
Hiding the process in the Windows task manager
Intro
Often anonymity and secrecy play a key role in the successful execution of any action, both in reality and in virtuality, in particular in operating systems. This article will talk about how to become anonymous in OS Windows. All information is provided for informational purposes only.
So, we will try to hide from the user's eyes in the Windows task manager. The way in which we will achieve this is extremely simple with respect to those that are based on intercepting nuclear (often undocumented) functions and creating your own drivers.
The essence of the method: search for the Task Manager window -> search in it for a child window (list) containing the names of all processes -> remove our process from the list.
As you can see, there will be no manipulations with our process: it worked as it will and will work for itself. Since a standard ordinary Windows user, as a rule, does not use any other tools to view running processes on his computer, this will only play into our hands. The process in most cases will not be detected.
What was used for research:
1) Spy ++ from Microsoft (to study the hierarchy of child windows of the Task Manager)
2) OllyDBG to view the functions used by the manager to obtain a snapshot of processes.
3) Actually, taskmng.exe itself (Task Manager)
We will use the Delphi environment to write code. Rather, Delphi will be more convenient in our case than C ++. But this is just my
Well, first of all, let's try to find out what a list of processes is and how it works. From a half-view, it’s clear that this is a regular SysListView32 class window (list), which is updated at a frequency of 2 frames per second (once every 0.5 seconds). We look at the hierarchy of windows:

As you can see, the list of processes, in fact, is a regular window of the "SysListView32" class, which is a child of the "Processes" window (tab), which is also a child of the main window of the Task Manager. We have only a double level of nesting. In addition, the list has one child window of the SysHeader32 class, which, as you might guess, is the heading (field marker) for the list of processes.
Since we have a regular list, we have at our disposal a whole set of macros to manage its contents.Their diversity, at first glance, is amazing. But many of them work only from the parent process, that is, in order for us to use them, it will be necessary to imitate that they are executed in the parent process. But not everyone has this property, in particular, the ListView_DeleteItem macro , which removes an item from the list box (class "SysListView32").
We will use it in the process of our application. This function receives the index of the deleted item as the second parameter.
Now we need to find out somehow what index the element with the label of the hidden process in the task manager has. To do this, we need to somehow remove from the list of processes in the task manager all the elements (labels with the names of the processes) and sequentially compare them with the name of the process that we want to hide.
Using macros like ListView_GetItemText, our actions would be something like this:
1) Allocating a portion of memory during the task manager ( VirtualAllocEx )
2) Sending the LVM_GETITEMTEXT ( SendMessage ) message to the child list of the Task Manager
3) Writing information about the list item ( WriteProcessMemory ) to the allocated memory area of the Task Manager
4) Reading that information from the memory of the manager that interests us about the process ( ReadProcessMemory )
Using this method, you can easily "shoot yourself in the foot" by counting the offset bytes from the beginning of the various structures used in the code. Also, this method will be quite difficult for those who are not particularly deep in WinAPI, so we will immediately remove it to the side. In other matters, finding an implementation of this method on the Internet will not be difficult. Instead, I suggest that you create your own list of processes, and already guided by it, look for the coveted process index in the list of tasks of the Task Manager.
Microsoft decided not to worry about the tool called the Task Manager, and used the usual WinAPI functions to get all the processes in the system. Superficially look at taskmng.exe under the debugger:
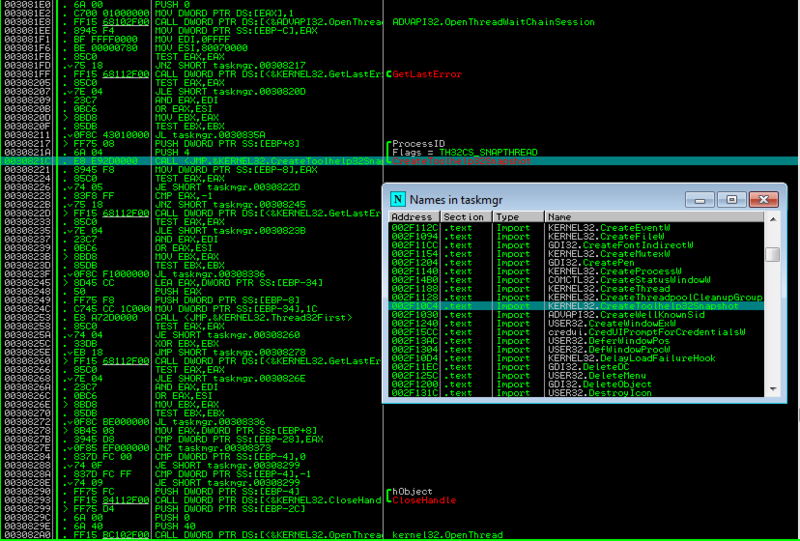
We see the use of the WinAPI function CreateToolHelp32SnapShot.
