React Training Course, Part 15: Workshop on Working with Component State
- Transfer
- Tutorial
Today we offer you to perform two practical classes on working with the state of the components. In particular, by completing today's tasks, you can not only better understand the concept of properties, but also work on debugging React-applications in which there are errors.
→ Part 1: course overview, reasons for the popularity of React, ReactDOM and JSX
→ Part 2: functional components
→ Part 3: component files, project structure
→ Part 4: parent and child components
→ Part 5: starting work on a TODO application, basics of styling
→ Part 6: About some features of the course, JSX and JavaScript
→ Part 7: Inline Styles
→
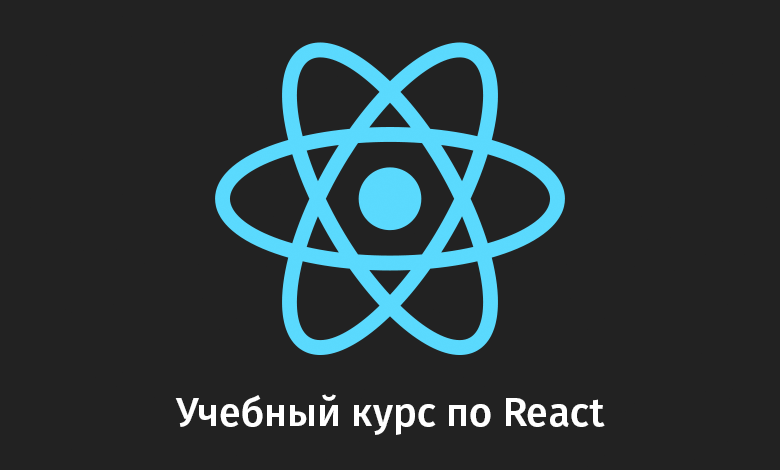
Part 8: continued work on a TODO application, familiarity with the properties of components
→ Part 9: properties of components
→ Part 10: a workshop on working with properties of components and styling
→ Part 11: dynamic formation of markup and the array method map
→ Part 12: workshop, third stage of working on a TODO application
→ Part 13: components based on classes
→ Part 14: a workshop on components based on classes, the state of components
→ Part 15: workshops on working with the state of components
→ Original
Analyze the following class code
A component
Your task is to bring this code into working condition.
If you come across some unknown error message - do not rush to look into the solution. Try it yourself, for example, carefully read the code and look for information about the problem on the Internet, find out the cause of the error and fix it. Debugging code is a valuable skill that will definitely come in handy when working on real projects.
The body of a class is similar to the body of a functional component. It has only a command
If you continue the analysis of the code, looking at the error messages displayed in the browser, you can understand that although the design
True, this problem does not end there. The application does not work, an error message appears in the browser
The point here is that when declaring a component, which, as we already understood, is a component that is based on a class, after the name of the parent class there is a pair of parentheses. They are not needed here, this is not a functional component, so we will get rid of them. Now the application code is more or less efficient, the component based on the class no longer looks completely wrong, but the system continues to report errors to us. Now the error message looks like this:
Here is the finished working component code
This is how the application page will look like in a browser.

Application page in the browser
→ Original
In this practice session, you will have another chance to work with the state of the components.
Below is the code of the functional component. Taking it as a basis, do the following:
Here is the file code
We have a functional component at our disposal. In order to equip it with a state, it must be converted into a component based on the class and initialized with its state. Here is the code for the converted component:
Check the performance of the application.
