to use with Office 365 API (such as with Microsoft Graph API):
Then we made a simple static HTML page on which our vanilla JavaScript made a request to the Microsoft Graph API and displayed a list of letters from Office 365. In this note, we will develop an example and do the same on Angular5.

We’ll also talk about the differences between Work (or school account) and personal (Personal Account) accounts when using the Office 365 API. GitHub
example . We will assume that you are familiar with the previous article , understand the process of obtaining and using token, have already registered the application in Azure, received the Application ID.
Microsoft has two types of accounts:
- Work or school Account
- A Personal Account A work
or school account is created (and deleted) by the administrator of the company that purchased the Office 365 subscription. Employees use it, for example, to connect to corporate mail, SharePoint, OneDrive.
You create a Personal Account yourself; it is used to connect to personal services, for example, personal OneDrive.
We already know that token for API calls produces “Azure AD Authorization Endpoint”. There are 2 authorization points:
Why then do we need an “Authorization Endpoint”? The peculiarity is that “Authorization Endpoint v2.0” will issue a token only for those API features that are available for both Work and Personal accounts. Those. using Endpoint v2.0 and Work account you will be able to access mail, but there will be no access to the SharePoint API (for more information about which APIs Endpoint v2.0 supports see the links at the end).
Currently v2.0 endpoint does not work with applications that are registered in the corporate Azure portal (we did this registration in the last post), you need to use a separate Microsoft Application Registration Portal .
Microsoft promises to expand the capabilities of v2.0 endpoint in the future, and provide support for applications registered in the corporate Azure portal - v2.0 endpoint will be able to issue tokens for them. In the meantime, for enterprise applications, Microsoft recommends using the “Authorization Endpoint”:
We need installed Node.js, npm, and the Angular CLI , a utility that helps you create and build Angular projects. The whole process is well described in Angular QuickStart .
Let's make a new project:
There are no official (from Microsoft) TypeScript d.ts descriptions for Adal.js. Several use cases for Adal.js in Angular 5:
Option 5 looks the simplest and most convenient: you use the original Adal.js from Microsoft and use the d.ts description to add intellisense and build checks.
We establish proven d.ts description for Adal.js . Being in the project folder:
We have a test example, so we will make it as simple as possible: we will connect to the Microsoft Graph API directly in the AppComponent.
In the file app.component.ts we import HttpClient and adal
In app.module.ts we will connect HttpClientModule
Connect the js library to the page.
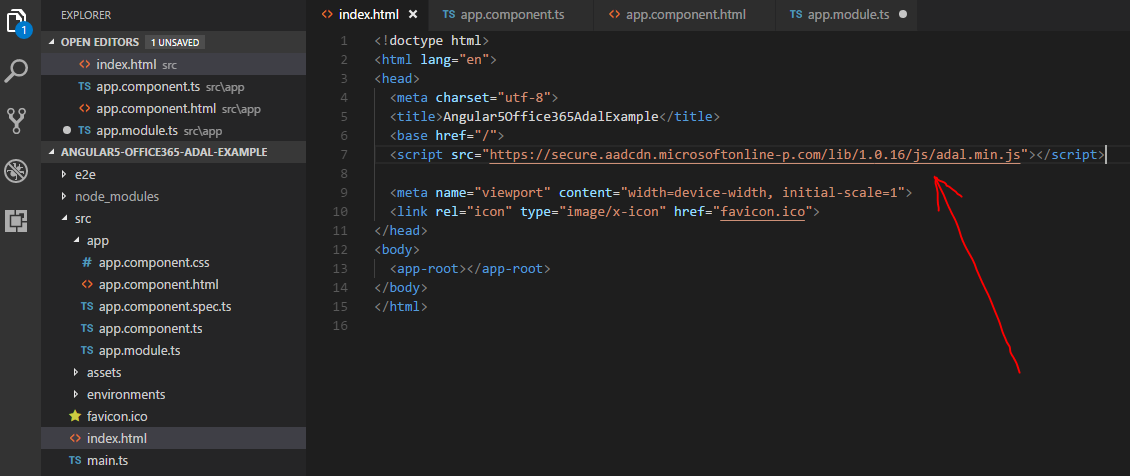
Next will be exactly the same code as in the previous example on JS.
