Download, save and view PDF in Swift
- Transfer
- Tutorial
Good afternoon, I present to your attention the translation of the article on the work of PDF in Swift.
All interested, welcome under cat.
I am working on an application that should be able to download PDF files, save them and open them. For this task, you need to perform 3 steps:
Step 1. DownloadTask
To download a file via a URL, you need to use downloadTask. It is also necessary to track where our files have moved, so the observer, which in my case is a ViewController , must support URLSessionDownloadDelegate .
To understand where the file was saved, simply print the output of the location variable in the Xcode console .
After I clicked the “Download” button, the file was loaded in less than a second and then killed by the system. This behavior is repeated both on the simulator and on the real device.
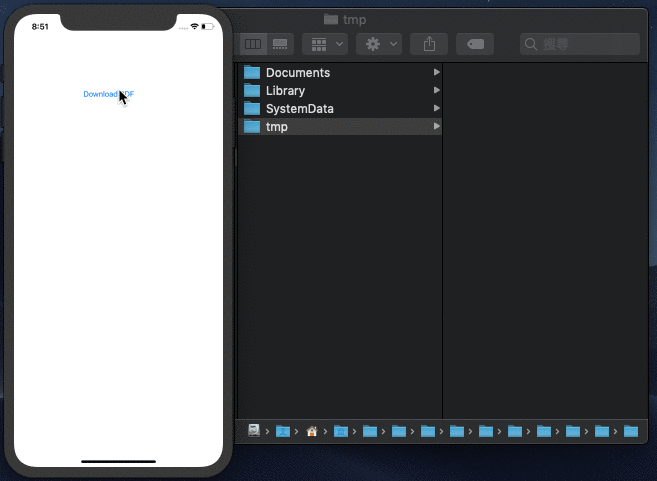
Step 2. File Management
Each application in iOS has its own test environment. Inside it there are 3 components that every iOS developer should know: Bundle Container, Data Container, and iCloud Container. In this article we will take a closer look at only Data Container, since we will need it for our task - to download a PDF file.
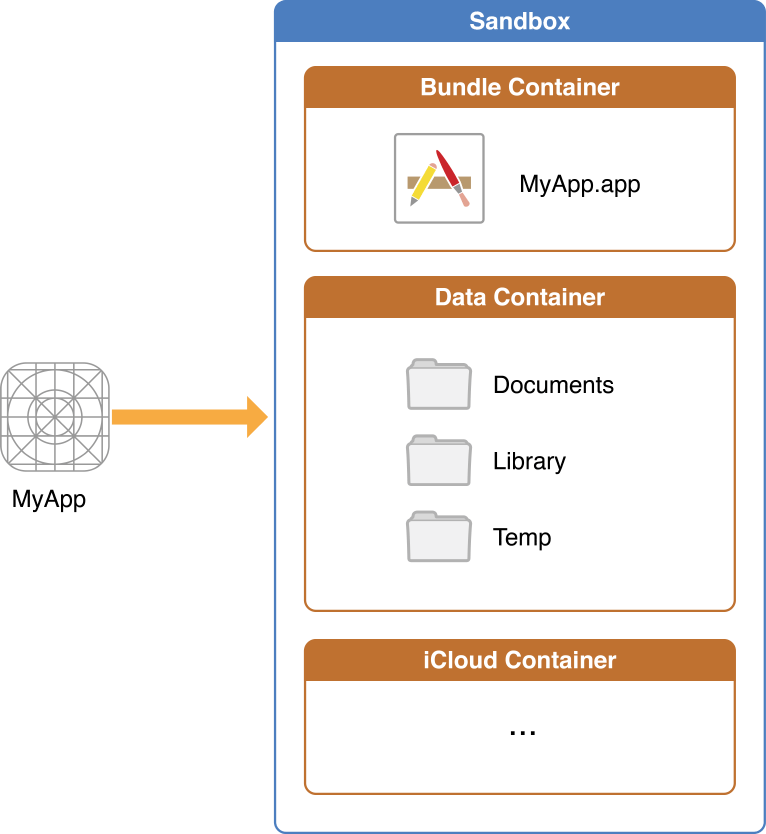
With the help of Data container we can manage the files saved from the Internet. Below I will list the important properties:
It follows that our next step will be to save the file from tmp to Documents. Here is what I did:

Step 3. PDFView
So, we have moved the PDF file, and now the user has access to it. Let's now figure out how to open it using PDFView, embedded in PDFKit — Apple's convenient framework available from iOS 11.
Although many PDFKit tutorials use storyboard to create PDFView using the UIView property, this cannot be done through Xibs . Therefore, I will create it through code.
