
Internet of Things: Arduino in conjunction with the cloud
In our century, many have thought about creating their own startup. But to develop some interesting and useful product on your own is often difficult. Partly to save power, you can use the cloud. In particular, for IoT projects in the cloud, you can find a sufficient number of services. What is the “Internet of things” and how can it be used productively? Read about all this under the cut.
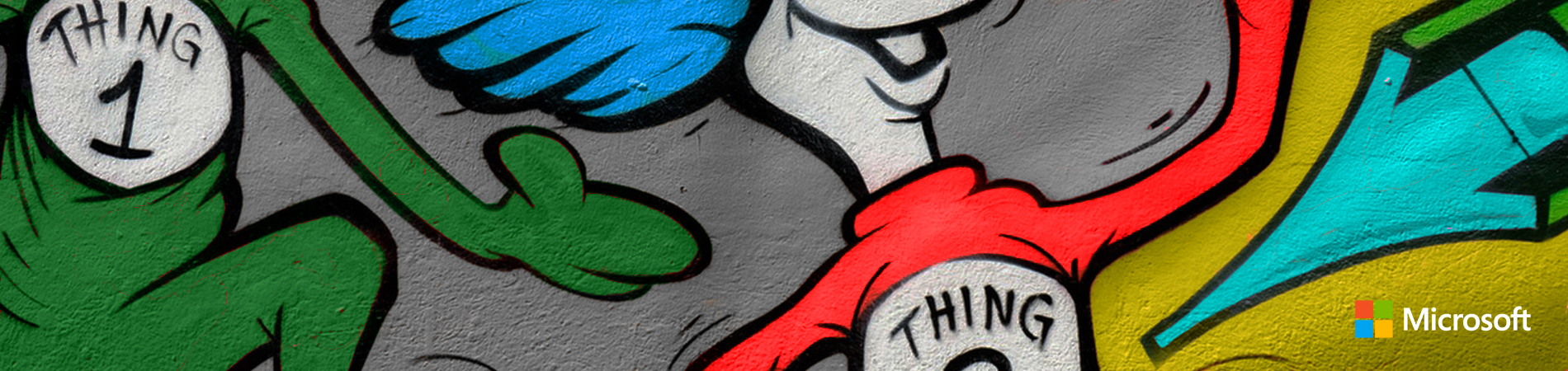
Note: we continue the series of publications of full versions of articles from the Hacker magazine. Spelling and punctuation of the author saved.
The Internet of things is not just a smart home or smart business. Experts predict that in the near future billions of devices will solve a variety of problems in a wide variety of areas. Yes, they already solve many problems.
For example, let's analyze the most stereotypical task: a device equipped with a sensor sends data taken from this sensor to the cloud. You can see the simplest example of a device that takes data from a light sensor (photoresistor) in the following photo: You can

power this device from the micro USB connector. On board the Genuino MKR1000 is an integrated Wi-Fi module. The fee is about 35 USD. Replacing one sensor with another usually does not particularly complicate the circuit. As you can see, a resistor is used in it, which stabilizes the signal being picked up and does not allow various interferences to affect it. But there are quite a lot of specialized literature about this. Now about something else.
Let's look at the following functional diagram of the device working with the Azure cloud, which can be called typical:

On it, the device interacts with the IoT hub. IoT hub is translated into Russian as the Center for the Internet of Things. This name quite conveys its essence to itself, since, as a rule, this service is used to interact with devices. It can both receive data from devices and send them messages / commands.
In addition to the Internet of Things Center, another service called Event Hub can interact with devices. The biggest difference in these services is that the IoT Hub can work with millions of devices, while the Event Hub works with thousands of devices, but it can receive millions of messages per second. The second big difference is that the IoT hub can send a command to the device, while the Event hub does not. In general, the IoT hub has more options. But the Event Hub retire early. As already written, it has a very high bandwidth. Often, Event hubs are used in projects not for interacting with devices, but as intermediate services.
A detailed comparison of the Azure IoT Center and Azure Event Hubs is available here .
IoT hub supports protocols such as MQTT, MQTT via WebSocket, AMQP, AMQP via WebSocket and HTTP. Event hub supports AMQP, AMQP via WebSocket and HTTP.
You can write code that generates a message according to the rules of the protocol and sends it, but it is better to use the SDK. The formation of a message using the HTTP protocol from the Arduino board was already discussed in this article .
This code works, but is far from ideal. In the same article, IoT hub configuration and device pairing were discussed.
Now there are new features and let me consider the use of the SDK and reconsider the configuration of the hub in a slightly different aspect. In addition, consider the configuration of other Azure IoT services.
Create an IoT hub on one device. When using a single device, IoT hub can be used for free. The name of the hub we come up with any arbitrary.
Hint: The IoT hub has the ability to receive files from the device. This feature is sometimes used as a legal hack. Data from several devices is transferred to one main device, which already saves them in a text file of a certain format and sends it to the cloud.