In ngOnInit we will check whether the user is authorized or not, if not authorized or token is rotten, we will send the user to the registration page.
Now you can use token to call the Microsoft Graph API
Full example on github .
Active Directory Authentication Library (ADAL) for JavaScript
Microsoft Authentication Library Preview for JavaScript (MSAL.js)
Should I use the v2.0 endpoint?
What's different about the v2.0 endpoint?
Understanding Microsoft Work And Personal Accounts
- every API call needs to pass token. Token has a limited lifespan
- token issues a Microsoft service, the so-called “Azure AD Authorization Endpoint”
- You can get token without the server side using only JavaScript in the browser. For this, Microsoft made the ADAL JS JavaScript library , which simplifies communication with the “Azure AD Authorization Endpoint” to obtain a token.
Then we made a simple static HTML page on which our vanilla JavaScript made a request to the Microsoft Graph API and displayed a list of letters from Office 365. In this note, we will develop an example and do the same on Angular5.

We’ll also talk about the differences between Work (or school account) and personal (Personal Account) accounts when using the Office 365 API. GitHub
example . We will assume that you are familiar with the previous article , understand the process of obtaining and using token, have already registered the application in Azure, received the Application ID.
ADAL JS and MSAL JS
Microsoft has two types of accounts:
- Work or school Account
- A Personal Account A work
or school account is created (and deleted) by the administrator of the company that purchased the Office 365 subscription. Employees use it, for example, to connect to corporate mail, SharePoint, OneDrive.
You create a Personal Account yourself; it is used to connect to personal services, for example, personal OneDrive.
More details about Work and Personal accounts can be found here “ Understanding Microsoft Work And Personal Accounts ”.Office 365 API (for example Microsoft Graph API) has different support for personal and work accounts. For example, both Work account and Personal account can work with mail, but there is no personal SharePoint (and, therefore, the SharePoint API for Personal account).
We already know that token for API calls produces “Azure AD Authorization Endpoint”. There are 2 authorization points:
- Authorization Endpoint
Issues tokens only for Work Accounts.
When login, the user is sent to
https: //login.microsoftonline.com/common/oauth2/authorize?client_id = ... - Authorization Endpoint v2.0
Issues tokens for both Work and Personal
Accounts. When logging in, the user goes to
https: //login.microsoftonline.com/common/oauth2/v2.0/authorize?
client_id = ...
Why then do we need an “Authorization Endpoint”? The peculiarity is that “Authorization Endpoint v2.0” will issue a token only for those API features that are available for both Work and Personal accounts. Those. using Endpoint v2.0 and Work account you will be able to access mail, but there will be no access to the SharePoint API (for more information about which APIs Endpoint v2.0 supports see the links at the end).
Currently v2.0 endpoint does not work with applications that are registered in the corporate Azure portal (we did this registration in the last post), you need to use a separate Microsoft Application Registration Portal .
Microsoft promises to expand the capabilities of v2.0 endpoint in the future, and provide support for applications registered in the corporate Azure portal - v2.0 endpoint will be able to issue tokens for them. In the meantime, for enterprise applications, Microsoft recommends using the “Authorization Endpoint”:
If your application only needs to support Microsoft work and school accounts, don't use the v2.0 endpointADAL JS works with “Authorization Endpoint”, MSAL JS with v2.0 endpoint. In this article we will use the Authorization Endpoint of the first version and, accordingly, ADAL JS.
Angular 5 and ADAL JS
We need installed Node.js, npm, and the Angular CLI , a utility that helps you create and build Angular projects. The whole process is well described in Angular QuickStart .
Let's make a new project:
ng new angular5-office365-adal-example
There are no official (from Microsoft) TypeScript d.ts descriptions for Adal.js. Several use cases for Adal.js in Angular 5:
- Do not use d.ts descriptions, declare an AuthenticationContext object (from Adal.js) as global any, and write untyped code as in normal JavaScript
- Do it yourself d.ts description
- Take advantage of the adal-angular5 project , which is a tracing-paper of the original adal-angular.js for Microsoft's AngularJS ( Adal js )
- There are projects in which Adal js is completely rewritten in TypeScript ( adal-ts , adal-typescript )
- Use the ready d.ts description.
