The book "Spring. All design patterns »

We offer you to familiarize yourself with the passage “Pattern“ Object of access to data ””
The Data Access Object (DAO) is an extremely popular design pattern for the persistence layer in J2EE applications. It separates the level of business logic from the level of conservation. The DAO pattern is based on object-oriented principles of encapsulation and abstraction. DAO pattern usage context - data access and storage depending on the specific implementation and type of storage, such as an object-oriented database, unstructured files, relational databases, etc. Based on the DAO pattern, you can create a DAO interface and implement it, to abstract and encapsulate all calls to the data source. Such a DAO implementation manages database resources such as connections to a data source.
DAO interfaces are very easy to adapt to all underlying data source mechanisms; they do not need to be replaced with changes in storage technologies at lower levels. This pattern allows you to implement various data access technologies without in any way affecting the business logic of the enterprise application. Consider rice. 8.1 to better understand the principles of the DAO pattern.
As you can see in the diagram, the following objects participate in the pattern.
BusinessObject is a business-level object that is a client for a data access level. It needs data to model business processes, as well as prepare Java objects for supporting functions or application controllers.
DataAccessObject is the main object of the DAO pattern. Hides from BusinessObject the entire low-level implementation of the underlying database.
A DataSource is also an object containing all low-level information about what exactly the underlying database is: RDBMS, unstructured files, or XML.
TransferObject is an object used as a storage medium. Used by a DataAccessObject object to return data to a BusinessObject object.
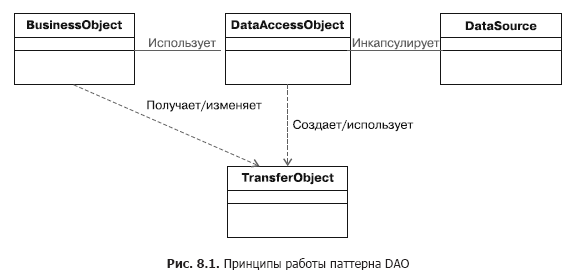
Consider the following example of a DAO pattern, in which AccountDao is the interface of the DataAccessObject object, and AccountDaoImpl is the class that implements the AccountDao interface:
public interface AccountDao {
Integer totalAccountsByBranch(String branchName);
}
public class AccountDaoImpl extends JdbcDaoSupport implements
AccountDao {
@Override
public Integer totalAccountsByBranch(String branchName) {
String sql = "SELECT count(*) FROM Account WHERE branchName =
"+branchName;
return this.getJdbcTemplate().queryForObject(sql,
Integer.class);
}
}
Creating DAO objects in Spring using the Factory design pattern
As you know, there are many design patterns involved in the Spring framework. The “Factory” pattern is a generating design pattern and is used to create an object without disclosing the underlying logic to the client, as well as assigning it to the caller of a new object using a common interface or an abstract class. Thanks to the “Factory Method” and “Abstract Factory” design patterns, a very high flexibility of the DAO pattern can be achieved.
Let us find out where in our example we are implementing a strategy in which the factory produces DAO objects for the implementation of a common database (Fig. 8.2).
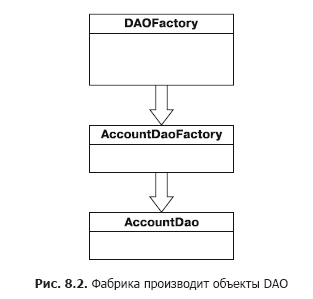
You can see in the previous diagram that AccountDaoFactory produces an AccountDao object, that is, it is a factory for it. You can replace the underlying database at any time, and you don’t need to change the business code - the factory takes over the job. Spring supports storing all DAOs in a component factory, as well as in a DAO factory.
Pattern "Display data"
The ORM framework provides the mapping between objects and relational databases, because the objects and tables of relational databases store application data in different ways. In addition, there are various mechanisms for structuring data in objects and tables. When using any ORM solution in a Spring application, such as Hibernate, JPA, or JDO, there is no need to worry about the display mechanism between objects and relational databases.
Consider rice. 8.3 to better understand the “Data Mapper” pattern.
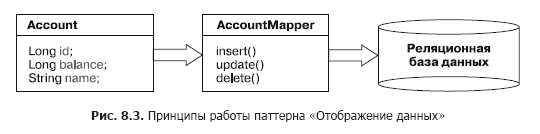
On the diagram, the Account object is mapped to a relational database through the AccountMapper interface. AccountMapper plays the role of an intermediary between the Java object and the underlying database in the application. Consider another pattern used at the data access level.
Pattern "Model domain"
A domain model is an object that has both data and behavior, where behavior defines the business logic of an enterprise application, and data represents information about business results. The domain model combines data and workflow. In a corporate application, the data model is located below the business layer and defines the business logic, returning the results of business behavior. Consider the following diagram for clarity (Fig. 8.4).

As you can see in the diagram, the application has two domain models defined in accordance with the business requirements. The business algorithm for transferring money from one account to another is described in the TransferService class. The TransferService and AccountService classes are related to the domain model model in a corporate application.
Proxy for the pattern "delayed loading"
“Delayed loading” is a design pattern used by some of the ORM solutions, for example, Hibernate, in corporate applications to postpone the initialization of an object until another object refers to it, that is, the moment it is needed. The purpose of this design pattern is to optimize the memory in the application. The Hibernate delayed load design pattern is implemented using a virtual proxy object. When demonstrating deferred loading, we use a proxy, but it does not belong to the “Deputy” pattern.
