
ROBOT based on: android, arduino, bluetooth. Start
Creating a robot, even a simple one with limited functionality, is a rather interesting and fascinating task. Recently, amateur robotics is experiencing a real boom, even those people who are very far from electronics (I belong to them) have begun to get involved in it. The time has passed when you had to sit at night with a soldering iron, or poison boards. Everything has become much simpler, you just need to buy an Arduino, a set of wires, sensors, sensors, motors and go on to assemble your first robot. Thus, the focus of amateur developers has shifted from electronics and the mechanical to programming.
This series of articles will contain information for a quick start on creating your first robot, from buying the necessary parts to launching it in our world.
I note that I write articles in the course of independent study of the material and the creation of my first robot.
It is assumed that the person reading the article already has experience in:
BT - Bluetooth;
Android - a device with android OS, for example, a smartphone;
Sketch - an application for Arduino;
Arduino - Arduino Nano.
Let the robot have the following general scheme (Fig. 1). Android is the “brain” in which all data is processed and calculations are carried out. Based on the results of the calculation, the BT channel receives the commands received by the BT module and subsequently received via the serial port in Arduino. Arduino processes the received commands and executes them with the help of the “muscles” (motors LEDs, etc.). In addition, Arduino receives data from the “sensory organs” (sensors, sensors, etc.), which it brings in a convenient form and sends using the BT module to the “brain”. And so it repeats to infinity.
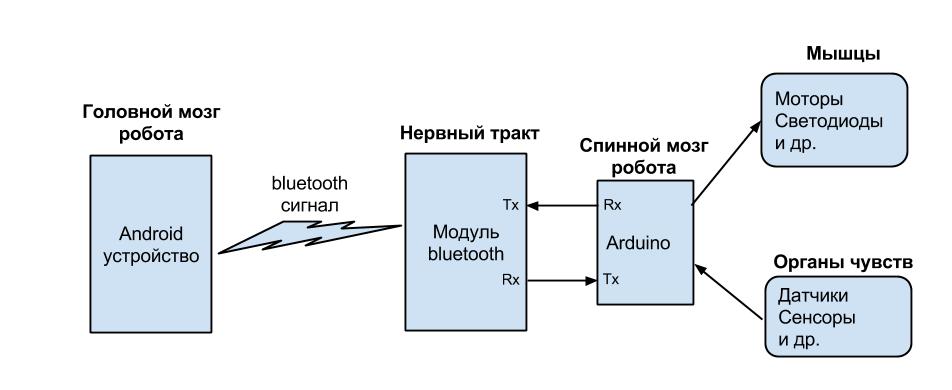
Figure 1 The
rationale for just such a robot circuit is as follows:
-BT module and Arduino Nano have a low cost, as well as a small volume and weight.
-Android, not an expensive and affordable device, already has a huge number of people, including me.
-Android has its own sensors, sensors and a screen for displaying information
-Easy to write code for applications for both android and arduino.
To simplify the task of building a robot, I suggest using the “from simple to complex” method, i.e. as in the study of the programming language, we will create the first program “Hello word”. Of course, this will not be one program, but at least two (for arduino and android). In each subsequent article, the functionality of the robot will increase.
Assemble a simple device that has:
-2 buttons (b1, b2).
-1 LED (Led).
And the functionality is executed:
-the main activity contains 2 buttons “Send 0” and “Send 1”, when clicked, data is transferred from the android to the arduino via the BT channel, respectively “0” and “1”, the arduino processes it and lights or extinguishes Light-emitting diode.
-data about pressing or releasing buttons from the arduino is transferred to android, information about this is displayed on the screen of the main activity.
1) Android device - LG P500 smartphone (Android version 2.3.3), or any other Android device with version 2.3.3 and higher. I don’t indicate the price, because I didn’t buy it separately, but I use my smartphone.
2) Arduino NanoV3.0 ( dx.com/en/p/nano-v3-0-avr-atmega328-p-20au-module-board-usb-cable-for-arduino-118037 ) - $ 11.32
3) Bluetooth module ( dx.com/en/p/bluetooth-board-module-4-pin-121326 ) - $ 10.57
4) Breadboard wires ( dx.com/en/p/30cm-breadboard-wires-for-electronic -diy-40-cable-pack-80207 ) - $ 2.51
5) Buttons - 2 pcs., LED - 1 pc. - 50 rubles
Total: 855.20 rubles.
