
We are writing a Twitter bot that predicts the Bitcoin exchange rate
- Transfer
The programmer Ogien Gatalo, the author of the article, the translation that we publish today, has been interested in cryptocurrencies for some time. He is especially interested in forecasting the course for the next few days. He says that he tried some algorithms, but in the end he got the feeling that none of the approaches allows us to give short-term forecasts with confidence. Then, concentrating on the market leader - Bitcoin, he decided to work on his own method of predicting rates. The algorithm he proposes, as well as its implementation as a twitter bot, of course, with some changes, are also suitable for working with other digital currencies.
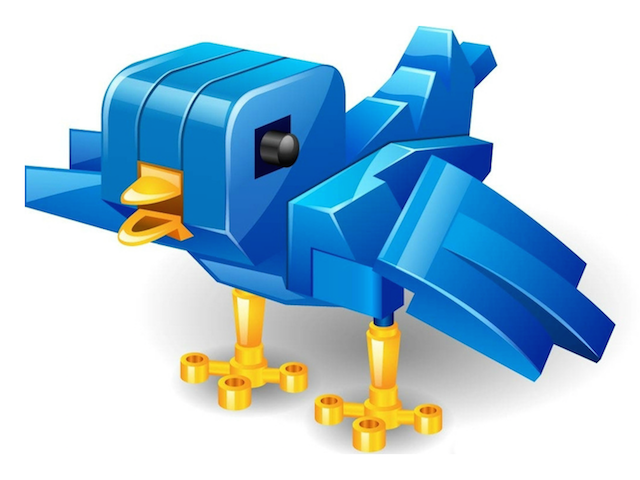
Twitter seems to me a convenient platform for cryptocurrency projects like mine. Here you can, in particular, organize convenient interaction between users and programs for which their own accounts are open. That is why I decided to design my development in the form of a bot for Twitter. In particular, my bot had to, every 2 hours, tweet a forecast of the value of Bitcoin for the next N days. N is the period in days, the forecast for which is most interesting for people who have requested the bot with relevant information. So, for example, if 3 people made a request for a 5-day forecast, and 7 people are interested in a forecast for 2 days, the bot will tweet a forecast for 2 days.
The bot is implemented on Node.js, I developed it pretty quickly. It took some time to search for sources of historical and current data on prices and to select ways to work with them. The main issue was the algorithm. In the end, I decided that they would use the K method of the nearest neighbors in combination with some more things.
The considered method of price forecasting includes the following sequence of actions:
Consider the main steps of this process in more detail.
In this step, using the module
When the bot, once every 2 hours, tweets the forecast, the number of days that was used in this forecast is stored in the black list represented by the array. This array contains the duration of the forecasts for the last 4 tweets. This approach avoids the frequent appearance of similar tweets and the issuance of the same forecasts that have already been tweeted in the last 8 hours.
The feature presented here is very simple. She selects the most frequently requested forecast durations from an array of numbers. If the found number is already in
You can find out the current value of bitcoin using the blockchain.info API. The resulting value is stored in a variable.
This function starts 2 minutes after the start of the algorithm.
Here I will not provide a description of all the functions used at this step, such as executing a request to the Coindesk API to load the data necessary for indicators
Since we calculated the differences between all bitcoin prices in the last 2 months and its current value, we need to find the dates at which these values are closest to 0. Therefore, we first call
At this stage, you can find the first 10 dates in which the price of Bitcoin was closest to its current price.
At this step, we get an array of objects, each of which contains the properties
Here we go through all the elements from
Now it remains only to form a forecast. This is done using the following function.
That's all, the forecast is formed, it remains only to tweet it, appropriately issued.
If you want to get to know my bot better, I suggest you look into the aforementioned repository , which stores the full project code. In addition, I would like to note that this material describes my approach to predicting the price of bitcoin for the next few days, it is still in development, so if you have anything to say about the proposed algorithm, let me know . The forecasts made by the bot are not always accurate, but I noticed that in most cases the predicted value differs from the real one only by $ 100-200. So, apparently, we can say that the bot is usually not so wrong, especially given the way cryptocurrency rates behave insanely.
The main problem of the described algorithm is that it analyzes only historical data on bitcoin and makes predictions based on them. There is no mechanism for prediction, for example, sharp price drops. I am working to take into account the “human factor” in the forecast. It is planned to realize this by collecting, for example, articles from websites, having analyzed which you can find a hint of the possibility of sudden changes in the rate and add this data to the equation.
By the way, all this time I talked about the bot, but never presented it to you. A special account is open for him, coin_instinct . You can tweet him a forecast request.
Dear readers!Are you developing Twitter bots or forecasting cryptocurrency rates? If so, please share your experience.
