
ES6 and beyond. Chapter 1: ES? Present and Future
- Transfer
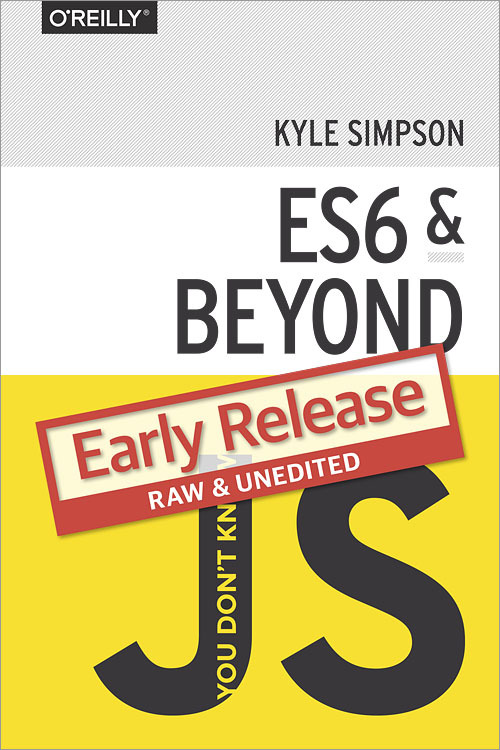
I want to pay attention to the book that Kyle Simpson wrote - "ES6 and Beyond" (Eng. "ES6 & Beyond"). Of course, not only he contributed, but many wonderful contributors. Acknowledgments .
At the moment it has a status - a completed draft.
Table of contents:
- Chapter 1: ES? Present & Future
- Chapter 2: Syntax
- Chapter 3: Organization
- Chapter 4: Asynchronous Flow Control
- Chapter 5: Collections
- Chapter 6: Add-ons API
- Chapter 7: Meta Programming
- Chapter 8: Beyond ES6
Before you start reading this book, I believe that you already have a solid knowledge of JavaScript right up to the most popular standard (at the time of writing this book), which is usually called ES5 (technically it is ES5.1). Here we will talk about the upcoming ES6 standard and try to understand the further development of JS.
ES6 is not just a modest set of new APIs added to the language, as it was with ES5. It includes a lot of new syntactic forms, the understanding of which may take some time before they become a habit. Several new organizational forms and a new API with many helpers for existing data types have also appeared.
ES6 is a radical leap forward. Even if you think you know JS (ES5), ES6 is full of new things that you still have to learn about. So get ready! This book will cover all the main topics of ES6, which will help you quickly get used to it and even more - look into the future to be ready for new changes.
Note: all the code in this book requires the ES6 environment.
Versioning
JavaScript is officially called "ECMAScript" (abbreviation "ES") and until recently, the version was an integer (that is, "5", "5th edition").
Earlier versions of ES1 and ES2 were not widely implemented, but ES3 was the first widely accepted JavaScript framework. ES3 is the JavaScript standard for browsers like IE6-8 and older Android 2.x mobile browsers. ES4 for "political" reasons, we will not consider. This standard has been dropped.
In 2009, ES5 was officially completed (later ES5.1 in 2011), and became the widespread standard for a whole bunch of browsers like Firefox, Chrome, Opera, Safari and many more.
Waiting for the next version of JS (in 2013, then in 2014, and then in 2015), the most talked about new label was ES6.
However, later in the ES6 specification tape, suggestions surfaced that the versioning model should have an annual mark at the bottom. For example, ES2016 (ES7), meaning that the standard was completed at the end of 2016. Many disagree with this, and in any case, renaming ES6 is too late. But as a fact, a similar versioned model can be.
Also, thanks to simple observation, we can say that the evolution of JS is proceeding faster than the annual versioning. As soon as the idea for a new standard begins to progress in discussions, browsers begin to prototype features (functions, APIs) and adapt them to code experiments before (we all know experimental flags).
Usually, before an official approval stamp is put, the de facto language features go through the standardization stage due to early prototyping. Therefore, it is worth talking about the future JS versioning model which will be based on new pre-functions rather than on the annual model or the model of the pre-annual-collection of basic functions that is currently being used.
I will introduce that version labels like ES6 are no longer important, and JavaScript should be a kind of evergreen living standard. The best way to overcome tags is to stop thinking that your code is dependent on an ES6 version in favor of a feature-by-feature for further support.
Transpiling
For developers who want to use the new features of the language, there is a problem of supporting applications / sites in older browsers that do not support them.
The main thinking was to expect adoption of ES5 until most or even all environments fall out of the support spectrum. As a result, many recently just started to implement things on their own, such as, for example, strict mode, which took root in ES5 many years ago.
Many would agree that such an approach of waiting and trampling down specifications is detrimental to the JS ecosystem. Those people who are responsible for the development of the language want the developers to use the new features of the language and its patterns as soon as they are stabilized in the specification; browsers have a chance to implement them.
So how do we solve this small contradiction? The answer is tuning, namely, this technique is called transpiling (transformation + compilation).
For example, consider the abbreviated declaration of a parameter (Object literals will be discussed in Chapter 2). Here's what it looks like in ES6:
var foo = [1,2,3];
var obj = {
foo // ввиду имеется `foo: foo`
};
obj.foo; // [1,2,3]
And here is how it will look after transpiling:
var foo = [1,2,3];
var obj = {
foo: foo
};
obj.foo; // [1,2,3]
This is not a significant, but nice transformation that allows us to shorten foo: foo in the description of an object literal. Using only foo if the names are the same.
Transpiling usually takes a place on a par with linting, minification, etc.
Shima / Polyphiles
Not all new ES6 features require transpiling. Polyphils (shims) are such a pattern that determines the equivalent behavior of the new environment in the older one, of course, when it is possible. The syntax cannot be polyphilic, but the API can.
For example, Object.is (..) is a new utility for checking the strict equivalence of two values, but without exceptions, which === has for values NaN and -0. The polyphil of the Object.is (..) method is quite simple:
if (!Object.is) {
Object.is = function(v1, v2) {
// test for `-0`
if (v1 === 0 && v2 === 0) {
return 1 / v1 === 1 / v2;
}
// тест на значение `NaN`
if (v1 !== v1) {
return v2 !== v2;
}
// что либо другое
return v1 === v2;
};
}
Pay attention to the outer wrapper around the polyphile - if. This is an important detail, which means that the snippet is defined only for the old environment where this API has not yet been implemented. Rarely enough will you have to override existing APIs.
There is a great collection of ES6 shims called ES6 Shims , which you can use as part of your new project.
Obviously, JS will invariably evolve with browsers that will roll out new features gradually, rather than in large chunks. Therefore, the best strategy to keep up with the updates and evolution of the language is to embed polyphiles in your base code and add transpiling to the development process and thereby get used to the new reality.
If you decide to stick to the status quo and just wait for all browsers to fully support the language, you'll be far behind. You run the risk of skipping those innovations that were invented in order to write JavaScript more efficiently and reliably.
Review
It’s important to set your thinking in a new way that JavaScript will evolve. Do not wait for years until the new standard is approved. At the moment, new JavaScript features are being implemented in browsers as far as possible, whether you are ready to use them now or wait - it is up to you.
No matter what tags future JavaScript will receive, the development of the language will be much faster than it was before. Transpiling and polyphiles are important tools to help you keep up with the development and emergence of new opportunities.