We will assemble the circuit from the available parts (Fig. 2)
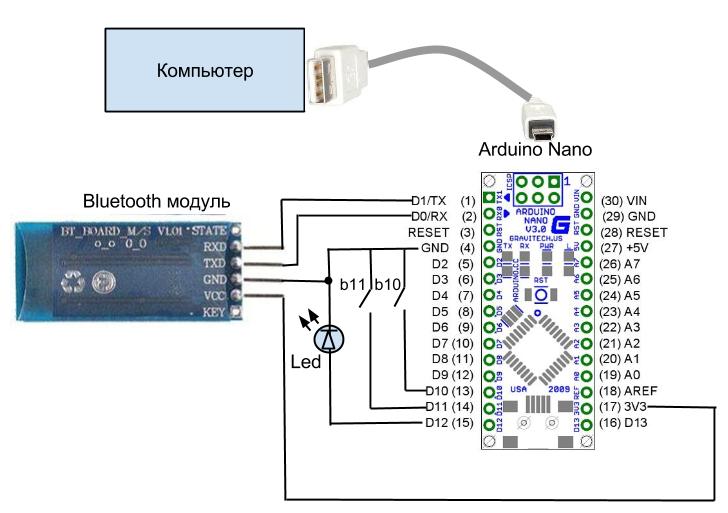
Figure 2
Power is supplied to the arduino via a USB cable that connects to the computer, and the software (sketch) running on it is also loaded on it. It should be noted that you can upload a sketch to arduino only when the power from the Bluetooth module is disconnected (Output (17) 3V3), otherwise an error occurs.
Details of installing the Arduino development environment and drivers can be found on the official website: arduino.ru/Guide/Windows
The following is a sketch that must be downloaded to arduino:
We declare the variables, each one has a comment.
We initiate a serial connection and set the data transfer rate in bit / c (baud). My BT module works at a speed of 38400, but there can be a speed of 9600 (the speed of a BT module can be set using AT commands). We set the operation mode of a given input / output (pin) as an input or as an output. We give a HIGH value to the inputs and outputs.
The void contact_bounce (int buttton) function processes button presses and eliminates contact chatter that occurs when contacts or contacts diverge in mechanical switching devices, such as a button, repeatedly close and open.
In the main Loop loop, we listen to the serial port, and if data came to it, we process them. In addition, we call the function of processing button presses and eliminating contact bounce.
Having loaded the sketch into arduino, we can check its operability by launching the port monitor. When the buttons are pressed, the message “Press button b” will be displayed in the monitor window. It will be possible to verify the operation of the LED only after writing the application for the android.
In this article, only this sketch will be used for arduino. Let's start developing an application for android.
For the convenience of debugging android applications, I recommend using not an “Android virtual device”, but a real smartphone with Android OS version 2.3.3 connected via a USB cable to a computer in the “Debugging” mode. There are a huge number of articles on how to do this.
We create a new project "Android application project"
To work with BT, you need to set the rights to use it with our application. To do this, go to the manifest, select the Permissions tab, click add, then Uses permission, and set the following rights: android.permission.BLUETOOTH, android.permission.BLUETOOTH_ADMIN
Now let's execute the main activity, put the code in res / layout / activity_main.xml:
Thus, the main activity will take the form:

Figure 3 The
text field “txtrobot” will display all the information we need. Buttons b1 and b2 will send commands to arduino.
Now go to src /../ MainActivity.java here and our main code will be located. Connect Api package for Bluetooth:
Before using BT, you need to make sure that it is present in our android. Let's create an instance of the BluetoothAdapter class (responsible for working with the BT module installed in android):
If the android does not have BT then null will be returned:
In this form, the program can already be launched. On the android screen you should see the inscription: "Bluetooth is present."
Now you need to make sure that the BT is turned on, or offer to turn it on. Add a constant:
and code:
By launching the modified application on the android, you will be given a “Request permission to enable Bluetooth”, confirming it, thereby activating the BT.
Full application code:
For further experiments, it is necessary to “pair” our android and BT module, for this, on the android in the BT settings, perform a search and connect to the BT module, the password is “1234”.
If suddenly the remote BT module does not work properly, just disconnect all the wires from it (VCC, GND, RX, TX), thereby making a hard reboot, and reconnect them - this should help.
Now let's try programmatically connecting to the remote BT module: we will place the main connection code in onResume. onResume is one of the states of our Activity, namely, the Activity is visible on the screen, it is in focus, the user can interact with it. The following is the main activity code:
Access to the remote BT module is obtained by its MAC address btAdapter.getRemoteDevice (MacAdress). You can find out the MAC address of the BT module using the program for android: Bluetooth Terminal.
To make sure that access to the BT module is obtained, we use the getName () method, which allows you to get the name of the remote BT module, and display the result on the android screen.
Also in this example, the ability to maintain a log was added, which, during the execution of the program, you can view and detect existing errors. In addition, a function MyError has been created, which is called if you need to crash the application.
Having launched the application, the name of the remote BT module will be displayed in the “txtrobot” text box on the android screen.
Access to the remote BT module is obtained, our next step is to transfer data from the android to it. To do this in onResume (), create a socket:
where UUID (Universally Unique Identifier) is an identification standard used in software development. Add the UUID constant to the definition:
In order not to slow down the connection, cancel the search for other BT devices:
Let's try to connect:
If not successful, close the socket:
Since the methods of sending and receiving data are blocking, they should be performed in a separate thread to prevent the main application from freezing. To do this, create a class:
In the constructor of public ConnectedThred (BluetoothSocket socket), an object is created that controls the transmission of data through the socket:
To send data from the main activity, the sendData (String message) method is called with the text message parameter, which is converted to the byte type. The cancel () method allows you to close the socket.
