
Programming Contest: JSDash
UPDATE: The competition is over. The results .
The company Hola announces the long-awaited summer programming contest! Winners are expected prizes:
Authors of interesting decisions will be invited for interviews.
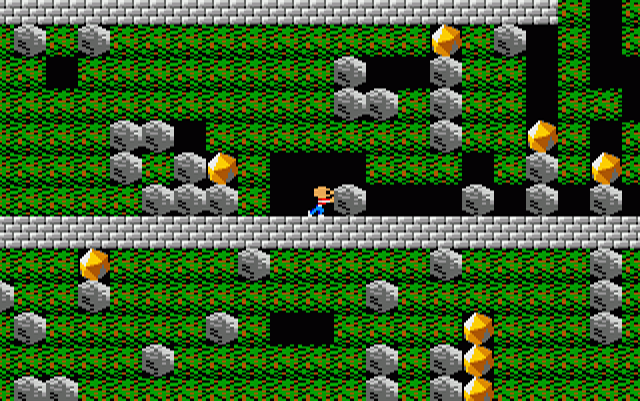
The terms of the competition in English are available on GitHub . Below is a translation into Russian.
Let's play a text game! Undoubtedly, you recognize in it a clone of the classic video game, first published in 1984.
Your goal is to write artificial intelligence that plays this game, making decisions instead of humans. The game will take place in real time, and your script will have as much time for making decisions as a person.
We recommend that you run the game / jsdash.js script and play the game several times before reading further. (Note: we developed and tested the game under xterm on Linux. On other systems it may or may not work.)
The arrow keys can be navigated in four directions. The green letter
For each diamond taken, 1 point is awarded. If you collect 3 diamonds at intervals of less than 2 seconds, in addition to points for each of them, 3 prize points are awarded. If you continue to quickly collect diamonds without making intervals of 2 seconds or longer, you will get 5 points after the fifth diamond, 7 points after the seventh (in addition to all previous bonuses), and so on for each prime number. For a killed butterfly, 10 points are awarded, provided that the player was not killed by an explosion.
The time limit for the game is 2 minutes. You can interrupt the game earlier by pressing Q, Esc or Ctrl-C; scored points are not lost. The P key allows you to pause the game.
The rest of the time, points and messages about prize streams (hot streak) are displayed in the status bar under the playing field.
The mechanics of our game as a whole correspond to the 1984 model (it is known for its detailed description on the Boulder Dash fan site ), but for simplicity we decided not to use all types of objects. The biggest differences are the scoring system described above and the fact that there is no way out at the level. The goal of the game is to score as many points as possible before the game ends in the death of a character or after a time has passed. If you are interested in the details of game mechanics, read the source code for the game.js module .
The solution is a Node.js module without dependencies. The module should export one function:
The game will load the module and call the function
If your function raises an exception, the game will end with a score of 0 points. This will not prevent your program from playing and gaining points at other levels.
Your script will be run in a separate process. Even if it freezes, this will not slow down the game (while the character will stand still).
The game status is updated every 100 ms. If the function
A very simple example of a working artificial intelligence script is given in the game / example.js file . Each round he finds all possible moves (directions in which, next to the player’s position, there is empty space, earth, a stone that can be moved, or a diamond) and selects one of them randomly. Usually this script is killed shortly after the start of the game.
The jsdash.js script we provide on GitHub is not only an interactive game, but also a powerful testing tool. Run it with a parameter
Each solution sent to us will be launched on at least 20 automatically generated levels. The participant whose decision will score the most points at all levels will be recognized as the winner. We reserve the right to increase the number of levels (for all participants), if necessary, in order to exclude a “draw” between leaders; if this does not help, the one who sent his decision earlier will win.
When testing each solution, the same set of seeds will be used for the pseudo-random number generator so that the programs of all participants receive the same levels. When launching your solutions, we will use the default settings:
However, we recommend that you pay attention to the full range of available command-line options that can help you with debugging.
At the end of the competition, the records of the games of each solution at each of the test levels will be published. These records can be played back with the following command:
Testing of all solutions will take place on the c3.large virtual server (see hardware specifications here) on Amazon AWS running Ubuntu 14.04 (amd64). Solutions will be tested one by one in the absence of other load on the machine.
We fix bugs in the test script that the participants tell us about. Stay tuned for the game / CHANGELOG.md file !
To submit decisions, use the form on our website . Email decisions are not accepted!