Now we need to create a virtual device in the Azure cloud that will match our real device. In English, this device is called device-twin, which means a double device.
Previously, you could create such a device using the Windows desktop utility Device Explorer. You can download it at the following link , finding the latest file
Now you don’t need to bother and you can create a device double right on the Azure portal by finding Device Explorer in the hub settings.

By clicking on the created device in Device Explorer, you can open device information. Of these, you need to take the connection string - the primary key. We will use it in Arduino code.
As an Azure IoT authentication, the hub uses two options: SSL + SAS or SSL + X.509 certificate. But the resources of Arduino are enough only for the first option. Still, this device is low power and does not have large sufficient computing capabilities.
It turns out that in any case, we need to flash our board with an SSL certificate.
This can be done using the cross-platform utility WiFi101 Firmware Updater or using the functionality built into the Arduino IDE.
But before that, you need to make some preparations in the Arduino IDE.
Install WiFi101 Library


After installing it in the IDE, examples of using this library will appear. One of these examples, called FirmwareUpdater, needs to be opened and written to the board. First, open:

Then install and select our board (boards for IoT are not yet included in the IDE's default package).
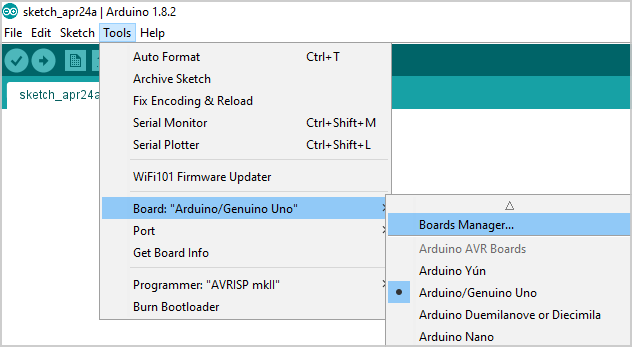
We open the board manager and look for the necessary board. In my case, this is MKR1000.

After installation, it is necessary to mark the board used in the same menu item.
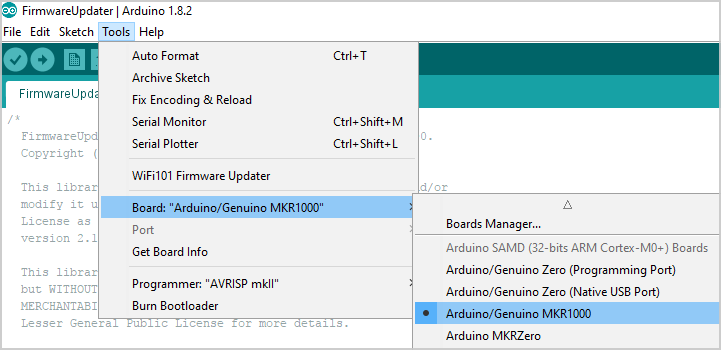
And select the port on which it is located:

Successive clicks on the Sketch - Upload menu will upload the sketch to the board. Now you can flash.
The first option is to open the Tools menu in the IDE - WiFi101 FirmwareUpdater, click Add domain and add the IoT hub URL.

Then click “Upload Certificates to WiFi module”.
Or open a separate WiFi101 Firmware Updater utility, enter the hub address (in my case ArduinoAzureHub.azure-devices.net) in the upper text field and click the Fetch button.
The certificate will be downloaded to the computer. After that, you need to select the COM port to which the device is connected and click the “Upload certificates” button that appears.

