
Konva.js - HTML5 2d canvas framework
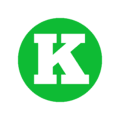
Greetings. Introducing the Konva.js project to the community .
Konva.js is a framework that allows you to work with canvas 2d in an object style with event support.
A brief list of features looks like this:
- Object API
- Nested objects and event bubbling
- Support for multiple layers (multiple canvas elements)
- Object Caching
- Animation Support
- Custom drag & drop
- Filters
- Ready-to-use objects including rectangle, circle, image, text, line, SVG path, ..
- Easily create your own shapes.
- An event architecture that allows developers to subscribe to attribute change events, rendering, and so on
- Serialization and Deserialization
- Advanced search with stage.get ('# foo') and layer.get ('. Bar') selectors
- Desktop and Mobile Events
- Built-in support for HDPI devices
- and much more
Next, we’ll take a closer look at the possibilities of a framework with code examples.
Introduction
It all starts with Stage, which combines custom layers (Layer).
Each layer (Layer) is one canvas element on the page and can contain figures, groups of figures or groups of groups.
Each element can be stylized and transformed.
As soon as you set up Stage and layers, add shapes, you can subscribe to events, change the properties of elements, start animation, create filters.
Minimum Code Example [Result] :
// сначала создаём контейнер
var stage = new Konva.Stage({
container: 'container', // индификатор div контейнера
width: 500,
height: 500
});
// далее создаём слой
var layer = new Konva.Layer();
// создаём фигуру
var circle = new Konva.Circle({
x: stage.width() / 2,
y: stage.height() / 2,
radius: 70,
fill: 'red',
stroke: 'black',
strokeWidth: 4
});
// добавляем круг на слой
layer.add(circle);
// добавляем слой
stage.add(layer);
Base shapes
Konva.js supports the following shapes: rectangle (Rect), circle (Circle), oval (Ellipse), line (Line), image (Image), text (Text), text path (TextPath), star (Star), label ( Label), svg path (Path), regular polygon (RegularPolygon). You can also create your own shape :
var triangle = new Konva.Shape({
drawFunc: function(context) {
context.beginPath();
context.moveTo(20, 50);
context.lineTo(220, 80);
context.quadraticCurveTo(150, 100, 260, 170);
context.closePath();
// специальный метод KonvaJS
context.fillStrokeShape(this);
},
fill: '#00D2FF',
stroke: 'black',
strokeWidth: 4
});
Styles
Each shape supports the following style properties:
- Fill - Solid color, gradients and images are supported.
- Outline (stroke, strokeWidth)
- Shadow (shadowColor, shadowOffset, shadowOpacity, shadowBlur)
- Transparency
var pentagon = new Konva.RegularPolygon({
x: stage.getWidth() / 2,
y: stage.getHeight() / 2,
sides: 5,
radius: 70,
fill: 'red',
stroke: 'black',
strokeWidth: 4,
shadowOffsetX : 20,
shadowOffsetY : 25,
shadowBlur : 40,
opacity : 0.5
});
Result:
Events
Using Konva.js, you can easily subscribe to input events (click, dblclick, mouseover, tap, dbltap, touchstart, etc.), attribute change events (scaleXChange, fillChange), and drag & drop events (dragstart, dragmove, dragend) .
circle.on('mouseout touchend', function() {
console.log('user input');
});
circle.on('xChange', function() {
console.log('position change');
});
circle.on('dragend', function() {
console.log('drag stopped');
});
Work code + demo
DRAG AND DROP
The framework has built-in drag support. At the moment, there is no support for drop events (drop, dragenter, dragleave, dragover), but they are quite easily implemented using the framework .
To drag an element, just set the properties draggable = true.
shape.draggable('true');
At the same time, you will be able to subscribe to drag & drop events and configure movement restrictions. [Demo] .
Filters
Konva.js includes many filters: blur, invert, sepia, noise and so on. A complete list of available filters can be found in the filter documentation API .
Filter usage example:
image.cache();
image.filters([Konva.Filters.Invert]);
Animation
There are two ways to create animations:
1. Through the “Animation” object:
var anim = new Konva.Animation(function(frame) {
var time = frame.time,
timeDiff = frame.timeDiff,
frameRate = frame.frameRate;
// update stuff
}, layer);
anim.start();
[Demo]
2. Via the Tween Object:
var tween = new Konva.Tween({
node: rect,
duration: 1,
x: 140,
rotation: Math.PI * 2,
opacity: 1,
strokeWidth: 6
});
tween.play();
[Demo]
Selectors
When building a large application, it is extremely convenient to use the search on the created elements. Konva.js allows you to search for objects using selectors using the find methods (returns the collection) and findOne (returns the first element of the collection):
var circle = new Konva.Circle({
radius: 10,
fill: 'red',
id : 'face',
name : 'red circle'
});
layer.add(circle);
// далее производим поиск
// поиск по типу фигуры
layer.find('Circle'); // все круги
// поиск по id
layer.findOne('#face');
// поиск по имени (аналогия с css классами)
layer.find('.red')
Serialization and Deserialization
You can save all created objects in JSON format, for example, to save to a server or local storage:
var json = stage.toJSON();
And also create elements from JSON:
var json = '{"attrs":{"width":578,"height":200},"className":"Stage","children":[{"attrs":{},"className":"Layer","children":[{"attrs":{"x":100,"y":100,"sides":6,"radius":70,"fill":"red","stroke":"black","strokeWidth":4},"className":"RegularPolygon"}]}]}';
var stage = Konva.Node.create(json, 'container');
Performance
Konva.js has many tools to significantly increase productivity.
1. Caching allows you to draw some element into a buffered canvas and then draw it from there. This can significantly improve the rendering performance of complex objects such as text or objects with shadows and outlines.
shape.cache();
[Demo]
2. Work with layers. Since the framework supports several canvas elements, you can distribute objects at your discretion. Suppose an application consists of a complex background with a text and several movable shapes. It will be logical to transfer the background and text to one layer, and the shapes to another. In this case, when updating the position of the figures, the background layer can not be redrawn.
[Demo]
A more detailed list of performance tips is available here: http://konvajs.github.io/docs/performance/All_Performance_Tips.html
Conclusion
GitHub: https://github.com/konvajs/konva
Homepage: http://konvajs.github.io/
Documentation with examples: http://konvajs.github.io/docs/
Full API: http: // konvajs .github.io / api / Konva.html
This project is a fork of the famous KineticJS library , which is no longer supported by the author.
A list of changes from the latest official version of KineticJS can be seen here .