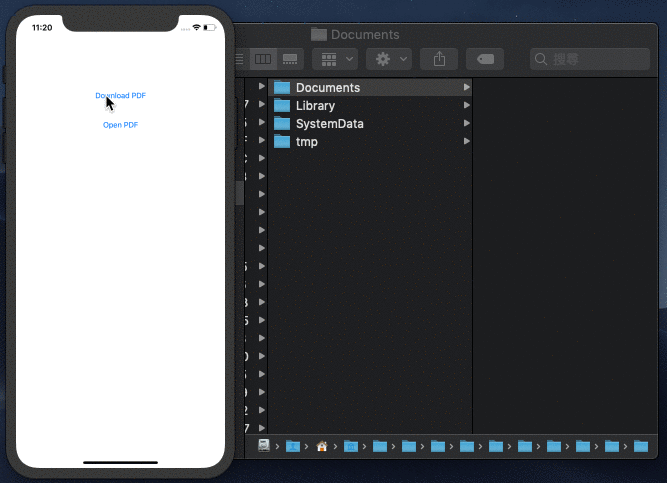
Voila! We extracted and opened the PDF file. I know that the format of the book is a little different, which is why we will take a closer look at PDFKit in the next article .
All interested, welcome under cat.
I am working on an application that should be able to download PDF files, save them and open them. For this task, you need to perform 3 steps:
- DownloadTask
- File management
- PDFView
Step 1. DownloadTask
To download a file via a URL, you need to use downloadTask. It is also necessary to track where our files have moved, so the observer, which in my case is a ViewController , must support URLSessionDownloadDelegate .
import UIKitclass ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
}
@IBAction func downloadButtonPressed(_ sender: Any) {
guard let url = URL(string: "https://www.tutorialspoint.com/swift/swift_tutorial.pdf") else { return }
let urlSession = URLSession(configuration: .default, delegate: self, delegateQueue: OperationQueue())
let downloadTask = urlSession.downloadTask(with: url)
downloadTask.resume()
}
}
extension ViewController: URLSessionDownloadDelegate {
func urlSession(_ session: URLSession, downloadTask: URLSessionDownloadTask, didFinishDownloadingTo location: URL) {
print("downloadLocation:", location)
}
}
To understand where the file was saved, simply print the output of the location variable in the Xcode console .
After I clicked the “Download” button, the file was loaded in less than a second and then killed by the system. This behavior is repeated both on the simulator and on the real device.
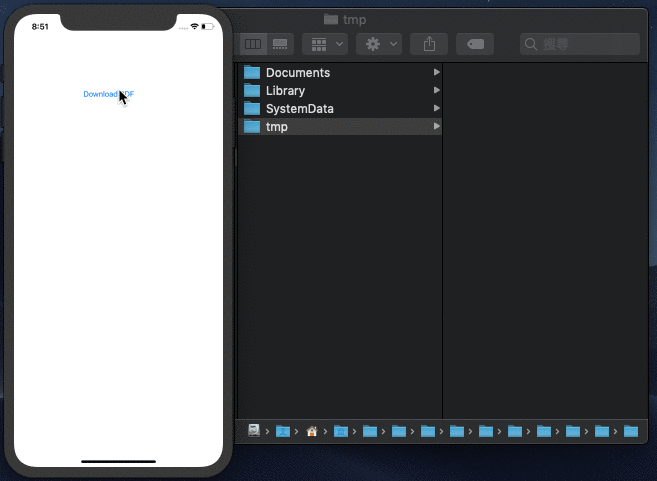
Step 2. File Management
Each application in iOS has its own test environment. Inside it there are 3 components that every iOS developer should know: Bundle Container, Data Container, and iCloud Container. In this article we will take a closer look at only Data Container, since we will need it for our task - to download a PDF file.