Since the solution code is often generated, minimized, or translated from another language, the form also contains a field for sending an archive with source tests. If the code is generated, turn on the generator there; if minimized, enable the original version; if the code is translated from CoffeeScript or another language, include the code in the language in which it is written. It is also advisable to include in the archive a README file with a brief description of the approach to the solution (in English). The archive must be in the format tar.gz, tar.bz2 or zip. The contents of the archive will be published, but will not be tested (we only test the JS file that you send outside the archive).
The maximum size of the JS file is set to 64 MiB. This is an arbitrarily chosen figure, which exists mainly so that someone's “solution” does not immediately fill up the disk for us. If your solution is really more than 64 MiB, write to us and we will increase the limit.
If you have questions about the conditions of the problem or problems with sending the solution, please write a comment or letter .
Good luck to all participants!
The company Hola announces the long-awaited summer programming contest! Winners are expected prizes:
- First place: 3000 USD.
- Second place: 2000 USD.
- Third place: 1000 USD.
- If you send someone a link to this contest by putting our address in CC, and this person takes the prize, you will receive half the amount of the prize (of course, not to the detriment of the winner’s award). For one winner, only one person can receive such an award - the one who sent the link first.
Authors of interesting decisions will be invited for interviews.
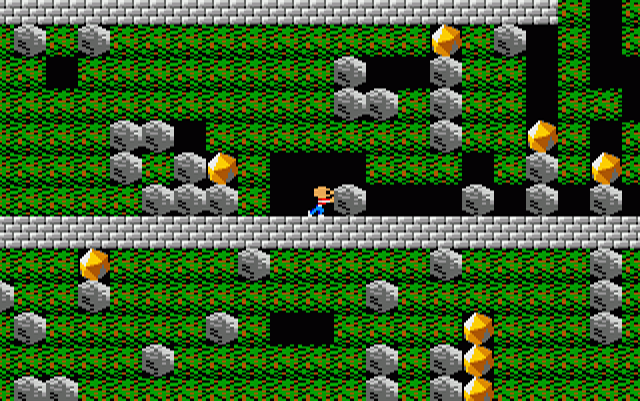
rules
The terms of the competition in English are available on GitHub . Below is a translation into Russian.
- Submit solutions using this form . No decisions are made by email.
- Decisions are made until August 17, 2017 , 23:59:59 UTC.
- Preliminary results will be published on August 24, 2017 , and the final announcement of the winners is August 31, 2017 .
- You can send decisions multiple times, but from each participant only the most recent decision will be considered, sent before the deadline for accepting work.
- For testing, we will use Node.js v8.1.3 (the current version at the time of publication). You can use any language features supported by the interpreter in the standard configuration.
- All solution code must be in a single JS file .
- The solution should be on JS. If you prefer CoffeeScript or similar languages, you must translate the solution in JS before submitting.
- If your JS file is a product of generation, minification and / or compilation from other languages like CoffeeScript, please attach an archive with source codes, as well as, preferably, a description of the approach. The contents of this archive will be published, but we will not test it.
- You cannot load any modules , even those included in the standard Node.js.
- We need to know your full name, but if you want, we will publish an alias instead. We will keep your email address confidential.
- Ask questions on the condition of the task in the comments on this publication or by email .
Jsdash
Let's play a text game! Undoubtedly, you recognize in it a clone of the classic video game, first published in 1984.
Your goal is to write artificial intelligence that plays this game, making decisions instead of humans. The game will take place in real time, and your script will have as much time for making decisions as a person.
We recommend that you run the game / jsdash.js script and play the game several times before reading further. (Note: we developed and tested the game under xterm on Linux. On other systems it may or may not work.)
The game
The arrow keys can be navigated in four directions. The green letter
A
is you. You can move through empty space or earth ( :
), push stones ( O
) horizontally into empty space and collect diamonds ( *
). It is impossible to pass through bricks ( +
) and steel ( #
). Stones and diamonds fall when they are left without support, and also roll sideways from each other and from bricks. Falling objects kill the player. Butterflies (animation /|\-
) explode in contact with the player, from hitting a falling object, as well as being locked without the possibility of movement. A butterfly explosion absorbs any material other than steel and can kill a player. After the explosion, diamonds are formed that can be collected.For each diamond taken, 1 point is awarded. If you collect 3 diamonds at intervals of less than 2 seconds, in addition to points for each of them, 3 prize points are awarded. If you continue to quickly collect diamonds without making intervals of 2 seconds or longer, you will get 5 points after the fifth diamond, 7 points after the seventh (in addition to all previous bonuses), and so on for each prime number. For a killed butterfly, 10 points are awarded, provided that the player was not killed by an explosion.
The time limit for the game is 2 minutes. You can interrupt the game earlier by pressing Q, Esc or Ctrl-C; scored points are not lost. The P key allows you to pause the game.
