
Tell-Don't-Ask
- Transfer
- Tutorial
Tell-Don't-Ask is a principle that helps you remember that object-oriented programming is designed to bundle data and functions for processing them. He reminds us that instead of asking for data from an object, we should tell the object what to do with it. To do this, all the behavior of the object must be enclosed in its methods.
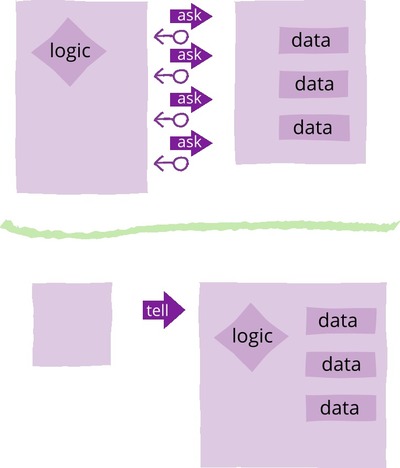
Let's look at an example. Let's imagine that we need to control the alarm, which will work if the number rises above a certain level. If we write it in the “ask” style, we get a data structure ...
... then compatible with data access methods:
And they would use it like this:
Tell-Don't-Ask reminds us that the behavior should be described inside the object (using the same fields):
And it will work like this:
Many people find the Tell-Don't-Ask principle useful. One of the fundamental principles of object-oriented design is the combination of data and behavior, so simple system elements (objects) combine this in themselves. This is often good, because data and its processing are closely related: changes in one cause changes in others. Things that are closely related should be one component. Keeping this principle in mind will help programmers see how data and behavior can be combined.
But personally, I do not use this principle. I just try to put data and behavior in one place, which often leads to the same results. One of the problems is that this principle encourages people to clean up methods that request information. But it happens when the objects cooperate effectively by providing information. A good example would be objects that simplify information for other objects. I saw code that is so twisted so that suitable requesting methods would solve this problem. For me, Tell-Don't-Ask is a step towards combining behavior and data, but this is not a priority.
Note translator: I doubt the need to translate the name of the principle, but as one of the options I propose - “ask, do not ask”
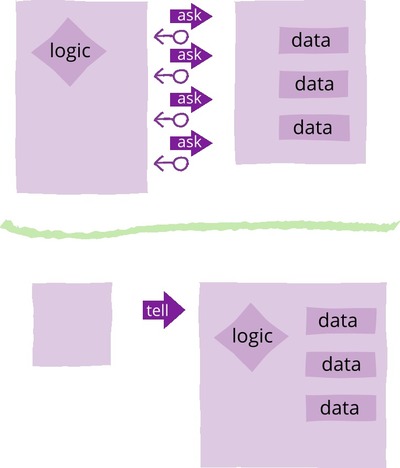
Let's look at an example. Let's imagine that we need to control the alarm, which will work if the number rises above a certain level. If we write it in the “ask” style, we get a data structure ...
class AskMonitor...
private int value;
private int limit;
private boolean isTooHigh;
private String name;
private Alarm alarm;
public AskMonitor (String name, int limit, Alarm alarm) {
this.name = name;
this.limit = limit;
this.alarm = alarm;
}
... then compatible with data access methods:
class AskMonitor...
public int getValue() {return value;}
public void setValue(int arg) {value = arg;}
public int getLimit() {return limit;}
public String getName() {return name;}
public Alarm getAlarm() {return alarm;}
And they would use it like this:
AskMonitor am = new AskMonitor("Time Vortex Hocus", 2, alarm);
am.setValue(3);
if (am.getValue() > am.getLimit())
am.getAlarm().warn(am.getName() + " too high");
Tell-Don't-Ask reminds us that the behavior should be described inside the object (using the same fields):
class TellMonitor...
public void setValue(int arg) {
value = arg;
if (value > limit) alarm.warn(name + " too high");
}
And it will work like this:
TellMonitor tm = new TellMonitor("Time Vortex Hocus", 2, alarm);
tm.setValue(3);
Many people find the Tell-Don't-Ask principle useful. One of the fundamental principles of object-oriented design is the combination of data and behavior, so simple system elements (objects) combine this in themselves. This is often good, because data and its processing are closely related: changes in one cause changes in others. Things that are closely related should be one component. Keeping this principle in mind will help programmers see how data and behavior can be combined.
But personally, I do not use this principle. I just try to put data and behavior in one place, which often leads to the same results. One of the problems is that this principle encourages people to clean up methods that request information. But it happens when the objects cooperate effectively by providing information. A good example would be objects that simplify information for other objects. I saw code that is so twisted so that suitable requesting methods would solve this problem. For me, Tell-Don't-Ask is a step towards combining behavior and data, but this is not a priority.
Note translator: I doubt the need to translate the name of the principle, but as one of the options I propose - “ask, do not ask”
Only registered users can participate in the survey. Please come in.
Do you follow the Tell Don't Ask principle?
- 33.4% Yes 74
- 19% No 42
- 21.2% Adhere to the position of Martin Fowler 47
- 23.5% I still do not understand the meaning of principle 52
- 2.7% Own, in the comments 6