We write the handlers for pressing the buttons b1 and b2 that contain the call to the sendData (String message) function and record this in the log. The full application code is given below:
The application we wrote allows you to transfer data via BT from the android to the arduino - “0” and “1”, which in turn are for the arduino commands “1” - to light the LED, and “0” - to put out. Thus, taking into account the general scheme of the robot, we learned to give commands from the “brain” to the “muscles”. It remains the case for small, to teach the android to accept data from the "senses."
Receiving data as well as sending, must be performed in a separate thread in order to avoid hanging of the main activity. We will display the received data from the BT module on the screen of the main activity in the text field - MyText. But the difficulty arises - working with view components is available only from the main stream. And the new threads that we create do not have access to the screen elements. To solve this problem, use the Handler mechanism.
Handler is a mechanism that allows you to work with a message queue. It is attached to a specific thread (thread) and works with its queue. Handler can queue messages. At the same time, he puts himself as the recipient of this message. And when the time comes, the system removes the message from the queue and sends it to the addressee (i.e. in Handler) for processing.
Declare Handler:
Create your Handler:
we implement the handleMessage message processing method in it. We extract the what, obj attribute and arguments of type int from the message. We transform the received message into a string and display it in the text field of the main activity: mytext.setText ("Data from Arduino:" + strIncom);
In the stream for data transfer, add the function to start this stream, and place a cycle with the function for reading data there:
The full application code is given below:
In step 4, an application is presented that allows you to transmit commands via the BT from the android to the arduino, as well as receive data from the arduino. Thus, we learned to give commands and receive data, and therefore completed the task.
In addition, this application is a template, on its basis you can create more complex applications, which in turn will be able to process data from an ultrasonic sensor, for example, and also give commands to motors to move the robot.
For the next article, I ordered new parts and modules. Below is a list and prices:
TOTAL: 1933.8
This series of articles will contain information for a quick start on creating your first robot, from buying the necessary parts to launching it in our world.
I note that I write articles in the course of independent study of the material and the creation of my first robot.
It is assumed that the person reading the article already has experience in:
- Install and configure the development environment for android devices.
- Writing the first Hello World program for an android device.
- Installing drivers and development environments for Arduino.
- Writing the first program (turn on, turn off) LEDs on the Arduino.
Abbreviations
BT - Bluetooth;
Android - a device with android OS, for example, a smartphone;
Sketch - an application for Arduino;
Arduino - Arduino Nano.
The general scheme of the robot
Let the robot have the following general scheme (Fig. 1). Android is the “brain” in which all data is processed and calculations are carried out. Based on the results of the calculation, the BT channel receives the commands received by the BT module and subsequently received via the serial port in Arduino. Arduino processes the received commands and executes them with the help of the “muscles” (motors LEDs, etc.). In addition, Arduino receives data from the “sensory organs” (sensors, sensors, etc.), which it brings in a convenient form and sends using the BT module to the “brain”. And so it repeats to infinity.
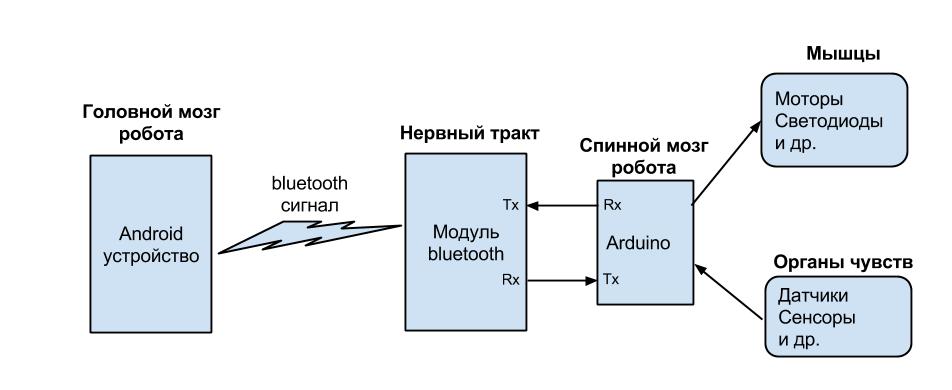
Figure 1 The
rationale for just such a robot circuit is as follows:
-BT module and Arduino Nano have a low cost, as well as a small volume and weight.
-Android, not an expensive and affordable device, already has a huge number of people, including me.
-Android has its own sensors, sensors and a screen for displaying information
-Easy to write code for applications for both android and arduino.