Everyone knows that this function can be used not only to obtain a snapshot of processes, but also threads of a process or modules, for example. But in this case, this is unlikely. It is unlikely that they will use something in the form of a process enumerator ( EnumProcesses ).
We settled on the fact that we want to create our list of processes and look for our process in it. To do this, use the function that was found in the debugger. If we open the task manager on the “Processes” tab, we note that all processes are sorted alphabetically for easy search. Therefore, we need to get a list of the names of all processes in the system and sort them in ascending order in alphabetical order . Let's start writing code in Delphi.
First, create a demo window application with two timers: the first will re-process the list with processes at the same frequency as the Windows Task Manager (once every two seconds); the second one will fire 1000 times per second and will serve to track updates to the list of processes in the manager and, therefore, the appearance of our hidden process. Also add a button to the form.
The code:
var
ind:integer;
h:Thandle;
last_c:integer;
procedure UpdateList();
var
th:THandle;
entry:PROCESSENTRY32;
b:boolean;
i,new_ind:integer;
plist:TStringList;
begin
// Список процессов
plist:=TStringList.Create;
// Формируем список процессов
th := CreateToolHelp32SnapShot(TH32CS_SNAPPROCESS,0);
entry.dwSize:=sizeof(PROCESSENTRY32);
b:=Process32First(th,entry);
while(b) do
begin
plist.Add(entry.szExeFile);
b:=Process32Next(th,entry);
end;
// Сортируем его, чтобы индексы элементов
// совпадали с теми, что в диспетчере задач
plist.Sort;
last_c:=plist.Count;
// Поиск индекса нашего процесса 'explorer.exe'
for i:=1 to plist.Count-1 do
if(LowerCase(plist[i])='explorer.exe') then new_ind:=i-1;
// Удаление объекта из списка
if(new_ind<>ind) then ListView_DeleteItem(h,ind);
ind:=new_ind;
plist.Free;
// Запускаем таймер отслеживания обновлений в списке процессов
if(Form1.Timer2.Enabled=false) then Form1.Timer2.Enabled:=true;
end;
procedure TForm1.HideProcessButton(Sender: TObject);
begin
// Ищем дочернее окно класса 'SysListView32'
h:=FindWindow(nil,'Диспетчер задач Windows');
h:=FindWindowEx(h,0,nil,'Процессы');
h:=FindWindowEx(h,0,'SysListView32',nil);
// Запускаем таймер переформирования списка процессов
Timer1.Enabled:=true;
end;
procedure TForm1.Timer1Timer(Sender: TObject);
begin
UpdateList();
end;
procedure TForm1.Timer2Timer(Sender: TObject);
begin
// Поиск изменений в списке
if(ListView_GetItemCount(h)>last_c) then
ListView_DeleteItem(h,ind);
last_c:=ListView_GetItemCount(h);
end;
Here, in fact, is the whole code.
For example, we will hide the process of the Task Manager itself in the Task Manager:
Here it is:

And by clicking on the “Hide process” button, the process disappears from the list:
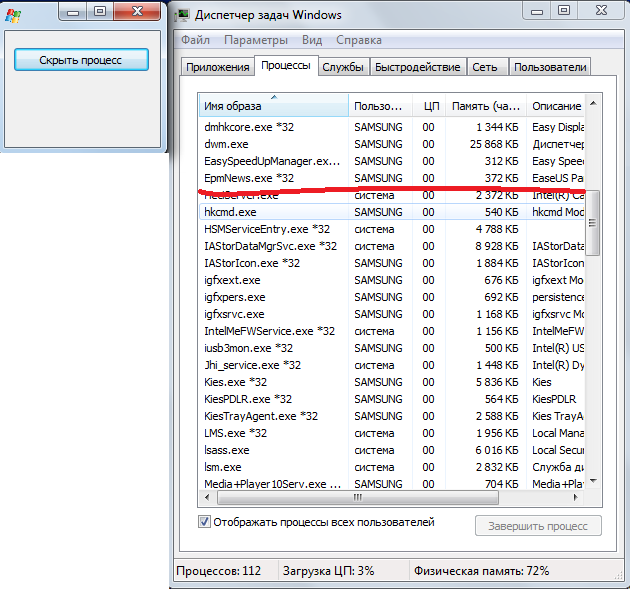
All traces of the presence in the system are erased, and it quietly runs in the normal mode somewhere in the depths processor :)
Outro
Well, I think this way deserves to exist, although it does require minor modifications. Yes, of course, you cannot use it to hide the process from the system itself, but hiding in the standard Windows tool, which is used by the lion's share of all users, is also good.
I hope I managed to interest you in this topic at least a little bit.
See you later! And may the power of anonymity be with you ...