The book "React in action"
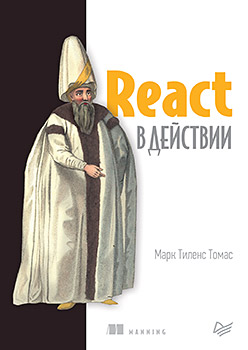
In the second half of the book, various ways of interacting with React are discussed. You will learn basic life-cycle methods, learn how to create data flow, forms, and test applications. For a snack, you will find material on the architecture of the React-application, interaction with Redux, an excursion into the server rendering and review of React Native.
Excerpt Chapter 11. Redux and React Integration
??????
- Gearboxes - Redux's way to determine how the state should change. ????
- Apply Redux with React.
- Convert Letters Social to use the Redux application architecture.
- Adding functionality to the application likes and comments.
Here you will continue the work you did in the previous chapter to create the basic elements of the Redux architecture. You will work on integrating React with Redux actions and storage and learn how gearboxes work. Redux is a variant of the Flux template, designed with React in mind, it works well with unidirectional data flow and the React API. Although this is not a universal choice, many large React applications consider Redux as one of the best options when implementing a state management solution. Follow their lead and apply it to the Letters Social.
Getting the source code
As before, you can get the source code for the examples in this chapter by going to the GitHub repository at github.com/react-in-action/letters-social . If you plan to start working here yourself from scratch, take the source code of the examples from Chapters 7 and 8 (if you studied them and completed the examples yourself) or refer to the branch relating to this chapter (chapter-10-11).
Remember that each branch contains the final chapter code (for example, chapter-10-11 contains the code obtained at the end of chapters 10 and 11). You can execute one of the following commands of your choice in the command line shell to get the example code from the current chapter. If there is no repository at all, run the command:git clone git@github.com:react-in-action/letters-social.git
If you already have a repository cloned, then the following:git checkout chapter-10-11
You may have moved here from another chapter, so it’s worth checking whether you have all the necessary dependencies installed using the command:npm install
11.1. Gearboxes determine how the state should change
You can create and send actions and handle errors, but they have no effect on the state. To handle incoming actions, you must configure the gearboxes. Remember that actions are just ways to report that an event has occurred, and to provide some information about what happened, nothing more. The task of gearboxes is to indicate how the state of the storage will change in response to these actions. In fig. Figure 11.1 shows how gearboxes fit into the more general picture of Redux, which we have already seen.
But what are gearboxes? If you still enjoyed the simplicity of Redux, then you will not be disappointed: these are just simple functions that have one goal. Gearboxes are pure functions that take a previous state and action as arguments and return the next state. According to the Redux documentation, they are called gearboxes, because the signature of their method looks like data passed to Array.prototype.reduce (for example, [1,2,3]. Reduce ((a, b) => a + b, 0).
Gear units must be pure functions, which means that, taking into account the input, they will each time produce the same corresponding output. This contrasts with actions or middleware where side effects occur and API calls often occur. Doing something asynchronous or unclean (for example, calling Date.now or Math.random ()) on gearboxes is an anti-pattern that can affect the performance or reliability of an application. Redux documents contain the following clause: “After receiving the same arguments, it must calculate the next state and return it. No surprises. No side effects. No API calls. No changes. Just a calculation. ” For more on this, see redux.js.org/basics/reducers .
11.1.1. State form and initial state
Gearboxes will begin to work on changing the only Redux store, so it's time to talk about what shape the store will take. Designing the state form of any application will affect how the user interface of the application works (and at the same time it is influenced by this work), but as a rule, it is recommended to keep the raw data as separate as possible from the user interface data. One way to do this is to store values like identifiers separate from their data and use identifiers to search for data.
You will create an initial state file that will help determine the shape and structure of the state. In the folder of constants, create a file named initialState.js.