To simplify the task of building a robot, I suggest using the “from simple to complex” method, i.e. as in the study of the programming language, we will create the first program “Hello word”. Of course, this will not be one program, but at least two (for arduino and android). In each subsequent article, the functionality of the robot will increase.
Formulation of the problem
Assemble a simple device that has:
-2 buttons (b1, b2).
-1 LED (Led).
And the functionality is executed:
-the main activity contains 2 buttons “Send 0” and “Send 1”, when clicked, data is transferred from the android to the arduino via the BT channel, respectively “0” and “1”, the arduino processes it and lights or extinguishes Light-emitting diode.
-data about pressing or releasing buttons from the arduino is transferred to android, information about this is displayed on the screen of the main activity.
Purchase of necessary parts and assemblies.
1) Android device - LG P500 smartphone (Android version 2.3.3), or any other Android device with version 2.3.3 and higher. I don’t indicate the price, because I didn’t buy it separately, but I use my smartphone.
2) Arduino NanoV3.0 ( dx.com/en/p/nano-v3-0-avr-atmega328-p-20au-module-board-usb-cable-for-arduino-118037 ) - $ 11.32
3) Bluetooth module ( dx.com/en/p/bluetooth-board-module-4-pin-121326 ) - $ 10.57
4) Breadboard wires ( dx.com/en/p/30cm-breadboard-wires-for-electronic -diy-40-cable-pack-80207 ) - $ 2.51
5) Buttons - 2 pcs., LED - 1 pc. - 50 rubles
Total: 855.20 rubles.
Let's get to work
Arduino
We will assemble the circuit from the available parts (Fig. 2)
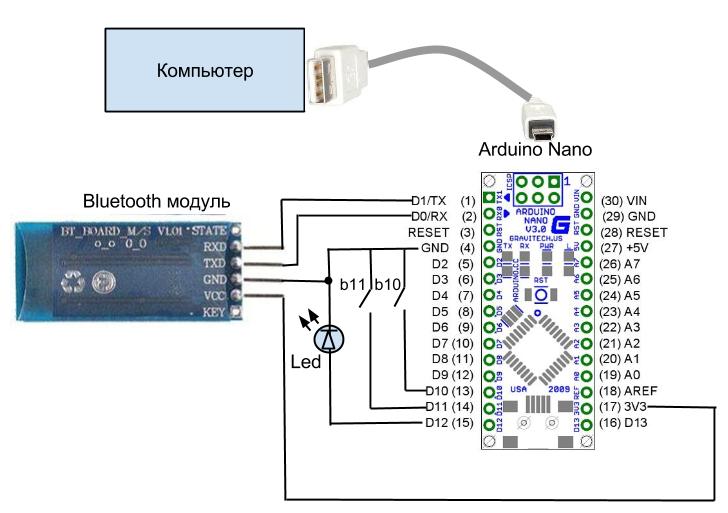
Figure 2
Power is supplied to the arduino via a USB cable that connects to the computer, and the software (sketch) running on it is also loaded on it. It should be noted that you can upload a sketch to arduino only when the power from the Bluetooth module is disconnected (Output (17) 3V3), otherwise an error occurs.
Details of installing the Arduino development environment and drivers can be found on the official website: arduino.ru/Guide/Windows
The following is a sketch that must be downloaded to arduino:
//Объявляем переменные
int led = 12; // Светодиод
int b1 = 11; // Кнопка
int b2 = 10; // Кнопка
int value_1,value_2 = 0; // Переменные необходимые для устранения дребезга контак
char incomingbyte; // переменная для приема данных
//Инициализация переменных
void setup() {
Serial.begin(38400);
pinMode(led,OUTPUT);
digitalWrite(led, HIGH);
pinMode(b1,INPUT);
digitalWrite(b1, HIGH);
pinMode(b2,INPUT);
digitalWrite(b2, HIGH);
}
//Обрабатываем нажатие кнопок и устраняем дребезг контактов
void contact_bounce(int buttton){
value_1 = digitalRead(buttton);
if (!value_1){
delay(80);
value_2 = digitalRead(buttton);
if (!value_2){
Serial.print("Press button b");
Serial.println(buttton);
}
}
}
//Основной цикл программы
void loop() {
if (Serial.available() > 0){
incomingbyte = Serial.read();
if (incomingbyte == '1'){
digitalWrite(led,HIGH);
Serial.println("LED ON");
}
if (incomingbyte=='0'){
digitalWrite(led,LOW);
Serial.println("LED OFF");
}
}
contact_bounce(b1);
contact_bounce(b2);
}
We declare the variables, each one has a comment.
We initiate a serial connection and set the data transfer rate in bit / c (baud). My BT module works at a speed of 38400, but there can be a speed of 9600 (the speed of a BT module can be set using AT commands). We set the operation mode of a given input / output (pin) as an input or as an output. We give a HIGH value to the inputs and outputs.
The void contact_bounce (int buttton) function processes button presses and eliminates contact chatter that occurs when contacts or contacts diverge in mechanical switching devices, such as a button, repeatedly close and open.
