
Create a bot for Skype. Step by step, through the REST API and in Python
- Tutorial
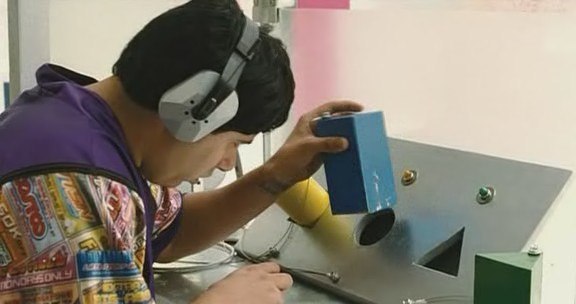
When looking for long-polling in the Skype API
A year ago, Microsoft introduced a platform for creating bots for Skype. The platform provides a convenient message format, you can send cards with buttons, as in a telegram, in a word, everything looks very cool.
Recently, I needed to write a bot for Skype. And despite the fact that the topic was being raised on a hub ( for example ), I ran into some difficulties, I really missed the step-by-step guide for working with the REST API.
In fact, the Skype bot is written quite quickly if you know about the pitfalls and know wheresee the documentation. The main idea that I had to learn: No web polling. If the Telegram to choose from provides long polling and webhooks, then Skype is only costed by webhooks. The following problems arise from this - communication with Skype servers occurs only via https, and only with a valid certificate. You have to find a domain name, hosting, bother with a certificate.
How to do it?
So, from the very beginning. Let's say that we have bare hosting (I had it Raspberry Pi, on which I tested the bot, but it can also be a server on Amazon, for example). First, you need to get a domain name and certificate for it. Free domain names can be obtained, for example, here . Next, we need to get a certificate for this name. This is easiest to do with Let's Encrypt . In the process of installing the certificate, select the standalone server option - certbot will launch its server to verify that you own this domain name before issuing a certificate for it. After you succeed, you should have certificates in the folder “/ etc / letsencrypt / archive”.
Now that everything is ready for work, we’ll start writing the bot.
1.Much has been described in this article , we need two points: Here we create our application, and here we register the bot. When registering, you need to specify the endpoint where Microsoft will send messages. It is indicated in the same form as written in the browser. After endpoint, you can specify a port, then it will look like this: “https: // endpoint_url: port” After registration, you will need to generate a password, and that’s all we needed to get at the moment. At the moment, we should have: application_id (with which we registered the bot) and password (which we just received).
2.You have not forgotten about webhooks? It is time to raise the server for which we previously received certificates. On python, a flask is suitable for this. First of all, we define global variables (not a very beautiful solution, but very simple), and begin to automatically receive and update the token in a separate stream:
View code
FLASK = Flask(__name__)
APP_ID = ''
PASSWORD = '' # секрет от бота
context =('fullchain.pem', 'privkey.pem') # относительные или абсолютные пути к файлам, которые сгенерировал certbot
TOKEN = {}
def get_token():
global TOKEN
url = 'https://login.microsoftonline.com/botframework.com/oauth2/v2.0/token'
payload = {'grant_type': 'client_credentials',
'client_id': APP_ID,
'client_secret': PASSWORD,
'scope': 'https://api.botframework.com/.default',
}
token = requests.post('https://login.microsoftonline.com/botframework.com/oauth2/v2.0/token', data=payload).content
TOKEN = json.loads(str(token)[2:-1])
return json.loads(str(token)[2:-1])
def send_token_to_connector(token):
url = 'https://groupme.botframework.com/v3/conversations'
headers = {'Authorization': 'Bearer ' + token}
r = requests.post(url, headers=headers)
return r
def get_and_verify_token():
global TOKEN
while True:
get_token()
send_token_to_connector(TOKEN['access_token'])
time.sleep(TOKEN['expires_in']*0.9)
3. We will write an inbound message handler function:
View code
@FLASK.route('/', methods=['GET', 'POST'])
def handle():
data = request.get_json()
talk_id = data['conversation']['id']
msg = {
"type": "message",
"from": {
"id": APP_ID,
"name": "habraechobot"
},
"conversation": {
"id": talk_id,
},
"text": data['text'],
}
url = data['serviceUrl'] + '/v3/conversations/{}/activities/'.format(data['conversation']['id'])
headers = {'Authorization': 'Bearer ' + TOKEN['access_token'],
'content-type': 'application/json; charset=utf8'}
r = requests.post(url, headers=headers, data=json.dumps(msg))
return 'success'
4. And finally, start the server:
View code
if __name__ == '__main__':
thread = Thread( target=get_and_verify_token )
thread.start()
FLASK.run(host='0.0.0.0', port=8080, ssl_context=context)
That's all - the full bot code can be taken from here , I hope my article was useful and will save someone time and effort.