Pattern Method Pattern for Spring Hibernate Support
The Spring framework provides a helper class for data access at the DAO level, based on the GoF Template Method Design Pattern. The HibernateTemplate class of the Spring framework supports database operations such as save, create, delete, and update. This class ensures that only one Hibernate session is used for each transaction.
Hibernate integration with Spring
Hibernate is an open source ORM storage framework that not only displays simple object relationships between Java objects and database tables, but also offers many advanced features to increase application performance and also helps improve resource utilization, such as caching. , deferred loading, immediate data retrieval and distributed caching.
Spring provides full support for integration with the Hibernate framework, it has several built-in libraries that allow you to use Hibernate 100%. To set the Hibernate settings in the application, you can use the dependency injection pattern and the Spring framework IoC container.
In the next section, we'll figure out how to properly configure Hibernate in the Spring framework's IoC container.
Setting the SessionFactory object of the Hibernate framework in the Spring container
The best approach to customizing Hibernate and other storage technologies in any enterprise application is to separate business objects with hardwired resource directories, such as the DataSource in JDBC or the SessionFactory in Hibernate. These resources can be described as components in a Spring container. But to access them, business objects need links to them. Consider the following DAO class, using the SessionFactory object to retrieve data for an application:
public class AccountDaoImpl implements AccountDao {
private SessionFactory sessionFactory;
public void setSessionFactory(SessionFactory sessionFactory) {
this.sessionFactory = sessionFactory;
}
// ...
}
As you can see, the DAO class, AccountDaoImpl, follows the dependency injection pattern. To access the data, the Hibernate framework's SessionFactory object is embedded in it, and it feels great in the IoC Spring container. The SessionFactory object of the Hibernate framework is a single object, it generates the main Hibernate interface object of the org.hibernate.Session. The SessionFactory object manages the Session object, and is also responsible for opening and closing it. The Session interface contains real data access functionality - saving (save), updating (updating), deleting (deleting) and loading (loading) objects from the database. In an application, an object of class AccountDaoImpl or any other repository performs all the necessary data storage operations using this Session object.
The Spring framework provides built-in Hibernate modules, so you can use the SessionFactory Hibernate components in your applications.
The org.springframework.orm.hibernate5.LocalSessionFactoryBean component is an implementation of the Spring framework FactoryBean interface. LocalSessionFactoryBean is based on the Abstract Factory pattern; it generates a SessionFactory object in the application. This object can be configured as a component in the context of a Spring application as follows:
@Bean
public LocalSessionFactoryBean sessionFactory(DataSource
dataSource) {
LocalSessionFactoryBean sfb = new LocalSessionFactoryBean();
sfb.setDataSource(dataSource);
sfb.setPackagesToScan(new String[] {
"com.packt.patterninspring.chapter8.bankapp.model" });
Properties props = new Properties();
props.setProperty("dialect",
"org.hibernate.dialect.H2Dialect");
sfb.setHibernateProperties(props);
return sfb;
}
In this code, we configured the SessionFactory object as a component using the Spring framework LocalSessionFactoryBean class. The method of this component accepts as input an object of the DataSource type as an argument that defines the location and method of connecting to the database. We also specified which package to view by setting the “com.packt.patterninspring.chapter8.bankapp.model” value for the setPackagesToScan property of the LocalSessionFactoryBean component, and setting the dialect property of the SessionFactory component using the setHibernateProperties method to specify what type of database we have matter in the application.
Now, after configuring the SessionFactory Hibernate component, in the context of the Spring application, let's see how we can implement data access objects for the storage level of our application.
about the author
Dinesh Rajput is the chief editor of Dineshonjava, a technical blog dedicated to Java and Spring. The site contains articles on Java technology. Dinesh is a blogger, author of books, c 2008 Spring enthusiast, certified specialist of the company Pivotal (Pivotal Certified Spring Professional). He has more than ten years of design and development experience using Java and Spring. Specializes in working with the latest version of the Spring Framework, Spring Boot, Spring Security, creating REST API, microservice architecture, reactive programming, aspect-oriented programming using Spring, design patterns, Struts, Hibernate, web services, Spring Batch, Cassandra, MongoDB, web application architecture.
Currently, Dinesh works as a technology manager in one of the companies leading in the field of software development. He was a developer and team leader at Bennett, Coleman & Co. Ltd, and before that, the lead developer at Paytm. Dinesh is enthusiastic about the latest Java technology and loves to write about them in technical blogs. He is an active participant in the Java and Spring communities in various forums. Dinesh is one of the best specialists in Java and Spring.
About Reviewer
Rajiv Kumar Mohan has extensive experience in software development and corporate training. For 18 years he has worked at such major IT companies as IBM, Pentasoft, Sapient and Deft Infosystems. He started his career as a programmer, led many projects.
He is an expert in the domain of Java, J2EE and related frameworks, Android, UI-technologies. Sun certified as a Java Programmer (SCJP, Sun Certified Java Programmer) and Java Web Developer (Sun Certified Web Component Developer, SCWCD). Rajeev has four higher educations: in the field of computer science (Computer Science), applied computer science (Computer Applications), organic chemistry and business administration (MBA). Recruitment consultant and training expert for HCL, Amdocs, Steria, TCS, Wipro, Oracle University, IBM, CSC, Genpact, Sapient Infosys and Capgemini.
Rajeev is the founder of SNS Infotech, located in the city of Greater Noida. In addition, he worked at the National Institute of Fashion Technology (National Institute of Fashion Technology, NIFT).
»More information about the book can be found on the publisher's website
» Table of contents
» Excerpt
For Habrozhiteley 20% discount on the coupon - Spring