
5 best ORM for Android
- Transfer

As you know, we are directly involved in the development of applications for Android, because this OS is installed on YotaPhone. And with this post we are opening a series of publications in which we will cover various aspects of developing applications for Android, share experience, both our own and other specialists. By the way, some time ago we already wrote about the SDK and the features of the YotaPhone architecture, which also partly echoes the topic of this publication: the fully developed SDK is built on the principles similar to the standard Android SDK to give developers intuitive tools.
If you are developing applications for Android, then you most likely need to store data somewhere. You can choose one of the cloud services (in this case, it’s convenient to use SyncAdapter) or use the built-in SQLite database. In the second case, you have to choose between recording SQL queries using the Content Provider (if you plan to use the data in multiple applications) or ORM .
In this post, we will look at some of the Android ORMs that you can use in your applications.
Ormite
Perhaps this is the first ORM that comes to mind. However, this is not Android ORM, but Java ORM with support for SQL databases. It can be used wherever Java is used, for example, in JDBC connections , Spring, and Android.
Annotations are actively used here, for example,
@DatabaseTable
for each class defining a table, and @DatabaseField
for each field in the class. A simple example of using OrmLite to define a table:
@DatabaseTable(tableName = "users")
public class User {
@DatabaseField(id = true)
private String username;
@DatabaseField
private String password;
public User() {
// ORMLite needs a no-arg constructor
}
public User(String username, String password) {
this.username = username;
this.password = password;
}
// Implementing getter and setter methods
public String getUserame() {
return this.username;
}
public void setName(String username) {
this.username = username;
}
public String getPassword() {
return this.password;
}
public void setPassword(String password) {
this.password = password;
}
}
This is an open source project, you can find it on GitHub . For more information, see the official documentation .
Sugarorm
This ORM was created specifically for Android. The kit comes with an API that is easy to learn and remember. He can create the necessary tables himself and contains simple methods for forming one-to-one and one-to-many relationships. Also facilitates SugarORM create, read, update and delete (the CRUD) with just three options:
save()
, delete()
and find()
(or findById()
). To use SugarORM in your application, you need to
AndroidManifest.xml
add four tags in meta-data
:
Now you can use this ORM in the classes you want to turn into tables:
public class User extends SugarRecord {
String username;
String password;
int age;
@Ignore
String bio; //this will be ignored by SugarORM
public User() { }
public User(String username, String password,int age){
this.username = username;
this.password = password;
this.age = age;
}
}
Adding a new user:
User johndoe = new User(getContext(),"john.doe","secret",19);
johndoe.save(); //stores the new user into the database
Removing all users aged 19:
List nineteens = User.find(User.class,"age = ?",new int[]{19});
foreach(user in nineteens) {
user.delete();
}
You can learn more about the capabilities of SugarORM in its documentation .
Greendao
If you need high performance, then be sure to look at GreenDAO . As stated on their website, “most entities can be added, updated, or downloaded with a throughput of several thousand operations per second .” And if the authors were cunning about the capabilities of their offspring, then it would hardly be used in these well-known applications . Compared to the same OrmLite, GreenDAO is almost 4.5 times faster:
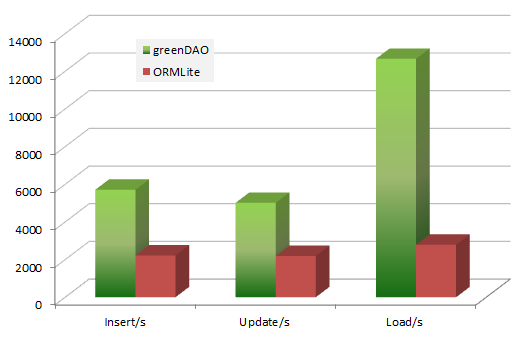
It is the smallest by 100 Kb in size, which does not really matter.
You can see examples of using GreenDAO in the tutorial using Android Studio. Those who wish can also familiarize themselves with the project code on GitHub and studythe documentation .
ActiveAndroid
Like many other ORMs, ActiveAndroid helps you store and retrieve records from SQLite without creating SQL queries.
To use ActiveAndroid, you need to add the jar file to the folder
/libs
. As stated in the initial instructions , you need to copy the source code from GitHub and compile using Maven . After connecting ActiveAndroid to your project, add to the AndroidManifest.xml
tags meta-data
:
After that, if necessary, you can call
ActiveAndroid.initialize()
:
public class MyActivity extends Activity {
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
ActiveAndroid.initialize(this);
//rest of the app
}
}
Now you can, using annotations, create models as Java classes:
@Table(name = "User")
public class User extends Model {
@Column(name = "username")
public String username;
@Column(name = "password")
public String password;
public User() {
super();
}
public User(String username,String password) {
super();
this.username = username;
this.password = password;
}
}
This is a simple example of using ActiveAndroid, for more complex ways to use, read the project documentation .
Realm
The last ORM in our review, Realm is written in C ++ and runs right on your device (without interpretation), which provides very high performance. The iOS version code, if anyone is interested, can be found on GitHub . You can also find off-line examples of using Realm in Objective-C and Swift.
Conclusion
Of course, these are far from the only ORMs that exist in nature. Out of the scope of this review are, for example, Androrm and ORMDroid . Sure, every developer should be able to work with SQL, but creating queries is boring and lazy, so why not use one of the many ready-made ORMs to automate the process? They can greatly simplify your work.