
6 things I would like to know before developing my first Android application
- Transfer
From the translator : the translation was done right away, in one sitting, so in some places it can be a little clumsy. In any case, you know where to write about errors.
In the comments to the original article, there are several common thoughts, such as not using libraries from the world of "big" Java, since they are too voluminous, or using all sorts of Glides instead of Picasso (which I agree, however). You can see if interested.
And yet, I did not figure out how to make snippets from the github appear, so I just copied the code.
My first application was terrible. In fact, it was so terrible that I deleted it from the market and will not even mention it in my resume anymore. That application might not be so terrible if I knew a few things about Android development before writing it.
The following is a list of things to understand when developing your first Android application. These lessons were learned from the real mistakes that I made in the source code of my first program, a mistake that I will cite below. Understanding these things will help you write an application that you will be proud of a little more.
Of course, if you write something while studying Android development, most likely, later you will hate your creation anyway. As @codestandards said:
(If the code you wrote a year ago doesn’t seem bad to you, you probably haven’t studied enough)
If you are an experienced Java developer, steps 1, 2, and 5 may not be of interest to you. On the other hand, points 3 and 4 can show you some cool things that you can do with Android Studio that you have not heard about, even if you did not make the mistakes in the examples for these points.
It may look like a mistake that no one would have thought of making. This is not true. I thought of it. I saw people who made the same mistake, and I interviewed people who could not immediately explain why this is a serious mistake. Do not do so. This is Nubian.
If MeTrackerStore stores a reference to an Activity passed to the constructor, this Activity will never be destroyed by the garbage collector (until this static link is reassigned to another Activity). This is because mMeTrackerStore is static, and memory for static variables is not freed until the process in which the application is running is completed. If you are tempted to do so, apparently something is seriously wrong with your code. Seek help. Maybe Google’s course on Udacity will help you: " Android Development for Beginners".
Note: technically, you can store a link to the application context without causing a memory leak, but I still do not recommend you to do this .
There are several problems with this code. I will focus on one. In Java, (non-static) inner classes have an implicit reference to an instance of the class that contains them.
In this example, any GetLatAndLongAndUpdateMapCameraAsyncTask would have a link to the DefineGeofenceFragment that contains it. The same is true for anonymous classes.
GetLatAndLongAndUpdateMapCameraAsyncTask has an implicit reference to a fragment, an object, than we do not control the life cycle. The responsibility for creating and destroying fragments lies with the Android SDK, and if GetLatAndLongAndUpdateCameraAsyncTask cannot be cleaned by the collector because it is still executing, its implicit reference to DefineGeofenceFragment will prevent the collector from deleting the fragment as well.
Here is a great Google I / O video whereexplains why such things happen .
This snippet contains what you get when using “Generate Getter” in Android Studio. The getter leaves the prefix “m” in the name of the generated method. This is not the best option.
(If you are more worried about the prefix “m” being added to the instance variable: “m” is often added according to code-convention. It stands for “member”)
Regardless of whether you think adding a prefix is a good idea, you should know that Android Studio Allows you to write code in accordance with any convention that you follow. For example, you can use the code style settings to tell Android Studio to automatically add a prefix to your instance variables and delete it when generating getters, setters, and constructor parameters.

In general, Android Studio can do a lot more.Learning about shortcuts and templates is where you can start.
In one of the classes I wrote, there was a method longer than 100 lines. Such methods are difficult to read, modify, and reuse. Try writing methods that do just one thing. As a rule, all methods longer than 20 lines should be suspected. In search of such methods, you can ask for help from Android Studio.
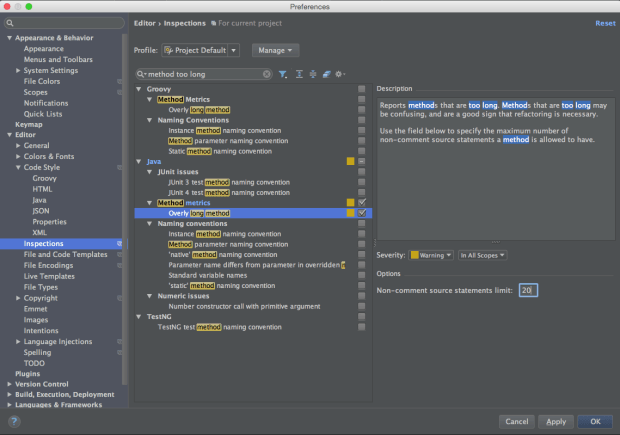
It may sound trivial, but I made this mistake when writing the first application.
