What is Node.js really?
- Transfer

Node.js is a JavaScript runtime. What does this mean, and how does it work?
The Node.js environment includes everything you need to run a program written in JavaScript.

Previously, you could run JavaScript only in a browser, but once the developers expanded it, and now you can run JS on your computer as a separate application. This is how Node.js appeared.
Now you can do much more with JavaScript than just interactive websites.
Now JavaScript has the ability to do what other scripting programming languages, such as Python, can do.
Both browser javascript and Node.js run in the V8 runtime. This engine uses your JS code, and converts it into faster machine code. Machine - low-level code that a computer can run without having to first interpret it.
Why Node.js?
Here is the formal definition given on the official Node.js website:
- Node.js - JavaScript runtime based on the V8 JavaScript engine from Chrome.
- Node.js uses an event-driven, non-blocking I / O model, which makes it easy and efficient.
“The Node.js package ecosystem, npm, is the largest open source library ecosystem in the world.
We have already discussed the first line of this definition: "Node.js is a JavaScript runtime based on the V8 JavaScript engine from Chrome." Now let's understand the other two lines, so we can understand why Node.js is so popular.
I / O means input / output. This can be anything: from reading / writing local files to an HTTP request to the API. I / O takes time and therefore blocks other functions.
Consider a scenario in which we request user1 and user2 from the backend, and then print them on the screen / console. The response to this request takes time, but both user data requests can be executed independently and at the same time.