We install the following libraries in the same way as we recently installed the WiFi101 library:
Download the source code and in the folder
In the
The sketch code becomes operational and can be uploaded to Arduino.
The following code describes the model:
An example is, of course, only a starting point for your project. A template that can be changed to fit your requirements. I prefer to delete created Actions and create my own. My gist code is hosted on github .
I have a model with two fields that store the name of the device and the number taken from the sensor and one event:
The action code in my case is this:
It turns on or off the LED, depending on whether the integer 1 or 0 is received by the value of the light parameter.
The event fires if the following JSON is sent to the board:
If you find the following variable in the code:,
In the code,
Here, the analogue data is taken from the analog pin number 1 connected to the Arduino every second. This data is sent to the cloud as a string deviceId (device name) and the data itself as an integer iotdata.
For example, let's save data in a SQL Server database. Azure supports many data formats, but I think that SQL Server is the most popular format. Although, for storing JSON data in large volumes, the NOSQL format would probably be more suitable. Prices for SQL Server databases start at 5 USD per month. That is, for this money you can get a completely functional 2 Gb cloud base.
Since the database creation manual is quite voluminous, but there are no special nuances, I’ll leave a link to the official manuals: creating an Azure SQL database on the Azure portal and creating a table in the database using SQL Server Data Tools .
Often, if you already have Visual Studio installed, then nothing extra needs to be installed.
The easiest way to open the database for editing is to select View - Server Explorer from the Visual Studio menu. Here you can connect to your Azure subscription and open the database in SQL Server Object Explorer.

Well, already in this window you can create a table.

One point that must be mentioned. By default, access to SQL Servery in Azure and, accordingly, to its database is closed from all IP addresses for security reasons. That is, even knowing the username and password cannot be connected to the database. You need to go to the Azure portal and add the current IP to the list of allowed.

The joke about the fact that in IoT the letter S is responsible for Security does not work in this case.
The second most popular Azure IoT service is Stream Analytics. If we want to save data from the IoT hub to the database, then we need this service.
It is created very simply. It’s enough to come up with a name and select a group of resources with a region.
The result is such a window:
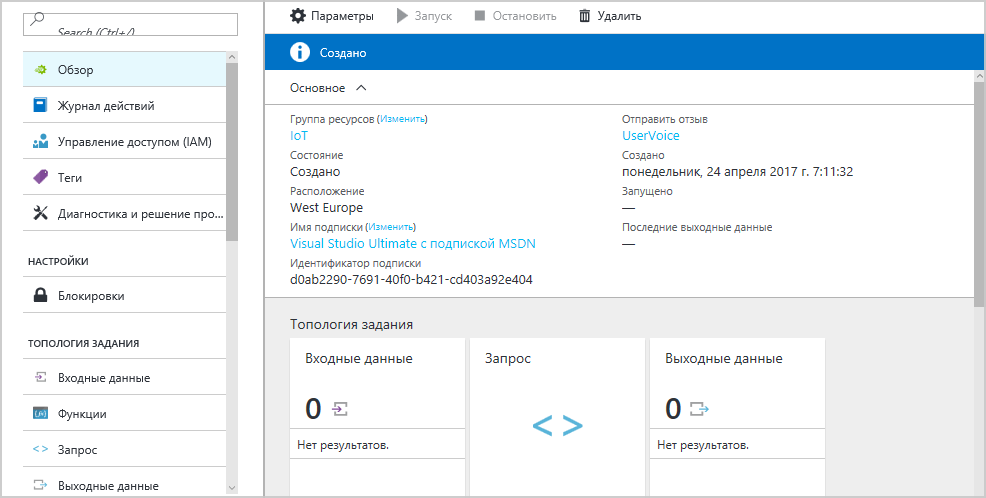
By clicking on the square with the input data, we can come up with an alias for the input data and fill out a form in which we indicate our IoT hub as the source.

And you need to similarly configure the output by clicking on the box with them:

Now you can create a request that will transfer data from the IoT hub to the database. This is the square between the input and output. In my example, the query is quite simple:
SELECT
iotdata, deviceId, System.Timestamp as eventtime
INTO dataout
FROM
indata
As you can see, the query language is quite similar to SQL. In this case, both the database and the Arduino code have the iotdata (integer) and deviceId (string) fields. In addition, an eventtime field of type datetime is created in the database. And in this field the value of time at the time of the movement from the incoming source to the receiver is recorded.
After downloading and installing the free Power BI utility, you can connect to the SQL Server database and get a representation of this data in the form of a graph or chart. The utility itself is no more complicated than Excel. Although the title contains serious words. BI stands for Business Intelligence.
Further, a small manual in the explanations and screenshots.
Button “Get data” - “Additional information ...”