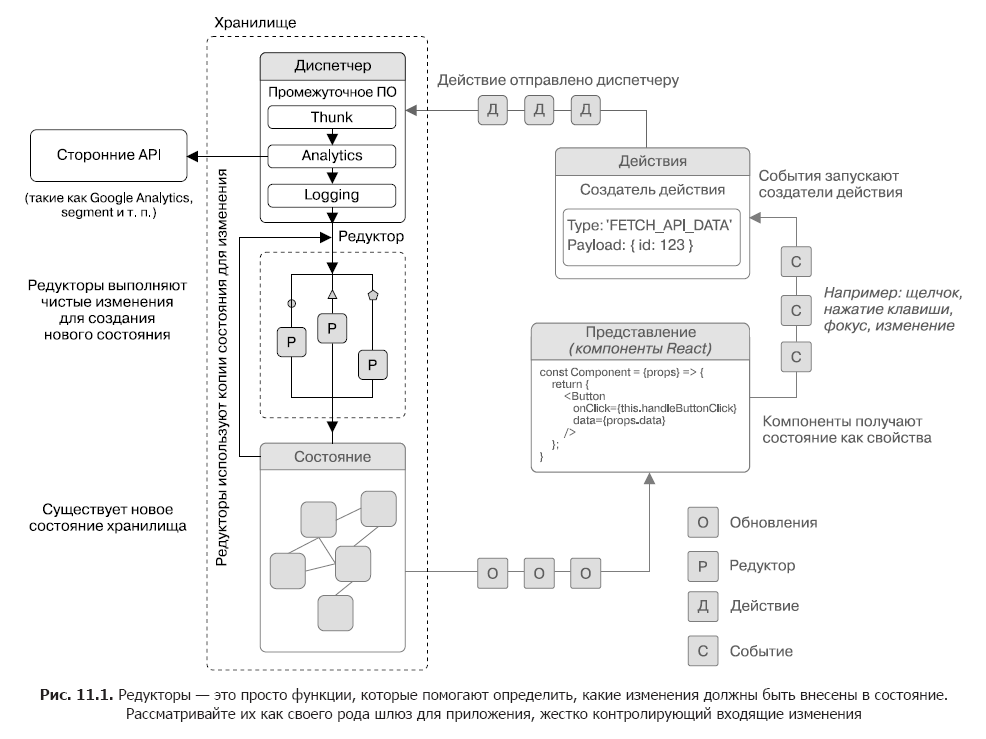
This is the state of the Redux application before any actions are submitted or changes are made. You will enter information about errors and download states, as well as some information about messages, comments and user. Store identifiers for comments and messages in arrays and basic information for them will become in objects that are easy to refer to. Listing 11.1 shows an example of setting the initial state.
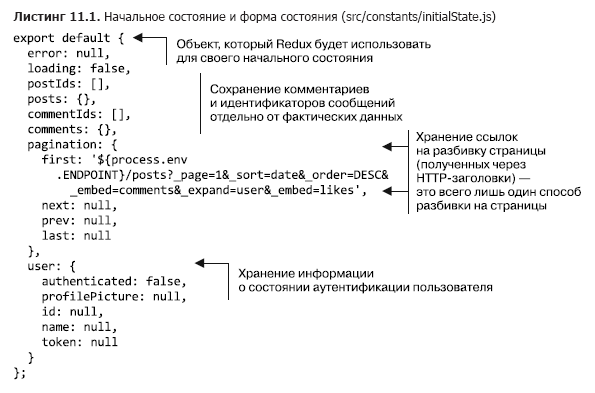
11.1.2. Configuring gearboxes to respond to incoming actions
When setting up the initial state, you must create several gearboxes to process incoming actions so that the vault can be updated. Gearboxes typically use a switch statement to update the state according to the type of inbound action. They return a new copy of the state (not the same version with the changes), which will then be used to update the repository. Gearboxes also operate on the “catch all” principle to ensure that unknown actions simply return an existing condition. I have already noted this, but it is important to say once again that gearboxes perform calculations and should return the same result each time based on the specified input - there should be no side effects or obscure processes.
Gearboxes are responsible for calculating how the storage should change. In most applications, you will have a lot of gearboxes, each of which is responsible for a part of the storage. This helps to keep files concise and focused. As a result, you will use the combineReducers method, available in Redux, to combine the gearboxes into one. Most gearboxes use a switch statement with cases for different types of actions and a default “catch all” command for everything else to ensure that unknown types of actions (probably created by chance, if any) will not affect the state.