I / O Blocking
In the blocking method, the user2 data query does not start until the user1 data is printed on the screen.
If it was a web server, we would need to start a new thread for each new user. But JavaScript is single-threaded (but it has a single-threaded event loop, which we'll talk about later). Thus, it will make JavaScript not very suitable for multithreaded tasks.
Non-blocking I / O
On the other hand, using a non-blocking request, you can initiate a data request for user2, without waiting for a response from user1. You can initiate both requests in parallel.
Non-blocking I / O eliminates the need for multithreading, since the server can handle multiple requests at the same time.
JavaScript event loop
If you have 26 minutes, watch the excellent video explanation of the Node Event Loop:
If not, here is a step-by-step explanation of how the Event Loop works in JavaScript:
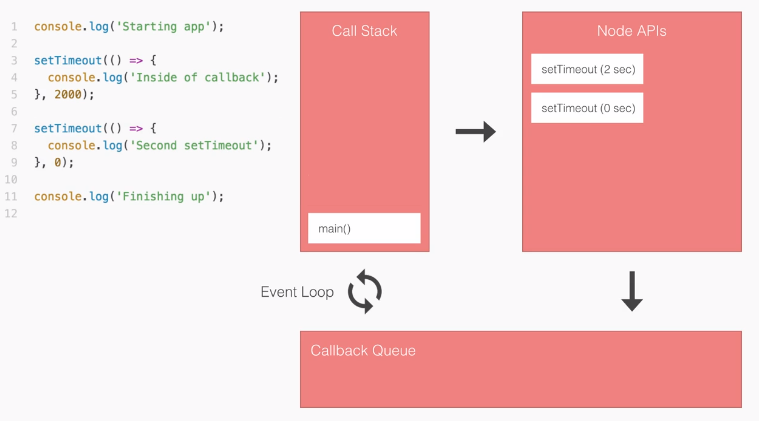
1. Send main () to the call stack.
2. Send console.log () to the call stack. It starts immediately and appears.
3. Send setTimeout (2000) to the stack. setTimeout (2000) is the Node API. When we call it, we register a callback event pair. The event will wait 2000 milliseconds, and then it will call a callback.
4. After registration, setTimeout (2000) appears on the call stack.
5. Now the second setTimeout (0) is registered in the same way. We now have two pending Node APIs.
6. After waiting for 0 seconds, setTimeout (0) moves to the callback queue, and the same happens with setTimeout (2000).
7. In the queue for performing callbacks, functions are waited for the call stack to be empty, because only one function can be executed at a time. This provides an event loop.
8. The last console.log () is called, and main () is called from the call stack.
9. The event loop sees that the call stack is empty, and the callback queue is not. Thus, it moves the callbacks (in order) to the call stack for execution.
Npm
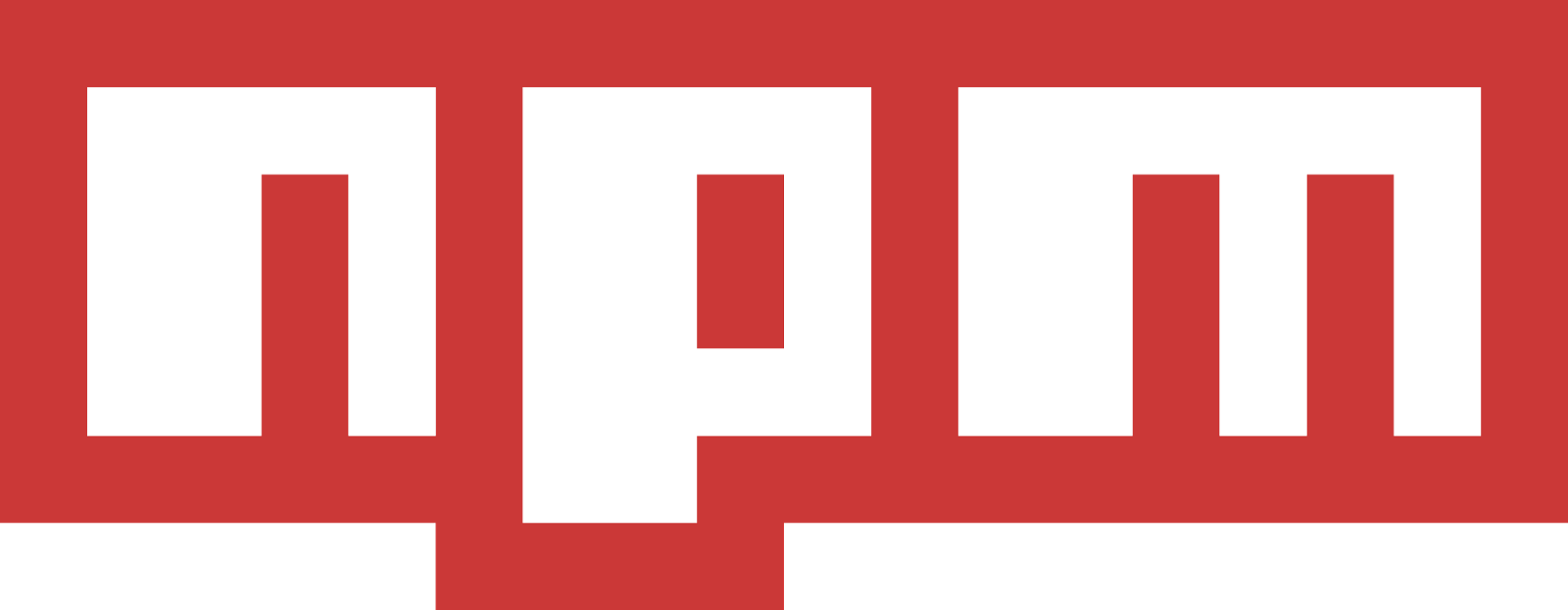
These are libraries built by the community. They will solve most common problems. npm (Node Package Manager) contains packages that you can use in your applications to make your development faster and more efficient.
Require
Require performs three functions: It
loads modules that come bundled with Node.js, for example, from the file system or HTTP, from the Node.js API.
Loads third-party libraries, such as Express and Mongoose, which you install from npm.
Allows you to create your own files and divide the project into modules.
Require is a function, and it takes the path parameter and returns module.exports.
Node modules
Node modules are reusable code blocks, the existence of which does not accidentally affect other code.
You can write your own modules and use them in various applications. Node.js has a set of built-in modules that you can use without special installation.
V8 speeds up javascript using C ++
V8 is an open source engine written in C ++.
JavaScript -> V8 (C ++) ->
V8 native code implements an ECMAScript script, as specified in ECMA-262. ECMAScript was created by Ecma International to standardize JavaScript.
V8 can work autonomously or can be embedded in any C ++ application. Because of this, you can write your own C ++ code, and make it available for JavaScript.
Developments
Events are all that happened in our application and to which we can respond.
There are two types of events in Node:
- System events: C ++ core from libuv library. (For example, the end of the reading file).
- Custom events: core JavaScript.
Writing Hello World in Node.js
Why not?
Create an app.js file and add the following to it.
console.log ("Hello World!");
Open a Node terminal, change the directory to the folder where the file is saved, and run node app.js.
Voila - you just wrote “Hello World” in Node.js.
There are a wealth of resources you can use to learn more about Node.js, including freeCodeCamp.org.