When you create programs, you will be wrong. Other people have already made these mistakes. Learn from these people. By repeating problems solved by others, you are wasting your time. I spent a lot of time on my first application, making mistakes that I could have avoided if I had just spent a little more time learning from experienced developers.
Read the Pragmatic Programmer . Then Effective Java . These two books will help you avoid the basic mistakes that beginners make. When you're done with these books, keep looking for smart people to learn from.
When you write your application, you are likely to run into problems that more intelligent and experienced people have already solved. Moreover, many of these solutions are available as open source libraries. Take advantage of them.
In my first application, I wrote code that provided the functionality already provided by libraries. Some of the libraries were standard. Others are third-party, such as Retrofit and Picasso. If you are not sure which libraries to use, you should do the following three things:
Writing good Android programs can be very difficult. Do not complicate your life even more by repeating my mistakes. If you find a mistake in what I wrote, report it in the comments (erroneous comments are no worse than their absence). If you think this will be useful for some newbie, share an article with him. Save them from some headache.
In the comments to the original article, there are several common thoughts, such as not using libraries from the world of "big" Java, since they are too voluminous, or using all sorts of Glides instead of Picasso (which I agree, however). You can see if interested.
And yet, I did not figure out how to make snippets from the github appear, so I just copied the code.
My first application was terrible. In fact, it was so terrible that I deleted it from the market and will not even mention it in my resume anymore. That application might not be so terrible if I knew a few things about Android development before writing it.
The following is a list of things to understand when developing your first Android application. These lessons were learned from the real mistakes that I made in the source code of my first program, a mistake that I will cite below. Understanding these things will help you write an application that you will be proud of a little more.
Of course, if you write something while studying Android development, most likely, later you will hate your creation anyway. As @codestandards said:
If the code you wrote a year ago doesn't seem bad to you, you're probably not learning enough.— Code Standards (@codestandards) May 21, 2015
(If the code you wrote a year ago doesn’t seem bad to you, you probably haven’t studied enough)
If you are an experienced Java developer, steps 1, 2, and 5 may not be of interest to you. On the other hand, points 3 and 4 can show you some cool things that you can do with Android Studio that you have not heard about, even if you did not make the mistakes in the examples for these points.
1. Do not create static context references
public class MainActivity extends LocationManagingActivity implements ActionBar.OnNavigationListener,
GooglePlayServicesClient.ConnectionCallbacks,
GooglePlayServicesClient.OnConnectionFailedListener {
//...
private static MeTrackerStore mMeTrackerStore;
//...
@Override
protected void onCreate(Bundle savedInstanceState) {
//...
mMeTrackerStore = new MeTrackerStore(this);
}
}
It may look like a mistake that no one would have thought of making. This is not true. I thought of it. I saw people who made the same mistake, and I interviewed people who could not immediately explain why this is a serious mistake. Do not do so. This is Nubian.
If MeTrackerStore stores a reference to an Activity passed to the constructor, this Activity will never be destroyed by the garbage collector (until this static link is reassigned to another Activity). This is because mMeTrackerStore is static, and memory for static variables is not freed until the process in which the application is running is completed. If you are tempted to do so, apparently something is seriously wrong with your code. Seek help. Maybe Google’s course on Udacity will help you: " Android Development for Beginners".
Note: technically, you can store a link to the application context without causing a memory leak, but I still do not recommend you to do this .
2. Beware of “implicit references” to objects whose life cycle you do not control
public class DefineGeofenceFragment extends Fragment {
public class GetLatAndLongAndUpdateMapCameraAsyncTask extends AsyncTask {
@Override
protected LatLng doInBackground(String... params) {
//...
try {
//Here we make the http request for the place search suggestions
httpResponse = httpClient.execute(httpPost);
HttpEntity entity = httpResponse.getEntity();
inputStream = entity.getContent();
//..
}
}
}
}
There are several problems with this code. I will focus on one. In Java, (non-static) inner classes have an implicit reference to an instance of the class that contains them.