Enter the address of our server and the name of the database. This data is set when creating the SQL Server database.

As well as username and password to access the database.

Select the necessary table.

And as a result, we can get a similar schedule.

As a result, we got an example of how you can access the data taken from the board while at the same time anywhere in the world. If desired, you can not only receive data from the device, but also send data / commands to it.
Cloud technologies of the Internet of things allow you to create projects with a minimum of programming. Most of the time is administration and configuration.
There are a lot of various examples of local projects created on the Arduino platform, and on other platforms. But if the device is available only via wi-fi or Bluetooth, then this pretty much limits the tasks performed. The future is with the Internet of things.
We remind you that this is the full version of an article from the Hacker magazine . Its author is Alexey Sommer .
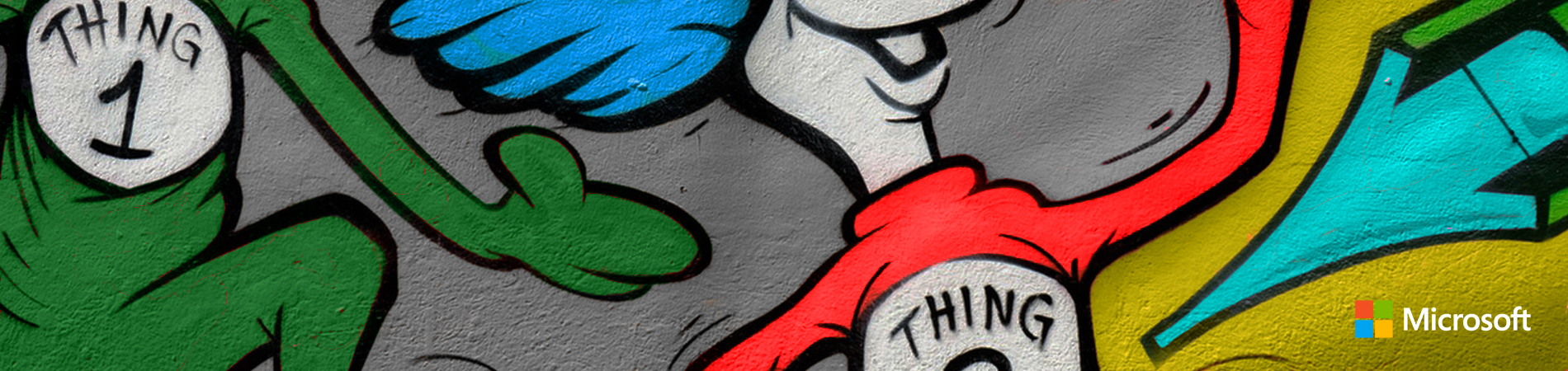
Note: we continue the series of publications of full versions of articles from the Hacker magazine. Spelling and punctuation of the author saved.
The Internet of things is not just a smart home or smart business. Experts predict that in the near future billions of devices will solve a variety of problems in a wide variety of areas. Yes, they already solve many problems.
For example, let's analyze the most stereotypical task: a device equipped with a sensor sends data taken from this sensor to the cloud. You can see the simplest example of a device that takes data from a light sensor (photoresistor) in the following photo: You can

power this device from the micro USB connector. On board the Genuino MKR1000 is an integrated Wi-Fi module. The fee is about 35 USD. Replacing one sensor with another usually does not particularly complicate the circuit. As you can see, a resistor is used in it, which stabilizes the signal being picked up and does not allow various interferences to affect it. But there are quite a lot of specialized literature about this. Now about something else.
Let's look at the following functional diagram of the device working with the Azure cloud, which can be called typical:

On it, the device interacts with the IoT hub. IoT hub is translated into Russian as the Center for the Internet of Things. This name quite conveys its essence to itself, since, as a rule, this service is used to interact with devices. It can both receive data from devices and send them messages / commands.
In addition to the Internet of Things Center, another service called Event Hub can interact with devices. The biggest difference in these services is that the IoT Hub can work with millions of devices, while the Event Hub works with thousands of devices, but it can receive millions of messages per second. The second big difference is that the IoT hub can send a command to the device, while the Event hub does not. In general, the IoT hub has more options. But the Event Hub retire early. As already written, it has a very high bandwidth. Often, Event hubs are used in projects not for interacting with devices, but as intermediate services.
A detailed comparison of the Azure IoT Center and Azure Event Hubs is available here .
IoT hub supports protocols such as MQTT, MQTT via WebSocket, AMQP, AMQP via WebSocket and HTTP. Event hub supports AMQP, AMQP via WebSocket and HTTP.
You can write code that generates a message according to the rules of the protocol and sends it, but it is better to use the SDK. The formation of a message using the HTTP protocol from the Arduino board was already discussed in this article .
This code works, but is far from ideal. In the same article, IoT hub configuration and device pairing were discussed.
Now there are new features and let me consider the use of the SDK and reconsider the configuration of the hub in a slightly different aspect. In addition, consider the configuration of other Azure IoT services.
Creating an IoT hub
Create an IoT hub on one device. When using a single device, IoT hub can be used for free. The name of the hub we come up with any arbitrary.
Hint: The IoT hub has the ability to receive files from the device. This feature is sometimes used as a legal hack. Data from several devices is transferred to one main device, which already saves them in a text file of a certain format and sends it to the cloud.

Create device-twin and get SAS
Now we need to create a virtual device in the Azure cloud that will match our real device. In English, this device is called device-twin, which means a double device.
Previously, you could create such a device using the Windows desktop utility Device Explorer. You can download it at the following link , finding the latest file
SetupDeviceExplorer.msi
or using the cross-platform utility iothub-explorer, which can be installed using NPM (Node.js Package Manager). Now you don’t need to bother and you can create a device double right on the Azure portal by finding Device Explorer in the hub settings.

By clicking on the created device in Device Explorer, you can open device information. Of these, you need to take the connection string - the primary key. We will use it in Arduino code.
Arduino board firmware SSL certificate
As an Azure IoT authentication, the hub uses two options: SSL + SAS or SSL + X.509 certificate. But the resources of Arduino are enough only for the first option. Still, this device is low power and does not have large sufficient computing capabilities.
It turns out that in any case, we need to flash our board with an SSL certificate.
This can be done using the cross-platform utility WiFi101 Firmware Updater or using the functionality built into the Arduino IDE.
But before that, you need to make some preparations in the Arduino IDE.
Install WiFi101 Library


After installing it in the IDE, examples of using this library will appear. One of these examples, called FirmwareUpdater, needs to be opened and written to the board. First, open:

Then install and select our board (boards for IoT are not yet included in the IDE's default package).
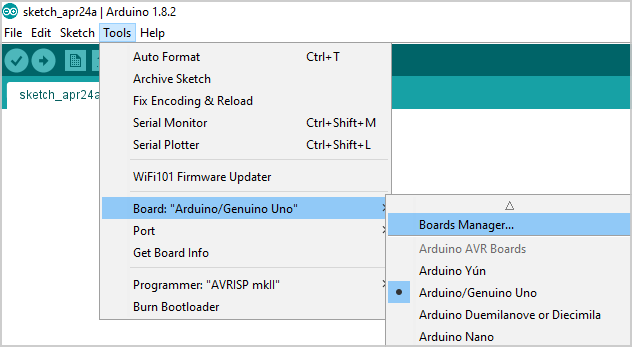
We open the board manager and look for the necessary board. In my case, this is MKR1000.

After installation, it is necessary to mark the board used in the same menu item.
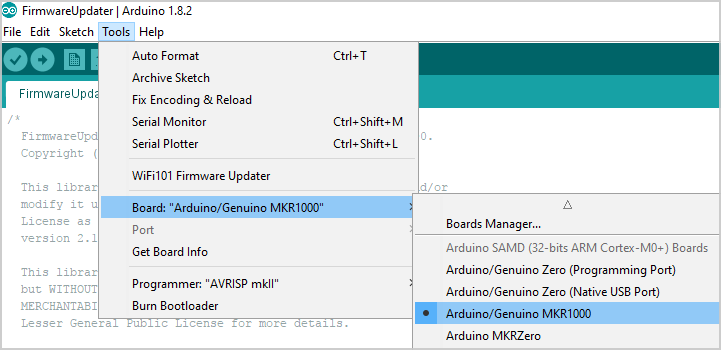
And select the port on which it is located:

Successive clicks on the Sketch - Upload menu will upload the sketch to the board. Now you can flash.
The first option is to open the Tools menu in the IDE - WiFi101 FirmwareUpdater, click Add domain and add the IoT hub URL.