The rest of the time, points and messages about prize streams (hot streak) are displayed in the status bar under the playing field.
The mechanics of our game as a whole correspond to the 1984 model (it is known for its detailed description on the Boulder Dash fan site ), but for simplicity we decided not to use all types of objects. The biggest differences are the scoring system described above and the fact that there is no way out at the level. The goal of the game is to score as many points as possible before the game ends in the death of a character or after a time has passed. If you are interested in the details of game mechanics, read the source code for the game.js module .
Solutions
The solution is a Node.js module without dependencies. The module should export one function:
play(screen)
The game will load the module and call the function
play
once, passing the initial state of the game as a parameter screen
. It is an array of lines, one for each line of the screen from top to bottom, including the status bar. The lines will contain exactly what you see on the screen, only without coloring in ANSI colors (the game can be seen in this mode on the console if you run it with the parameter --no-color
). The function play
must be a generator. To make a move, it must generate (yield) value 'u'
, 'd'
, 'r'
or 'l'
to step up, down, right or left, respectively. You can also generate'q'
or just shut down the generator (return) to end the game ahead of schedule (the points gained are not lost). If you generate any other value, it means the move "stay in place." Trying to go in a direction in which it is impossible to move (for example, into a wall) is not prohibited: the character will simply remain in place. After each yield, the contents of the array are screen
updated, and your code can analyze it again for further decisions. If your function raises an exception, the game will end with a score of 0 points. This will not prevent your program from playing and gaining points at other levels.
Your script will be run in a separate process. Even if it freezes, this will not slow down the game (while the character will stand still).
The game status is updated every 100 ms. If the function
play
will generate commands faster, then the character will move 10 times per second. After each command, the generator will be blocked on instructions yield
until the end of the round, 100 ms long. If the function “thinks” over a move for longer than 100 ms, it starts skipping moves, and then the character will remain in place in those rounds when the function did not manage to make a move. In this case, the generator will also not see some intermediate states of the screen. For example, if a script “thinks” for 250 ms between two instructionsyield
, then he will not see two states of the screen, but the character will remain motionless for two rounds; Thus, two opportunities to make a move, which would be if the program worked faster, would be missed. After that, the generator will be blocked for 50 ms until the end of the round, and the command generated by it will be executed. A very simple example of a working artificial intelligence script is given in the game / example.js file . Each round he finds all possible moves (directions in which, next to the player’s position, there is empty space, earth, a stone that can be moved, or a diamond) and selects one of them randomly. Usually this script is killed shortly after the start of the game.
Testing
The jsdash.js script we provide on GitHub is not only an interactive game, but also a powerful testing tool. Run it with a parameter
--help
to find out about all its features. Each solution sent to us will be launched on at least 20 automatically generated levels. The participant whose decision will score the most points at all levels will be recognized as the winner. We reserve the right to increase the number of levels (for all participants), if necessary, in order to exclude a “draw” between leaders; if this does not help, the one who sent his decision earlier will win.
When testing each solution, the same set of seeds will be used for the pseudo-random number generator so that the programs of all participants receive the same levels. When launching your solutions, we will use the default settings:
jsdash.js --ai=submission.js --log=log.json --seed=N
However, we recommend that you pay attention to the full range of available command-line options that can help you with debugging.
At the end of the competition, the records of the games of each solution at each of the test levels will be published. These records can be played back with the following command:
jsdash.js --replay=log.json
Testing of all solutions will take place on the c3.large virtual server (see hardware specifications here) on Amazon AWS running Ubuntu 14.04 (amd64). Solutions will be tested one by one in the absence of other load on the machine.
We fix bugs in the test script that the participants tell us about. Stay tuned for the game / CHANGELOG.md file !
Submitting Solutions
To submit decisions, use the form on our website . Email decisions are not accepted!
Since the solution code is often generated, minimized, or translated from another language, the form also contains a field for sending an archive with source tests. If the code is generated, turn on the generator there; if minimized, enable the original version; if the code is translated from CoffeeScript or another language, include the code in the language in which it is written. It is also advisable to include in the archive a README file with a brief description of the approach to the solution (in English). The archive must be in the format tar.gz, tar.bz2 or zip. The contents of the archive will be published, but will not be tested (we only test the JS file that you send outside the archive).
The maximum size of the JS file is set to 64 MiB. This is an arbitrarily chosen figure, which exists mainly so that someone's “solution” does not immediately fill up the disk for us. If your solution is really more than 64 MiB, write to us and we will increase the limit.
If you have questions about the conditions of the problem or problems with sending the solution, please write a comment or letter .
Good luck to all participants!