In the main Loop loop, we listen to the serial port, and if data came to it, we process them. In addition, we call the function of processing button presses and eliminating contact bounce.
Having loaded the sketch into arduino, we can check its operability by launching the port monitor. When the buttons are pressed, the message “Press button b” will be displayed in the monitor window. It will be possible to verify the operation of the LED only after writing the application for the android.
In this article, only this sketch will be used for arduino. Let's start developing an application for android.
Android
For the convenience of debugging android applications, I recommend using not an “Android virtual device”, but a real smartphone with Android OS version 2.3.3 connected via a USB cable to a computer in the “Debugging” mode. There are a huge number of articles on how to do this.
Android STEP 1
We create a new project "Android application project"
To work with BT, you need to set the rights to use it with our application. To do this, go to the manifest, select the Permissions tab, click add, then Uses permission, and set the following rights: android.permission.BLUETOOTH, android.permission.BLUETOOTH_ADMIN
Now let's execute the main activity, put the code in res / layout / activity_main.xml:
Thus, the main activity will take the form:

Figure 3 The
text field “txtrobot” will display all the information we need. Buttons b1 and b2 will send commands to arduino.
Now go to src /../ MainActivity.java here and our main code will be located. Connect Api package for Bluetooth:
import android.bluetooth.*;
Before using BT, you need to make sure that it is present in our android. Let's create an instance of the BluetoothAdapter class (responsible for working with the BT module installed in android):
btAdapter = BluetoothAdapter.getDefaultAdapter();
If the android does not have BT then null will be returned:
TextView mytext = (TextView) findViewById(R.id.txtrobot);
if (btAdapter != null){
mytext.setText("Bluetooth присутствует");
}else
{
mytext.setText("Bluetooth отсутствует");
}
In this form, the program can already be launched. On the android screen you should see the inscription: "Bluetooth is present."
Now you need to make sure that the BT is turned on, or offer to turn it on. Add a constant:
private static final int REQUEST_ENABLE_BT = 1;
and code:
if (btAdapter.isEnabled()){
mytext.setText("Bluetooth включен. Все отлично.");
}else
{
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT);
}
By launching the modified application on the android, you will be given a “Request permission to enable Bluetooth”, confirming it, thereby activating the BT.
Full application code:
package com.robot.bluetest;
import android.os.Bundle;
import android.app.Activity;
import android.widget.TextView;
import android.bluetooth.*;
import android.content.Intent;
public class MainActivity extends Activity {
private static final int REQUEST_ENABLE_BT = 0;
public BluetoothAdapter btAdapter;
public TextView mytext;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
btAdapter = BluetoothAdapter.getDefaultAdapter();
mytext = (TextView) findViewById(R.id.txtrobot);
if (btAdapter != null){
if (btAdapter.isEnabled()){
mytext.setText("Bluetooth включен. Все отлично.");
}else
{
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT);
}
}else
{
mytext.setText("Bluetooth отсутствует");
}
}
}
Android STEP 2
For further experiments, it is necessary to “pair” our android and BT module, for this, on the android in the BT settings, perform a search and connect to the BT module, the password is “1234”.
If suddenly the remote BT module does not work properly, just disconnect all the wires from it (VCC, GND, RX, TX), thereby making a hard reboot, and reconnect them - this should help.
Now let's try programmatically connecting to the remote BT module: we will place the main connection code in onResume. onResume is one of the states of our Activity, namely, the Activity is visible on the screen, it is in focus, the user can interact with it. The following is the main activity code:
package com.robot.bluetoothrob2;
import java.io.IOException;
import android.os.Bundle;
import android.app.Activity;
import android.util.Log;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
import android.bluetooth.*;
import android.content.Intent;
public class MainActivity extends Activity {
private static final int REQUEST_ENABLE_BT = 0;
final String LOG_TAG = "myLogs";
public BluetoothAdapter btAdapter;
private BluetoothSocket btSocket = null;
// MAC-адрес Bluetooth модуля
private static String MacAdress = "20:11:02:47:01:60";
public TextView mytext;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
btAdapter = BluetoothAdapter.getDefaultAdapter();
mytext = (TextView) findViewById(R.id.txtrobot);
if (btAdapter != null){
if (btAdapter.isEnabled()){
mytext.setText("Bluetooth включен. Все отлично.");
}else
{
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT);
}
}else
{
MyError("Fatal Error", "Bluetooth ОТСУТСТВУЕТ");
}
}
@Override
public void onResume() {
super.onResume();
Log.d(LOG_TAG, "***Пытаемся соединиться***");
// Получаем удаленное устройство по его MAC адресу
BluetoothDevice device = btAdapter.getRemoteDevice(MacAdress);
mytext.setText("***Получили device = " + device.getName() + "***");
}
private void MyError(String title, String message){
Toast.makeText(getBaseContext(), title + " - " + message, Toast.LENGTH_LONG).show();
finish();
}
}
Access to the remote BT module is obtained by its MAC address btAdapter.getRemoteDevice (MacAdress). You can find out the MAC address of the BT module using the program for android: Bluetooth Terminal.