Gearboxes also make copies of the state and do not directly change the existing state of the repository. If you look at pic. 11.1, you will see that the gearboxes use the state when performing their work. This approach is similar to how unchanged data structures usually work: instead of direct changes, modified copies are created. Listing 11.2 shows how to configure a boot reducer. Note that in this case you are dealing with a flat state slice, a boolean loading property, so you simply return true or false for the new state. You will often work with a state object that has many keys or nested properties, in which case the gear unit will need to do more than just return true or false.
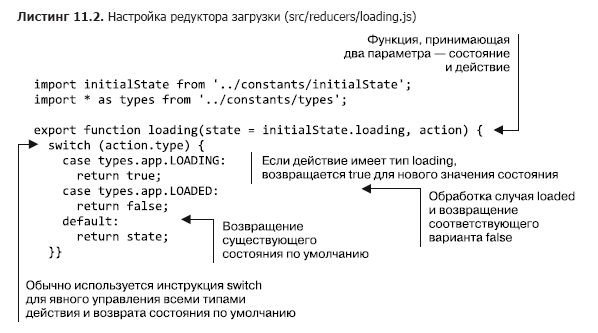
Now that the download action is sent, the Redux repository can do something with it. When an action enters and passes through any existing middleware, Redux uses gearboxes to determine which new state should be created based on the action. The repository had no way to know the information about the changes contained in the action before you configured any gearboxes. To show this, in fig. 11.2 gears removed from the flow; See why actions cannot reach the repository.
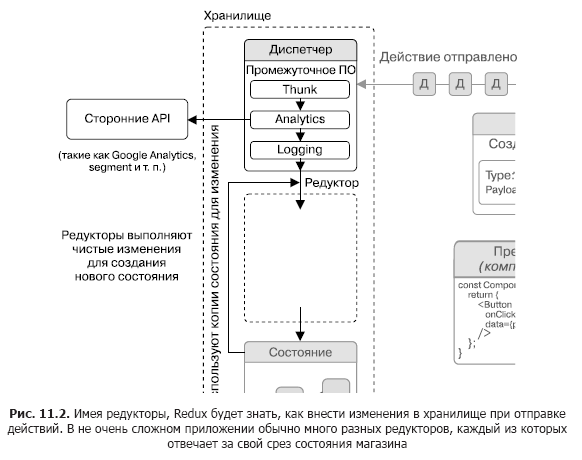
Then you will create another reducer to apply your Redux skills. In the end, many gearboxes will not simply return true or false. Or at least in the calculations it will be more than to give just true or false. Another key part of the Letters Social app shows and creates messages, and you need to transfer it to Redux. You must save most of the existing logic used by the application and translate it into a Redux-friendly form, as it would if you had adapted a real React application to use Redux. Create two gears to process the messages themselves and one to track message identifiers. In a larger application, you can combine them together under a different key, but now it’s good to keep them separate. This is also an example of how you can configure multiple gearboxes to handle a single action. Listing 11.3 shows how to write a reducer for comments. Here you will create a lot of gears, and as soon as this is done, the application will receive not only a detailed description of the events that may occur, but also ways to change the state.