Then click “Upload Certificates to WiFi module”.
Or open a separate WiFi101 Firmware Updater utility, enter the hub address (in my case ArduinoAzureHub.azure-devices.net) in the upper text field and click the Fetch button.
The certificate will be downloaded to the computer. After that, you need to select the COM port to which the device is connected and click the “Upload certificates” button that appears.

Sketching and Using the Azure IoT library for the Arduino
We install the following libraries in the same way as we recently installed the WiFi101 library:
- AzureIoTHub
- AzureIoTUtility
- AzureIoTProtocol_HTTP
- RTCZero
Download the source code and in the folder
examples
we find the project simplesample_http
where you can find the code for samd
(Atmel SAMD Based boards). In the
iot_configs.h
c file IOT_CONFIG_CONNECTION_STRING
, we assign the value to the value of the connection string that we received on the Azure portal. We fill in the values IOT_CONFIG_WIFI_SSID
and, IOT_CONFIG_WIFI_PASSWORD
accordingly, the name of your Wi-Fi access point and its password. The sketch code becomes operational and can be uploaded to Arduino.
The following code describes the model:
DECLARE_MODEL(ContosoAnemometer,
WITH_DATA(ascii_char_ptr, DeviceId),
WITH_DATA(int, WindSpeed),
WITH_DATA(float, Temperature),
WITH_DATA(float, Humidity),
WITH_ACTION(TurnFanOn),
WITH_ACTION(TurnFanOff),
WITH_ACTION(SetAirResistance, int, Position)
);
WITH_DATA
describes the data that can be sent from the device. WITH_ACTION
describes the events that may occur when the device receives any commands. An example is, of course, only a starting point for your project. A template that can be changed to fit your requirements. I prefer to delete created Actions and create my own. My gist code is hosted on github .
I have a model with two fields that store the name of the device and the number taken from the sensor and one event:
BEGIN_NAMESPACE(IoTSample);
DECLARE_MODEL
(LightSensorDataModel,
WITH_DATA(ascii_char_ptr, deviceId),
WITH_DATA(int, iotdata),
WITH_ACTION(DuckAction, int, light)
);
END_NAMESPACE(IoTSample);
The action code in my case is this:
EXECUTE_COMMAND_RESULT DuckAction(LightSensorDataModel* device, int light)
{
digitalWrite(2, light);
return EXECUTE_COMMAND_SUCCESS;
}
It turns on or off the LED, depending on whether the integer 1 or 0 is received by the value of the light parameter.
The event fires if the following JSON is sent to the board:
{"Name" : "DuckAction", "Parameters" : {"light":1}}
If you find the following variable in the code:,
unsigned int minimumPollingTime = 9;
you can change the delay in polling new messages. In other words, how often the device checks to see if new messages have appeared for it. The value is in seconds. In the code,
void simplesample_http_run(void)
I add the following code:while (1)
{
myIoTdata->deviceId = "ArduinoAzureTwin";
myIoTdata->iotdata = analogRead(1);
unsigned char* destination;
size_t destinationSize;
if (SERIALIZE(&destination, &destinationSize, myIoTdata->deviceId, myIoTdata->iotdata) != CODEFIRST_OK)
{
(void)printf("Failed to serializern");
}
else
{
sendMessage(iotHubClientHandle, destination, destinationSize);
}
IoTHubClient_LL_DoWork(iotHubClientHandle);
ThreadAPI_Sleep(1000);
}
DESTROY_MODEL_INSTANCE(myIoTdata);
Here, the analogue data is taken from the analog pin number 1 connected to the Arduino every second. This data is sent to the cloud as a string deviceId (device name) and the data itself as an integer iotdata.
Connect to other Azure services
For example, let's save data in a SQL Server database. Azure supports many data formats, but I think that SQL Server is the most popular format. Although, for storing JSON data in large volumes, the NOSQL format would probably be more suitable. Prices for SQL Server databases start at 5 USD per month. That is, for this money you can get a completely functional 2 Gb cloud base.
Since the database creation manual is quite voluminous, but there are no special nuances, I’ll leave a link to the official manuals: creating an Azure SQL database on the Azure portal and creating a table in the database using SQL Server Data Tools .
Often, if you already have Visual Studio installed, then nothing extra needs to be installed.
The easiest way to open the database for editing is to select View - Server Explorer from the Visual Studio menu. Here you can connect to your Azure subscription and open the database in SQL Server Object Explorer.