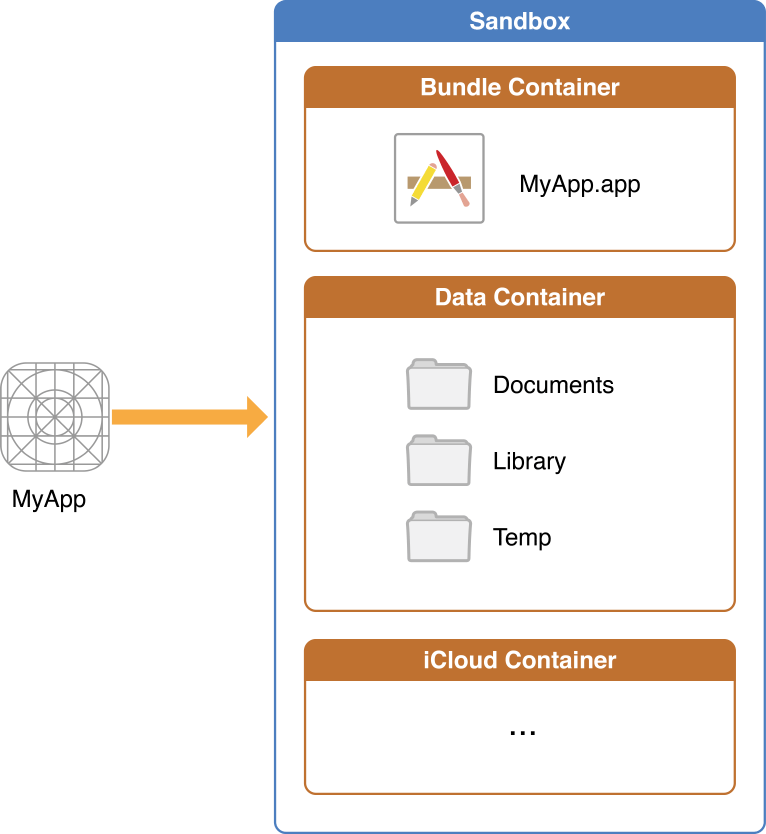
With the help of Data container we can manage the files saved from the Internet. Below I will list the important properties:
- Files inside the Library as well as tmp files will be automatically deleted.
- iTunes backs up all files except Caches, tmp and files marked as .isExcludedFromBackup = true . During App Review, if Apple finds in iTines saved files that should not be there, the application is likely to be rejected.
- Saved files should be stored in Documents .
It follows that our next step will be to save the file from tmp to Documents. Here is what I did:
- Copied the name of the pdf file
- Created url in Documents
- Deleted a file with the same name to avoid copying errors: “CFNetworkDownload_mdrFNb.tmp” couldn't be copied to “Documents”.
- Copy the file to Documents.
extension ViewController: URLSessionDownloadDelegate {
func urlSession(_ session: URLSession, downloadTask: URLSessionDownloadTask, didFinishDownloadingTo location: URL) {
print("downloadLocation:", location)
// create destination URL with the original pdf name
guard let url = downloadTask.originalRequest?.url else { return }
let documentsPath = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask)[0]
let destinationURL = documentsPath.appendingPathComponent(url.lastPathComponent)
// delete original copy
try? FileManager.default.removeItem(at: destinationURL)
// copy from temp to Documentdo {
try FileManager.default.copyItem(at: location, to: destinationURL)
self.pdfURL = destinationURL
} catch let error {
print("Copy Error: \(error.localizedDescription)")
}
}
}

Step 3. PDFView
So, we have moved the PDF file, and now the user has access to it. Let's now figure out how to open it using PDFView, embedded in PDFKit — Apple's convenient framework available from iOS 11.
Although many PDFKit tutorials use storyboard to create PDFView using the UIView property, this cannot be done through Xibs . Therefore, I will create it through code.
@IBAction func openPDFButtonPressed(_ sender: Any) {
let pdfViewController = PDFViewController()
pdfViewController.pdfURL = self.pdfURL
present(pdfViewController, animated: false, completion: nil)
}
import UIKit
import PDFKit
class PDFViewController: UIViewController {
var pdfView = PDFView()
var pdfURL: URL!
override func viewDidLoad() {
super.viewDidLoad()
view.addSubview(pdfView)
if let document = PDFDocument(url: pdfURL) {
pdfView.document = document
}
DispatchQueue.main.asyncAfter(deadline: .now()+3) {
self.dismiss(animated: true, completion: nil)
}
}
override func viewDidLayoutSubviews() {
pdfView.frame = view.frame
}
}
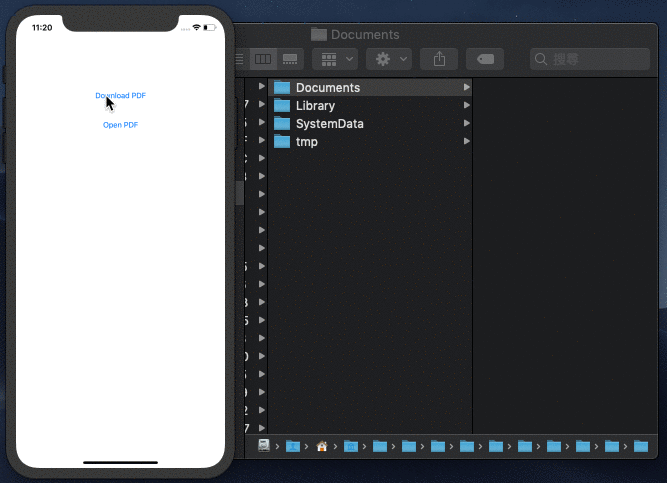
Voila! We extracted and opened the PDF file. I know that the format of the book is a little different, which is why we will take a closer look at PDFKit in the next article .