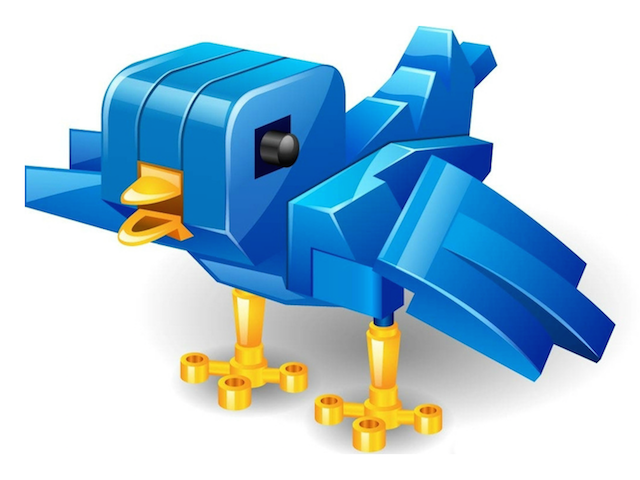
General overview of the algorithm and its implementation
Twitter seems to me a convenient platform for cryptocurrency projects like mine. Here you can, in particular, organize convenient interaction between users and programs for which their own accounts are open. That is why I decided to design my development in the form of a bot for Twitter. In particular, my bot had to, every 2 hours, tweet a forecast of the value of Bitcoin for the next N days. N is the period in days, the forecast for which is most interesting for people who have requested the bot with relevant information. So, for example, if 3 people made a request for a 5-day forecast, and 7 people are interested in a forecast for 2 days, the bot will tweet a forecast for 2 days.
The bot is implemented on Node.js, I developed it pretty quickly. It took some time to search for sources of historical and current data on prices and to select ways to work with them. The main issue was the algorithm. In the end, I decided that they would use the K method of the nearest neighbors in combination with some more things.
The considered method of price forecasting includes the following sequence of actions:
- Collection of user requests for the next forecast.
- Finding the duration of the forecast that is most often requested.
- Getting the current value of bitcoin.
- Finding the
K(10)
closest dates in the last 2 months in which the price of Bitcoin was most similar to its current price. - For the price for each of the found dates (
PAST_DATE
), the BTC price is foundN
days after it (N_DAYS_AFTER_PAST_DATE
). - For each date, the calculation of the difference between the price
N_DAYS_AFTER_PAST_DATE
andPAST_DATE
. - The sum of all the differences found and the division of what happened by
K
. - Getting the result in the video of the average change in the bitcoin exchange rate between all groups of
PAST_DATE
and valuesN_DAYS_AFTER_PAST_DATE
. This result is used when generating a tweet.
Consider the main steps of this process in more detail.
Step 1. Collect user requests
In this step, using the module
twit
and API Twitter, searches for tweets that contain the following structure: . Then, the numbers representing the number of days for which users want to get a forecast are extracted from the tweets found, and an array of these numbers is created.@coin_instinct Predict for days
Step 2. Finding the forecast duration that interests most users
When the bot, once every 2 hours, tweets the forecast, the number of days that was used in this forecast is stored in the black list represented by the array. This array contains the duration of the forecasts for the last 4 tweets. This approach avoids the frequent appearance of similar tweets and the issuance of the same forecasts that have already been tweeted in the last 8 hours.
async function findMostFrequent(array, blackListArr)
{
if(array.length == 0)
return null;
var modeMap = {};
var maxEl = array[0], maxCount = 1;
// Сначала пройдёмся по массиву blackList и запишем в maxEl первый элемент, отсутствующий в чёрном списке. Если все числа в массиве в чёрном списке присутствуют, вернём generateRandom
var containsValidNumbers = false;
for(var i = 0; i < array.length; i++) {
if(!blackListArr.includes(array[i])) {
maxEl = array[i];
containsValidNumbers = true;
break;
}
}
if(!containsValidNumbers) return await generateRandom(blackListArr);
for(var i = 0; i < array.length; i++)
{
var el = array[i];
if(blackListArr.includes(el)) continue;
if(modeMap[el] == null)
modeMap[el] = 1;
else
modeMap[el]++;
if(modeMap[el] > maxCount)
{
maxEl = el;
maxCount = modeMap[el];
}
}
await addToBlackList(maxEl);
return maxEl;
}
The feature presented here is very simple. She selects the most frequently requested forecast durations from an array of numbers. If the found number is already in
blackListArr
, the function returns the second most requested forecast, and so on. If all the requested forecast durations are already in the black list, then the bot will give a prediction for a randomly selected period.Step 3. Getting the current value of Bitcoin
You can find out the current value of bitcoin using the blockchain.info API. The resulting value is stored in a variable.
function refreshBitcoinPrices() {
const request = async () => {
var results = await fetch("https://blockchain.info/ticker");
results = await results.json();
bitcoinData.results = results;
if(bitcoinData.results) {console.log('Blockchain API works!');}
else console.log('Blockchain API is down at the moment.');
// Узнаем сегодняшнюю дату
this.todayDate = new Date();
this.todayDate = this.todayDate.toISOString().split('T')[0];
console.log(this.todayDate);
console.log('New prices fetched.');
console.log('Most recent bitcoin price: '+bitcoinData.results.USD.last);
console.log('Time: '+new Date().getHours()+':'+new Date().getMinutes());
}
request();
// Включено в рабочем боте
setInterval(() => {
request();
},COIN_FETCH_TIMEOUT); // 1000*60*118
}
This function starts 2 minutes after the start of the algorithm.