Option 5 looks the simplest and most convenient: you use the original Adal.js from Microsoft and use the d.ts description to add intellisense and build checks.
We establish proven d.ts description for Adal.js . Being in the project folder:
npm install --save @types/adal
We have a test example, so we will make it as simple as possible: we will connect to the Microsoft Graph API directly in the AppComponent.
In the file app.component.ts we import HttpClient and adal
import { HttpClient, HttpHeaders } from '@angular/common/http';
import { } from 'adal'; // без этого приложение будет собираться, но будут сообщения об ошибках
In app.module.ts we will connect HttpClientModule
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { HttpClientModule } from '@angular/common/http'; //<- ADD
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule //<- ADD
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Connect the js library to the page.
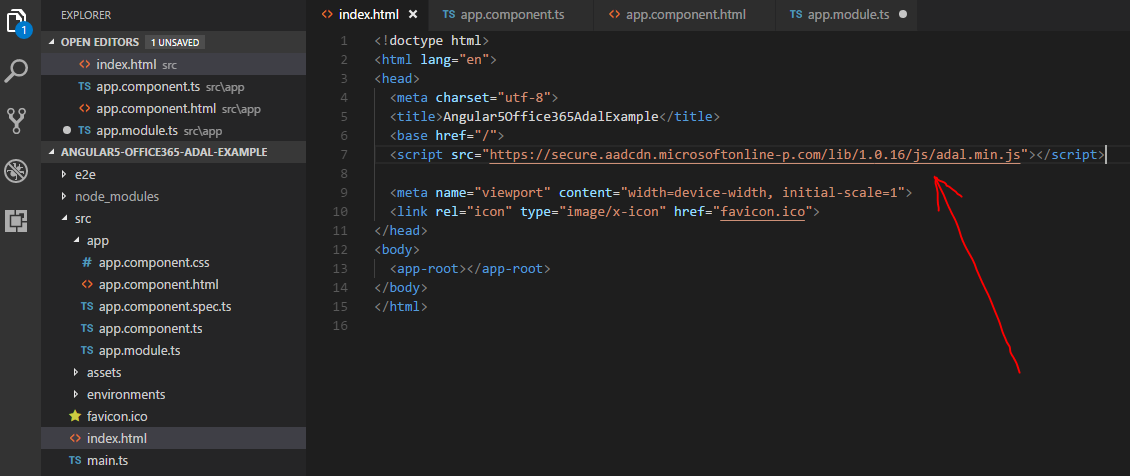
Next will be exactly the same code as in the previous example on JS.
In ngOnInit we will check whether the user is authorized or not, if not authorized or token is rotten, we will send the user to the registration page.
let config: adal.Config = {
tenant: 'igtit.onmicrosoft.com',
clientId: '21XXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX',
postLogoutRedirectUri: window.location.origin,
endpoints: {
graphApiUri: "https://graph.microsoft.com",
},
cacheLocation: "localStorage",
redirectUri: ''
};
let authContext = new AuthenticationContext(config);
let isCallback = authContext.isCallback(window.location.hash);
authContext.handleWindowCallback();
if (isCallback && !authContext.getLoginError()) {
window.location.href = (authContext)._getItem((authContext).CONSTANTS.STORAGE.LOGIN_REQUEST);
}
// check if user need to login
let user = authContext.getCachedUser();
let token = authContext.getCachedToken(config.clientId);
if (!user || !token) {
authContext.login();
}
Now you can use token to call the Microsoft Graph API
authContext.acquireToken(config.endpoints.graphApiUri, (error, token) => {
// call Microsoft Graph API with token
let url = config.endpoints.graphApiUri + "/v1.0/me/messages";
this.http.get(url, {
headers: {
"Authorization": "Bearer " + token
}
}).subscribe(mgs => this.messages = (mgs).value);
});
Full example on github .
References
Active Directory Authentication Library (ADAL) for JavaScript
Microsoft Authentication Library Preview for JavaScript (MSAL.js)
Should I use the v2.0 endpoint?
What's different about the v2.0 endpoint?
Understanding Microsoft Work And Personal Accounts