Well, already in this window you can create a table.

One point that must be mentioned. By default, access to SQL Servery in Azure and, accordingly, to its database is closed from all IP addresses for security reasons. That is, even knowing the username and password cannot be connected to the database. You need to go to the Azure portal and add the current IP to the list of allowed.

The joke about the fact that in IoT the letter S is responsible for Security does not work in this case.
Stream analytics
The second most popular Azure IoT service is Stream Analytics. If we want to save data from the IoT hub to the database, then we need this service.
It is created very simply. It’s enough to come up with a name and select a group of resources with a region.
The result is such a window:
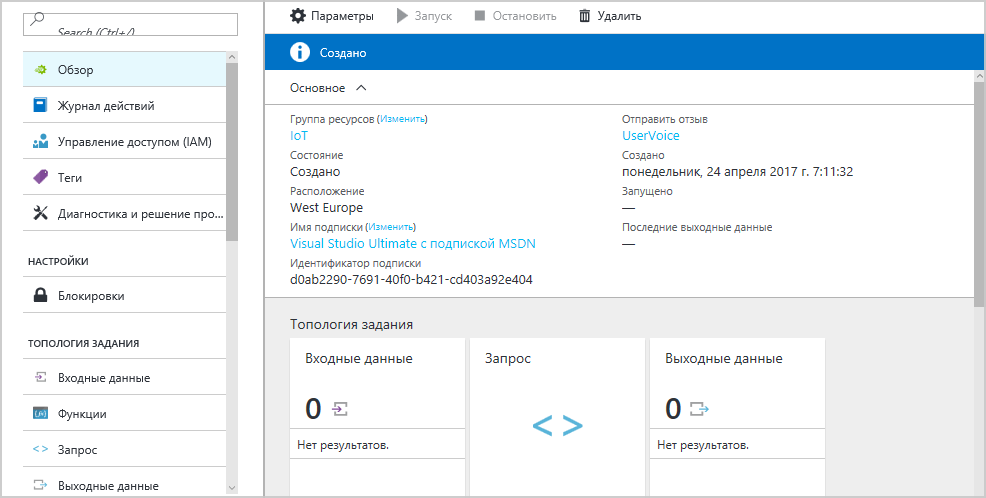
By clicking on the square with the input data, we can come up with an alias for the input data and fill out a form in which we indicate our IoT hub as the source.

And you need to similarly configure the output by clicking on the box with them:

Now you can create a request that will transfer data from the IoT hub to the database. This is the square between the input and output. In my example, the query is quite simple:
SELECT
iotdata, deviceId, System.Timestamp as eventtime
INTO dataout
FROM
indata
As you can see, the query language is quite similar to SQL. In this case, both the database and the Arduino code have the iotdata (integer) and deviceId (string) fields. In addition, an eventtime field of type datetime is created in the database. And in this field the value of time at the time of the movement from the incoming source to the receiver is recorded.
Power BI
After downloading and installing the free Power BI utility, you can connect to the SQL Server database and get a representation of this data in the form of a graph or chart. The utility itself is no more complicated than Excel. Although the title contains serious words. BI stands for Business Intelligence.
Further, a small manual in the explanations and screenshots.
Button “Get data” - “Additional information ...”


Enter the address of our server and the name of the database. This data is set when creating the SQL Server database.

As well as username and password to access the database.

Select the necessary table.

And as a result, we can get a similar schedule.

Conclusion
As a result, we got an example of how you can access the data taken from the board while at the same time anywhere in the world. If desired, you can not only receive data from the device, but also send data / commands to it.
Cloud technologies of the Internet of things allow you to create projects with a minimum of programming. Most of the time is administration and configuration.
There are a lot of various examples of local projects created on the Arduino platform, and on other platforms. But if the device is available only via wi-fi or Bluetooth, then this pretty much limits the tasks performed. The future is with the Internet of things.
We remind you that this is the full version of an article from the Hacker magazine . Its author is Alexey Sommer .