Step 4. Search for K nearest neighbors
Here I will not provide a description of all the functions used at this step, such as executing a request to the Coindesk API to load the data necessary for indicators
PAST_DATE
and N_DAYS_AFTER_PAST_DATE
. Shown here is a search for nearby neighbors based on how similar they are to the current price. The full project code can be found in my GitHub repository .async function getNearestNeighbours(similarities) {
// Пройдёмся по массиву и найдём k(10), которые ближе всего к 0
var absSimilarities = [];
similarities.forEach( (similarity) => {
absSimilarities.push({
date: similarity.date,
similarityScore: Math.abs(similarity.similarityScore)
})
})
absSimilarities = absSimilarities.sort(function(a,b) {
return (a.similarityScore > b.similarityScore) ? 1 : ((b.similarityScore > a.similarityScore) ? -1 : 0);
});
var kNearest = [];
for(var i = 0; i < K; i++) {
kNearest.push(absSimilarities[i].date);
}
return kNearest;
}
Since we calculated the differences between all bitcoin prices in the last 2 months and its current value, we need to find the dates at which these values are closest to 0. Therefore, we first call
Math.abs
for all the properties of the similatityScore
objects that are in the array, and then sort the array in descending order based on these properties. At this stage, you can find the first 10 dates in which the price of Bitcoin was closest to its current price.
Step 5. Formation of an array of results
At this step, we get an array of objects, each of which contains the properties
start
and end
. The property start
represents the bitcoin price for one of the previous dates, the property is end
used to store the price N
days after this day. Based on these data, we can form a forecast, conclude that the price will rise or fall.async function getFinalResults(kNearest,nDays) {
var finalResults = [];
var finalResult = {};
await forEach(kNearest, async(date) => {
var dateTime = new Date(date);
var pastDate = dateTime.toISOString().split('T')[0];
var futureDate = new Date(date);
futureDate.setDate(futureDate.getDate() + nDays);
futureDate = futureDate.toISOString().split('T')[0];
var valueForThatDay = this.coinDeskApiResults.bpi[pastDate];
var valueForFutureDay = this.coinDeskApiResults.bpi[futureDate];
finalResult = {
start: valueForThatDay,
end: valueForFutureDay
}
finalResults.push(finalResult);
})
return finalResults;
}
Here we go through all the elements from
kNearest
and get data for specific dates, after which we save the results in an array finalResults
and return it.Step 6. Prediction
Now it remains only to form a forecast. This is done using the following function.
/**
* Вычисление прогнозных данных
* Возвращает объект, содержащий данные для прогноза
* @param {*Array} data Массив объектов, содержащий цены биткойна в свойствах start и end
* @param {*Float} currentBitcoinValue Текущая цена биткойна
*/
async function calculatePrediction(data,currentBitcoinValue) {
var finalPredictionData = {
raw: 0,
percentage: 0,
positive: '',
finalValue: 0
}
var sum = 0;
await forEach(data, async (value) => {
sum += value.end - value.start;
})
sum = sum / K;
finalPredictionData.raw = sum;
finalPredictionData.finalValue = currentBitcoinValue + sum;
finalPredictionData.positive = sum > 0 ? 'true' : 'false';
finalPredictionData.percentage = ((finalPredictionData.finalValue - currentBitcoinValue) / currentBitcoinValue) * 100;
return finalPredictionData;
}
That's all, the forecast is formed, it remains only to tweet it, appropriately issued.
Summary
If you want to get to know my bot better, I suggest you look into the aforementioned repository , which stores the full project code. In addition, I would like to note that this material describes my approach to predicting the price of bitcoin for the next few days, it is still in development, so if you have anything to say about the proposed algorithm, let me know . The forecasts made by the bot are not always accurate, but I noticed that in most cases the predicted value differs from the real one only by $ 100-200. So, apparently, we can say that the bot is usually not so wrong, especially given the way cryptocurrency rates behave insanely.
The main problem of the described algorithm is that it analyzes only historical data on bitcoin and makes predictions based on them. There is no mechanism for prediction, for example, sharp price drops. I am working to take into account the “human factor” in the forecast. It is planned to realize this by collecting, for example, articles from websites, having analyzed which you can find a hint of the possibility of sudden changes in the rate and add this data to the equation.
By the way, all this time I talked about the bot, but never presented it to you. A special account is open for him, coin_instinct . You can tweet him a forecast request.
Dear readers!Are you developing Twitter bots or forecasting cryptocurrency rates? If so, please share your experience.