At the behest ... (Pike programming language)
- Tutorial
- Recovery mode
This article is a very brief introduction to Pike. Admit it - few of you have heard about this language. However, the Pike language is even used in production (for Opera to work in Turbo mode).
Brief characteristics:
- interpreted (you will not think what to do during compilation);
- syntax: based on C (with minimal differences);
- license - GNU GPL, GNU LGPL and MPL;
- object oriented;
- with a garbage collector (which, incidentally, can be prompted if you really need to);
- ...
History
Language appeared back in 1994. Posted by Fredrik Hübinette. The predecessor is considered the LPC language (an object-oriented language based on the C language, created primarily for game development - LPC This is actually an interesting story - but there is no point in copy-paste the wiki. In short, if it weren’t for the game, there would be no language.
I have to say right away that the documentation is not all right. Not all examples (even for beginners) will work (and it could even be Hello world!).
You can start the interpreter to get started. To do this, you can simply type pike in the console without parameters. And experiment. But we will not do that. Let's just write (for example, in a notebook).
Hello World!
Program text:
We save this text in hello.pike and run it on the command line: pike hello.pike
Now with a window:
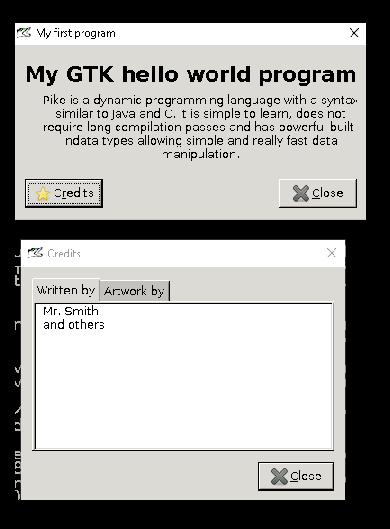
As you can see, the work goes through the GTK. The return of -1 from the main function is necessary so that the program does not immediately stop working, otherwise you will not see the window. Exit and programs is done by clicking the button to close the window using the attached lambda function lambda () {exit (0);}
In the official tutorial, GTK.Alert (“Hello world!”) Is used for this, but however it didn’t work for me (version 8.0) - apparently the tutorial is out of date.
Data Structures:
The syntax of working with basic data structures is good.
Arrays :
Result :
redgreenwhite
redgreenyellow
redgreenyellowredgreenwhite
redgreen
redgreenyellowwhite
yellowwhite
yellow
Maps :
Result :
red: 4 green: 8 white: 15 blue: 88
blue: 88
red: 4 white: 15
red: 4 white: 15 green: 8 blue: 88
green: 8 blue: 88
There are also so-called multisets . Essentially the same as mapping, but without values:
Result :
1: 1 1: 1 3.0: 1: 1 hi!: 1
Objects
Result :
vaz red
mers green
mers
The create method plays the role of a constructor. There are access modifiers. But be careful with the static modifier. Not only does it mean not at all what you thought - it is also depricated.
Connection with Java
And now we jerk Java code (and why not if we can?):
As you can see from the example, accessing the Java classes comes through Java.pkg. When printing, you must remember to cast Java objects to a string using (string). You can call ordinary Java methods. As you can see from the example, there is even a special design for HashMap to facilitate the work (which, however, is not surprising).
Working with the Internet
Download the page from the Internet and display it in the console:
Pike remembers the USSR:
Conclusion
My personal opinion is a curious language, but very crude. Or you can consider it as a language created for a specific project. The documentation is very lame. But let all the flowers grow.
Links
→ Home
→ Wikipedia
→ Language article
→ github
→ Roxen (Pike web server)
Brief characteristics:
- interpreted (you will not think what to do during compilation);
- syntax: based on C (with minimal differences);
- license - GNU GPL, GNU LGPL and MPL;
- object oriented;
- with a garbage collector (which, incidentally, can be prompted if you really need to);
- ...
History
Language appeared back in 1994. Posted by Fredrik Hübinette. The predecessor is considered the LPC language (an object-oriented language based on the C language, created primarily for game development - LPC This is actually an interesting story - but there is no point in copy-paste the wiki. In short, if it weren’t for the game, there would be no language.
I have to say right away that the documentation is not all right. Not all examples (even for beginners) will work (and it could even be Hello world!).
You can start the interpreter to get started. To do this, you can simply type pike in the console without parameters. And experiment. But we will not do that. Let's just write (for example, in a notebook).
Hello World!