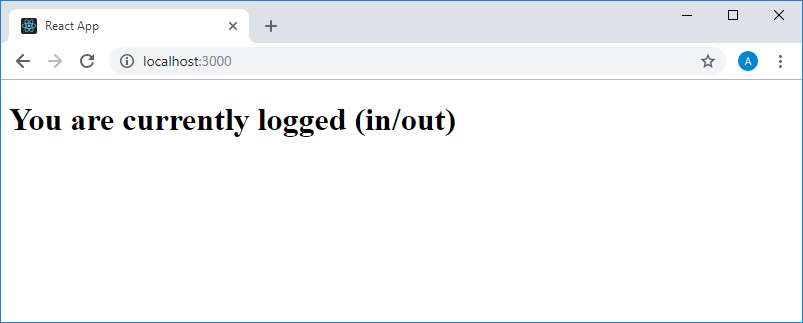
Application in the browser
In fact, if you yourself have reached this point, it means that you have learned the course material on components based on classes and the state of the components. Now we will be engaged in the optional task.
In essence, we will discuss what needs to be done to complete this task in a lesson that is devoted to conditional rendering, so here we are a little ahead. So, we are going to declare and initialize a variable that will contain a string
Note that a variable
Here is what the application page will now look like:
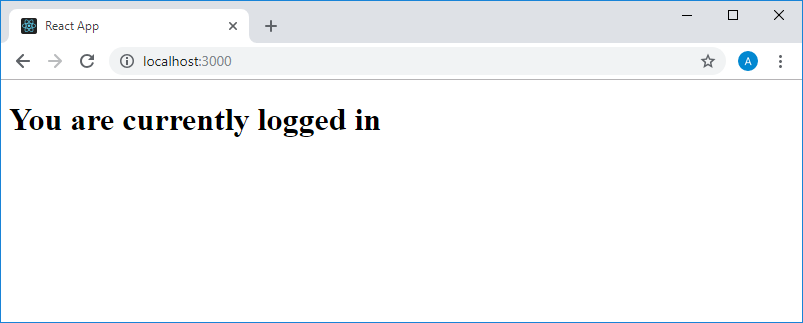
Application page in browser
The status property is
It should be noted that this problem can be solved in other ways. But the code that we got is clear and workable, so we’ll dwell on it, although, for example, given that it
Today you practiced working with the state of components, in particular, you fixed errors in your code, reworked the functional component into a component based on classes, did conditional rendering. The next time you are waiting for another practical work and a new topic.
Dear readers! Did you manage to find and correct all the mistakes yourself, performing the first of the practical works presented here?

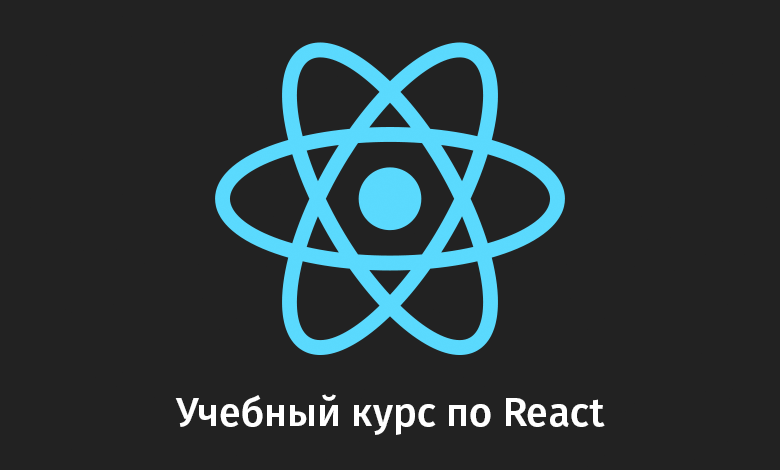
Part 8: continued work on a TODO application, familiarity with the properties of components
→ Part 9: properties of components
→ Part 10: a workshop on working with properties of components and styling
→ Part 11: dynamic formation of markup and the array method map
→ Part 12: workshop, third stage of working on a TODO application
→ Part 13: components based on classes
→ Part 14: a workshop on components based on classes, the state of components
→ Part 15: workshops on working with the state of components
Lesson 27. Workshop. Component Status, Debugging
→ Original
▍Job
Analyze the following class code
App
from the App.js
standard React application file created by the tools create-react-app
. This code is incomplete, it has errors.import React from"react"classAppextendsComponent() {
return (
<div>
<h1>{this.state.name}</h1>
<h3><fontcolor="#3AC1EF">▍{this.state.age} years old</font></h3>
</div>
)
}
exportdefault App
A component
App
based on a class does not have a constructor, its state is not initialized, but when forming what it returns, it means that it has a state with some data. Your task is to bring this code into working condition.