To make sure that access to the BT module is obtained, we use the getName () method, which allows you to get the name of the remote BT module, and display the result on the android screen.
Also in this example, the ability to maintain a log was added, which, during the execution of the program, you can view and detect existing errors. In addition, a function MyError has been created, which is called if you need to crash the application.
Having launched the application, the name of the remote BT module will be displayed in the “txtrobot” text box on the android screen.
Android STEP 3
Access to the remote BT module is obtained, our next step is to transfer data from the android to it. To do this in onResume (), create a socket:
btSocket = device.createRfcommSocketToServiceRecord(MY_UUID);
where UUID (Universally Unique Identifier) is an identification standard used in software development. Add the UUID constant to the definition:
private static final UUID MY_UUID = UUID.fromString("00001101-0000-1000-8000-00805F9B34FB");
In order not to slow down the connection, cancel the search for other BT devices:
btAdapter.cancelDiscovery();
Let's try to connect:
btSocket.connect();
If not successful, close the socket:
btSocket.close();
Since the methods of sending and receiving data are blocking, they should be performed in a separate thread to prevent the main application from freezing. To do this, create a class:
//Класс отдельного потока для передачи данных
private class ConnectedThred extends Thread{
private final BluetoothSocket copyBtSocket;
private final OutputStream OutStrem;
public ConnectedThred(BluetoothSocket socket){
copyBtSocket = socket;
OutputStream tmpOut = null;
try{
tmpOut = socket.getOutputStream();
} catch (IOException e){}
OutStrem = tmpOut;
}
public void sendData(String message) {
byte[] msgBuffer = message.getBytes();
Log.d(LOG_TAG, "***Отправляем данные: " + message + "***" );
try {
OutStrem.write(msgBuffer);
} catch (IOException e) {}
}
public void cancel(){
try {
copyBtSocket.close();
}catch(IOException e){}
}
public Object status_OutStrem(){
if (OutStrem == null){return null;
}else{return OutStrem;}
}
}
In the constructor of public ConnectedThred (BluetoothSocket socket), an object is created that controls the transmission of data through the socket:
tmpOut = socket.getOutputStream();
To send data from the main activity, the sendData (String message) method is called with the text message parameter, which is converted to the byte type. The cancel () method allows you to close the socket.
We write the handlers for pressing the buttons b1 and b2 that contain the call to the sendData (String message) function and record this in the log. The full application code is given below:
package com.robot.bluetoothrob2;
import java.io.IOException;
import java.io.OutputStream;
import java.net.Socket;
import java.util.UUID;
import com.robot.bluetoothrob2.R;
import android.os.Bundle;
import android.app.Activity;
import android.util.Log;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
import android.bluetooth.*;
import android.content.Intent;
public class MainActivity extends Activity {
private static final int REQUEST_ENABLE_BT = 1;
final String LOG_TAG = "myLogs";
private BluetoothAdapter btAdapter = null;
private BluetoothSocket btSocket = null;
private static String MacAddress = "20:11:02:47:01:60"; // MAC-адрес БТ модуля
private static final UUID MY_UUID = UUID.fromString("00001101-0000-1000-8000-00805F9B34FB");
private ConnectedThred MyThred = null;
public TextView mytext;
Button b1, b2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
btAdapter = BluetoothAdapter.getDefaultAdapter();
mytext = (TextView) findViewById(R.id.txtrobot);
if (btAdapter != null){
if (btAdapter.isEnabled()){
mytext.setText("Bluetooth включен. Все отлично.");
}else
{
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT);
}
}else
{
MyError("Fatal Error", "Bluetooth ОТСУТСТВУЕТ");
}
b1 = (Button) findViewById(R.id.b1);
b2 = (Button) findViewById(R.id.b2);
b1.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
MyThred.sendData("0");
mytext.setText("Отправлены данные: 0");
}
});
b2.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
MyThred.sendData("1");
mytext.setText("Отправлены данные: 1");
}
});
}
@Override
public void onResume() {
super.onResume();
BluetoothDevice device = btAdapter.getRemoteDevice(MacAddress);
Log.d(LOG_TAG, "***Получили удаленный Device***"+device.getName());
try {
btSocket = device.createRfcommSocketToServiceRecord(MY_UUID);
Log.d(LOG_TAG, "...Создали сокет...");
} catch (IOException e) {
MyError("Fatal Error", "В onResume() Не могу создать сокет: " + e.getMessage() + ".");