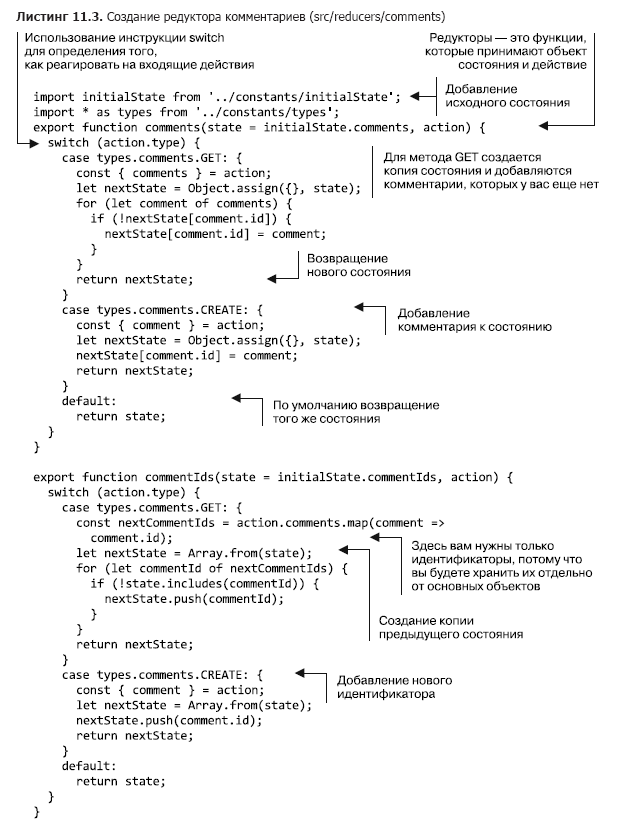
Now, when you submit actions related to comments, the status of the store will be updated accordingly. Have you noticed how you can respond to actions that are not strictly the same type? Gearboxes can respond to actions that are within their competence, even if they are not of the same type. This is possible because, despite the fact that the status message slice controls messages, there may be other actions that can affect it. Conclusion: the reducer is responsible for deciding how a specific status indicator should change, no matter what action or type of action comes. Some gearboxes may need to be aware of the many different types of actions that are not associated with the resource (messages) that they model.
Now, having created a comment reducer, you can execute one that will process messages. It will be very similar to the comments reducer, because it uses the same strategy of storing them separately, as identifiers and objects. He also needs to know how to handle messages with likes and without (you created actions for this functionality in Chapter 10). Listing 11.4 shows how to implement all this.
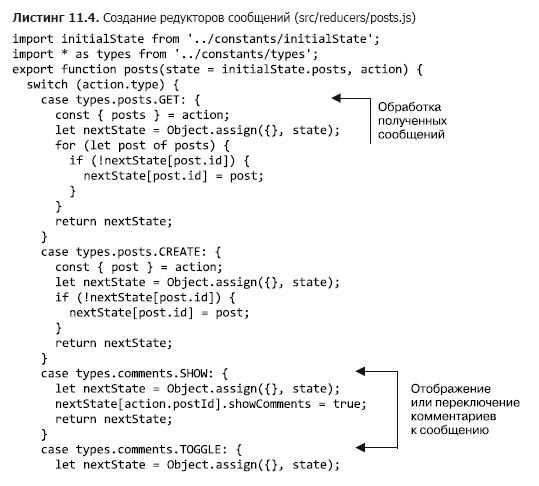

I (the author) included two gears in these files, because they are very closely related to each other and both act on the same fundamental data (messages and comments), but you probably want to use one gear to simplify the work in file. In most cases, the installation of your gearbox will reflect the structure of the storage or at least correspond to it. You may have noticed that the development of a repository state form (see the initial state that you set earlier in this chapter) greatly affects how gearboxes are defined and, to a lesser extent, actions. One conclusion from this is this: it is better to spend more time developing the form of the state than to give it a gloss. If too little time is allotted to the design, you may have to refine the state form for a long time to improve it,
Redux Migration: Is It Necessary?»More information about the book can be found on the publisher's website
I mentioned several times in this chapter that Redux may require a lot of work for the initial setup (you may have already felt this), but in the end it will bear fruit. This is especially true of the projects that I and the developers have worked on. One project in which I participated included the complete migration of the application from the Flux architecture to Redux. The whole team worked for about a month, but we were able to start rewriting the application, achieving minimal instability and reducing the number of errors to a minimum.
And the overall result was the possibility of faster iterative reproduction of the product using templates that Redux helped us put in the right place. A few months after the migration of Redux, we completed a series of complete reworkings of the application. Despite the fact that we redid a large part of the React application, the Redux architecture allowed us to make very few changes to the state management and business logic of the application. Moreover, the Redux templates have simplified making additions to the application state, where necessary. The integration of Redux has justified the work of setting up and translating the application to it and continues to bring dividends.
» Table of contents
» Excerpt
For Habrozhiteley 20% discount on the coupon - React