In this example, any GetLatAndLongAndUpdateMapCameraAsyncTask would have a link to the DefineGeofenceFragment that contains it. The same is true for anonymous classes.
GetLatAndLongAndUpdateMapCameraAsyncTask has an implicit reference to a fragment, an object, than we do not control the life cycle. The responsibility for creating and destroying fragments lies with the Android SDK, and if GetLatAndLongAndUpdateCameraAsyncTask cannot be cleaned by the collector because it is still executing, its implicit reference to DefineGeofenceFragment will prevent the collector from deleting the fragment as well.
Here is a great Google I / O video whereexplains why such things happen .
3. Make Android Studio work for you
public ViewPager getmViewPager() {
return mViewPager;
}
This snippet contains what you get when using “Generate Getter” in Android Studio. The getter leaves the prefix “m” in the name of the generated method. This is not the best option.
(If you are more worried about the prefix “m” being added to the instance variable: “m” is often added according to code-convention. It stands for “member”)
Regardless of whether you think adding a prefix is a good idea, you should know that Android Studio Allows you to write code in accordance with any convention that you follow. For example, you can use the code style settings to tell Android Studio to automatically add a prefix to your instance variables and delete it when generating getters, setters, and constructor parameters.

In general, Android Studio can do a lot more.Learning about shortcuts and templates is where you can start.
4. Methods should do one thing
In one of the classes I wrote, there was a method longer than 100 lines. Such methods are difficult to read, modify, and reuse. Try writing methods that do just one thing. As a rule, all methods longer than 20 lines should be suspected. In search of such methods, you can ask for help from Android Studio.
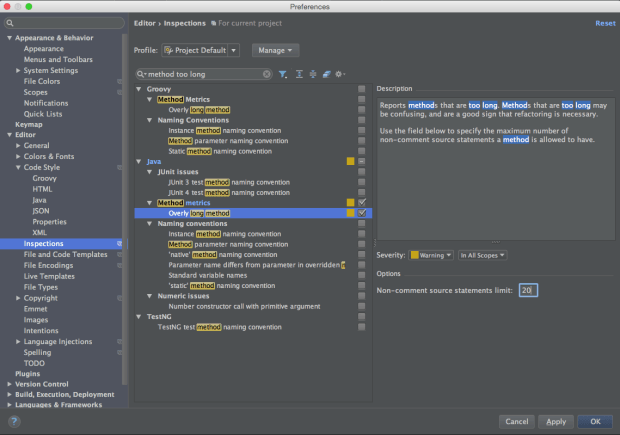
5. Learn from people who are smarter and more experienced than you
It may sound trivial, but I made this mistake when writing the first application.
When you create programs, you will be wrong. Other people have already made these mistakes. Learn from these people. By repeating problems solved by others, you are wasting your time. I spent a lot of time on my first application, making mistakes that I could have avoided if I had just spent a little more time learning from experienced developers.
Read the Pragmatic Programmer . Then Effective Java . These two books will help you avoid the basic mistakes that beginners make. When you're done with these books, keep looking for smart people to learn from.
6. Use libraries
When you write your application, you are likely to run into problems that more intelligent and experienced people have already solved. Moreover, many of these solutions are available as open source libraries. Take advantage of them.
In my first application, I wrote code that provided the functionality already provided by libraries. Some of the libraries were standard. Others are third-party, such as Retrofit and Picasso. If you are not sure which libraries to use, you should do the following three things:
- Listen to the Google I / O Fragmented podcast series . In this series, developers are asked which third-party libraries they consider important when developing for Android. Spoiler: Mostly it's Dagger, Retrofit, Picasso, and Mockito.
- Sign up for Android Weekly . They have a section containing the latest libraries that have appeared. Follow him, there you can find something useful.
- Look for open source applications that solve problems similar to those you encounter in your application. There you can see that the developer used a third-party library that you did not find, or a standard Java library that you did not know about.
Conclusion
Writing good Android programs can be very difficult. Do not complicate your life even more by repeating my mistakes. If you find a mistake in what I wrote, report it in the comments (erroneous comments are no worse than their absence). If you think this will be useful for some newbie, share an article with him. Save them from some headache.