}
btAdapter.cancelDiscovery();
Log.d(LOG_TAG, "***Отменили поиск других устройств***");
Log.d(LOG_TAG, "***Соединяемся...***");
try {
btSocket.connect();
Log.d(LOG_TAG, "***Соединение успешно установлено***");
} catch (IOException e) {
try {
btSocket.close();
} catch (IOException e2) {
MyError("Fatal Error", "В onResume() не могу закрыть сокет" + e2.getMessage() + ".");
}
}
MyThred = new ConnectedThred(btSocket);
}
@Override
public void onPause() {
super.onPause();
Log.d(LOG_TAG, "...In onPause()...");
if (MyThred.status_OutStrem() != null) {
MyThred.cancel();
}
try {
btSocket.close();
} catch (IOException e2) {
MyError("Fatal Error", "В onPause() Не могу закрыть сокет" + e2.getMessage() + ".");
}
}
private void MyError(String title, String message){
Toast.makeText(getBaseContext(), title + " - " + message, Toast.LENGTH_LONG).show();
finish();
}
//Отдельный поток для передачи данных
private class ConnectedThred extends Thread{
private final BluetoothSocket copyBtSocket;
private final OutputStream OutStrem;
public ConnectedThred(BluetoothSocket socket){
copyBtSocket = socket;
OutputStream tmpOut = null;
try{
tmpOut = socket.getOutputStream();
} catch (IOException e){}
OutStrem = tmpOut;
}
public void sendData(String message) {
byte[] msgBuffer = message.getBytes();
Log.d(LOG_TAG, "***Отправляем данные: " + message + "***" );
try {
OutStrem.write(msgBuffer);
} catch (IOException e) {}
}
public void cancel(){
try {
copyBtSocket.close();
}catch(IOException e){}
}
public Object status_OutStrem(){
if (OutStrem == null){return null;
}else{return OutStrem;}
}
}
}
The application we wrote allows you to transfer data via BT from the android to the arduino - “0” and “1”, which in turn are for the arduino commands “1” - to light the LED, and “0” - to put out. Thus, taking into account the general scheme of the robot, we learned to give commands from the “brain” to the “muscles”. It remains the case for small, to teach the android to accept data from the "senses."
Android STEP 4
Receiving data as well as sending, must be performed in a separate thread in order to avoid hanging of the main activity. We will display the received data from the BT module on the screen of the main activity in the text field - MyText. But the difficulty arises - working with view components is available only from the main stream. And the new threads that we create do not have access to the screen elements. To solve this problem, use the Handler mechanism.
Handler is a mechanism that allows you to work with a message queue. It is attached to a specific thread (thread) and works with its queue. Handler can queue messages. At the same time, he puts himself as the recipient of this message. And when the time comes, the system removes the message from the queue and sends it to the addressee (i.e. in Handler) for processing.
Declare Handler:
Handler h;
Create your Handler:
h = new Handler() {
public void handleMessage(android.os.Message msg) {
switch (msg.what) {
case ArduinoData:
byte[] readBuf = (byte[]) msg.obj;
String strIncom = new String(readBuf, 0, msg.arg1);
mytext.setText("Данные от Arduino: " + strIncom);
break;
}
};
};
we implement the handleMessage message processing method in it. We extract the what, obj attribute and arguments of type int from the message. We transform the received message into a string and display it in the text field of the main activity: mytext.setText ("Data from Arduino:" + strIncom);
In the stream for data transfer, add the function to start this stream, and place a cycle with the function for reading data there:
public void run()
{
byte[] buffer = new byte[1024];
int bytes;
while(true){
try{
bytes = InStrem.read(buffer);
h.obtainMessage(ArduinoData, bytes, -1, buffer).sendToTarget();
}catch(IOException e){break;}
}
}
The full application code is given below:
package com.robot.bluetoothrob2;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.Socket;
import java.util.UUID;
import com.robot.bluetoothrob2.R;
import android.os.Bundle;
import android.os.Handler;
import android.app.Activity;
import android.util.Log;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.TextView;
import android.widget.Toast;
import android.bluetooth.*;
import android.content.Intent;
public class MainActivity extends Activity {
private static final int REQUEST_ENABLE_BT = 1;
final int ArduinoData = 1;
final String LOG_TAG = "myLogs";
private BluetoothAdapter btAdapter = null;
private BluetoothSocket btSocket = null;
private static String MacAddress = "20:11:02:47:01:60"; // MAC-адрес БТ модуля
private static final UUID MY_UUID = UUID.fromString("00001101-0000-1000-8000-00805F9B34FB");
private ConnectedThred MyThred = null;