If you come across some unknown error message - do not rush to look into the solution. Try it yourself, for example, carefully read the code and look for information about the problem on the Internet, find out the cause of the error and fix it. Debugging code is a valuable skill that will definitely come in handy when working on real projects.
▍Decision
The body of a class is similar to the body of a functional component. It has only a command
return
, but in class-based components, this command is used in the method render()
, not in the body of the class. Fix it.import React from"react"classAppextendsComponent() {
render() {
return (
<div>
<h1>{this.state.name}</h1>
<h3><fontcolor="#3AC1EF">▍{this.state.age} years old</font></h3>
</div>
)
}
}
exportdefault App
If you continue the analysis of the code, looking at the error messages displayed in the browser, you can understand that although the design
class App extends Component
looks quite normal, with what we refer to by name Component
, there is still something wrong. The problem is that in the import command,, import React from "react"
we import into the project only React
, but not Component
. That is, we need to either edit the command, bringing it to mind import React, {Component} from "react"
, then when you create a class, we can use existing code, or rewrite the class declaration of the form: class App extends React.Component
. We will focus on the first option. Now the component code looks like this:import React, {Component} from"react"classAppextendsComponent() {
render() {
return (
<div>
<h1>{this.state.name}</h1>
<h3><fontcolor="#3AC1EF">▍{this.state.age} years old</font></h3>
</div>
)
}
}
exportdefault App
True, this problem does not end there. The application does not work, an error message appears in the browser
TypeError: Cannot set property 'props' of undefined
, we are informed that something is wrong with the first line of the class declaration. The point here is that when declaring a component, which, as we already understood, is a component that is based on a class, after the name of the parent class there is a pair of parentheses. They are not needed here, this is not a functional component, so we will get rid of them. Now the application code is more or less efficient, the component based on the class no longer looks completely wrong, but the system continues to report errors to us. Now the error message looks like this:
TypeError: Cannot read property 'name' of null
. Obviously, it refers to an attempt to use data stored in a component state that has not yet been initialized. Therefore, now we will create a class constructor, not forgetting to call it super()
, and initialize the state of the component, adding to it the values that the component is trying to work with. Here is the finished working component code
App
:import React, {Component} from"react"classAppextendsComponent{
constructor() {
super()
this.state = {
name: "Sally",
age: 13
}
}
render() {
return (
<div>
<h1>{this.state.name}</h1>
<h3><fontcolor="#3AC1EF">▍{this.state.age} years old</font></h3>
</div>
)
}
}
exportdefault App
This is how the application page will look like in a browser.

Application page in the browser
Session 28. Workshop. The state of the components, working with data stored in the state
→ Original
In this practice session, you will have another chance to work with the state of the components.
▍Job
Below is the code of the functional component. Taking it as a basis, do the following:
- Convert it so that the component has a state.
- In the component state, there must be a property
isLoggedIn
that stores a boolean valuetrue
if the user is logged in, andfalse
if not (in our case there are no “logon" mechanisms here, the corresponding value must be set manually when initializing the state). - Try to make sure that if the user logs into the system, the component would display the text
You are currently logged in
, and if not, the textYou are currently logged out
. This task is optional, if you have difficulties in carrying it out, you can, for example, search for ideas on the Internet.
Here is the file code
App.js
:import React from"react"functionApp() {
return (
<div>
<h1>You are currently logged (in/out)</h1>
</div>
)
}
exportdefault App
▍Decision
We have a functional component at our disposal. In order to equip it with a state, it must be converted into a component based on the class and initialized with its state. Here is the code for the converted component:
import React from"react"classAppextendsReact.Component{
constructor() {
super()
this.state = {
isLoggedIn: true
}
}
render() {
return (
<div>
<h1>You are currently logged (in/out)</h1>
</div>
)
}
}
exportdefault App
Check the performance of the application.