Program text:
intmain(){
write("Hello world!\n");
return0;
}
We save this text in hello.pike and run it on the command line: pike hello.pike
Now with a window:
intmain(){
GTK.setup_gtk();
object w = GTK.AboutDialog();
w.set_program_name("My GTK hello world program");
w.signal_connect("destroy", lambda(){exit(0);});
w.set_title("My first program");
w.set_comments("Pike is a dynamic programming language with a syntax similar to Java and C. "+
"It is simple to learn, does not require long compilation passes and has powerful built-in" +
"data types allowing simple and really fast data manipulation.");
array(string) arr1=({"Mr. Smith", "and others"});
array(string) arr2=({"Mrs. Smith", "and others"});
w.set_authors(arr1);
w.set_artists(arr2);
w.show_now();
return-1;
}
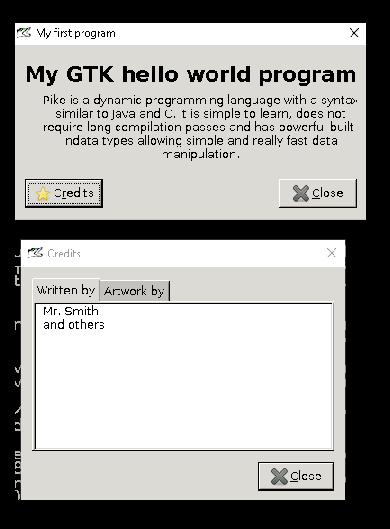
As you can see, the work goes through the GTK. The return of -1 from the main function is necessary so that the program does not immediately stop working, otherwise you will not see the window. Exit and programs is done by clicking the button to close the window using the attached lambda function lambda () {exit (0);}
In the official tutorial, GTK.Alert (“Hello world!”) Is used for this, but however it didn’t work for me (version 8.0) - apparently the tutorial is out of date.
Data Structures:
The syntax of working with basic data structures is good.
Arrays :
intmain(){
array(string) arr1 = ({ "red", "green", "white" });
write(arr1);
write("\n");
array(string) arr2 = ({ "red", "green", "yellow" });
write(arr2);
write("\n");
write(arr2 + arr1); //просто все элементы двух массивов
write("\n");
write(arr2 & arr1); //пересечение
write("\n");
write(arr2 | arr1); //объединение множеств
write("\n");
write(arr2 ^ arr1); //xor - т.е. только те элементы которые не являются общими
write("\n");
write(arr2 - arr1); //разность
write("\n");
return0;
}
Result :
redgreenwhite
redgreenyellow
redgreenyellowredgreenwhite
redgreen
redgreenyellowwhite
yellowwhite
yellow
Maps :
intmain(){
mapping map2 = (["red":4, "white":42, "blue": 88]);
mapping map1 = (["red":4, "green":8, "white":15]);
print_map(map2 + map1);
print_map(map2 - map1);
print_map(map2 & map1);
print_map(map2 | map1);
print_map(map2 ^ map1);
return0;
}
voidprint_map(mapping m){
array(string) arr;
arr = indices(m);
foreach(arr, string key){
write(key + ":" + m[key] + " ");
write("\n");
}
Result :
red: 4 green: 8 white: 15 blue: 88
blue: 88
red: 4 white: 15
red: 4 white: 15 green: 8 blue: 88
green: 8 blue: 88
There are also so-called multisets . Essentially the same as mapping, but without values:
intmain(){
multiset o = (< "", 1, 3.0, 1, "hi!" >);
print_multiset(o);
return0;
}
voidprint_multiset(multiset m){
array(string) arr;
arr = indices(m);
foreach(arr, string key){
write(key + ":" + m[key] + " ");
};
write("\n");
}
Result :
1: 1 1: 1 3.0: 1: 1 hi!: 1
Objects
classcar {publicstring color;
publicstring mark;
privatestring driver;
voidcreate(string c, string m, string d){
color = c;
mark = m;
driver = d;
}
stringwho(){
return mark + " " + color + "\n";
}
}
intmain(){
car car1 = car("red", "vaz", "Mike");
write(car1.who());
car car2 = car("green", "mers", "Nik");
write(car2.who());
write(car2.mark);
return0;
}
Result :
vaz red
mers green
mers
The create method plays the role of a constructor. There are access modifiers. But be careful with the static modifier. Not only does it mean not at all what you thought - it is also depricated.
Connection with Java
And now we jerk Java code (and why not if we can?):
intmain(){
float pi = Java.pkg.java.lang.Math.PI;
write("Pi = " + pi + "\n");
object syst = Java.pkg.java.lang.System;
write("time = " + syst.currentTimeMillis() + "\n");
object str = Java.pkg.java.lang.String("...Hello!...");
write((string)str.substring(3,str.length()-3) + "\n");
object map2 = Java.pkg.java.util.HashMap();
object key = Java.pkg.java.lang.String("oops");
object val = Java.pkg.java.lang.String("ha-ha");
map2.put(key, val);
write((string) map2.get("oops") + "\n");
object map = Java.JHashMap(([ "one":1, "two":2 ]));
write((string) map.get("two") + "\n");
return0;
}
As you can see from the example, accessing the Java classes comes through Java.pkg. When printing, you must remember to cast Java objects to a string using (string). You can call ordinary Java methods. As you can see from the example, there is even a special design for HashMap to facilitate the work (which, however, is not surprising).
Working with the Internet
Download the page from the Internet and display it in the console:
intmain(){
Protocols.HTTP.Query web_page;
web_page = Protocols.HTTP.get_url("https://pike.lysator.liu.se/about/");
string page_contents = web_page->data();
write(page_contents);
return0;
}
Pike remembers the USSR:
intmain(){
Geography.Countries.Country c = Geography.Countries.USSR;
write(c.name + "\n");
return0;
}
Deviation from the topic
(Готовя этот материал, параллельно экспериментировал с задачей определения страны по IP адресу — по своему адресу конечно. И вздрогнул когда перепутал программы и мне в ответ пришло «USSR»).
Conclusion
My personal opinion is a curious language, but very crude. Or you can consider it as a language created for a specific project. The documentation is very lame. But let all the flowers grow.
Links
→ Home
→ Wikipedia
→ Language article
→ github
→ Roxen (Pike web server)