public TextView mytext;
Button b1, b2;
Handler h;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
btAdapter = BluetoothAdapter.getDefaultAdapter();
mytext = (TextView) findViewById(R.id.txtrobot);
if (btAdapter != null){
if (btAdapter.isEnabled()){
mytext.setText("Bluetooth включен. Все отлично.");
}else
{
Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT);
}
}else
{
MyError("Fatal Error", "Bluetooth ОТСУТСТВУЕТ");
}
b1 = (Button) findViewById(R.id.b1);
b2 = (Button) findViewById(R.id.b2);
b1.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
MyThred.sendData("0");
//mytext.setText("Отправлены данные: 0");
}
});
b2.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
MyThred.sendData("1");
// mytext.setText("Отправлены данные: 1");
}
});
h = new Handler() {
public void handleMessage(android.os.Message msg) {
switch (msg.what) {
case ArduinoData:
byte[] readBuf = (byte[]) msg.obj;
String strIncom = new String(readBuf, 0, msg.arg1);
mytext.setText("Данные от Arduino: " + strIncom);
break;
}
};
};
}
@Override
public void onResume() {
super.onResume();
BluetoothDevice device = btAdapter.getRemoteDevice(MacAddress);
Log.d(LOG_TAG, "***Получили удаленный Device***"+device.getName());
try {
btSocket = device.createRfcommSocketToServiceRecord(MY_UUID);
Log.d(LOG_TAG, "...Создали сокет...");
} catch (IOException e) {
MyError("Fatal Error", "В onResume() Не могу создать сокет: " + e.getMessage() + ".");
}
btAdapter.cancelDiscovery();
Log.d(LOG_TAG, "***Отменили поиск других устройств***");
Log.d(LOG_TAG, "***Соединяемся...***");
try {
btSocket.connect();
Log.d(LOG_TAG, "***Соединение успешно установлено***");
} catch (IOException e) {
try {
btSocket.close();
} catch (IOException e2) {
MyError("Fatal Error", "В onResume() не могу закрыть сокет" + e2.getMessage() + ".");
}
}
MyThred = new ConnectedThred(btSocket);
MyThred.start();
}
@Override
public void onPause() {
super.onPause();
Log.d(LOG_TAG, "...In onPause()...");
if (MyThred.status_OutStrem() != null) {
MyThred.cancel();
}
try {
btSocket.close();
} catch (IOException e2) {
MyError("Fatal Error", "В onPause() Не могу закрыть сокет" + e2.getMessage() + ".");
}
}
private void MyError(String title, String message){
Toast.makeText(getBaseContext(), title + " - " + message, Toast.LENGTH_LONG).show();
finish();
}
//Отдельный поток для передачи данных
private class ConnectedThred extends Thread{
private final BluetoothSocket copyBtSocket;
private final OutputStream OutStrem;
private final InputStream InStrem;
public ConnectedThred(BluetoothSocket socket){
copyBtSocket = socket;
OutputStream tmpOut = null;
InputStream tmpIn = null;
try{
tmpOut = socket.getOutputStream();
tmpIn = socket.getInputStream();
} catch (IOException e){}
OutStrem = tmpOut;
InStrem = tmpIn;
}
public void run()
{
byte[] buffer = new byte[1024];
int bytes;
while(true){
try{
bytes = InStrem.read(buffer);
h.obtainMessage(ArduinoData, bytes, -1, buffer).sendToTarget();
}catch(IOException e){break;}
}
}
public void sendData(String message) {
byte[] msgBuffer = message.getBytes();
Log.d(LOG_TAG, "***Отправляем данные: " + message + "***" );
try {
OutStrem.write(msgBuffer);
} catch (IOException e) {}
}
public void cancel(){
try {
copyBtSocket.close();
}catch(IOException e){}
}
public Object status_OutStrem(){
if (OutStrem == null){return null;
}else{return OutStrem;}
}
}
}
In step 4, an application is presented that allows you to transmit commands via the BT from the android to the arduino, as well as receive data from the arduino. Thus, we learned to give commands and receive data, and therefore completed the task.
In addition, this application is a template, on its basis you can create more complex applications, which in turn will be able to process data from an ultrasonic sensor, for example, and also give commands to motors to move the robot.
For the next article, I ordered new parts and modules. Below is a list and prices:
Name | Link | Price ye | Price, rub | Qty | Amount |
Layout | dx.com/p/solderless-breadboard-with-400-tie-point-white-121534 | 3,1 | 102.3 | 2 | 204.6 |
Ultrasonic sensor | dx.com/p/ultrasonic-sensor-distance-measuring-module-138563 | 3.9 | 128.7 | 3 | 386.1 |
Driver for motor 2 pcs. | dx.com/en/p/hg7881-two-channel-motor-driver-board-blue-green-black-2-5-12v-215795 | 2,8 | 92.4 | 2 | 184.8 |
Platform | dx.com/en/p/zl-4-smart-car-chassis-kit-for-arduino-black-yellow-152992 | 28,2 | 930.6 | 1 | 930.6 |
Connection wiring | dx.com/p/breadboard-jumper-wire-cord-kit-for-arduino-diy-140-piece-pack-138220 | 6.9 | 227.7 | 1 | 227.7 |
Nutrition | dx.com/en/p/dc-power-converter-module-for-electronic-diy-219232 | 2,3 | 75.9 | 1 | 75.9 |
TOTAL: 1933.8