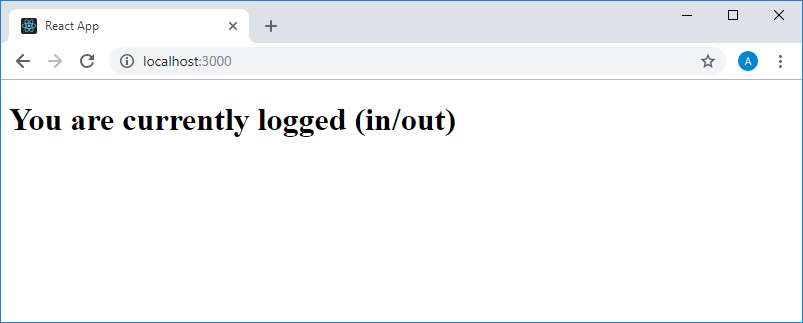
Application in the browser
In fact, if you yourself have reached this point, it means that you have learned the course material on components based on classes and the state of the components. Now we will be engaged in the optional task.
In essence, we will discuss what needs to be done to complete this task in a lesson that is devoted to conditional rendering, so here we are a little ahead. So, we are going to declare and initialize a variable that will contain a string
in
orout
depending on what is stored in the state propertyisLoggedIn
. We will work with this variable in the methodrender()
, first analyzing the data and writing down the desired value in it, and then using it in the JSX code returned by the component. This is what we ended up with:import React from"react"classAppextendsReact.Component{
constructor() {
super()
this.state = {
isLoggedIn: true
}
}
render() {
let wordDisplay
if (this.state.isLoggedIn === true) {
wordDisplay = "in"
} else {
wordDisplay = "out"
}
return (
<div>
<h1>You are currently logged {wordDisplay}</h1>
</div>
)
}
}
exportdefault App
Note that a variable
wordDisplay
is a regular local variable declared in a method render()
, so to access it within this method, you just need to specify its name. Here is what the application page will now look like:
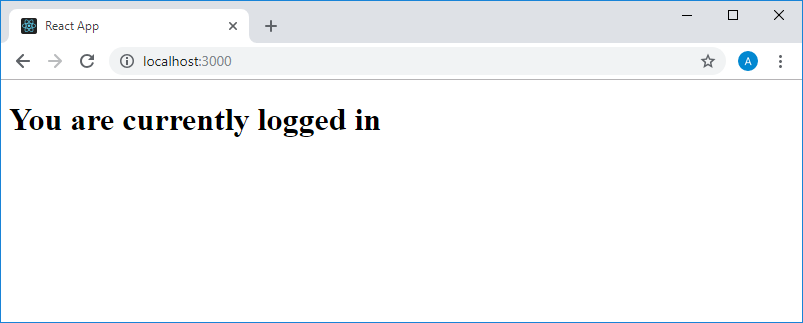
Application page in browser
The status property is
isLoggedIn
set to a valuetrue
, so text is displayed on the pageYou are currently logged in
. To display the textYou are currently logged out
you need to change, in the component code, the valueisLoggedIn
tofalse
. It should be noted that this problem can be solved in other ways. But the code that we got is clear and workable, so we’ll dwell on it, although, for example, given that it
isLoggedIn
is a logical variable, the conditionif (this.state.isLoggedIn === true)
can be rewritten asif (this.state.isLoggedIn)
.Results
Today you practiced working with the state of components, in particular, you fixed errors in your code, reworked the functional component into a component based on classes, did conditional rendering. The next time you are waiting for another practical work and a new topic.
Dear readers! Did you manage to find and correct all the mistakes yourself, performing the first of the practical works presented here?
